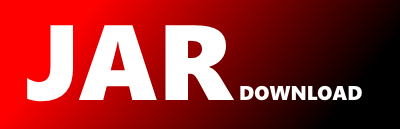
com.pulumi.aws.sqs.kotlin.RedrivePolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.sqs.kotlin
import com.pulumi.aws.sqs.RedrivePolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Allows you to set a redrive policy of an SQS Queue
* while referencing ARN of the dead letter queue inside the redrive policy.
* This is useful when you want to set a dedicated
* dead letter queue for a standard or FIFO queue, but need
* the dead letter queue to exist before setting the redrive policy.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const q = new aws.sqs.Queue("q", {name: "examplequeue"});
* const ddl = new aws.sqs.Queue("ddl", {
* name: "examplequeue-ddl",
* redriveAllowPolicy: pulumi.jsonStringify({
* redrivePermission: "byQueue",
* sourceQueueArns: [q.arn],
* }),
* });
* const qRedrivePolicy = new aws.sqs.RedrivePolicy("q", {
* queueUrl: q.id,
* redrivePolicy: pulumi.jsonStringify({
* deadLetterTargetArn: ddl.arn,
* maxReceiveCount: 4,
* }),
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* q = aws.sqs.Queue("q", name="examplequeue")
* ddl = aws.sqs.Queue("ddl",
* name="examplequeue-ddl",
* redrive_allow_policy=pulumi.Output.json_dumps({
* "redrivePermission": "byQueue",
* "sourceQueueArns": [q.arn],
* }))
* q_redrive_policy = aws.sqs.RedrivePolicy("q",
* queue_url=q.id,
* redrive_policy=pulumi.Output.json_dumps({
* "deadLetterTargetArn": ddl.arn,
* "maxReceiveCount": 4,
* }))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var q = new Aws.Sqs.Queue("q", new()
* {
* Name = "examplequeue",
* });
* var ddl = new Aws.Sqs.Queue("ddl", new()
* {
* Name = "examplequeue-ddl",
* RedriveAllowPolicy = Output.JsonSerialize(Output.Create(new Dictionary
* {
* ["redrivePermission"] = "byQueue",
* ["sourceQueueArns"] = new[]
* {
* q.Arn,
* },
* })),
* });
* var qRedrivePolicy = new Aws.Sqs.RedrivePolicy("q", new()
* {
* QueueUrl = q.Id,
* RedrivePolicyName = Output.JsonSerialize(Output.Create(new Dictionary
* {
* ["deadLetterTargetArn"] = ddl.Arn,
* ["maxReceiveCount"] = 4,
* })),
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/sqs"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* q, err := sqs.NewQueue(ctx, "q", &sqs.QueueArgs{
* Name: pulumi.String("examplequeue"),
* })
* if err != nil {
* return err
* }
* ddl, err := sqs.NewQueue(ctx, "ddl", &sqs.QueueArgs{
* Name: pulumi.String("examplequeue-ddl"),
* RedriveAllowPolicy: q.Arn.ApplyT(func(arn string) (pulumi.String, error) {
* var _zero pulumi.String
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "redrivePermission": "byQueue",
* "sourceQueueArns": []string{
* arn,
* },
* })
* if err != nil {
* return _zero, err
* }
* json0 := string(tmpJSON0)
* return pulumi.String(json0), nil
* }).(pulumi.StringOutput),
* })
* if err != nil {
* return err
* }
* _, err = sqs.NewRedrivePolicy(ctx, "q", &sqs.RedrivePolicyArgs{
* QueueUrl: q.ID(),
* RedrivePolicy: ddl.Arn.ApplyT(func(arn string) (pulumi.String, error) {
* var _zero pulumi.String
* tmpJSON1, err := json.Marshal(map[string]interface{}{
* "deadLetterTargetArn": arn,
* "maxReceiveCount": 4,
* })
* if err != nil {
* return _zero, err
* }
* json1 := string(tmpJSON1)
* return pulumi.String(json1), nil
* }).(pulumi.StringOutput),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sqs.Queue;
* import com.pulumi.aws.sqs.QueueArgs;
* import com.pulumi.aws.sqs.RedrivePolicy;
* import com.pulumi.aws.sqs.RedrivePolicyArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var q = new Queue("q", QueueArgs.builder()
* .name("examplequeue")
* .build());
* var ddl = new Queue("ddl", QueueArgs.builder()
* .name("examplequeue-ddl")
* .redriveAllowPolicy(q.arn().applyValue(arn -> serializeJson(
* jsonObject(
* jsonProperty("redrivePermission", "byQueue"),
* jsonProperty("sourceQueueArns", jsonArray(arn))
* ))))
* .build());
* var qRedrivePolicy = new RedrivePolicy("qRedrivePolicy", RedrivePolicyArgs.builder()
* .queueUrl(q.id())
* .redrivePolicy(ddl.arn().applyValue(arn -> serializeJson(
* jsonObject(
* jsonProperty("deadLetterTargetArn", arn),
* jsonProperty("maxReceiveCount", 4)
* ))))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* q:
* type: aws:sqs:Queue
* properties:
* name: examplequeue
* ddl:
* type: aws:sqs:Queue
* properties:
* name: examplequeue-ddl
* redriveAllowPolicy:
* fn::toJSON:
* redrivePermission: byQueue
* sourceQueueArns:
* - ${q.arn}
* qRedrivePolicy:
* type: aws:sqs:RedrivePolicy
* name: q
* properties:
* queueUrl: ${q.id}
* redrivePolicy:
* fn::toJSON:
* deadLetterTargetArn: ${ddl.arn}
* maxReceiveCount: 4
* ```
*
* ## Import
* Using `pulumi import`, import SQS Queue Redrive Policies using the queue URL. For example:
* ```sh
* $ pulumi import aws:sqs/redrivePolicy:RedrivePolicy test https://queue.amazonaws.com/123456789012/myqueue
* ```
* @property queueUrl The URL of the SQS Queue to which to attach the policy
* @property redrivePolicy The JSON redrive policy for the SQS queue. Accepts two key/val pairs: `deadLetterTargetArn` and `maxReceiveCount`. Learn more in the [Amazon SQS dead-letter queues documentation](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/SQSDeveloperGuide/sqs-dead-letter-queues.html).
*/
public data class RedrivePolicyArgs(
public val queueUrl: Output? = null,
public val redrivePolicy: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.sqs.RedrivePolicyArgs =
com.pulumi.aws.sqs.RedrivePolicyArgs.builder()
.queueUrl(queueUrl?.applyValue({ args0 -> args0 }))
.redrivePolicy(redrivePolicy?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RedrivePolicyArgs].
*/
@PulumiTagMarker
public class RedrivePolicyArgsBuilder internal constructor() {
private var queueUrl: Output? = null
private var redrivePolicy: Output? = null
/**
* @param value The URL of the SQS Queue to which to attach the policy
*/
@JvmName("jfclislgqftaddep")
public suspend fun queueUrl(`value`: Output) {
this.queueUrl = value
}
/**
* @param value The JSON redrive policy for the SQS queue. Accepts two key/val pairs: `deadLetterTargetArn` and `maxReceiveCount`. Learn more in the [Amazon SQS dead-letter queues documentation](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/SQSDeveloperGuide/sqs-dead-letter-queues.html).
*/
@JvmName("uwtacpdnhsciotnb")
public suspend fun redrivePolicy(`value`: Output) {
this.redrivePolicy = value
}
/**
* @param value The URL of the SQS Queue to which to attach the policy
*/
@JvmName("ibcodxrifbygbsvy")
public suspend fun queueUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queueUrl = mapped
}
/**
* @param value The JSON redrive policy for the SQS queue. Accepts two key/val pairs: `deadLetterTargetArn` and `maxReceiveCount`. Learn more in the [Amazon SQS dead-letter queues documentation](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/SQSDeveloperGuide/sqs-dead-letter-queues.html).
*/
@JvmName("kasjbqlbjsuvafah")
public suspend fun redrivePolicy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.redrivePolicy = mapped
}
internal fun build(): RedrivePolicyArgs = RedrivePolicyArgs(
queueUrl = queueUrl,
redrivePolicy = redrivePolicy,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy