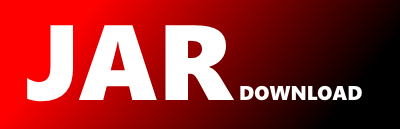
com.pulumi.aws.ssm.kotlin.inputs.MaintenanceWindowTaskTaskInvocationParametersRunCommandParametersArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ssm.kotlin.inputs
import com.pulumi.aws.ssm.inputs.MaintenanceWindowTaskTaskInvocationParametersRunCommandParametersArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property cloudwatchConfig Configuration options for sending command output to CloudWatch Logs. Documented below.
* @property comment Information about the command(s) to execute.
* @property documentHash The SHA-256 or SHA-1 hash created by the system when the document was created. SHA-1 hashes have been deprecated.
* @property documentHashType SHA-256 or SHA-1. SHA-1 hashes have been deprecated. Valid values: `Sha256` and `Sha1`
* @property documentVersion The version of an Automation document to use during task execution.
* @property notificationConfig Configurations for sending notifications about command status changes on a per-instance basis. Documented below.
* @property outputS3Bucket The name of the Amazon S3 bucket.
* @property outputS3KeyPrefix The Amazon S3 bucket subfolder.
* @property parameters The parameters for the RUN_COMMAND task execution. Documented below.
* @property serviceRoleArn The Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) service role to use to publish Amazon Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
* @property timeoutSeconds If this time is reached and the command has not already started executing, it doesn't run.
*/
public data class MaintenanceWindowTaskTaskInvocationParametersRunCommandParametersArgs(
public val cloudwatchConfig: Output? =
null,
public val comment: Output? = null,
public val documentHash: Output? = null,
public val documentHashType: Output? = null,
public val documentVersion: Output? = null,
public val notificationConfig: Output? =
null,
public val outputS3Bucket: Output? = null,
public val outputS3KeyPrefix: Output? = null,
public val parameters: Output>? =
null,
public val serviceRoleArn: Output? = null,
public val timeoutSeconds: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ssm.inputs.MaintenanceWindowTaskTaskInvocationParametersRunCommandParametersArgs =
com.pulumi.aws.ssm.inputs.MaintenanceWindowTaskTaskInvocationParametersRunCommandParametersArgs.builder()
.cloudwatchConfig(cloudwatchConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.comment(comment?.applyValue({ args0 -> args0 }))
.documentHash(documentHash?.applyValue({ args0 -> args0 }))
.documentHashType(documentHashType?.applyValue({ args0 -> args0 }))
.documentVersion(documentVersion?.applyValue({ args0 -> args0 }))
.notificationConfig(
notificationConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.outputS3Bucket(outputS3Bucket?.applyValue({ args0 -> args0 }))
.outputS3KeyPrefix(outputS3KeyPrefix?.applyValue({ args0 -> args0 }))
.parameters(
parameters?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.serviceRoleArn(serviceRoleArn?.applyValue({ args0 -> args0 }))
.timeoutSeconds(timeoutSeconds?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MaintenanceWindowTaskTaskInvocationParametersRunCommandParametersArgs].
*/
@PulumiTagMarker
public class MaintenanceWindowTaskTaskInvocationParametersRunCommandParametersArgsBuilder internal constructor() {
private var cloudwatchConfig:
Output? =
null
private var comment: Output? = null
private var documentHash: Output? = null
private var documentHashType: Output? = null
private var documentVersion: Output? = null
private var notificationConfig:
Output? =
null
private var outputS3Bucket: Output? = null
private var outputS3KeyPrefix: Output? = null
private var parameters:
Output>? =
null
private var serviceRoleArn: Output? = null
private var timeoutSeconds: Output? = null
/**
* @param value Configuration options for sending command output to CloudWatch Logs. Documented below.
*/
@JvmName("csxffsidxwceuliq")
public suspend fun cloudwatchConfig(`value`: Output) {
this.cloudwatchConfig = value
}
/**
* @param value Information about the command(s) to execute.
*/
@JvmName("ykkgthcjcurwdawr")
public suspend fun comment(`value`: Output) {
this.comment = value
}
/**
* @param value The SHA-256 or SHA-1 hash created by the system when the document was created. SHA-1 hashes have been deprecated.
*/
@JvmName("fxouiqcfwjjweyro")
public suspend fun documentHash(`value`: Output) {
this.documentHash = value
}
/**
* @param value SHA-256 or SHA-1. SHA-1 hashes have been deprecated. Valid values: `Sha256` and `Sha1`
*/
@JvmName("wxjtwgijgtjkymlm")
public suspend fun documentHashType(`value`: Output) {
this.documentHashType = value
}
/**
* @param value The version of an Automation document to use during task execution.
*/
@JvmName("qsxalvhruvfhoylw")
public suspend fun documentVersion(`value`: Output) {
this.documentVersion = value
}
/**
* @param value Configurations for sending notifications about command status changes on a per-instance basis. Documented below.
*/
@JvmName("aalyfkxhajcpjwnk")
public suspend fun notificationConfig(`value`: Output) {
this.notificationConfig = value
}
/**
* @param value The name of the Amazon S3 bucket.
*/
@JvmName("jolukwjtimyrtrwj")
public suspend fun outputS3Bucket(`value`: Output) {
this.outputS3Bucket = value
}
/**
* @param value The Amazon S3 bucket subfolder.
*/
@JvmName("hteuknuyynqdusky")
public suspend fun outputS3KeyPrefix(`value`: Output) {
this.outputS3KeyPrefix = value
}
/**
* @param value The parameters for the RUN_COMMAND task execution. Documented below.
*/
@JvmName("aexukwydmtuwqinx")
public suspend fun parameters(`value`: Output>) {
this.parameters = value
}
@JvmName("otoipdiwqjwdpubf")
public suspend fun parameters(vararg values: Output) {
this.parameters = Output.all(values.asList())
}
/**
* @param values The parameters for the RUN_COMMAND task execution. Documented below.
*/
@JvmName("ymlgdptlgmbdfgay")
public suspend fun parameters(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy