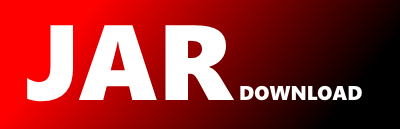
com.pulumi.aws.ssm.kotlin.Document.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ssm.kotlin
import com.pulumi.aws.ssm.kotlin.outputs.DocumentAttachmentsSource
import com.pulumi.aws.ssm.kotlin.outputs.DocumentParameter
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.ssm.kotlin.outputs.DocumentAttachmentsSource.Companion.toKotlin as documentAttachmentsSourceToKotlin
import com.pulumi.aws.ssm.kotlin.outputs.DocumentParameter.Companion.toKotlin as documentParameterToKotlin
/**
* Builder for [Document].
*/
@PulumiTagMarker
public class DocumentResourceBuilder internal constructor() {
public var name: String? = null
public var args: DocumentArgs = DocumentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend DocumentArgsBuilder.() -> Unit) {
val builder = DocumentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Document {
val builtJavaResource = com.pulumi.aws.ssm.Document(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Document(builtJavaResource)
}
}
/**
* Provides an SSM Document resource
* > **NOTE on updating SSM documents:** Only documents with a schema version of 2.0
* or greater can update their content once created, see [SSM Schema Features](http://docs.aws.amazon.com/systems-manager/latest/userguide/sysman-ssm-docs.html#document-schemas-features). To update a document with an older schema version you must recreate the resource. Not all document types support a schema version of 2.0 or greater. Refer to [SSM document schema features and examples](https://docs.aws.amazon.com/systems-manager/latest/userguide/document-schemas-features.html) for information about which schema versions are supported for the respective `document_type`.
* ## Example Usage
* ### Create an ssm document in JSON format
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const foo = new aws.ssm.Document("foo", {
* name: "test_document",
* documentType: "Command",
* content: ` {
* "schemaVersion": "1.2",
* "description": "Check ip configuration of a Linux instance.",
* "parameters": {
* },
* "runtimeConfig": {
* "aws:runShellScript": {
* "properties": [
* {
* "id": "0.aws:runShellScript",
* "runCommand": ["ifconfig"]
* }
* ]
* }
* }
* }
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* foo = aws.ssm.Document("foo",
* name="test_document",
* document_type="Command",
* content=""" {
* "schemaVersion": "1.2",
* "description": "Check ip configuration of a Linux instance.",
* "parameters": {
* },
* "runtimeConfig": {
* "aws:runShellScript": {
* "properties": [
* {
* "id": "0.aws:runShellScript",
* "runCommand": ["ifconfig"]
* }
* ]
* }
* }
* }
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var foo = new Aws.Ssm.Document("foo", new()
* {
* Name = "test_document",
* DocumentType = "Command",
* Content = @" {
* ""schemaVersion"": ""1.2"",
* ""description"": ""Check ip configuration of a Linux instance."",
* ""parameters"": {
* },
* ""runtimeConfig"": {
* ""aws:runShellScript"": {
* ""properties"": [
* {
* ""id"": ""0.aws:runShellScript"",
* ""runCommand"": [""ifconfig""]
* }
* ]
* }
* }
* }
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssm"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssm.NewDocument(ctx, "foo", &ssm.DocumentArgs{
* Name: pulumi.String("test_document"),
* DocumentType: pulumi.String("Command"),
* Content: pulumi.String(` {
* "schemaVersion": "1.2",
* "description": "Check ip configuration of a Linux instance.",
* "parameters": {
* },
* "runtimeConfig": {
* "aws:runShellScript": {
* "properties": [
* {
* "id": "0.aws:runShellScript",
* "runCommand": ["ifconfig"]
* }
* ]
* }
* }
* }
* `),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssm.Document;
* import com.pulumi.aws.ssm.DocumentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var foo = new Document("foo", DocumentArgs.builder()
* .name("test_document")
* .documentType("Command")
* .content("""
* {
* "schemaVersion": "1.2",
* "description": "Check ip configuration of a Linux instance.",
* "parameters": {
* },
* "runtimeConfig": {
* "aws:runShellScript": {
* "properties": [
* {
* "id": "0.aws:runShellScript",
* "runCommand": ["ifconfig"]
* }
* ]
* }
* }
* }
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foo:
* type: aws:ssm:Document
* properties:
* name: test_document
* documentType: Command
* content: |2
* {
* "schemaVersion": "1.2",
* "description": "Check ip configuration of a Linux instance.",
* "parameters": {
* },
* "runtimeConfig": {
* "aws:runShellScript": {
* "properties": [
* {
* "id": "0.aws:runShellScript",
* "runCommand": ["ifconfig"]
* }
* ]
* }
* }
* }
* ```
*
* ### Create an ssm document in YAML format
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const foo = new aws.ssm.Document("foo", {
* name: "test_document",
* documentFormat: "YAML",
* documentType: "Command",
* content: `schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* foo = aws.ssm.Document("foo",
* name="test_document",
* document_format="YAML",
* document_type="Command",
* content="""schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var foo = new Aws.Ssm.Document("foo", new()
* {
* Name = "test_document",
* DocumentFormat = "YAML",
* DocumentType = "Command",
* Content = @"schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssm"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssm.NewDocument(ctx, "foo", &ssm.DocumentArgs{
* Name: pulumi.String("test_document"),
* DocumentFormat: pulumi.String("YAML"),
* DocumentType: pulumi.String("Command"),
* Content: pulumi.String(`schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* `),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssm.Document;
* import com.pulumi.aws.ssm.DocumentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var foo = new Document("foo", DocumentArgs.builder()
* .name("test_document")
* .documentFormat("YAML")
* .documentType("Command")
* .content("""
* schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foo:
* type: aws:ssm:Document
* properties:
* name: test_document
* documentFormat: YAML
* documentType: Command
* content: |
* schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* ```
*
* ## Import
* Using `pulumi import`, import SSM Documents using the name. For example:
* ```sh
* $ pulumi import aws:ssm/document:Document example example
* ```
* The `attachments_source` argument does not have an SSM API method for reading the attachment information detail after creation. If the argument is set in the Pulumi program on an imported resource, Pulumi will always show a difference. To workaround this behavior, either omit the argument from the Pulumi program or use `ignore_changes` to hide the difference. For example:
*/
public class Document internal constructor(
override val javaResource: com.pulumi.aws.ssm.Document,
) : KotlinCustomResource(javaResource, DocumentMapper) {
/**
* The Amazon Resource Name (ARN) of the document.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* One or more configuration blocks describing attachments sources to a version of a document. See `attachments_source` block below for details.
*/
public val attachmentsSources: Output>?
get() = javaResource.attachmentsSources().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
documentAttachmentsSourceToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The content for the SSM document in JSON or YAML format. The content of the document must not exceed 64KB. This quota also includes the content specified for input parameters at runtime. We recommend storing the contents for your new document in an external JSON or YAML file and referencing the file in a command.
*/
public val content: Output
get() = javaResource.content().applyValue({ args0 -> args0 })
/**
* The date the document was created.
*/
public val createdDate: Output
get() = javaResource.createdDate().applyValue({ args0 -> args0 })
/**
* The default version of the document.
*/
public val defaultVersion: Output
get() = javaResource.defaultVersion().applyValue({ args0 -> args0 })
/**
* A description of what the parameter does, how to use it, the default value, and whether or not the parameter is optional.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* The format of the document. Valid values: `JSON`, `TEXT`, `YAML`.
*/
public val documentFormat: Output?
get() = javaResource.documentFormat().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The type of the document. For a list of valid values, see the [API Reference](https://docs.aws.amazon.com/systems-manager/latest/APIReference/API_CreateDocument.html#systemsmanager-CreateDocument-request-DocumentType).
*/
public val documentType: Output
get() = javaResource.documentType().applyValue({ args0 -> args0 })
/**
* The document version.
*/
public val documentVersion: Output
get() = javaResource.documentVersion().applyValue({ args0 -> args0 })
/**
* The Sha256 or Sha1 hash created by the system when the document was created.
*/
public val hash: Output
get() = javaResource.hash().applyValue({ args0 -> args0 })
/**
* The hash type of the document. Valid values: `Sha256`, `Sha1`.
*/
public val hashType: Output
get() = javaResource.hashType().applyValue({ args0 -> args0 })
/**
* The latest version of the document.
*/
public val latestVersion: Output
get() = javaResource.latestVersion().applyValue({ args0 -> args0 })
/**
* The name of the document.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The Amazon Web Services user that created the document.
*/
public val owner: Output
get() = javaResource.owner().applyValue({ args0 -> args0 })
/**
* One or more configuration blocks describing the parameters for the document. See `parameter` block below for details.
*/
public val parameters: Output>
get() = javaResource.parameters().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
documentParameterToKotlin(args0)
})
})
})
/**
* Additional permissions to attach to the document. See Permissions below for details.
*/
public val permissions: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy