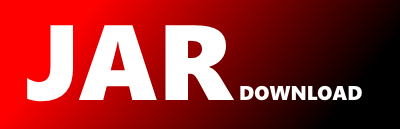
com.pulumi.aws.securitylake.kotlin.inputs.SubscriberNotificationConfigurationHttpsNotificationConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.securitylake.kotlin.inputs
import com.pulumi.aws.securitylake.inputs.SubscriberNotificationConfigurationHttpsNotificationConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property authorizationApiKeyName The API key name for the notification subscription.
* @property authorizationApiKeyValue The API key value for the notification subscription.
* @property endpoint The subscription endpoint in Security Lake.
* If you prefer notification with an HTTPS endpoint, populate this field.
* @property httpMethod The HTTP method used for the notification subscription.
* Valid values are `POST` and `PUT`.
* @property targetRoleArn The Amazon Resource Name (ARN) of the EventBridge API destinations IAM role that you created.
* For more information about ARNs and how to use them in policies, see Managing data access and AWS Managed Policies in the Amazon Security Lake User Guide.
*/
public data class SubscriberNotificationConfigurationHttpsNotificationConfigurationArgs(
public val authorizationApiKeyName: Output? = null,
public val authorizationApiKeyValue: Output? = null,
public val endpoint: Output,
public val httpMethod: Output? = null,
public val targetRoleArn: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.securitylake.inputs.SubscriberNotificationConfigurationHttpsNotificationConfigurationArgs =
com.pulumi.aws.securitylake.inputs.SubscriberNotificationConfigurationHttpsNotificationConfigurationArgs.builder()
.authorizationApiKeyName(authorizationApiKeyName?.applyValue({ args0 -> args0 }))
.authorizationApiKeyValue(authorizationApiKeyValue?.applyValue({ args0 -> args0 }))
.endpoint(endpoint.applyValue({ args0 -> args0 }))
.httpMethod(httpMethod?.applyValue({ args0 -> args0 }))
.targetRoleArn(targetRoleArn.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SubscriberNotificationConfigurationHttpsNotificationConfigurationArgs].
*/
@PulumiTagMarker
public class SubscriberNotificationConfigurationHttpsNotificationConfigurationArgsBuilder internal constructor() {
private var authorizationApiKeyName: Output? = null
private var authorizationApiKeyValue: Output? = null
private var endpoint: Output? = null
private var httpMethod: Output? = null
private var targetRoleArn: Output? = null
/**
* @param value The API key name for the notification subscription.
*/
@JvmName("ugvpaqroabfkmcqm")
public suspend fun authorizationApiKeyName(`value`: Output) {
this.authorizationApiKeyName = value
}
/**
* @param value The API key value for the notification subscription.
*/
@JvmName("etftdvsdqrbbpvdj")
public suspend fun authorizationApiKeyValue(`value`: Output) {
this.authorizationApiKeyValue = value
}
/**
* @param value The subscription endpoint in Security Lake.
* If you prefer notification with an HTTPS endpoint, populate this field.
*/
@JvmName("ibtfdavvmlbrnwcn")
public suspend fun endpoint(`value`: Output) {
this.endpoint = value
}
/**
* @param value The HTTP method used for the notification subscription.
* Valid values are `POST` and `PUT`.
*/
@JvmName("tgeuupdjtsihilsj")
public suspend fun httpMethod(`value`: Output) {
this.httpMethod = value
}
/**
* @param value The Amazon Resource Name (ARN) of the EventBridge API destinations IAM role that you created.
* For more information about ARNs and how to use them in policies, see Managing data access and AWS Managed Policies in the Amazon Security Lake User Guide.
*/
@JvmName("vqvxsgpsvpipfbad")
public suspend fun targetRoleArn(`value`: Output) {
this.targetRoleArn = value
}
/**
* @param value The API key name for the notification subscription.
*/
@JvmName("swxhvkjdhxepcnoc")
public suspend fun authorizationApiKeyName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authorizationApiKeyName = mapped
}
/**
* @param value The API key value for the notification subscription.
*/
@JvmName("kckmheljcumymjjs")
public suspend fun authorizationApiKeyValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authorizationApiKeyValue = mapped
}
/**
* @param value The subscription endpoint in Security Lake.
* If you prefer notification with an HTTPS endpoint, populate this field.
*/
@JvmName("eddamddqrginpyir")
public suspend fun endpoint(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.endpoint = mapped
}
/**
* @param value The HTTP method used for the notification subscription.
* Valid values are `POST` and `PUT`.
*/
@JvmName("wvuidojpqaxjlaxx")
public suspend fun httpMethod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.httpMethod = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of the EventBridge API destinations IAM role that you created.
* For more information about ARNs and how to use them in policies, see Managing data access and AWS Managed Policies in the Amazon Security Lake User Guide.
*/
@JvmName("qtlwxusjkvkomkqe")
public suspend fun targetRoleArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.targetRoleArn = mapped
}
internal fun build(): SubscriberNotificationConfigurationHttpsNotificationConfigurationArgs =
SubscriberNotificationConfigurationHttpsNotificationConfigurationArgs(
authorizationApiKeyName = authorizationApiKeyName,
authorizationApiKeyValue = authorizationApiKeyValue,
endpoint = endpoint ?: throw PulumiNullFieldException("endpoint"),
httpMethod = httpMethod,
targetRoleArn = targetRoleArn ?: throw PulumiNullFieldException("targetRoleArn"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy