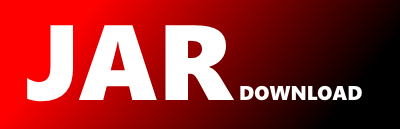
com.pulumi.aws.ssm.kotlin.AssociationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ssm.kotlin
import com.pulumi.aws.ssm.AssociationArgs.builder
import com.pulumi.aws.ssm.kotlin.inputs.AssociationOutputLocationArgs
import com.pulumi.aws.ssm.kotlin.inputs.AssociationOutputLocationArgsBuilder
import com.pulumi.aws.ssm.kotlin.inputs.AssociationTargetArgs
import com.pulumi.aws.ssm.kotlin.inputs.AssociationTargetArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Associates an SSM Document to an instance or EC2 tag.
* ## Example Usage
* ### Create an association for a specific instance
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.ssm.Association("example", {
* name: exampleAwsSsmDocument.name,
* targets: [{
* key: "InstanceIds",
* values: [exampleAwsInstance.id],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.ssm.Association("example",
* name=example_aws_ssm_document["name"],
* targets=[{
* "key": "InstanceIds",
* "values": [example_aws_instance["id"]],
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Ssm.Association("example", new()
* {
* Name = exampleAwsSsmDocument.Name,
* Targets = new[]
* {
* new Aws.Ssm.Inputs.AssociationTargetArgs
* {
* Key = "InstanceIds",
* Values = new[]
* {
* exampleAwsInstance.Id,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssm"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssm.NewAssociation(ctx, "example", &ssm.AssociationArgs{
* Name: pulumi.Any(exampleAwsSsmDocument.Name),
* Targets: ssm.AssociationTargetArray{
* &ssm.AssociationTargetArgs{
* Key: pulumi.String("InstanceIds"),
* Values: pulumi.StringArray{
* exampleAwsInstance.Id,
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssm.Association;
* import com.pulumi.aws.ssm.AssociationArgs;
* import com.pulumi.aws.ssm.inputs.AssociationTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Association("example", AssociationArgs.builder()
* .name(exampleAwsSsmDocument.name())
* .targets(AssociationTargetArgs.builder()
* .key("InstanceIds")
* .values(exampleAwsInstance.id())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ssm:Association
* properties:
* name: ${exampleAwsSsmDocument.name}
* targets:
* - key: InstanceIds
* values:
* - ${exampleAwsInstance.id}
* ```
*
* ### Create an association for all managed instances in an AWS account
* To target all managed instances in an AWS account, set the `key` as `"InstanceIds"` with `values` set as `["*"]`. This example also illustrates how to use an Amazon owned SSM document named `AmazonCloudWatch-ManageAgent`.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.ssm.Association("example", {
* name: "AmazonCloudWatch-ManageAgent",
* targets: [{
* key: "InstanceIds",
* values: ["*"],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.ssm.Association("example",
* name="AmazonCloudWatch-ManageAgent",
* targets=[{
* "key": "InstanceIds",
* "values": ["*"],
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Ssm.Association("example", new()
* {
* Name = "AmazonCloudWatch-ManageAgent",
* Targets = new[]
* {
* new Aws.Ssm.Inputs.AssociationTargetArgs
* {
* Key = "InstanceIds",
* Values = new[]
* {
* "*",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssm"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssm.NewAssociation(ctx, "example", &ssm.AssociationArgs{
* Name: pulumi.String("AmazonCloudWatch-ManageAgent"),
* Targets: ssm.AssociationTargetArray{
* &ssm.AssociationTargetArgs{
* Key: pulumi.String("InstanceIds"),
* Values: pulumi.StringArray{
* pulumi.String("*"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssm.Association;
* import com.pulumi.aws.ssm.AssociationArgs;
* import com.pulumi.aws.ssm.inputs.AssociationTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Association("example", AssociationArgs.builder()
* .name("AmazonCloudWatch-ManageAgent")
* .targets(AssociationTargetArgs.builder()
* .key("InstanceIds")
* .values("*")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ssm:Association
* properties:
* name: AmazonCloudWatch-ManageAgent
* targets:
* - key: InstanceIds
* values:
* - '*'
* ```
*
* ### Create an association for a specific tag
* This example shows how to target all managed instances that are assigned a tag key of `Environment` and value of `Development`.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.ssm.Association("example", {
* name: "AmazonCloudWatch-ManageAgent",
* targets: [{
* key: "tag:Environment",
* values: ["Development"],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.ssm.Association("example",
* name="AmazonCloudWatch-ManageAgent",
* targets=[{
* "key": "tag:Environment",
* "values": ["Development"],
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Ssm.Association("example", new()
* {
* Name = "AmazonCloudWatch-ManageAgent",
* Targets = new[]
* {
* new Aws.Ssm.Inputs.AssociationTargetArgs
* {
* Key = "tag:Environment",
* Values = new[]
* {
* "Development",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssm"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssm.NewAssociation(ctx, "example", &ssm.AssociationArgs{
* Name: pulumi.String("AmazonCloudWatch-ManageAgent"),
* Targets: ssm.AssociationTargetArray{
* &ssm.AssociationTargetArgs{
* Key: pulumi.String("tag:Environment"),
* Values: pulumi.StringArray{
* pulumi.String("Development"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssm.Association;
* import com.pulumi.aws.ssm.AssociationArgs;
* import com.pulumi.aws.ssm.inputs.AssociationTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Association("example", AssociationArgs.builder()
* .name("AmazonCloudWatch-ManageAgent")
* .targets(AssociationTargetArgs.builder()
* .key("tag:Environment")
* .values("Development")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ssm:Association
* properties:
* name: AmazonCloudWatch-ManageAgent
* targets:
* - key: tag:Environment
* values:
* - Development
* ```
*
* ### Create an association with a specific schedule
* This example shows how to schedule an association in various ways.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.ssm.Association("example", {
* name: exampleAwsSsmDocument.name,
* scheduleExpression: "cron(0 2 ? * SUN *)",
* targets: [{
* key: "InstanceIds",
* values: [exampleAwsInstance.id],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.ssm.Association("example",
* name=example_aws_ssm_document["name"],
* schedule_expression="cron(0 2 ? * SUN *)",
* targets=[{
* "key": "InstanceIds",
* "values": [example_aws_instance["id"]],
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Ssm.Association("example", new()
* {
* Name = exampleAwsSsmDocument.Name,
* ScheduleExpression = "cron(0 2 ? * SUN *)",
* Targets = new[]
* {
* new Aws.Ssm.Inputs.AssociationTargetArgs
* {
* Key = "InstanceIds",
* Values = new[]
* {
* exampleAwsInstance.Id,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssm"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssm.NewAssociation(ctx, "example", &ssm.AssociationArgs{
* Name: pulumi.Any(exampleAwsSsmDocument.Name),
* ScheduleExpression: pulumi.String("cron(0 2 ? * SUN *)"),
* Targets: ssm.AssociationTargetArray{
* &ssm.AssociationTargetArgs{
* Key: pulumi.String("InstanceIds"),
* Values: pulumi.StringArray{
* exampleAwsInstance.Id,
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssm.Association;
* import com.pulumi.aws.ssm.AssociationArgs;
* import com.pulumi.aws.ssm.inputs.AssociationTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Association("example", AssociationArgs.builder()
* .name(exampleAwsSsmDocument.name())
* .scheduleExpression("cron(0 2 ? * SUN *)")
* .targets(AssociationTargetArgs.builder()
* .key("InstanceIds")
* .values(exampleAwsInstance.id())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ssm:Association
* properties:
* name: ${exampleAwsSsmDocument.name}
* scheduleExpression: cron(0 2 ? * SUN *)
* targets:
* - key: InstanceIds
* values:
* - ${exampleAwsInstance.id}
* ```
*
* ## Import
* Using `pulumi import`, import SSM associations using the `association_id`. For example:
* ```sh
* $ pulumi import aws:ssm/association:Association test-association 10abcdef-0abc-1234-5678-90abcdef123456
* ```
* @property applyOnlyAtCronInterval By default, when you create a new or update associations, the system runs it immediately and then according to the schedule you specified. Enable this option if you do not want an association to run immediately after you create or update it. This parameter is not supported for rate expressions. Default: `false`.
* @property associationName The descriptive name for the association.
* @property automationTargetParameterName Specify the target for the association. This target is required for associations that use an `Automation` document and target resources by using rate controls. This should be set to the SSM document `parameter` that will define how your automation will branch out.
* @property complianceSeverity The compliance severity for the association. Can be one of the following: `UNSPECIFIED`, `LOW`, `MEDIUM`, `HIGH` or `CRITICAL`
* @property documentVersion The document version you want to associate with the target(s). Can be a specific version or the default version.
* @property instanceId The instance ID to apply an SSM document to. Use `targets` with key `InstanceIds` for document schema versions 2.0 and above. Use the `targets` attribute instead.
* @property maxConcurrency The maximum number of targets allowed to run the association at the same time. You can specify a number, for example 10, or a percentage of the target set, for example 10%.
* @property maxErrors The number of errors that are allowed before the system stops sending requests to run the association on additional targets. You can specify a number, for example 10, or a percentage of the target set, for example 10%. If you specify a threshold of 3, the stop command is sent when the fourth error is returned. If you specify a threshold of 10% for 50 associations, the stop command is sent when the sixth error is returned.
* @property name The name of the SSM document to apply.
* @property outputLocation An output location block. Output Location is documented below.
* @property parameters A block of arbitrary string parameters to pass to the SSM document.
* @property scheduleExpression A [cron or rate expression](https://docs.aws.amazon.com/systems-manager/latest/userguide/reference-cron-and-rate-expressions.html) that specifies when the association runs.
* @property syncCompliance The mode for generating association compliance. You can specify `AUTO` or `MANUAL`.
* @property tags A map of tags to assign to the object. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property targets A block containing the targets of the SSM association. Targets are documented below. AWS currently supports a maximum of 5 targets.
* @property waitForSuccessTimeoutSeconds The number of seconds to wait for the association status to be `Success`. If `Success` status is not reached within the given time, create opration will fail.
* Output Location (`output_location`) is an S3 bucket where you want to store the results of this association:
*/
public data class AssociationArgs(
public val applyOnlyAtCronInterval: Output? = null,
public val associationName: Output? = null,
public val automationTargetParameterName: Output? = null,
public val complianceSeverity: Output? = null,
public val documentVersion: Output? = null,
@Deprecated(
message = """
use 'targets' argument instead.
https://docs.aws.amazon.com/systems-manager/latest/APIReference/API_CreateAssociation.html#systemsmanager-CreateAssociation-request-InstanceId
""",
)
public val instanceId: Output? = null,
public val maxConcurrency: Output? = null,
public val maxErrors: Output? = null,
public val name: Output? = null,
public val outputLocation: Output? = null,
public val parameters: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy