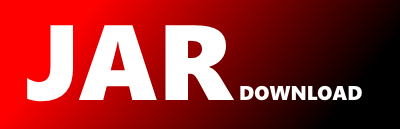
com.pulumi.aws.ssmincidents.kotlin.ResponsePlan.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ssmincidents.kotlin
import com.pulumi.aws.ssmincidents.kotlin.outputs.ResponsePlanAction
import com.pulumi.aws.ssmincidents.kotlin.outputs.ResponsePlanIncidentTemplate
import com.pulumi.aws.ssmincidents.kotlin.outputs.ResponsePlanIntegration
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.ssmincidents.kotlin.outputs.ResponsePlanAction.Companion.toKotlin as responsePlanActionToKotlin
import com.pulumi.aws.ssmincidents.kotlin.outputs.ResponsePlanIncidentTemplate.Companion.toKotlin as responsePlanIncidentTemplateToKotlin
import com.pulumi.aws.ssmincidents.kotlin.outputs.ResponsePlanIntegration.Companion.toKotlin as responsePlanIntegrationToKotlin
/**
* Builder for [ResponsePlan].
*/
@PulumiTagMarker
public class ResponsePlanResourceBuilder internal constructor() {
public var name: String? = null
public var args: ResponsePlanArgs = ResponsePlanArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ResponsePlanArgsBuilder.() -> Unit) {
val builder = ResponsePlanArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ResponsePlan {
val builtJavaResource = com.pulumi.aws.ssmincidents.ResponsePlan(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ResponsePlan(builtJavaResource)
}
}
/**
* Provides a resource to manage response plans in AWS Systems Manager Incident Manager.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.ssmincidents.ResponsePlan("example", {
* name: "name",
* incidentTemplate: {
* title: "title",
* impact: 3,
* },
* tags: {
* key: "value",
* },
* }, {
* dependsOn: [exampleAwsSsmincidentsReplicationSet],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.ssmincidents.ResponsePlan("example",
* name="name",
* incident_template={
* "title": "title",
* "impact": 3,
* },
* tags={
* "key": "value",
* },
* opts = pulumi.ResourceOptions(depends_on=[example_aws_ssmincidents_replication_set]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.SsmIncidents.ResponsePlan("example", new()
* {
* Name = "name",
* IncidentTemplate = new Aws.SsmIncidents.Inputs.ResponsePlanIncidentTemplateArgs
* {
* Title = "title",
* Impact = 3,
* },
* Tags =
* {
* { "key", "value" },
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleAwsSsmincidentsReplicationSet,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssmincidents"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssmincidents.NewResponsePlan(ctx, "example", &ssmincidents.ResponsePlanArgs{
* Name: pulumi.String("name"),
* IncidentTemplate: &ssmincidents.ResponsePlanIncidentTemplateArgs{
* Title: pulumi.String("title"),
* Impact: pulumi.Int(3),
* },
* Tags: pulumi.StringMap{
* "key": pulumi.String("value"),
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleAwsSsmincidentsReplicationSet,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssmincidents.ResponsePlan;
* import com.pulumi.aws.ssmincidents.ResponsePlanArgs;
* import com.pulumi.aws.ssmincidents.inputs.ResponsePlanIncidentTemplateArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResponsePlan("example", ResponsePlanArgs.builder()
* .name("name")
* .incidentTemplate(ResponsePlanIncidentTemplateArgs.builder()
* .title("title")
* .impact("3")
* .build())
* .tags(Map.of("key", "value"))
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleAwsSsmincidentsReplicationSet)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ssmincidents:ResponsePlan
* properties:
* name: name
* incidentTemplate:
* title: title
* impact: '3'
* tags:
* key: value
* options:
* dependson:
* - ${exampleAwsSsmincidentsReplicationSet}
* ```
*
* ### Usage With All Fields
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.ssmincidents.ResponsePlan("example", {
* name: "name",
* incidentTemplate: {
* title: "title",
* impact: 3,
* dedupeString: "dedupe",
* incidentTags: {
* key: "value",
* },
* notificationTargets: [
* {
* snsTopicArn: example1.arn,
* },
* {
* snsTopicArn: example2.arn,
* },
* ],
* summary: "summary",
* },
* displayName: "display name",
* chatChannels: [topic.arn],
* engagements: ["arn:aws:ssm-contacts:us-east-2:111122223333:contact/test1"],
* action: {
* ssmAutomations: [{
* documentName: document1.name,
* roleArn: role1.arn,
* documentVersion: "version1",
* targetAccount: "RESPONSE_PLAN_OWNER_ACCOUNT",
* parameters: [
* {
* name: "key",
* values: [
* "value1",
* "value2",
* ],
* },
* {
* name: "foo",
* values: ["bar"],
* },
* ],
* dynamicParameters: {
* someKey: "INVOLVED_RESOURCES",
* anotherKey: "INCIDENT_RECORD_ARN",
* },
* }],
* },
* integration: {
* pagerduties: [{
* name: "pagerdutyIntergration",
* serviceId: "example",
* secretId: "example",
* }],
* },
* tags: {
* key: "value",
* },
* }, {
* dependsOn: [exampleAwsSsmincidentsReplicationSet],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.ssmincidents.ResponsePlan("example",
* name="name",
* incident_template={
* "title": "title",
* "impact": 3,
* "dedupe_string": "dedupe",
* "incident_tags": {
* "key": "value",
* },
* "notification_targets": [
* {
* "sns_topic_arn": example1["arn"],
* },
* {
* "sns_topic_arn": example2["arn"],
* },
* ],
* "summary": "summary",
* },
* display_name="display name",
* chat_channels=[topic["arn"]],
* engagements=["arn:aws:ssm-contacts:us-east-2:111122223333:contact/test1"],
* action={
* "ssm_automations": [{
* "document_name": document1["name"],
* "role_arn": role1["arn"],
* "document_version": "version1",
* "target_account": "RESPONSE_PLAN_OWNER_ACCOUNT",
* "parameters": [
* {
* "name": "key",
* "values": [
* "value1",
* "value2",
* ],
* },
* {
* "name": "foo",
* "values": ["bar"],
* },
* ],
* "dynamic_parameters": {
* "someKey": "INVOLVED_RESOURCES",
* "anotherKey": "INCIDENT_RECORD_ARN",
* },
* }],
* },
* integration={
* "pagerduties": [{
* "name": "pagerdutyIntergration",
* "service_id": "example",
* "secret_id": "example",
* }],
* },
* tags={
* "key": "value",
* },
* opts = pulumi.ResourceOptions(depends_on=[example_aws_ssmincidents_replication_set]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.SsmIncidents.ResponsePlan("example", new()
* {
* Name = "name",
* IncidentTemplate = new Aws.SsmIncidents.Inputs.ResponsePlanIncidentTemplateArgs
* {
* Title = "title",
* Impact = 3,
* DedupeString = "dedupe",
* IncidentTags =
* {
* { "key", "value" },
* },
* NotificationTargets = new[]
* {
* new Aws.SsmIncidents.Inputs.ResponsePlanIncidentTemplateNotificationTargetArgs
* {
* SnsTopicArn = example1.Arn,
* },
* new Aws.SsmIncidents.Inputs.ResponsePlanIncidentTemplateNotificationTargetArgs
* {
* SnsTopicArn = example2.Arn,
* },
* },
* Summary = "summary",
* },
* DisplayName = "display name",
* ChatChannels = new[]
* {
* topic.Arn,
* },
* Engagements = new[]
* {
* "arn:aws:ssm-contacts:us-east-2:111122223333:contact/test1",
* },
* Action = new Aws.SsmIncidents.Inputs.ResponsePlanActionArgs
* {
* SsmAutomations = new[]
* {
* new Aws.SsmIncidents.Inputs.ResponsePlanActionSsmAutomationArgs
* {
* DocumentName = document1.Name,
* RoleArn = role1.Arn,
* DocumentVersion = "version1",
* TargetAccount = "RESPONSE_PLAN_OWNER_ACCOUNT",
* Parameters = new[]
* {
* new Aws.SsmIncidents.Inputs.ResponsePlanActionSsmAutomationParameterArgs
* {
* Name = "key",
* Values = new[]
* {
* "value1",
* "value2",
* },
* },
* new Aws.SsmIncidents.Inputs.ResponsePlanActionSsmAutomationParameterArgs
* {
* Name = "foo",
* Values = new[]
* {
* "bar",
* },
* },
* },
* DynamicParameters =
* {
* { "someKey", "INVOLVED_RESOURCES" },
* { "anotherKey", "INCIDENT_RECORD_ARN" },
* },
* },
* },
* },
* Integration = new Aws.SsmIncidents.Inputs.ResponsePlanIntegrationArgs
* {
* Pagerduties = new[]
* {
* new Aws.SsmIncidents.Inputs.ResponsePlanIntegrationPagerdutyArgs
* {
* Name = "pagerdutyIntergration",
* ServiceId = "example",
* SecretId = "example",
* },
* },
* },
* Tags =
* {
* { "key", "value" },
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleAwsSsmincidentsReplicationSet,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ssmincidents"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ssmincidents.NewResponsePlan(ctx, "example", &ssmincidents.ResponsePlanArgs{
* Name: pulumi.String("name"),
* IncidentTemplate: &ssmincidents.ResponsePlanIncidentTemplateArgs{
* Title: pulumi.String("title"),
* Impact: pulumi.Int(3),
* DedupeString: pulumi.String("dedupe"),
* IncidentTags: pulumi.StringMap{
* "key": pulumi.String("value"),
* },
* NotificationTargets: ssmincidents.ResponsePlanIncidentTemplateNotificationTargetArray{
* &ssmincidents.ResponsePlanIncidentTemplateNotificationTargetArgs{
* SnsTopicArn: pulumi.Any(example1.Arn),
* },
* &ssmincidents.ResponsePlanIncidentTemplateNotificationTargetArgs{
* SnsTopicArn: pulumi.Any(example2.Arn),
* },
* },
* Summary: pulumi.String("summary"),
* },
* DisplayName: pulumi.String("display name"),
* ChatChannels: pulumi.StringArray{
* topic.Arn,
* },
* Engagements: pulumi.StringArray{
* pulumi.String("arn:aws:ssm-contacts:us-east-2:111122223333:contact/test1"),
* },
* Action: &ssmincidents.ResponsePlanActionArgs{
* SsmAutomations: ssmincidents.ResponsePlanActionSsmAutomationArray{
* &ssmincidents.ResponsePlanActionSsmAutomationArgs{
* DocumentName: pulumi.Any(document1.Name),
* RoleArn: pulumi.Any(role1.Arn),
* DocumentVersion: pulumi.String("version1"),
* TargetAccount: pulumi.String("RESPONSE_PLAN_OWNER_ACCOUNT"),
* Parameters: ssmincidents.ResponsePlanActionSsmAutomationParameterArray{
* &ssmincidents.ResponsePlanActionSsmAutomationParameterArgs{
* Name: pulumi.String("key"),
* Values: pulumi.StringArray{
* pulumi.String("value1"),
* pulumi.String("value2"),
* },
* },
* &ssmincidents.ResponsePlanActionSsmAutomationParameterArgs{
* Name: pulumi.String("foo"),
* Values: pulumi.StringArray{
* pulumi.String("bar"),
* },
* },
* },
* DynamicParameters: pulumi.StringMap{
* "someKey": pulumi.String("INVOLVED_RESOURCES"),
* "anotherKey": pulumi.String("INCIDENT_RECORD_ARN"),
* },
* },
* },
* },
* Integration: &ssmincidents.ResponsePlanIntegrationArgs{
* Pagerduties: ssmincidents.ResponsePlanIntegrationPagerdutyArray{
* &ssmincidents.ResponsePlanIntegrationPagerdutyArgs{
* Name: pulumi.String("pagerdutyIntergration"),
* ServiceId: pulumi.String("example"),
* SecretId: pulumi.String("example"),
* },
* },
* },
* Tags: pulumi.StringMap{
* "key": pulumi.String("value"),
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleAwsSsmincidentsReplicationSet,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssmincidents.ResponsePlan;
* import com.pulumi.aws.ssmincidents.ResponsePlanArgs;
* import com.pulumi.aws.ssmincidents.inputs.ResponsePlanIncidentTemplateArgs;
* import com.pulumi.aws.ssmincidents.inputs.ResponsePlanActionArgs;
* import com.pulumi.aws.ssmincidents.inputs.ResponsePlanIntegrationArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResponsePlan("example", ResponsePlanArgs.builder()
* .name("name")
* .incidentTemplate(ResponsePlanIncidentTemplateArgs.builder()
* .title("title")
* .impact("3")
* .dedupeString("dedupe")
* .incidentTags(Map.of("key", "value"))
* .notificationTargets(
* ResponsePlanIncidentTemplateNotificationTargetArgs.builder()
* .snsTopicArn(example1.arn())
* .build(),
* ResponsePlanIncidentTemplateNotificationTargetArgs.builder()
* .snsTopicArn(example2.arn())
* .build())
* .summary("summary")
* .build())
* .displayName("display name")
* .chatChannels(topic.arn())
* .engagements("arn:aws:ssm-contacts:us-east-2:111122223333:contact/test1")
* .action(ResponsePlanActionArgs.builder()
* .ssmAutomations(ResponsePlanActionSsmAutomationArgs.builder()
* .documentName(document1.name())
* .roleArn(role1.arn())
* .documentVersion("version1")
* .targetAccount("RESPONSE_PLAN_OWNER_ACCOUNT")
* .parameters(
* ResponsePlanActionSsmAutomationParameterArgs.builder()
* .name("key")
* .values(
* "value1",
* "value2")
* .build(),
* ResponsePlanActionSsmAutomationParameterArgs.builder()
* .name("foo")
* .values("bar")
* .build())
* .dynamicParameters(Map.ofEntries(
* Map.entry("someKey", "INVOLVED_RESOURCES"),
* Map.entry("anotherKey", "INCIDENT_RECORD_ARN")
* ))
* .build())
* .build())
* .integration(ResponsePlanIntegrationArgs.builder()
* .pagerduties(ResponsePlanIntegrationPagerdutyArgs.builder()
* .name("pagerdutyIntergration")
* .serviceId("example")
* .secretId("example")
* .build())
* .build())
* .tags(Map.of("key", "value"))
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleAwsSsmincidentsReplicationSet)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ssmincidents:ResponsePlan
* properties:
* name: name
* incidentTemplate:
* title: title
* impact: '3'
* dedupeString: dedupe
* incidentTags:
* key: value
* notificationTargets:
* - snsTopicArn: ${example1.arn}
* - snsTopicArn: ${example2.arn}
* summary: summary
* displayName: display name
* chatChannels:
* - ${topic.arn}
* engagements:
* - arn:aws:ssm-contacts:us-east-2:111122223333:contact/test1
* action:
* ssmAutomations:
* - documentName: ${document1.name}
* roleArn: ${role1.arn}
* documentVersion: version1
* targetAccount: RESPONSE_PLAN_OWNER_ACCOUNT
* parameters:
* - name: key
* values:
* - value1
* - value2
* - name: foo
* values:
* - bar
* dynamicParameters:
* someKey: INVOLVED_RESOURCES
* anotherKey: INCIDENT_RECORD_ARN
* integration:
* pagerduties:
* - name: pagerdutyIntergration
* serviceId: example
* secretId: example
* tags:
* key: value
* options:
* dependson:
* - ${exampleAwsSsmincidentsReplicationSet}
* ```
*
* ## Import
* Using `pulumi import`, import an Incident Manager response plan using the response plan ARN. You can find the response plan ARN in the AWS Management Console. For example:
* ```sh
* $ pulumi import aws:ssmincidents/responsePlan:ResponsePlan responsePlanName ARNValue
* ```
*/
public class ResponsePlan internal constructor(
override val javaResource: com.pulumi.aws.ssmincidents.ResponsePlan,
) : KotlinCustomResource(javaResource, ResponsePlanMapper) {
public val action: Output?
get() = javaResource.action().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
responsePlanActionToKotlin(args0)
})
}).orElse(null)
})
/**
* The ARN of the response plan.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
public val chatChannels: Output>?
get() = javaResource.chatChannels().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
public val displayName: Output?
get() = javaResource.displayName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val engagements: Output>?
get() = javaResource.engagements().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
public val incidentTemplate: Output
get() = javaResource.incidentTemplate().applyValue({ args0 ->
args0.let({ args0 ->
responsePlanIncidentTemplateToKotlin(args0)
})
})
public val integration: Output?
get() = javaResource.integration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
responsePlanIntegrationToKotlin(args0)
})
}).orElse(null)
})
/**
* The name of the response plan.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy