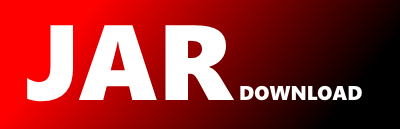
com.pulumi.aws.ssm.kotlin.DocumentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ssm.kotlin
import com.pulumi.aws.ssm.DocumentArgs.builder
import com.pulumi.aws.ssm.kotlin.inputs.DocumentAttachmentsSourceArgs
import com.pulumi.aws.ssm.kotlin.inputs.DocumentAttachmentsSourceArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides an SSM Document resource
* > **NOTE on updating SSM documents:** Only documents with a schema version of 2.0
* or greater can update their content once created, see [SSM Schema Features](http://docs.aws.amazon.com/systems-manager/latest/userguide/sysman-ssm-docs.html#document-schemas-features). To update a document with an older schema version you must recreate the resource. Not all document types support a schema version of 2.0 or greater. Refer to [SSM document schema features and examples](https://docs.aws.amazon.com/systems-manager/latest/userguide/document-schemas-features.html) for information about which schema versions are supported for the respective `document_type`.
* ## Example Usage
* ### Create an ssm document in JSON format
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const foo = new aws.ssm.Document("foo", {
* name: "test_document",
* documentType: "Command",
* content: ` {
* "schemaVersion": "1.2",
* "description": "Check ip configuration of a Linux instance.",
* "parameters": {
* },
* "runtimeConfig": {
* "aws:runShellScript": {
* "properties": [
* {
* "id": "0.aws:runShellScript",
* "runCommand": ["ifconfig"]
* }
* ]
* }
* }
* }
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* foo = aws.ssm.Document("foo",
* name="test_document",
* document_type="Command",
* content=""" {
* "schemaVersion": "1.2",
* "description": "Check ip configuration of a Linux instance.",
* "parameters": {
* },
* "runtimeConfig": {
* "aws:runShellScript": {
* "properties": [
* {
* "id": "0.aws:runShellScript",
* "runCommand": ["ifconfig"]
* }
* ]
* }
* }
* }
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var foo = new Aws.Ssm.Document("foo", new()
* {
* Name = "test_document",
* DocumentType = "Command",
* Content = @" {
* ""schemaVersion"": ""1.2"",
* ""description"": ""Check ip configuration of a Linux instance."",
* ""parameters"": {
* },
* ""runtimeConfig"": {
* ""aws:runShellScript"": {
* ""properties"": [
* {
* ""id"": ""0.aws:runShellScript"",
* ""runCommand"": [""ifconfig""]
* }
* ]
* }
* }
* }
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssm"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssm.NewDocument(ctx, "foo", &ssm.DocumentArgs{
* Name: pulumi.String("test_document"),
* DocumentType: pulumi.String("Command"),
* Content: pulumi.String(` {
* "schemaVersion": "1.2",
* "description": "Check ip configuration of a Linux instance.",
* "parameters": {
* },
* "runtimeConfig": {
* "aws:runShellScript": {
* "properties": [
* {
* "id": "0.aws:runShellScript",
* "runCommand": ["ifconfig"]
* }
* ]
* }
* }
* }
* `),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssm.Document;
* import com.pulumi.aws.ssm.DocumentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var foo = new Document("foo", DocumentArgs.builder()
* .name("test_document")
* .documentType("Command")
* .content("""
* {
* "schemaVersion": "1.2",
* "description": "Check ip configuration of a Linux instance.",
* "parameters": {
* },
* "runtimeConfig": {
* "aws:runShellScript": {
* "properties": [
* {
* "id": "0.aws:runShellScript",
* "runCommand": ["ifconfig"]
* }
* ]
* }
* }
* }
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foo:
* type: aws:ssm:Document
* properties:
* name: test_document
* documentType: Command
* content: |2
* {
* "schemaVersion": "1.2",
* "description": "Check ip configuration of a Linux instance.",
* "parameters": {
* },
* "runtimeConfig": {
* "aws:runShellScript": {
* "properties": [
* {
* "id": "0.aws:runShellScript",
* "runCommand": ["ifconfig"]
* }
* ]
* }
* }
* }
* ```
*
* ### Create an ssm document in YAML format
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const foo = new aws.ssm.Document("foo", {
* name: "test_document",
* documentFormat: "YAML",
* documentType: "Command",
* content: `schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* foo = aws.ssm.Document("foo",
* name="test_document",
* document_format="YAML",
* document_type="Command",
* content="""schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var foo = new Aws.Ssm.Document("foo", new()
* {
* Name = "test_document",
* DocumentFormat = "YAML",
* DocumentType = "Command",
* Content = @"schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssm"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssm.NewDocument(ctx, "foo", &ssm.DocumentArgs{
* Name: pulumi.String("test_document"),
* DocumentFormat: pulumi.String("YAML"),
* DocumentType: pulumi.String("Command"),
* Content: pulumi.String(`schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* `),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssm.Document;
* import com.pulumi.aws.ssm.DocumentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var foo = new Document("foo", DocumentArgs.builder()
* .name("test_document")
* .documentFormat("YAML")
* .documentType("Command")
* .content("""
* schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foo:
* type: aws:ssm:Document
* properties:
* name: test_document
* documentFormat: YAML
* documentType: Command
* content: |
* schemaVersion: '1.2'
* description: Check ip configuration of a Linux instance.
* parameters: {}
* runtimeConfig:
* 'aws:runShellScript':
* properties:
* - id: '0.aws:runShellScript'
* runCommand:
* - ifconfig
* ```
*
* ## Import
* Using `pulumi import`, import SSM Documents using the name. For example:
* ```sh
* $ pulumi import aws:ssm/document:Document example example
* ```
* The `attachments_source` argument does not have an SSM API method for reading the attachment information detail after creation. If the argument is set in the Pulumi program on an imported resource, Pulumi will always show a difference. To workaround this behavior, either omit the argument from the Pulumi program or use `ignore_changes` to hide the difference. For example:
* @property attachmentsSources One or more configuration blocks describing attachments sources to a version of a document. See `attachments_source` block below for details.
* @property content The content for the SSM document in JSON or YAML format. The content of the document must not exceed 64KB. This quota also includes the content specified for input parameters at runtime. We recommend storing the contents for your new document in an external JSON or YAML file and referencing the file in a command.
* @property documentFormat The format of the document. Valid values: `JSON`, `TEXT`, `YAML`.
* @property documentType The type of the document. For a list of valid values, see the [API Reference](https://docs.aws.amazon.com/systems-manager/latest/APIReference/API_CreateDocument.html#systemsmanager-CreateDocument-request-DocumentType).
* @property name The name of the document.
* @property permissions Additional permissions to attach to the document. See Permissions below for details.
* @property tags A map of tags to assign to the object. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property targetType The target type which defines the kinds of resources the document can run on. For example, `/AWS::EC2::Instance`. For a list of valid resource types, see [AWS resource and property types reference](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-template-resource-type-ref.html).
* @property versionName The version of the artifact associated with the document. For example, `12.6`. This value is unique across all versions of a document, and can't be changed.
*/
public data class DocumentArgs(
public val attachmentsSources: Output>? = null,
public val content: Output? = null,
public val documentFormat: Output? = null,
public val documentType: Output? = null,
public val name: Output? = null,
public val permissions: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy