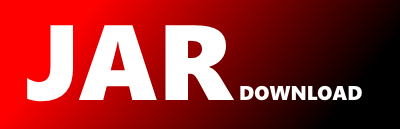
com.pulumi.aws.ses.kotlin.DomainIdentityVerificationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ses.kotlin
import com.pulumi.aws.ses.DomainIdentityVerificationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Represents a successful verification of an SES domain identity.
* Most commonly, this resource is used together with `aws.route53.Record` and
* `aws.ses.DomainIdentity` to request an SES domain identity,
* deploy the required DNS verification records, and wait for verification to complete.
* > **WARNING:** This resource implements a part of the verification workflow. It does not represent a real-world entity in AWS, therefore changing or deleting this resource on its own has no immediate effect.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.ses.DomainIdentity("example", {domain: "example.com"});
* const exampleAmazonsesVerificationRecord = new aws.route53.Record("example_amazonses_verification_record", {
* zoneId: exampleAwsRoute53Zone.zoneId,
* name: pulumi.interpolate`_amazonses.${example.id}`,
* type: aws.route53.RecordType.TXT,
* ttl: 600,
* records: [example.verificationToken],
* });
* const exampleVerification = new aws.ses.DomainIdentityVerification("example_verification", {domain: example.id}, {
* dependsOn: [exampleAmazonsesVerificationRecord],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.ses.DomainIdentity("example", domain="example.com")
* example_amazonses_verification_record = aws.route53.Record("example_amazonses_verification_record",
* zone_id=example_aws_route53_zone["zoneId"],
* name=example.id.apply(lambda id: f"_amazonses.{id}"),
* type=aws.route53.RecordType.TXT,
* ttl=600,
* records=[example.verification_token])
* example_verification = aws.ses.DomainIdentityVerification("example_verification", domain=example.id,
* opts = pulumi.ResourceOptions(depends_on=[example_amazonses_verification_record]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Ses.DomainIdentity("example", new()
* {
* Domain = "example.com",
* });
* var exampleAmazonsesVerificationRecord = new Aws.Route53.Record("example_amazonses_verification_record", new()
* {
* ZoneId = exampleAwsRoute53Zone.ZoneId,
* Name = example.Id.Apply(id => $"_amazonses.{id}"),
* Type = Aws.Route53.RecordType.TXT,
* Ttl = 600,
* Records = new[]
* {
* example.VerificationToken,
* },
* });
* var exampleVerification = new Aws.Ses.DomainIdentityVerification("example_verification", new()
* {
* Domain = example.Id,
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleAmazonsesVerificationRecord,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/route53"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ses"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := ses.NewDomainIdentity(ctx, "example", &ses.DomainIdentityArgs{
* Domain: pulumi.String("example.com"),
* })
* if err != nil {
* return err
* }
* exampleAmazonsesVerificationRecord, err := route53.NewRecord(ctx, "example_amazonses_verification_record", &route53.RecordArgs{
* ZoneId: pulumi.Any(exampleAwsRoute53Zone.ZoneId),
* Name: example.ID().ApplyT(func(id string) (string, error) {
* return fmt.Sprintf("_amazonses.%v", id), nil
* }).(pulumi.StringOutput),
* Type: pulumi.String(route53.RecordTypeTXT),
* Ttl: pulumi.Int(600),
* Records: pulumi.StringArray{
* example.VerificationToken,
* },
* })
* if err != nil {
* return err
* }
* _, err = ses.NewDomainIdentityVerification(ctx, "example_verification", &ses.DomainIdentityVerificationArgs{
* Domain: example.ID(),
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleAmazonsesVerificationRecord,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ses.DomainIdentity;
* import com.pulumi.aws.ses.DomainIdentityArgs;
* import com.pulumi.aws.route53.Record;
* import com.pulumi.aws.route53.RecordArgs;
* import com.pulumi.aws.ses.DomainIdentityVerification;
* import com.pulumi.aws.ses.DomainIdentityVerificationArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new DomainIdentity("example", DomainIdentityArgs.builder()
* .domain("example.com")
* .build());
* var exampleAmazonsesVerificationRecord = new Record("exampleAmazonsesVerificationRecord", RecordArgs.builder()
* .zoneId(exampleAwsRoute53Zone.zoneId())
* .name(example.id().applyValue(id -> String.format("_amazonses.%s", id)))
* .type("TXT")
* .ttl("600")
* .records(example.verificationToken())
* .build());
* var exampleVerification = new DomainIdentityVerification("exampleVerification", DomainIdentityVerificationArgs.builder()
* .domain(example.id())
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleAmazonsesVerificationRecord)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ses:DomainIdentity
* properties:
* domain: example.com
* exampleAmazonsesVerificationRecord:
* type: aws:route53:Record
* name: example_amazonses_verification_record
* properties:
* zoneId: ${exampleAwsRoute53Zone.zoneId}
* name: _amazonses.${example.id}
* type: TXT
* ttl: '600'
* records:
* - ${example.verificationToken}
* exampleVerification:
* type: aws:ses:DomainIdentityVerification
* name: example_verification
* properties:
* domain: ${example.id}
* options:
* dependson:
* - ${exampleAmazonsesVerificationRecord}
* ```
*
* @property domain The domain name of the SES domain identity to verify.
*/
public data class DomainIdentityVerificationArgs(
public val domain: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ses.DomainIdentityVerificationArgs =
com.pulumi.aws.ses.DomainIdentityVerificationArgs.builder()
.domain(domain?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DomainIdentityVerificationArgs].
*/
@PulumiTagMarker
public class DomainIdentityVerificationArgsBuilder internal constructor() {
private var domain: Output? = null
/**
* @param value The domain name of the SES domain identity to verify.
*/
@JvmName("aolvdcewcxjuvglx")
public suspend fun domain(`value`: Output) {
this.domain = value
}
/**
* @param value The domain name of the SES domain identity to verify.
*/
@JvmName("kuyyvqakhexxfort")
public suspend fun domain(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.domain = mapped
}
internal fun build(): DomainIdentityVerificationArgs = DomainIdentityVerificationArgs(
domain = domain,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy