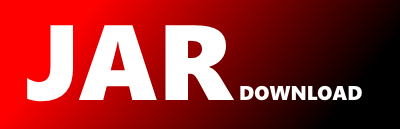
com.pulumi.aws.ses.kotlin.EventDestination.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ses.kotlin
import com.pulumi.aws.ses.kotlin.outputs.EventDestinationCloudwatchDestination
import com.pulumi.aws.ses.kotlin.outputs.EventDestinationKinesisDestination
import com.pulumi.aws.ses.kotlin.outputs.EventDestinationSnsDestination
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.aws.ses.kotlin.outputs.EventDestinationCloudwatchDestination.Companion.toKotlin as eventDestinationCloudwatchDestinationToKotlin
import com.pulumi.aws.ses.kotlin.outputs.EventDestinationKinesisDestination.Companion.toKotlin as eventDestinationKinesisDestinationToKotlin
import com.pulumi.aws.ses.kotlin.outputs.EventDestinationSnsDestination.Companion.toKotlin as eventDestinationSnsDestinationToKotlin
/**
* Builder for [EventDestination].
*/
@PulumiTagMarker
public class EventDestinationResourceBuilder internal constructor() {
public var name: String? = null
public var args: EventDestinationArgs = EventDestinationArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend EventDestinationArgsBuilder.() -> Unit) {
val builder = EventDestinationArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): EventDestination {
val builtJavaResource = com.pulumi.aws.ses.EventDestination(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return EventDestination(builtJavaResource)
}
}
/**
* Provides an SES event destination
* ## Example Usage
* ### CloudWatch Destination
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const cloudwatch = new aws.ses.EventDestination("cloudwatch", {
* name: "event-destination-cloudwatch",
* configurationSetName: example.name,
* enabled: true,
* matchingTypes: [
* "bounce",
* "send",
* ],
* cloudwatchDestinations: [{
* defaultValue: "default",
* dimensionName: "dimension",
* valueSource: "emailHeader",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* cloudwatch = aws.ses.EventDestination("cloudwatch",
* name="event-destination-cloudwatch",
* configuration_set_name=example["name"],
* enabled=True,
* matching_types=[
* "bounce",
* "send",
* ],
* cloudwatch_destinations=[{
* "default_value": "default",
* "dimension_name": "dimension",
* "value_source": "emailHeader",
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var cloudwatch = new Aws.Ses.EventDestination("cloudwatch", new()
* {
* Name = "event-destination-cloudwatch",
* ConfigurationSetName = example.Name,
* Enabled = true,
* MatchingTypes = new[]
* {
* "bounce",
* "send",
* },
* CloudwatchDestinations = new[]
* {
* new Aws.Ses.Inputs.EventDestinationCloudwatchDestinationArgs
* {
* DefaultValue = "default",
* DimensionName = "dimension",
* ValueSource = "emailHeader",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ses"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ses.NewEventDestination(ctx, "cloudwatch", &ses.EventDestinationArgs{
* Name: pulumi.String("event-destination-cloudwatch"),
* ConfigurationSetName: pulumi.Any(example.Name),
* Enabled: pulumi.Bool(true),
* MatchingTypes: pulumi.StringArray{
* pulumi.String("bounce"),
* pulumi.String("send"),
* },
* CloudwatchDestinations: ses.EventDestinationCloudwatchDestinationArray{
* &ses.EventDestinationCloudwatchDestinationArgs{
* DefaultValue: pulumi.String("default"),
* DimensionName: pulumi.String("dimension"),
* ValueSource: pulumi.String("emailHeader"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ses.EventDestination;
* import com.pulumi.aws.ses.EventDestinationArgs;
* import com.pulumi.aws.ses.inputs.EventDestinationCloudwatchDestinationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var cloudwatch = new EventDestination("cloudwatch", EventDestinationArgs.builder()
* .name("event-destination-cloudwatch")
* .configurationSetName(example.name())
* .enabled(true)
* .matchingTypes(
* "bounce",
* "send")
* .cloudwatchDestinations(EventDestinationCloudwatchDestinationArgs.builder()
* .defaultValue("default")
* .dimensionName("dimension")
* .valueSource("emailHeader")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* cloudwatch:
* type: aws:ses:EventDestination
* properties:
* name: event-destination-cloudwatch
* configurationSetName: ${example.name}
* enabled: true
* matchingTypes:
* - bounce
* - send
* cloudwatchDestinations:
* - defaultValue: default
* dimensionName: dimension
* valueSource: emailHeader
* ```
*
* ### Kinesis Destination
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const kinesis = new aws.ses.EventDestination("kinesis", {
* name: "event-destination-kinesis",
* configurationSetName: exampleAwsSesConfigurationSet.name,
* enabled: true,
* matchingTypes: [
* "bounce",
* "send",
* ],
* kinesisDestination: {
* streamArn: exampleAwsKinesisFirehoseDeliveryStream.arn,
* roleArn: example.arn,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* kinesis = aws.ses.EventDestination("kinesis",
* name="event-destination-kinesis",
* configuration_set_name=example_aws_ses_configuration_set["name"],
* enabled=True,
* matching_types=[
* "bounce",
* "send",
* ],
* kinesis_destination={
* "stream_arn": example_aws_kinesis_firehose_delivery_stream["arn"],
* "role_arn": example["arn"],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var kinesis = new Aws.Ses.EventDestination("kinesis", new()
* {
* Name = "event-destination-kinesis",
* ConfigurationSetName = exampleAwsSesConfigurationSet.Name,
* Enabled = true,
* MatchingTypes = new[]
* {
* "bounce",
* "send",
* },
* KinesisDestination = new Aws.Ses.Inputs.EventDestinationKinesisDestinationArgs
* {
* StreamArn = exampleAwsKinesisFirehoseDeliveryStream.Arn,
* RoleArn = example.Arn,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ses"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ses.NewEventDestination(ctx, "kinesis", &ses.EventDestinationArgs{
* Name: pulumi.String("event-destination-kinesis"),
* ConfigurationSetName: pulumi.Any(exampleAwsSesConfigurationSet.Name),
* Enabled: pulumi.Bool(true),
* MatchingTypes: pulumi.StringArray{
* pulumi.String("bounce"),
* pulumi.String("send"),
* },
* KinesisDestination: &ses.EventDestinationKinesisDestinationArgs{
* StreamArn: pulumi.Any(exampleAwsKinesisFirehoseDeliveryStream.Arn),
* RoleArn: pulumi.Any(example.Arn),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ses.EventDestination;
* import com.pulumi.aws.ses.EventDestinationArgs;
* import com.pulumi.aws.ses.inputs.EventDestinationKinesisDestinationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var kinesis = new EventDestination("kinesis", EventDestinationArgs.builder()
* .name("event-destination-kinesis")
* .configurationSetName(exampleAwsSesConfigurationSet.name())
* .enabled(true)
* .matchingTypes(
* "bounce",
* "send")
* .kinesisDestination(EventDestinationKinesisDestinationArgs.builder()
* .streamArn(exampleAwsKinesisFirehoseDeliveryStream.arn())
* .roleArn(example.arn())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* kinesis:
* type: aws:ses:EventDestination
* properties:
* name: event-destination-kinesis
* configurationSetName: ${exampleAwsSesConfigurationSet.name}
* enabled: true
* matchingTypes:
* - bounce
* - send
* kinesisDestination:
* streamArn: ${exampleAwsKinesisFirehoseDeliveryStream.arn}
* roleArn: ${example.arn}
* ```
*
* ### SNS Destination
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const sns = new aws.ses.EventDestination("sns", {
* name: "event-destination-sns",
* configurationSetName: exampleAwsSesConfigurationSet.name,
* enabled: true,
* matchingTypes: [
* "bounce",
* "send",
* ],
* snsDestination: {
* topicArn: example.arn,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* sns = aws.ses.EventDestination("sns",
* name="event-destination-sns",
* configuration_set_name=example_aws_ses_configuration_set["name"],
* enabled=True,
* matching_types=[
* "bounce",
* "send",
* ],
* sns_destination={
* "topic_arn": example["arn"],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var sns = new Aws.Ses.EventDestination("sns", new()
* {
* Name = "event-destination-sns",
* ConfigurationSetName = exampleAwsSesConfigurationSet.Name,
* Enabled = true,
* MatchingTypes = new[]
* {
* "bounce",
* "send",
* },
* SnsDestination = new Aws.Ses.Inputs.EventDestinationSnsDestinationArgs
* {
* TopicArn = example.Arn,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ses"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ses.NewEventDestination(ctx, "sns", &ses.EventDestinationArgs{
* Name: pulumi.String("event-destination-sns"),
* ConfigurationSetName: pulumi.Any(exampleAwsSesConfigurationSet.Name),
* Enabled: pulumi.Bool(true),
* MatchingTypes: pulumi.StringArray{
* pulumi.String("bounce"),
* pulumi.String("send"),
* },
* SnsDestination: &ses.EventDestinationSnsDestinationArgs{
* TopicArn: pulumi.Any(example.Arn),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ses.EventDestination;
* import com.pulumi.aws.ses.EventDestinationArgs;
* import com.pulumi.aws.ses.inputs.EventDestinationSnsDestinationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var sns = new EventDestination("sns", EventDestinationArgs.builder()
* .name("event-destination-sns")
* .configurationSetName(exampleAwsSesConfigurationSet.name())
* .enabled(true)
* .matchingTypes(
* "bounce",
* "send")
* .snsDestination(EventDestinationSnsDestinationArgs.builder()
* .topicArn(example.arn())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* sns:
* type: aws:ses:EventDestination
* properties:
* name: event-destination-sns
* configurationSetName: ${exampleAwsSesConfigurationSet.name}
* enabled: true
* matchingTypes:
* - bounce
* - send
* snsDestination:
* topicArn: ${example.arn}
* ```
*
* ## Import
* Using `pulumi import`, import SES event destinations using `configuration_set_name` together with the event destination's `name`. For example:
* ```sh
* $ pulumi import aws:ses/eventDestination:EventDestination sns some-configuration-set-test/event-destination-sns
* ```
*/
public class EventDestination internal constructor(
override val javaResource: com.pulumi.aws.ses.EventDestination,
) : KotlinCustomResource(javaResource, EventDestinationMapper) {
/**
* The SES event destination ARN.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* CloudWatch destination for the events
*/
public val cloudwatchDestinations: Output>?
get() = javaResource.cloudwatchDestinations().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
eventDestinationCloudwatchDestinationToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The name of the configuration set
*/
public val configurationSetName: Output
get() = javaResource.configurationSetName().applyValue({ args0 -> args0 })
/**
* If true, the event destination will be enabled
*/
public val enabled: Output?
get() = javaResource.enabled().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Send the events to a kinesis firehose destination
*/
public val kinesisDestination: Output?
get() = javaResource.kinesisDestination().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> eventDestinationKinesisDestinationToKotlin(args0) })
}).orElse(null)
})
/**
* A list of matching types. May be any of `"send"`, `"reject"`, `"bounce"`, `"complaint"`, `"delivery"`, `"open"`, `"click"`, or `"renderingFailure"`.
*/
public val matchingTypes: Output>
get() = javaResource.matchingTypes().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The name of the event destination
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Send the events to an SNS Topic destination
* > **NOTE:** You can specify `"cloudwatch_destination"` or `"kinesis_destination"` but not both
*/
public val snsDestination: Output?
get() = javaResource.snsDestination().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> eventDestinationSnsDestinationToKotlin(args0) })
}).orElse(null)
})
}
public object EventDestinationMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.aws.ses.EventDestination::class == javaResource::class
override fun map(javaResource: Resource): EventDestination = EventDestination(
javaResource as
com.pulumi.aws.ses.EventDestination,
)
}
/**
* @see [EventDestination].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [EventDestination].
*/
public suspend fun eventDestination(
name: String,
block: suspend EventDestinationResourceBuilder.() -> Unit,
): EventDestination {
val builder = EventDestinationResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [EventDestination].
* @param name The _unique_ name of the resulting resource.
*/
public fun eventDestination(name: String): EventDestination {
val builder = EventDestinationResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy