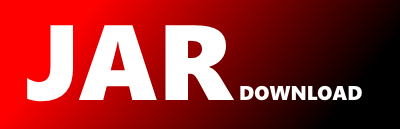
com.pulumi.aws.servicequotas.kotlin.ServiceQuota.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.servicequotas.kotlin
import com.pulumi.aws.servicequotas.kotlin.outputs.ServiceQuotaUsageMetric
import com.pulumi.aws.servicequotas.kotlin.outputs.ServiceQuotaUsageMetric.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [ServiceQuota].
*/
@PulumiTagMarker
public class ServiceQuotaResourceBuilder internal constructor() {
public var name: String? = null
public var args: ServiceQuotaArgs = ServiceQuotaArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ServiceQuotaArgsBuilder.() -> Unit) {
val builder = ServiceQuotaArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ServiceQuota {
val builtJavaResource = com.pulumi.aws.servicequotas.ServiceQuota(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ServiceQuota(builtJavaResource)
}
}
/**
* Manages an individual Service Quota.
* > **NOTE:** Global quotas apply to all AWS regions, but can only be accessed in `us-east-1` in the Commercial partition or `us-gov-west-1` in the GovCloud partition. In other regions, the AWS API will return the error `The request failed because the specified service does not exist.`
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.servicequotas.ServiceQuota("example", {
* quotaCode: "L-F678F1CE",
* serviceCode: "vpc",
* value: 75,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.servicequotas.ServiceQuota("example",
* quota_code="L-F678F1CE",
* service_code="vpc",
* value=75)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.ServiceQuotas.ServiceQuota("example", new()
* {
* QuotaCode = "L-F678F1CE",
* ServiceCode = "vpc",
* Value = 75,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/servicequotas"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := servicequotas.NewServiceQuota(ctx, "example", &servicequotas.ServiceQuotaArgs{
* QuotaCode: pulumi.String("L-F678F1CE"),
* ServiceCode: pulumi.String("vpc"),
* Value: pulumi.Float64(75),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.servicequotas.ServiceQuota;
* import com.pulumi.aws.servicequotas.ServiceQuotaArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ServiceQuota("example", ServiceQuotaArgs.builder()
* .quotaCode("L-F678F1CE")
* .serviceCode("vpc")
* .value(75)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:servicequotas:ServiceQuota
* properties:
* quotaCode: L-F678F1CE
* serviceCode: vpc
* value: 75
* ```
*
* ## Import
* Using `pulumi import`, import `aws_servicequotas_service_quota` using the service code and quota code, separated by a front slash (`/`). For example:
* ~> __NOTE:__ This resource does not require explicit import and will assume management of an existing service quota on Pulumi resource creation.
* ```sh
* $ pulumi import aws:servicequotas/serviceQuota:ServiceQuota example vpc/L-F678F1CE
* ```
*/
public class ServiceQuota internal constructor(
override val javaResource: com.pulumi.aws.servicequotas.ServiceQuota,
) : KotlinCustomResource(javaResource, ServiceQuotaMapper) {
/**
* Whether the service quota can be increased.
*/
public val adjustable: Output
get() = javaResource.adjustable().applyValue({ args0 -> args0 })
/**
* Amazon Resource Name (ARN) of the service quota.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Default value of the service quota.
*/
public val defaultValue: Output
get() = javaResource.defaultValue().applyValue({ args0 -> args0 })
/**
* Code of the service quota to track. For example: `L-F678F1CE`. Available values can be found with the [AWS CLI service-quotas list-service-quotas command](https://docs.aws.amazon.com/cli/latest/reference/service-quotas/list-service-quotas.html).
*/
public val quotaCode: Output
get() = javaResource.quotaCode().applyValue({ args0 -> args0 })
/**
* Name of the quota.
*/
public val quotaName: Output
get() = javaResource.quotaName().applyValue({ args0 -> args0 })
public val requestId: Output
get() = javaResource.requestId().applyValue({ args0 -> args0 })
public val requestStatus: Output
get() = javaResource.requestStatus().applyValue({ args0 -> args0 })
/**
* Code of the service to track. For example: `vpc`. Available values can be found with the [AWS CLI service-quotas list-services command](https://docs.aws.amazon.com/cli/latest/reference/service-quotas/list-services.html).
*/
public val serviceCode: Output
get() = javaResource.serviceCode().applyValue({ args0 -> args0 })
/**
* Name of the service.
*/
public val serviceName: Output
get() = javaResource.serviceName().applyValue({ args0 -> args0 })
/**
* Information about the measurement.
*/
public val usageMetrics: Output>
get() = javaResource.usageMetrics().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
toKotlin(args0)
})
})
})
/**
* Float specifying the desired value for the service quota. If the desired value is higher than the current value, a quota increase request is submitted. When a known request is submitted and pending, the value reflects the desired value of the pending request.
*/
public val `value`: Output
get() = javaResource.`value`().applyValue({ args0 -> args0 })
}
public object ServiceQuotaMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.aws.servicequotas.ServiceQuota::class == javaResource::class
override fun map(javaResource: Resource): ServiceQuota = ServiceQuota(
javaResource as
com.pulumi.aws.servicequotas.ServiceQuota,
)
}
/**
* @see [ServiceQuota].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ServiceQuota].
*/
public suspend fun serviceQuota(
name: String,
block: suspend ServiceQuotaResourceBuilder.() -> Unit,
): ServiceQuota {
val builder = ServiceQuotaResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ServiceQuota].
* @param name The _unique_ name of the resulting resource.
*/
public fun serviceQuota(name: String): ServiceQuota {
val builder = ServiceQuotaResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy