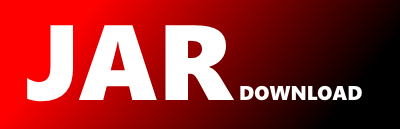
com.pulumi.aws.ssm.kotlin.MaintenanceWindow.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ssm.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [MaintenanceWindow].
*/
@PulumiTagMarker
public class MaintenanceWindowResourceBuilder internal constructor() {
public var name: String? = null
public var args: MaintenanceWindowArgs = MaintenanceWindowArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend MaintenanceWindowArgsBuilder.() -> Unit) {
val builder = MaintenanceWindowArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): MaintenanceWindow {
val builtJavaResource = com.pulumi.aws.ssm.MaintenanceWindow(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return MaintenanceWindow(builtJavaResource)
}
}
/**
* Provides an SSM Maintenance Window resource
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const production = new aws.ssm.MaintenanceWindow("production", {
* name: "maintenance-window-application",
* schedule: "cron(0 16 ? * TUE *)",
* duration: 3,
* cutoff: 1,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* production = aws.ssm.MaintenanceWindow("production",
* name="maintenance-window-application",
* schedule="cron(0 16 ? * TUE *)",
* duration=3,
* cutoff=1)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var production = new Aws.Ssm.MaintenanceWindow("production", new()
* {
* Name = "maintenance-window-application",
* Schedule = "cron(0 16 ? * TUE *)",
* Duration = 3,
* Cutoff = 1,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssm"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssm.NewMaintenanceWindow(ctx, "production", &ssm.MaintenanceWindowArgs{
* Name: pulumi.String("maintenance-window-application"),
* Schedule: pulumi.String("cron(0 16 ? * TUE *)"),
* Duration: pulumi.Int(3),
* Cutoff: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssm.MaintenanceWindow;
* import com.pulumi.aws.ssm.MaintenanceWindowArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var production = new MaintenanceWindow("production", MaintenanceWindowArgs.builder()
* .name("maintenance-window-application")
* .schedule("cron(0 16 ? * TUE *)")
* .duration(3)
* .cutoff(1)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* production:
* type: aws:ssm:MaintenanceWindow
* properties:
* name: maintenance-window-application
* schedule: cron(0 16 ? * TUE *)
* duration: 3
* cutoff: 1
* ```
*
* ## Import
* Using `pulumi import`, import SSM Maintenance Windows using the maintenance window `id`. For example:
* ```sh
* $ pulumi import aws:ssm/maintenanceWindow:MaintenanceWindow imported-window mw-0123456789
* ```
*/
public class MaintenanceWindow internal constructor(
override val javaResource: com.pulumi.aws.ssm.MaintenanceWindow,
) : KotlinCustomResource(javaResource, MaintenanceWindowMapper) {
/**
* Whether targets must be registered with the Maintenance Window before tasks can be defined for those targets.
*/
public val allowUnassociatedTargets: Output?
get() = javaResource.allowUnassociatedTargets().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The number of hours before the end of the Maintenance Window that Systems Manager stops scheduling new tasks for execution.
*/
public val cutoff: Output
get() = javaResource.cutoff().applyValue({ args0 -> args0 })
/**
* A description for the maintenance window.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The duration of the Maintenance Window in hours.
*/
public val duration: Output
get() = javaResource.duration().applyValue({ args0 -> args0 })
/**
* Whether the maintenance window is enabled. Default: `true`.
*/
public val enabled: Output?
get() = javaResource.enabled().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Timestamp in [ISO-8601 extended format](https://www.iso.org/iso-8601-date-and-time-format.html) when to no longer run the maintenance window.
*/
public val endDate: Output?
get() = javaResource.endDate().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The name of the maintenance window.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The schedule of the Maintenance Window in the form of a [cron or rate expression](https://docs.aws.amazon.com/systems-manager/latest/userguide/reference-cron-and-rate-expressions.html).
*/
public val schedule: Output
get() = javaResource.schedule().applyValue({ args0 -> args0 })
/**
* The number of days to wait after the date and time specified by a CRON expression before running the maintenance window.
*/
public val scheduleOffset: Output?
get() = javaResource.scheduleOffset().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Timezone for schedule in [Internet Assigned Numbers Authority (IANA) Time Zone Database format](https://www.iana.org/time-zones). For example: `America/Los_Angeles`, `etc/UTC`, or `Asia/Seoul`.
*/
public val scheduleTimezone: Output?
get() = javaResource.scheduleTimezone().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Timestamp in [ISO-8601 extended format](https://www.iso.org/iso-8601-date-and-time-format.html) when to begin the maintenance window.
*/
public val startDate: Output?
get() = javaResource.startDate().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* A map of tags to assign to the resource. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy