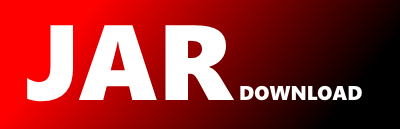
com.pulumi.aws.sesv2.kotlin.AccountVdmAttributesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.sesv2.kotlin
import com.pulumi.aws.sesv2.AccountVdmAttributesArgs.builder
import com.pulumi.aws.sesv2.kotlin.inputs.AccountVdmAttributesDashboardAttributesArgs
import com.pulumi.aws.sesv2.kotlin.inputs.AccountVdmAttributesDashboardAttributesArgsBuilder
import com.pulumi.aws.sesv2.kotlin.inputs.AccountVdmAttributesGuardianAttributesArgs
import com.pulumi.aws.sesv2.kotlin.inputs.AccountVdmAttributesGuardianAttributesArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Resource for managing an AWS SESv2 (Simple Email V2) Account VDM Attributes.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.sesv2.AccountVdmAttributes("example", {
* vdmEnabled: "ENABLED",
* dashboardAttributes: {
* engagementMetrics: "ENABLED",
* },
* guardianAttributes: {
* optimizedSharedDelivery: "ENABLED",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.sesv2.AccountVdmAttributes("example",
* vdm_enabled="ENABLED",
* dashboard_attributes={
* "engagement_metrics": "ENABLED",
* },
* guardian_attributes={
* "optimized_shared_delivery": "ENABLED",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.SesV2.AccountVdmAttributes("example", new()
* {
* VdmEnabled = "ENABLED",
* DashboardAttributes = new Aws.SesV2.Inputs.AccountVdmAttributesDashboardAttributesArgs
* {
* EngagementMetrics = "ENABLED",
* },
* GuardianAttributes = new Aws.SesV2.Inputs.AccountVdmAttributesGuardianAttributesArgs
* {
* OptimizedSharedDelivery = "ENABLED",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/sesv2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sesv2.NewAccountVdmAttributes(ctx, "example", &sesv2.AccountVdmAttributesArgs{
* VdmEnabled: pulumi.String("ENABLED"),
* DashboardAttributes: &sesv2.AccountVdmAttributesDashboardAttributesArgs{
* EngagementMetrics: pulumi.String("ENABLED"),
* },
* GuardianAttributes: &sesv2.AccountVdmAttributesGuardianAttributesArgs{
* OptimizedSharedDelivery: pulumi.String("ENABLED"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sesv2.AccountVdmAttributes;
* import com.pulumi.aws.sesv2.AccountVdmAttributesArgs;
* import com.pulumi.aws.sesv2.inputs.AccountVdmAttributesDashboardAttributesArgs;
* import com.pulumi.aws.sesv2.inputs.AccountVdmAttributesGuardianAttributesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new AccountVdmAttributes("example", AccountVdmAttributesArgs.builder()
* .vdmEnabled("ENABLED")
* .dashboardAttributes(AccountVdmAttributesDashboardAttributesArgs.builder()
* .engagementMetrics("ENABLED")
* .build())
* .guardianAttributes(AccountVdmAttributesGuardianAttributesArgs.builder()
* .optimizedSharedDelivery("ENABLED")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:sesv2:AccountVdmAttributes
* properties:
* vdmEnabled: ENABLED
* dashboardAttributes:
* engagementMetrics: ENABLED
* guardianAttributes:
* optimizedSharedDelivery: ENABLED
* ```
*
* ## Import
* Using `pulumi import`, import SESv2 (Simple Email V2) Account VDM Attributes using the word `ses-account-vdm-attributes`. For example:
* ```sh
* $ pulumi import aws:sesv2/accountVdmAttributes:AccountVdmAttributes example ses-account-vdm-attributes
* ```
* @property dashboardAttributes Specifies additional settings for your VDM configuration as applicable to the Dashboard.
* @property guardianAttributes Specifies additional settings for your VDM configuration as applicable to the Guardian.
* @property vdmEnabled Specifies the status of your VDM configuration. Valid values: `ENABLED`, `DISABLED`.
* The following arguments are optional:
*/
public data class AccountVdmAttributesArgs(
public val dashboardAttributes: Output? = null,
public val guardianAttributes: Output? = null,
public val vdmEnabled: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.sesv2.AccountVdmAttributesArgs =
com.pulumi.aws.sesv2.AccountVdmAttributesArgs.builder()
.dashboardAttributes(
dashboardAttributes?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.guardianAttributes(
guardianAttributes?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.vdmEnabled(vdmEnabled?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AccountVdmAttributesArgs].
*/
@PulumiTagMarker
public class AccountVdmAttributesArgsBuilder internal constructor() {
private var dashboardAttributes: Output? = null
private var guardianAttributes: Output? = null
private var vdmEnabled: Output? = null
/**
* @param value Specifies additional settings for your VDM configuration as applicable to the Dashboard.
*/
@JvmName("luawnkglgjhheahn")
public suspend fun dashboardAttributes(`value`: Output) {
this.dashboardAttributes = value
}
/**
* @param value Specifies additional settings for your VDM configuration as applicable to the Guardian.
*/
@JvmName("hfrxbjqhtfqrunkx")
public suspend fun guardianAttributes(`value`: Output) {
this.guardianAttributes = value
}
/**
* @param value Specifies the status of your VDM configuration. Valid values: `ENABLED`, `DISABLED`.
* The following arguments are optional:
*/
@JvmName("dgjlugqqrnouerjo")
public suspend fun vdmEnabled(`value`: Output) {
this.vdmEnabled = value
}
/**
* @param value Specifies additional settings for your VDM configuration as applicable to the Dashboard.
*/
@JvmName("skolrjtnxyxwahfx")
public suspend fun dashboardAttributes(`value`: AccountVdmAttributesDashboardAttributesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dashboardAttributes = mapped
}
/**
* @param argument Specifies additional settings for your VDM configuration as applicable to the Dashboard.
*/
@JvmName("jkyscruvgidwjywi")
public suspend fun dashboardAttributes(argument: suspend AccountVdmAttributesDashboardAttributesArgsBuilder.() -> Unit) {
val toBeMapped = AccountVdmAttributesDashboardAttributesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dashboardAttributes = mapped
}
/**
* @param value Specifies additional settings for your VDM configuration as applicable to the Guardian.
*/
@JvmName("jajovpydxcohikec")
public suspend fun guardianAttributes(`value`: AccountVdmAttributesGuardianAttributesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.guardianAttributes = mapped
}
/**
* @param argument Specifies additional settings for your VDM configuration as applicable to the Guardian.
*/
@JvmName("rsgppgycwqhprxns")
public suspend fun guardianAttributes(argument: suspend AccountVdmAttributesGuardianAttributesArgsBuilder.() -> Unit) {
val toBeMapped = AccountVdmAttributesGuardianAttributesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.guardianAttributes = mapped
}
/**
* @param value Specifies the status of your VDM configuration. Valid values: `ENABLED`, `DISABLED`.
* The following arguments are optional:
*/
@JvmName("oiwrscxkcewfbobv")
public suspend fun vdmEnabled(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vdmEnabled = mapped
}
internal fun build(): AccountVdmAttributesArgs = AccountVdmAttributesArgs(
dashboardAttributes = dashboardAttributes,
guardianAttributes = guardianAttributes,
vdmEnabled = vdmEnabled,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy