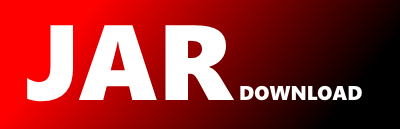
com.pulumi.aws.ssm.kotlin.inputs.PatchBaselineApprovalRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ssm.kotlin.inputs
import com.pulumi.aws.ssm.inputs.PatchBaselineApprovalRuleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property approveAfterDays Number of days after the release date of each patch matched by the rule the patch is marked as approved in the patch baseline. Valid Range: 0 to 360. Conflicts with `approve_until_date`.
* @property approveUntilDate Cutoff date for auto approval of released patches. Any patches released on or before this date are installed automatically. Date is formatted as `YYYY-MM-DD`. Conflicts with `approve_after_days`
* @property complianceLevel Compliance level for patches approved by this rule. Valid values are `CRITICAL`, `HIGH`, `MEDIUM`, `LOW`, `INFORMATIONAL`, and `UNSPECIFIED`. The default value is `UNSPECIFIED`.
* @property enableNonSecurity Boolean enabling the application of non-security updates. The default value is `false`. Valid for Linux instances only.
* @property patchFilters Patch filter group that defines the criteria for the rule. Up to 5 patch filters can be specified per approval rule using Key/Value pairs. Valid combinations of these Keys and the `operating_system` value can be found in the [SSM DescribePatchProperties API Reference](https://docs.aws.amazon.com/systems-manager/latest/APIReference/API_DescribePatchProperties.html). Valid Values are exact values for the patch property given as the key, or a wildcard `*`, which matches all values. `PATCH_SET` defaults to `OS` if unspecified
*/
public data class PatchBaselineApprovalRuleArgs(
public val approveAfterDays: Output? = null,
public val approveUntilDate: Output? = null,
public val complianceLevel: Output? = null,
public val enableNonSecurity: Output? = null,
public val patchFilters: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ssm.inputs.PatchBaselineApprovalRuleArgs =
com.pulumi.aws.ssm.inputs.PatchBaselineApprovalRuleArgs.builder()
.approveAfterDays(approveAfterDays?.applyValue({ args0 -> args0 }))
.approveUntilDate(approveUntilDate?.applyValue({ args0 -> args0 }))
.complianceLevel(complianceLevel?.applyValue({ args0 -> args0 }))
.enableNonSecurity(enableNonSecurity?.applyValue({ args0 -> args0 }))
.patchFilters(
patchFilters.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [PatchBaselineApprovalRuleArgs].
*/
@PulumiTagMarker
public class PatchBaselineApprovalRuleArgsBuilder internal constructor() {
private var approveAfterDays: Output? = null
private var approveUntilDate: Output? = null
private var complianceLevel: Output? = null
private var enableNonSecurity: Output? = null
private var patchFilters: Output>? = null
/**
* @param value Number of days after the release date of each patch matched by the rule the patch is marked as approved in the patch baseline. Valid Range: 0 to 360. Conflicts with `approve_until_date`.
*/
@JvmName("ubscewcgthpgsrym")
public suspend fun approveAfterDays(`value`: Output) {
this.approveAfterDays = value
}
/**
* @param value Cutoff date for auto approval of released patches. Any patches released on or before this date are installed automatically. Date is formatted as `YYYY-MM-DD`. Conflicts with `approve_after_days`
*/
@JvmName("umidptnoovaegnvd")
public suspend fun approveUntilDate(`value`: Output) {
this.approveUntilDate = value
}
/**
* @param value Compliance level for patches approved by this rule. Valid values are `CRITICAL`, `HIGH`, `MEDIUM`, `LOW`, `INFORMATIONAL`, and `UNSPECIFIED`. The default value is `UNSPECIFIED`.
*/
@JvmName("sewixhnjeoxitkwt")
public suspend fun complianceLevel(`value`: Output) {
this.complianceLevel = value
}
/**
* @param value Boolean enabling the application of non-security updates. The default value is `false`. Valid for Linux instances only.
*/
@JvmName("ihmmfcmqdeyayinx")
public suspend fun enableNonSecurity(`value`: Output) {
this.enableNonSecurity = value
}
/**
* @param value Patch filter group that defines the criteria for the rule. Up to 5 patch filters can be specified per approval rule using Key/Value pairs. Valid combinations of these Keys and the `operating_system` value can be found in the [SSM DescribePatchProperties API Reference](https://docs.aws.amazon.com/systems-manager/latest/APIReference/API_DescribePatchProperties.html). Valid Values are exact values for the patch property given as the key, or a wildcard `*`, which matches all values. `PATCH_SET` defaults to `OS` if unspecified
*/
@JvmName("vpdyumufudfbemvd")
public suspend fun patchFilters(`value`: Output>) {
this.patchFilters = value
}
@JvmName("lxfsrsfphuujexnt")
public suspend fun patchFilters(vararg values: Output) {
this.patchFilters = Output.all(values.asList())
}
/**
* @param values Patch filter group that defines the criteria for the rule. Up to 5 patch filters can be specified per approval rule using Key/Value pairs. Valid combinations of these Keys and the `operating_system` value can be found in the [SSM DescribePatchProperties API Reference](https://docs.aws.amazon.com/systems-manager/latest/APIReference/API_DescribePatchProperties.html). Valid Values are exact values for the patch property given as the key, or a wildcard `*`, which matches all values. `PATCH_SET` defaults to `OS` if unspecified
*/
@JvmName("opgecxhbxgtkpwtn")
public suspend fun patchFilters(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy