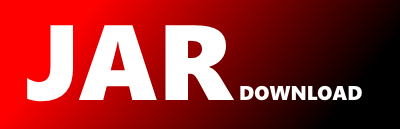
com.pulumi.aws.ses.kotlin.IdentityNotificationTopicArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ses.kotlin
import com.pulumi.aws.ses.IdentityNotificationTopicArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Resource for managing SES Identity Notification Topics
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = new aws.ses.IdentityNotificationTopic("test", {
* topicArn: exampleAwsSnsTopic.arn,
* notificationType: "Bounce",
* identity: example.domain,
* includeOriginalHeaders: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test = aws.ses.IdentityNotificationTopic("test",
* topic_arn=example_aws_sns_topic["arn"],
* notification_type="Bounce",
* identity=example["domain"],
* include_original_headers=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = new Aws.Ses.IdentityNotificationTopic("test", new()
* {
* TopicArn = exampleAwsSnsTopic.Arn,
* NotificationType = "Bounce",
* Identity = example.Domain,
* IncludeOriginalHeaders = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ses"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ses.NewIdentityNotificationTopic(ctx, "test", &ses.IdentityNotificationTopicArgs{
* TopicArn: pulumi.Any(exampleAwsSnsTopic.Arn),
* NotificationType: pulumi.String("Bounce"),
* Identity: pulumi.Any(example.Domain),
* IncludeOriginalHeaders: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ses.IdentityNotificationTopic;
* import com.pulumi.aws.ses.IdentityNotificationTopicArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new IdentityNotificationTopic("test", IdentityNotificationTopicArgs.builder()
* .topicArn(exampleAwsSnsTopic.arn())
* .notificationType("Bounce")
* .identity(example.domain())
* .includeOriginalHeaders(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:ses:IdentityNotificationTopic
* properties:
* topicArn: ${exampleAwsSnsTopic.arn}
* notificationType: Bounce
* identity: ${example.domain}
* includeOriginalHeaders: true
* ```
*
* ## Import
* Using `pulumi import`, import Identity Notification Topics using the ID of the record. The ID is made up as `IDENTITY|TYPE` where `IDENTITY` is the SES Identity and `TYPE` is the Notification Type. For example:
* ```sh
* $ pulumi import aws:ses/identityNotificationTopic:IdentityNotificationTopic test 'example.com|Bounce'
* ```
* @property identity The identity for which the Amazon SNS topic will be set. You can specify an identity by using its name or by using its Amazon Resource Name (ARN).
* @property includeOriginalHeaders Whether SES should include original email headers in SNS notifications of this type. `false` by default.
* @property notificationType The type of notifications that will be published to the specified Amazon SNS topic. Valid Values: `Bounce`, `Complaint` or `Delivery`.
* @property topicArn The Amazon Resource Name (ARN) of the Amazon SNS topic. Can be set to `""` (an empty string) to disable publishing.
*/
public data class IdentityNotificationTopicArgs(
public val identity: Output? = null,
public val includeOriginalHeaders: Output? = null,
public val notificationType: Output? = null,
public val topicArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ses.IdentityNotificationTopicArgs =
com.pulumi.aws.ses.IdentityNotificationTopicArgs.builder()
.identity(identity?.applyValue({ args0 -> args0 }))
.includeOriginalHeaders(includeOriginalHeaders?.applyValue({ args0 -> args0 }))
.notificationType(notificationType?.applyValue({ args0 -> args0 }))
.topicArn(topicArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [IdentityNotificationTopicArgs].
*/
@PulumiTagMarker
public class IdentityNotificationTopicArgsBuilder internal constructor() {
private var identity: Output? = null
private var includeOriginalHeaders: Output? = null
private var notificationType: Output? = null
private var topicArn: Output? = null
/**
* @param value The identity for which the Amazon SNS topic will be set. You can specify an identity by using its name or by using its Amazon Resource Name (ARN).
*/
@JvmName("pwbmbssbxfupkwwt")
public suspend fun identity(`value`: Output) {
this.identity = value
}
/**
* @param value Whether SES should include original email headers in SNS notifications of this type. `false` by default.
*/
@JvmName("gjwulwfvtcniqtwx")
public suspend fun includeOriginalHeaders(`value`: Output) {
this.includeOriginalHeaders = value
}
/**
* @param value The type of notifications that will be published to the specified Amazon SNS topic. Valid Values: `Bounce`, `Complaint` or `Delivery`.
*/
@JvmName("qexiumgjpvitwctq")
public suspend fun notificationType(`value`: Output) {
this.notificationType = value
}
/**
* @param value The Amazon Resource Name (ARN) of the Amazon SNS topic. Can be set to `""` (an empty string) to disable publishing.
*/
@JvmName("oriohaedbntjgenp")
public suspend fun topicArn(`value`: Output) {
this.topicArn = value
}
/**
* @param value The identity for which the Amazon SNS topic will be set. You can specify an identity by using its name or by using its Amazon Resource Name (ARN).
*/
@JvmName("tafthtukywwecfej")
public suspend fun identity(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identity = mapped
}
/**
* @param value Whether SES should include original email headers in SNS notifications of this type. `false` by default.
*/
@JvmName("wtscbmhihhwnwjrj")
public suspend fun includeOriginalHeaders(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeOriginalHeaders = mapped
}
/**
* @param value The type of notifications that will be published to the specified Amazon SNS topic. Valid Values: `Bounce`, `Complaint` or `Delivery`.
*/
@JvmName("tslvcmepagaayfse")
public suspend fun notificationType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.notificationType = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of the Amazon SNS topic. Can be set to `""` (an empty string) to disable publishing.
*/
@JvmName("upkjxprnaiahnnfo")
public suspend fun topicArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.topicArn = mapped
}
internal fun build(): IdentityNotificationTopicArgs = IdentityNotificationTopicArgs(
identity = identity,
includeOriginalHeaders = includeOriginalHeaders,
notificationType = notificationType,
topicArn = topicArn,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy