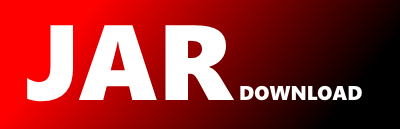
com.pulumi.aws.shield.kotlin.ApplicationLayerAutomaticResponseArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.shield.kotlin
import com.pulumi.aws.shield.ApplicationLayerAutomaticResponseArgs.builder
import com.pulumi.aws.shield.kotlin.inputs.ApplicationLayerAutomaticResponseTimeoutsArgs
import com.pulumi.aws.shield.kotlin.inputs.ApplicationLayerAutomaticResponseTimeoutsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Resource for managing an AWS Shield Application Layer Automatic Response for automatic DDoS mitigation.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const current = aws.getRegion({});
* const currentGetCallerIdentity = aws.getCallerIdentity({});
* const currentGetPartition = aws.getPartition({});
* const config = new pulumi.Config();
* // The Cloudfront Distribution on which to enable the Application Layer Automatic Response.
* const distributionId = config.require("distributionId");
* const example = new aws.shield.ApplicationLayerAutomaticResponse("example", {
* resourceArn: Promise.all([currentGetPartition, currentGetCallerIdentity]).then(([currentGetPartition, currentGetCallerIdentity]) => `arn:${currentGetPartition.partition}:cloudfront:${currentGetCallerIdentity.accountId}:distribution/${distributionId}`),
* action: "COUNT",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* current = aws.get_region()
* current_get_caller_identity = aws.get_caller_identity()
* current_get_partition = aws.get_partition()
* config = pulumi.Config()
* # The Cloudfront Distribution on which to enable the Application Layer Automatic Response.
* distribution_id = config.require("distributionId")
* example = aws.shield.ApplicationLayerAutomaticResponse("example",
* resource_arn=f"arn:{current_get_partition.partition}:cloudfront:{current_get_caller_identity.account_id}:distribution/{distribution_id}",
* action="COUNT")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var current = Aws.GetRegion.Invoke();
* var currentGetCallerIdentity = Aws.GetCallerIdentity.Invoke();
* var currentGetPartition = Aws.GetPartition.Invoke();
* var config = new Config();
* // The Cloudfront Distribution on which to enable the Application Layer Automatic Response.
* var distributionId = config.Require("distributionId");
* var example = new Aws.Shield.ApplicationLayerAutomaticResponse("example", new()
* {
* ResourceArn = Output.Tuple(currentGetPartition, currentGetCallerIdentity).Apply(values =>
* {
* var currentGetPartition = values.Item1;
* var currentGetCallerIdentity = values.Item2;
* return $"arn:{currentGetPartition.Apply(getPartitionResult => getPartitionResult.Partition)}:cloudfront:{currentGetCallerIdentity.Apply(getCallerIdentityResult => getCallerIdentityResult.AccountId)}:distribution/{distributionId}";
* }),
* Action = "COUNT",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/shield"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi/config"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := aws.GetRegion(ctx, &aws.GetRegionArgs{}, nil)
* if err != nil {
* return err
* }
* currentGetCallerIdentity, err := aws.GetCallerIdentity(ctx, &aws.GetCallerIdentityArgs{}, nil)
* if err != nil {
* return err
* }
* currentGetPartition, err := aws.GetPartition(ctx, &aws.GetPartitionArgs{}, nil)
* if err != nil {
* return err
* }
* cfg := config.New(ctx, "")
* // The Cloudfront Distribution on which to enable the Application Layer Automatic Response.
* distributionId := cfg.Require("distributionId")
* _, err = shield.NewApplicationLayerAutomaticResponse(ctx, "example", &shield.ApplicationLayerAutomaticResponseArgs{
* ResourceArn: pulumi.Sprintf("arn:%v:cloudfront:%v:distribution/%v", currentGetPartition.Partition, currentGetCallerIdentity.AccountId, distributionId),
* Action: pulumi.String("COUNT"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetRegionArgs;
* import com.pulumi.aws.inputs.GetCallerIdentityArgs;
* import com.pulumi.aws.inputs.GetPartitionArgs;
* import com.pulumi.aws.shield.ApplicationLayerAutomaticResponse;
* import com.pulumi.aws.shield.ApplicationLayerAutomaticResponseArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var config = ctx.config();
* final var current = AwsFunctions.getRegion();
* final var currentGetCallerIdentity = AwsFunctions.getCallerIdentity();
* final var currentGetPartition = AwsFunctions.getPartition();
* final var distributionId = config.get("distributionId");
* var example = new ApplicationLayerAutomaticResponse("example", ApplicationLayerAutomaticResponseArgs.builder()
* .resourceArn(String.format("arn:%s:cloudfront:%s:distribution/%s", currentGetPartition.applyValue(getPartitionResult -> getPartitionResult.partition()),currentGetCallerIdentity.applyValue(getCallerIdentityResult -> getCallerIdentityResult.accountId()),distributionId))
* .action("COUNT")
* .build());
* }
* }
* ```
* ```yaml
* configuration:
* distributionId:
* type: string
* resources:
* example:
* type: aws:shield:ApplicationLayerAutomaticResponse
* properties:
* resourceArn: arn:${currentGetPartition.partition}:cloudfront:${currentGetCallerIdentity.accountId}:distribution/${distributionId}
* action: COUNT
* variables:
* current:
* fn::invoke:
* Function: aws:getRegion
* Arguments: {}
* currentGetCallerIdentity:
* fn::invoke:
* Function: aws:getCallerIdentity
* Arguments: {}
* currentGetPartition:
* fn::invoke:
* Function: aws:getPartition
* Arguments: {}
* ```
*
* @property action One of `COUNT` or `BLOCK`
* @property resourceArn ARN of the resource to protect (Cloudfront Distributions and ALBs only at this time).
* @property timeouts
*/
public data class ApplicationLayerAutomaticResponseArgs(
public val action: Output? = null,
public val resourceArn: Output? = null,
public val timeouts: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.shield.ApplicationLayerAutomaticResponseArgs =
com.pulumi.aws.shield.ApplicationLayerAutomaticResponseArgs.builder()
.action(action?.applyValue({ args0 -> args0 }))
.resourceArn(resourceArn?.applyValue({ args0 -> args0 }))
.timeouts(timeouts?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ApplicationLayerAutomaticResponseArgs].
*/
@PulumiTagMarker
public class ApplicationLayerAutomaticResponseArgsBuilder internal constructor() {
private var action: Output? = null
private var resourceArn: Output? = null
private var timeouts: Output? = null
/**
* @param value One of `COUNT` or `BLOCK`
*/
@JvmName("vtbtatrfodxdsprb")
public suspend fun action(`value`: Output) {
this.action = value
}
/**
* @param value ARN of the resource to protect (Cloudfront Distributions and ALBs only at this time).
*/
@JvmName("kufsojrvhpbiifhr")
public suspend fun resourceArn(`value`: Output) {
this.resourceArn = value
}
/**
* @param value
*/
@JvmName("khiydqbjphkjdfcp")
public suspend fun timeouts(`value`: Output) {
this.timeouts = value
}
/**
* @param value One of `COUNT` or `BLOCK`
*/
@JvmName("mvyjksjllqpyktab")
public suspend fun action(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.action = mapped
}
/**
* @param value ARN of the resource to protect (Cloudfront Distributions and ALBs only at this time).
*/
@JvmName("fgvgaxjqpedqhlyj")
public suspend fun resourceArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceArn = mapped
}
/**
* @param value
*/
@JvmName("vbxsybwbemeojbon")
public suspend fun timeouts(`value`: ApplicationLayerAutomaticResponseTimeoutsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeouts = mapped
}
/**
* @param argument
*/
@JvmName("ujwgpkapwdpugrhj")
public suspend fun timeouts(argument: suspend ApplicationLayerAutomaticResponseTimeoutsArgsBuilder.() -> Unit) {
val toBeMapped = ApplicationLayerAutomaticResponseTimeoutsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.timeouts = mapped
}
internal fun build(): ApplicationLayerAutomaticResponseArgs =
ApplicationLayerAutomaticResponseArgs(
action = action,
resourceArn = resourceArn,
timeouts = timeouts,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy