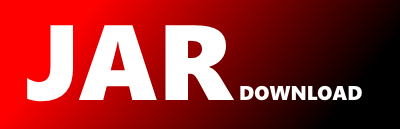
com.pulumi.aws.ssm.kotlin.Parameter.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ssm.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [Parameter].
*/
@PulumiTagMarker
public class ParameterResourceBuilder internal constructor() {
public var name: String? = null
public var args: ParameterArgs = ParameterArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ParameterArgsBuilder.() -> Unit) {
val builder = ParameterArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Parameter {
val builtJavaResource = com.pulumi.aws.ssm.Parameter(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Parameter(builtJavaResource)
}
}
/**
* Provides an SSM Parameter resource.
* > **Note:** `overwrite` also makes it possible to overwrite an existing SSM Parameter that's not created by the provider before. This argument has been deprecated and will be removed in v6.0.0 of the provider. For more information on how this affects the behavior of this resource, see this issue comment.
* ## Example Usage
* ### Basic example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const foo = new aws.ssm.Parameter("foo", {
* name: "foo",
* type: aws.ssm.ParameterType.String,
* value: "bar",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* foo = aws.ssm.Parameter("foo",
* name="foo",
* type=aws.ssm.ParameterType.STRING,
* value="bar")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var foo = new Aws.Ssm.Parameter("foo", new()
* {
* Name = "foo",
* Type = Aws.Ssm.ParameterType.String,
* Value = "bar",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssm"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssm.NewParameter(ctx, "foo", &ssm.ParameterArgs{
* Name: pulumi.String("foo"),
* Type: pulumi.String(ssm.ParameterTypeString),
* Value: pulumi.String("bar"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssm.Parameter;
* import com.pulumi.aws.ssm.ParameterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var foo = new Parameter("foo", ParameterArgs.builder()
* .name("foo")
* .type("String")
* .value("bar")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foo:
* type: aws:ssm:Parameter
* properties:
* name: foo
* type: String
* value: bar
* ```
*
* ### Encrypted string using default SSM KMS key
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const _default = new aws.rds.Instance("default", {
* allocatedStorage: 10,
* storageType: aws.rds.StorageType.GP2,
* engine: "mysql",
* engineVersion: "5.7.16",
* instanceClass: aws.rds.InstanceType.T2_Micro,
* dbName: "mydb",
* username: "foo",
* password: databaseMasterPassword,
* dbSubnetGroupName: "my_database_subnet_group",
* parameterGroupName: "default.mysql5.7",
* });
* const secret = new aws.ssm.Parameter("secret", {
* name: "/production/database/password/master",
* description: "The parameter description",
* type: aws.ssm.ParameterType.SecureString,
* value: databaseMasterPassword,
* tags: {
* environment: "production",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* default = aws.rds.Instance("default",
* allocated_storage=10,
* storage_type=aws.rds.StorageType.GP2,
* engine="mysql",
* engine_version="5.7.16",
* instance_class=aws.rds.InstanceType.T2_MICRO,
* db_name="mydb",
* username="foo",
* password=database_master_password,
* db_subnet_group_name="my_database_subnet_group",
* parameter_group_name="default.mysql5.7")
* secret = aws.ssm.Parameter("secret",
* name="/production/database/password/master",
* description="The parameter description",
* type=aws.ssm.ParameterType.SECURE_STRING,
* value=database_master_password,
* tags={
* "environment": "production",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Aws.Rds.Instance("default", new()
* {
* AllocatedStorage = 10,
* StorageType = Aws.Rds.StorageType.GP2,
* Engine = "mysql",
* EngineVersion = "5.7.16",
* InstanceClass = Aws.Rds.InstanceType.T2_Micro,
* DbName = "mydb",
* Username = "foo",
* Password = databaseMasterPassword,
* DbSubnetGroupName = "my_database_subnet_group",
* ParameterGroupName = "default.mysql5.7",
* });
* var secret = new Aws.Ssm.Parameter("secret", new()
* {
* Name = "/production/database/password/master",
* Description = "The parameter description",
* Type = Aws.Ssm.ParameterType.SecureString,
* Value = databaseMasterPassword,
* Tags =
* {
* { "environment", "production" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/rds"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssm"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := rds.NewInstance(ctx, "default", &rds.InstanceArgs{
* AllocatedStorage: pulumi.Int(10),
* StorageType: pulumi.String(rds.StorageTypeGP2),
* Engine: pulumi.String("mysql"),
* EngineVersion: pulumi.String("5.7.16"),
* InstanceClass: pulumi.String(rds.InstanceType_T2_Micro),
* DbName: pulumi.String("mydb"),
* Username: pulumi.String("foo"),
* Password: pulumi.Any(databaseMasterPassword),
* DbSubnetGroupName: pulumi.String("my_database_subnet_group"),
* ParameterGroupName: pulumi.String("default.mysql5.7"),
* })
* if err != nil {
* return err
* }
* _, err = ssm.NewParameter(ctx, "secret", &ssm.ParameterArgs{
* Name: pulumi.String("/production/database/password/master"),
* Description: pulumi.String("The parameter description"),
* Type: pulumi.String(ssm.ParameterTypeSecureString),
* Value: pulumi.Any(databaseMasterPassword),
* Tags: pulumi.StringMap{
* "environment": pulumi.String("production"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Instance;
* import com.pulumi.aws.rds.InstanceArgs;
* import com.pulumi.aws.ssm.Parameter;
* import com.pulumi.aws.ssm.ParameterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Instance("default", InstanceArgs.builder()
* .allocatedStorage(10)
* .storageType("gp2")
* .engine("mysql")
* .engineVersion("5.7.16")
* .instanceClass("db.t2.micro")
* .dbName("mydb")
* .username("foo")
* .password(databaseMasterPassword)
* .dbSubnetGroupName("my_database_subnet_group")
* .parameterGroupName("default.mysql5.7")
* .build());
* var secret = new Parameter("secret", ParameterArgs.builder()
* .name("/production/database/password/master")
* .description("The parameter description")
* .type("SecureString")
* .value(databaseMasterPassword)
* .tags(Map.of("environment", "production"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: aws:rds:Instance
* properties:
* allocatedStorage: 10
* storageType: gp2
* engine: mysql
* engineVersion: 5.7.16
* instanceClass: db.t2.micro
* dbName: mydb
* username: foo
* password: ${databaseMasterPassword}
* dbSubnetGroupName: my_database_subnet_group
* parameterGroupName: default.mysql5.7
* secret:
* type: aws:ssm:Parameter
* properties:
* name: /production/database/password/master
* description: The parameter description
* type: SecureString
* value: ${databaseMasterPassword}
* tags:
* environment: production
* ```
*
* ## Import
* Using `pulumi import`, import SSM Parameters using the parameter store `name`. For example:
* ```sh
* $ pulumi import aws:ssm/parameter:Parameter my_param /my_path/my_paramname
* ```
*/
public class Parameter internal constructor(
override val javaResource: com.pulumi.aws.ssm.Parameter,
) : KotlinCustomResource(javaResource, ParameterMapper) {
/**
* Regular expression used to validate the parameter value.
*/
public val allowedPattern: Output?
get() = javaResource.allowedPattern().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* ARN of the parameter.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Data type of the parameter. Valid values: `text`, `aws:ssm:integration` and `aws:ec2:image` for AMI format, see the [Native parameter support for Amazon Machine Image IDs](https://docs.aws.amazon.com/systems-manager/latest/userguide/parameter-store-ec2-aliases.html).
*/
public val dataType: Output
get() = javaResource.dataType().applyValue({ args0 -> args0 })
/**
* Description of the parameter.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Value of the parameter. **Use caution:** This value is _never_ marked as sensitive in the pulumi preview output. This argument is not valid with a `type` of `SecureString`.
*/
public val insecureValue: Output
get() = javaResource.insecureValue().applyValue({ args0 -> args0 })
/**
* KMS key ID or ARN for encrypting a SecureString.
*/
public val keyId: Output
get() = javaResource.keyId().applyValue({ args0 -> args0 })
/**
* Name of the parameter. If the name contains a path (e.g., any forward slashes (`/`)), it must be fully qualified with a leading forward slash (`/`). For additional requirements and constraints, see the [AWS SSM User Guide](https://docs.aws.amazon.com/systems-manager/latest/userguide/sysman-parameter-name-constraints.html).
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Overwrite an existing parameter. If not specified, defaults to `false` if the resource has not been created by Pulumi to avoid overwrite of existing resource, and will default to `true` otherwise (Pulumi lifecycle rules should then be used to manage the update behavior).
*/
@Deprecated(
message = """
this attribute has been deprecated
""",
)
public val overwrite: Output?
get() = javaResource.overwrite().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Map of tags to assign to the object. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy