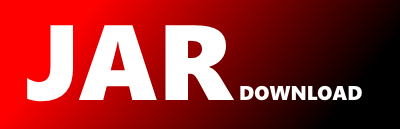
com.pulumi.aws.sns.kotlin.PlatformApplicationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.sns.kotlin
import com.pulumi.aws.sns.PlatformApplicationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides an SNS platform application resource
* ## Example Usage
* ### Apple Push Notification Service (APNS) using certificate-based authentication
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const apnsApplication = new aws.sns.PlatformApplication("apns_application", {
* name: "apns_application",
* platform: "APNS",
* platformCredential: "",
* platformPrincipal: "",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* apns_application = aws.sns.PlatformApplication("apns_application",
* name="apns_application",
* platform="APNS",
* platform_credential="",
* platform_principal="")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var apnsApplication = new Aws.Sns.PlatformApplication("apns_application", new()
* {
* Name = "apns_application",
* Platform = "APNS",
* PlatformCredential = "",
* PlatformPrincipal = "",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/sns"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sns.NewPlatformApplication(ctx, "apns_application", &sns.PlatformApplicationArgs{
* Name: pulumi.String("apns_application"),
* Platform: pulumi.String("APNS"),
* PlatformCredential: pulumi.String(""),
* PlatformPrincipal: pulumi.String(""),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sns.PlatformApplication;
* import com.pulumi.aws.sns.PlatformApplicationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var apnsApplication = new PlatformApplication("apnsApplication", PlatformApplicationArgs.builder()
* .name("apns_application")
* .platform("APNS")
* .platformCredential("")
* .platformPrincipal("")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* apnsApplication:
* type: aws:sns:PlatformApplication
* name: apns_application
* properties:
* name: apns_application
* platform: APNS
* platformCredential:
* platformPrincipal:
* ```
*
* ### Apple Push Notification Service (APNS) using token-based authentication
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const apnsApplication = new aws.sns.PlatformApplication("apns_application", {
* name: "apns_application",
* platform: "APNS",
* platformCredential: "",
* platformPrincipal: "",
* applePlatformTeamId: "",
* applePlatformBundleId: "",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* apns_application = aws.sns.PlatformApplication("apns_application",
* name="apns_application",
* platform="APNS",
* platform_credential="",
* platform_principal="",
* apple_platform_team_id="",
* apple_platform_bundle_id="")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var apnsApplication = new Aws.Sns.PlatformApplication("apns_application", new()
* {
* Name = "apns_application",
* Platform = "APNS",
* PlatformCredential = "",
* PlatformPrincipal = "",
* ApplePlatformTeamId = "",
* ApplePlatformBundleId = "",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/sns"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sns.NewPlatformApplication(ctx, "apns_application", &sns.PlatformApplicationArgs{
* Name: pulumi.String("apns_application"),
* Platform: pulumi.String("APNS"),
* PlatformCredential: pulumi.String(""),
* PlatformPrincipal: pulumi.String(""),
* ApplePlatformTeamId: pulumi.String(""),
* ApplePlatformBundleId: pulumi.String(""),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sns.PlatformApplication;
* import com.pulumi.aws.sns.PlatformApplicationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var apnsApplication = new PlatformApplication("apnsApplication", PlatformApplicationArgs.builder()
* .name("apns_application")
* .platform("APNS")
* .platformCredential("")
* .platformPrincipal("")
* .applePlatformTeamId("")
* .applePlatformBundleId("")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* apnsApplication:
* type: aws:sns:PlatformApplication
* name: apns_application
* properties:
* name: apns_application
* platform: APNS
* platformCredential:
* platformPrincipal:
* applePlatformTeamId:
* applePlatformBundleId:
* ```
*
* ### Google Cloud Messaging (GCM)
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const gcmApplication = new aws.sns.PlatformApplication("gcm_application", {
* name: "gcm_application",
* platform: "GCM",
* platformCredential: "",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* gcm_application = aws.sns.PlatformApplication("gcm_application",
* name="gcm_application",
* platform="GCM",
* platform_credential="")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var gcmApplication = new Aws.Sns.PlatformApplication("gcm_application", new()
* {
* Name = "gcm_application",
* Platform = "GCM",
* PlatformCredential = "",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/sns"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sns.NewPlatformApplication(ctx, "gcm_application", &sns.PlatformApplicationArgs{
* Name: pulumi.String("gcm_application"),
* Platform: pulumi.String("GCM"),
* PlatformCredential: pulumi.String(""),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sns.PlatformApplication;
* import com.pulumi.aws.sns.PlatformApplicationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var gcmApplication = new PlatformApplication("gcmApplication", PlatformApplicationArgs.builder()
* .name("gcm_application")
* .platform("GCM")
* .platformCredential("")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* gcmApplication:
* type: aws:sns:PlatformApplication
* name: gcm_application
* properties:
* name: gcm_application
* platform: GCM
* platformCredential:
* ```
*
* ## Import
* Using `pulumi import`, import SNS platform applications using the ARN. For example:
* ```sh
* $ pulumi import aws:sns/platformApplication:PlatformApplication gcm_application arn:aws:sns:us-west-2:123456789012:app/GCM/gcm_application
* ```
* @property applePlatformBundleId The bundle identifier that's assigned to your iOS app. May only include alphanumeric characters, hyphens (-), and periods (.).
* @property applePlatformTeamId The identifier that's assigned to your Apple developer account team. Must be 10 alphanumeric characters.
* @property eventDeliveryFailureTopicArn The ARN of the SNS Topic triggered when a delivery to any of the platform endpoints associated with your platform application encounters a permanent failure.
* @property eventEndpointCreatedTopicArn The ARN of the SNS Topic triggered when a new platform endpoint is added to your platform application.
* @property eventEndpointDeletedTopicArn The ARN of the SNS Topic triggered when an existing platform endpoint is deleted from your platform application.
* @property eventEndpointUpdatedTopicArn The ARN of the SNS Topic triggered when an existing platform endpoint is changed from your platform application.
* @property failureFeedbackRoleArn The IAM role ARN permitted to receive failure feedback for this application and give SNS write access to use CloudWatch logs on your behalf.
* @property name The friendly name for the SNS platform application
* @property platform The platform that the app is registered with. See [Platform](http://docs.aws.amazon.com/sns/latest/dg/mobile-push-send-register.html) for supported platforms.
* @property platformCredential Application Platform credential. See [Credential](http://docs.aws.amazon.com/sns/latest/dg/mobile-push-send-register.html) for type of credential required for platform. The value of this attribute when stored into the state is only a hash of the real value, so therefore it is not practical to use this as an attribute for other resources.
* @property platformPrincipal Application Platform principal. See [Principal](http://docs.aws.amazon.com/sns/latest/api/API_CreatePlatformApplication.html) for type of principal required for platform. The value of this attribute when stored into the state is only a hash of the real value, so therefore it is not practical to use this as an attribute for other resources.
* @property successFeedbackRoleArn The IAM role ARN permitted to receive success feedback for this application and give SNS write access to use CloudWatch logs on your behalf.
* @property successFeedbackSampleRate The sample rate percentage (0-100) of successfully delivered messages.
* The following attributes are needed only when using APNS token credentials:
*/
public data class PlatformApplicationArgs(
public val applePlatformBundleId: Output? = null,
public val applePlatformTeamId: Output? = null,
public val eventDeliveryFailureTopicArn: Output? = null,
public val eventEndpointCreatedTopicArn: Output? = null,
public val eventEndpointDeletedTopicArn: Output? = null,
public val eventEndpointUpdatedTopicArn: Output? = null,
public val failureFeedbackRoleArn: Output? = null,
public val name: Output? = null,
public val platform: Output? = null,
public val platformCredential: Output? = null,
public val platformPrincipal: Output? = null,
public val successFeedbackRoleArn: Output? = null,
public val successFeedbackSampleRate: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.sns.PlatformApplicationArgs =
com.pulumi.aws.sns.PlatformApplicationArgs.builder()
.applePlatformBundleId(applePlatformBundleId?.applyValue({ args0 -> args0 }))
.applePlatformTeamId(applePlatformTeamId?.applyValue({ args0 -> args0 }))
.eventDeliveryFailureTopicArn(eventDeliveryFailureTopicArn?.applyValue({ args0 -> args0 }))
.eventEndpointCreatedTopicArn(eventEndpointCreatedTopicArn?.applyValue({ args0 -> args0 }))
.eventEndpointDeletedTopicArn(eventEndpointDeletedTopicArn?.applyValue({ args0 -> args0 }))
.eventEndpointUpdatedTopicArn(eventEndpointUpdatedTopicArn?.applyValue({ args0 -> args0 }))
.failureFeedbackRoleArn(failureFeedbackRoleArn?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.platform(platform?.applyValue({ args0 -> args0 }))
.platformCredential(platformCredential?.applyValue({ args0 -> args0 }))
.platformPrincipal(platformPrincipal?.applyValue({ args0 -> args0 }))
.successFeedbackRoleArn(successFeedbackRoleArn?.applyValue({ args0 -> args0 }))
.successFeedbackSampleRate(successFeedbackSampleRate?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PlatformApplicationArgs].
*/
@PulumiTagMarker
public class PlatformApplicationArgsBuilder internal constructor() {
private var applePlatformBundleId: Output? = null
private var applePlatformTeamId: Output? = null
private var eventDeliveryFailureTopicArn: Output? = null
private var eventEndpointCreatedTopicArn: Output? = null
private var eventEndpointDeletedTopicArn: Output? = null
private var eventEndpointUpdatedTopicArn: Output? = null
private var failureFeedbackRoleArn: Output? = null
private var name: Output? = null
private var platform: Output? = null
private var platformCredential: Output? = null
private var platformPrincipal: Output? = null
private var successFeedbackRoleArn: Output? = null
private var successFeedbackSampleRate: Output? = null
/**
* @param value The bundle identifier that's assigned to your iOS app. May only include alphanumeric characters, hyphens (-), and periods (.).
*/
@JvmName("uduiqcpbnkqqmbur")
public suspend fun applePlatformBundleId(`value`: Output) {
this.applePlatformBundleId = value
}
/**
* @param value The identifier that's assigned to your Apple developer account team. Must be 10 alphanumeric characters.
*/
@JvmName("xamppuenhetevbud")
public suspend fun applePlatformTeamId(`value`: Output) {
this.applePlatformTeamId = value
}
/**
* @param value The ARN of the SNS Topic triggered when a delivery to any of the platform endpoints associated with your platform application encounters a permanent failure.
*/
@JvmName("sgqffywbxokqwroo")
public suspend fun eventDeliveryFailureTopicArn(`value`: Output) {
this.eventDeliveryFailureTopicArn = value
}
/**
* @param value The ARN of the SNS Topic triggered when a new platform endpoint is added to your platform application.
*/
@JvmName("fseftcfqodjrwfvc")
public suspend fun eventEndpointCreatedTopicArn(`value`: Output) {
this.eventEndpointCreatedTopicArn = value
}
/**
* @param value The ARN of the SNS Topic triggered when an existing platform endpoint is deleted from your platform application.
*/
@JvmName("fuplfmaoqpdbrsur")
public suspend fun eventEndpointDeletedTopicArn(`value`: Output) {
this.eventEndpointDeletedTopicArn = value
}
/**
* @param value The ARN of the SNS Topic triggered when an existing platform endpoint is changed from your platform application.
*/
@JvmName("ddtuuuyhrqyxehpi")
public suspend fun eventEndpointUpdatedTopicArn(`value`: Output) {
this.eventEndpointUpdatedTopicArn = value
}
/**
* @param value The IAM role ARN permitted to receive failure feedback for this application and give SNS write access to use CloudWatch logs on your behalf.
*/
@JvmName("vweydbsdwyphjmjj")
public suspend fun failureFeedbackRoleArn(`value`: Output) {
this.failureFeedbackRoleArn = value
}
/**
* @param value The friendly name for the SNS platform application
*/
@JvmName("jylkdqgmibfqtbxl")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The platform that the app is registered with. See [Platform](http://docs.aws.amazon.com/sns/latest/dg/mobile-push-send-register.html) for supported platforms.
*/
@JvmName("vilsidlqfldagkav")
public suspend fun platform(`value`: Output) {
this.platform = value
}
/**
* @param value Application Platform credential. See [Credential](http://docs.aws.amazon.com/sns/latest/dg/mobile-push-send-register.html) for type of credential required for platform. The value of this attribute when stored into the state is only a hash of the real value, so therefore it is not practical to use this as an attribute for other resources.
*/
@JvmName("thnruyksxcmppgjo")
public suspend fun platformCredential(`value`: Output) {
this.platformCredential = value
}
/**
* @param value Application Platform principal. See [Principal](http://docs.aws.amazon.com/sns/latest/api/API_CreatePlatformApplication.html) for type of principal required for platform. The value of this attribute when stored into the state is only a hash of the real value, so therefore it is not practical to use this as an attribute for other resources.
*/
@JvmName("uehtrheulvfjgtcy")
public suspend fun platformPrincipal(`value`: Output) {
this.platformPrincipal = value
}
/**
* @param value The IAM role ARN permitted to receive success feedback for this application and give SNS write access to use CloudWatch logs on your behalf.
*/
@JvmName("bbhkkwdllksiofmx")
public suspend fun successFeedbackRoleArn(`value`: Output) {
this.successFeedbackRoleArn = value
}
/**
* @param value The sample rate percentage (0-100) of successfully delivered messages.
* The following attributes are needed only when using APNS token credentials:
*/
@JvmName("dorrvpvlpooikfcs")
public suspend fun successFeedbackSampleRate(`value`: Output) {
this.successFeedbackSampleRate = value
}
/**
* @param value The bundle identifier that's assigned to your iOS app. May only include alphanumeric characters, hyphens (-), and periods (.).
*/
@JvmName("tymqpulcgncxpgbh")
public suspend fun applePlatformBundleId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.applePlatformBundleId = mapped
}
/**
* @param value The identifier that's assigned to your Apple developer account team. Must be 10 alphanumeric characters.
*/
@JvmName("bhxpjtdbsaqdthnc")
public suspend fun applePlatformTeamId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.applePlatformTeamId = mapped
}
/**
* @param value The ARN of the SNS Topic triggered when a delivery to any of the platform endpoints associated with your platform application encounters a permanent failure.
*/
@JvmName("tdhsdsnofhlefbvh")
public suspend fun eventDeliveryFailureTopicArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventDeliveryFailureTopicArn = mapped
}
/**
* @param value The ARN of the SNS Topic triggered when a new platform endpoint is added to your platform application.
*/
@JvmName("nevlrjphwlnpjkjl")
public suspend fun eventEndpointCreatedTopicArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventEndpointCreatedTopicArn = mapped
}
/**
* @param value The ARN of the SNS Topic triggered when an existing platform endpoint is deleted from your platform application.
*/
@JvmName("covvurnxqyewwbym")
public suspend fun eventEndpointDeletedTopicArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventEndpointDeletedTopicArn = mapped
}
/**
* @param value The ARN of the SNS Topic triggered when an existing platform endpoint is changed from your platform application.
*/
@JvmName("witflxcprqhecwcy")
public suspend fun eventEndpointUpdatedTopicArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventEndpointUpdatedTopicArn = mapped
}
/**
* @param value The IAM role ARN permitted to receive failure feedback for this application and give SNS write access to use CloudWatch logs on your behalf.
*/
@JvmName("kptykrrsjvcscjdo")
public suspend fun failureFeedbackRoleArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureFeedbackRoleArn = mapped
}
/**
* @param value The friendly name for the SNS platform application
*/
@JvmName("mbxpwyisaymqurpn")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The platform that the app is registered with. See [Platform](http://docs.aws.amazon.com/sns/latest/dg/mobile-push-send-register.html) for supported platforms.
*/
@JvmName("mdhpmolshpkqymib")
public suspend fun platform(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.platform = mapped
}
/**
* @param value Application Platform credential. See [Credential](http://docs.aws.amazon.com/sns/latest/dg/mobile-push-send-register.html) for type of credential required for platform. The value of this attribute when stored into the state is only a hash of the real value, so therefore it is not practical to use this as an attribute for other resources.
*/
@JvmName("sxgsqbnublneatte")
public suspend fun platformCredential(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.platformCredential = mapped
}
/**
* @param value Application Platform principal. See [Principal](http://docs.aws.amazon.com/sns/latest/api/API_CreatePlatformApplication.html) for type of principal required for platform. The value of this attribute when stored into the state is only a hash of the real value, so therefore it is not practical to use this as an attribute for other resources.
*/
@JvmName("ruxwqsfvrvnyyqwe")
public suspend fun platformPrincipal(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.platformPrincipal = mapped
}
/**
* @param value The IAM role ARN permitted to receive success feedback for this application and give SNS write access to use CloudWatch logs on your behalf.
*/
@JvmName("mxgckywdbrfnfmyb")
public suspend fun successFeedbackRoleArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successFeedbackRoleArn = mapped
}
/**
* @param value The sample rate percentage (0-100) of successfully delivered messages.
* The following attributes are needed only when using APNS token credentials:
*/
@JvmName("shhafoonpokrghjn")
public suspend fun successFeedbackSampleRate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successFeedbackSampleRate = mapped
}
internal fun build(): PlatformApplicationArgs = PlatformApplicationArgs(
applePlatformBundleId = applePlatformBundleId,
applePlatformTeamId = applePlatformTeamId,
eventDeliveryFailureTopicArn = eventDeliveryFailureTopicArn,
eventEndpointCreatedTopicArn = eventEndpointCreatedTopicArn,
eventEndpointDeletedTopicArn = eventEndpointDeletedTopicArn,
eventEndpointUpdatedTopicArn = eventEndpointUpdatedTopicArn,
failureFeedbackRoleArn = failureFeedbackRoleArn,
name = name,
platform = platform,
platformCredential = platformCredential,
platformPrincipal = platformPrincipal,
successFeedbackRoleArn = successFeedbackRoleArn,
successFeedbackSampleRate = successFeedbackSampleRate,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy