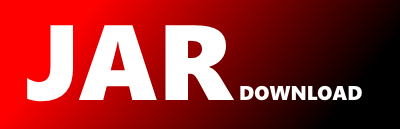
com.pulumi.aws.ses.kotlin.TemplateArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ses.kotlin
import com.pulumi.aws.ses.TemplateArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides a resource to create a SES template.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const myTemplate = new aws.ses.Template("MyTemplate", {
* name: "MyTemplate",
* subject: "Greetings, {{name}}!",
* html: "Hello {{name}},
Your favorite animal is {{favoriteanimal}}.
",
* text: `Hello {{name}},\x0d
* Your favorite animal is {{favoriteanimal}}.`,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* my_template = aws.ses.Template("MyTemplate",
* name="MyTemplate",
* subject="Greetings, {{name}}!",
* html="Hello {{name}},
Your favorite animal is {{favoriteanimal}}.
",
* text="""Hello {{name}},\x0d
* Your favorite animal is {{favoriteanimal}}.""")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var myTemplate = new Aws.Ses.Template("MyTemplate", new()
* {
* Name = "MyTemplate",
* Subject = "Greetings, {{name}}!",
* Html = "Hello {{name}},
Your favorite animal is {{favoriteanimal}}.
",
* Text = @"Hello {{name}},
* Your favorite animal is {{favoriteanimal}}.",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ses"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ses.NewTemplate(ctx, "MyTemplate", &ses.TemplateArgs{
* Name: pulumi.String("MyTemplate"),
* Subject: pulumi.String("Greetings, {{name}}!"),
* Html: pulumi.String("Hello {{name}},
Your favorite animal is {{favoriteanimal}}.
"),
* Text: pulumi.String("Hello {{name}},
\nYour favorite animal is {{favoriteanimal}}."),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ses.Template;
* import com.pulumi.aws.ses.TemplateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var myTemplate = new Template("myTemplate", TemplateArgs.builder()
* .name("MyTemplate")
* .subject("Greetings, {{name}}!")
* .html("Hello {{name}},
Your favorite animal is {{favoriteanimal}}.
")
* .text("""
* Hello {{name}},
* Your favorite animal is {{favoriteanimal}}. """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* myTemplate:
* type: aws:ses:Template
* name: MyTemplate
* properties:
* name: MyTemplate
* subject: Greetings, {{name}}!
* html: Hello {{name}},
Your favorite animal is {{favoriteanimal}}.
* text: "Hello {{name}},\r\nYour favorite animal is {{favoriteanimal}}."
* ```
*
* ## Import
* Using `pulumi import`, import SES templates using the template name. For example:
* ```sh
* $ pulumi import aws:ses/template:Template MyTemplate MyTemplate
* ```
* @property html The HTML body of the email. Must be less than 500KB in size, including both the text and HTML parts.
* @property name The name of the template. Cannot exceed 64 characters. You will refer to this name when you send email.
* @property subject The subject line of the email.
* @property text The email body that will be visible to recipients whose email clients do not display HTML. Must be less than 500KB in size, including both the text and HTML parts.
*/
public data class TemplateArgs(
public val html: Output? = null,
public val name: Output? = null,
public val subject: Output? = null,
public val text: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ses.TemplateArgs = com.pulumi.aws.ses.TemplateArgs.builder()
.html(html?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.subject(subject?.applyValue({ args0 -> args0 }))
.text(text?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TemplateArgs].
*/
@PulumiTagMarker
public class TemplateArgsBuilder internal constructor() {
private var html: Output? = null
private var name: Output? = null
private var subject: Output? = null
private var text: Output? = null
/**
* @param value The HTML body of the email. Must be less than 500KB in size, including both the text and HTML parts.
*/
@JvmName("vdbwhxnolujtcqyk")
public suspend fun html(`value`: Output) {
this.html = value
}
/**
* @param value The name of the template. Cannot exceed 64 characters. You will refer to this name when you send email.
*/
@JvmName("ausespxvmgyelqpe")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The subject line of the email.
*/
@JvmName("jupbmxfceacfylie")
public suspend fun subject(`value`: Output) {
this.subject = value
}
/**
* @param value The email body that will be visible to recipients whose email clients do not display HTML. Must be less than 500KB in size, including both the text and HTML parts.
*/
@JvmName("cemmbwjiwdklyesx")
public suspend fun text(`value`: Output) {
this.text = value
}
/**
* @param value The HTML body of the email. Must be less than 500KB in size, including both the text and HTML parts.
*/
@JvmName("hluijangeiqinifm")
public suspend fun html(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.html = mapped
}
/**
* @param value The name of the template. Cannot exceed 64 characters. You will refer to this name when you send email.
*/
@JvmName("jctprrbyhyrasvoh")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The subject line of the email.
*/
@JvmName("kavhwdiagenhayhe")
public suspend fun subject(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subject = mapped
}
/**
* @param value The email body that will be visible to recipients whose email clients do not display HTML. Must be less than 500KB in size, including both the text and HTML parts.
*/
@JvmName("awfvjdyrrvgutmbt")
public suspend fun text(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.text = mapped
}
internal fun build(): TemplateArgs = TemplateArgs(
html = html,
name = name,
subject = subject,
text = text,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy