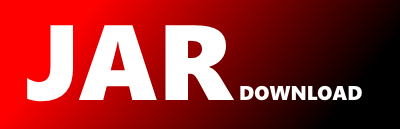
com.pulumi.aws.storagegateway.kotlin.Gateway.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.storagegateway.kotlin
import com.pulumi.aws.storagegateway.kotlin.outputs.GatewayGatewayNetworkInterface
import com.pulumi.aws.storagegateway.kotlin.outputs.GatewayMaintenanceStartTime
import com.pulumi.aws.storagegateway.kotlin.outputs.GatewaySmbActiveDirectorySettings
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.storagegateway.kotlin.outputs.GatewayGatewayNetworkInterface.Companion.toKotlin as gatewayGatewayNetworkInterfaceToKotlin
import com.pulumi.aws.storagegateway.kotlin.outputs.GatewayMaintenanceStartTime.Companion.toKotlin as gatewayMaintenanceStartTimeToKotlin
import com.pulumi.aws.storagegateway.kotlin.outputs.GatewaySmbActiveDirectorySettings.Companion.toKotlin as gatewaySmbActiveDirectorySettingsToKotlin
/**
* Builder for [Gateway].
*/
@PulumiTagMarker
public class GatewayResourceBuilder internal constructor() {
public var name: String? = null
public var args: GatewayArgs = GatewayArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend GatewayArgsBuilder.() -> Unit) {
val builder = GatewayArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Gateway {
val builtJavaResource = com.pulumi.aws.storagegateway.Gateway(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Gateway(builtJavaResource)
}
}
/**
* Manages an AWS Storage Gateway file, tape, or volume gateway in the provider region.
* > **NOTE:** The Storage Gateway API requires the gateway to be connected to properly return information after activation. If you are receiving `The specified gateway is not connected` errors during resource creation (gateway activation), ensure your gateway instance meets the [Storage Gateway requirements](https://docs.aws.amazon.com/storagegateway/latest/userguide/Requirements.html).
* ## Example Usage
* ### Local Cache
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const testVolumeAttachment = new aws.ec2.VolumeAttachment("test", {
* deviceName: "/dev/xvdb",
* volumeId: testAwsEbsVolume.id,
* instanceId: testAwsInstance.id,
* });
* const test = aws.storagegateway.getLocalDisk({
* diskNode: testAwsVolumeAttachment.deviceName,
* gatewayArn: testAwsStoragegatewayGateway.arn,
* });
* const testCache = new aws.storagegateway.Cache("test", {
* diskId: test.then(test => test.diskId),
* gatewayArn: testAwsStoragegatewayGateway.arn,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test_volume_attachment = aws.ec2.VolumeAttachment("test",
* device_name="/dev/xvdb",
* volume_id=test_aws_ebs_volume["id"],
* instance_id=test_aws_instance["id"])
* test = aws.storagegateway.get_local_disk(disk_node=test_aws_volume_attachment["deviceName"],
* gateway_arn=test_aws_storagegateway_gateway["arn"])
* test_cache = aws.storagegateway.Cache("test",
* disk_id=test.disk_id,
* gateway_arn=test_aws_storagegateway_gateway["arn"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var testVolumeAttachment = new Aws.Ec2.VolumeAttachment("test", new()
* {
* DeviceName = "/dev/xvdb",
* VolumeId = testAwsEbsVolume.Id,
* InstanceId = testAwsInstance.Id,
* });
* var test = Aws.StorageGateway.GetLocalDisk.Invoke(new()
* {
* DiskNode = testAwsVolumeAttachment.DeviceName,
* GatewayArn = testAwsStoragegatewayGateway.Arn,
* });
* var testCache = new Aws.StorageGateway.Cache("test", new()
* {
* DiskId = test.Apply(getLocalDiskResult => getLocalDiskResult.DiskId),
* GatewayArn = testAwsStoragegatewayGateway.Arn,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/storagegateway"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ec2.NewVolumeAttachment(ctx, "test", &ec2.VolumeAttachmentArgs{
* DeviceName: pulumi.String("/dev/xvdb"),
* VolumeId: pulumi.Any(testAwsEbsVolume.Id),
* InstanceId: pulumi.Any(testAwsInstance.Id),
* })
* if err != nil {
* return err
* }
* test, err := storagegateway.GetLocalDisk(ctx, &storagegateway.GetLocalDiskArgs{
* DiskNode: pulumi.StringRef(testAwsVolumeAttachment.DeviceName),
* GatewayArn: testAwsStoragegatewayGateway.Arn,
* }, nil)
* if err != nil {
* return err
* }
* _, err = storagegateway.NewCache(ctx, "test", &storagegateway.CacheArgs{
* DiskId: pulumi.String(test.DiskId),
* GatewayArn: pulumi.Any(testAwsStoragegatewayGateway.Arn),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.VolumeAttachment;
* import com.pulumi.aws.ec2.VolumeAttachmentArgs;
* import com.pulumi.aws.storagegateway.StoragegatewayFunctions;
* import com.pulumi.aws.storagegateway.inputs.GetLocalDiskArgs;
* import com.pulumi.aws.storagegateway.Cache;
* import com.pulumi.aws.storagegateway.CacheArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var testVolumeAttachment = new VolumeAttachment("testVolumeAttachment", VolumeAttachmentArgs.builder()
* .deviceName("/dev/xvdb")
* .volumeId(testAwsEbsVolume.id())
* .instanceId(testAwsInstance.id())
* .build());
* final var test = StoragegatewayFunctions.getLocalDisk(GetLocalDiskArgs.builder()
* .diskNode(testAwsVolumeAttachment.deviceName())
* .gatewayArn(testAwsStoragegatewayGateway.arn())
* .build());
* var testCache = new Cache("testCache", CacheArgs.builder()
* .diskId(test.applyValue(getLocalDiskResult -> getLocalDiskResult.diskId()))
* .gatewayArn(testAwsStoragegatewayGateway.arn())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* testVolumeAttachment:
* type: aws:ec2:VolumeAttachment
* name: test
* properties:
* deviceName: /dev/xvdb
* volumeId: ${testAwsEbsVolume.id}
* instanceId: ${testAwsInstance.id}
* testCache:
* type: aws:storagegateway:Cache
* name: test
* properties:
* diskId: ${test.diskId}
* gatewayArn: ${testAwsStoragegatewayGateway.arn}
* variables:
* test:
* fn::invoke:
* Function: aws:storagegateway:getLocalDisk
* Arguments:
* diskNode: ${testAwsVolumeAttachment.deviceName}
* gatewayArn: ${testAwsStoragegatewayGateway.arn}
* ```
*
* ### FSx File Gateway
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.storagegateway.Gateway("example", {
* gatewayIpAddress: "1.2.3.4",
* gatewayName: "example",
* gatewayTimezone: "GMT",
* gatewayType: "FILE_FSX_SMB",
* smbActiveDirectorySettings: {
* domainName: "corp.example.com",
* password: "avoid-plaintext-passwords",
* username: "Admin",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.storagegateway.Gateway("example",
* gateway_ip_address="1.2.3.4",
* gateway_name="example",
* gateway_timezone="GMT",
* gateway_type="FILE_FSX_SMB",
* smb_active_directory_settings={
* "domain_name": "corp.example.com",
* "password": "avoid-plaintext-passwords",
* "username": "Admin",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.StorageGateway.Gateway("example", new()
* {
* GatewayIpAddress = "1.2.3.4",
* GatewayName = "example",
* GatewayTimezone = "GMT",
* GatewayType = "FILE_FSX_SMB",
* SmbActiveDirectorySettings = new Aws.StorageGateway.Inputs.GatewaySmbActiveDirectorySettingsArgs
* {
* DomainName = "corp.example.com",
* Password = "avoid-plaintext-passwords",
* Username = "Admin",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/storagegateway"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storagegateway.NewGateway(ctx, "example", &storagegateway.GatewayArgs{
* GatewayIpAddress: pulumi.String("1.2.3.4"),
* GatewayName: pulumi.String("example"),
* GatewayTimezone: pulumi.String("GMT"),
* GatewayType: pulumi.String("FILE_FSX_SMB"),
* SmbActiveDirectorySettings: &storagegateway.GatewaySmbActiveDirectorySettingsArgs{
* DomainName: pulumi.String("corp.example.com"),
* Password: pulumi.String("avoid-plaintext-passwords"),
* Username: pulumi.String("Admin"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.storagegateway.Gateway;
* import com.pulumi.aws.storagegateway.GatewayArgs;
* import com.pulumi.aws.storagegateway.inputs.GatewaySmbActiveDirectorySettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Gateway("example", GatewayArgs.builder()
* .gatewayIpAddress("1.2.3.4")
* .gatewayName("example")
* .gatewayTimezone("GMT")
* .gatewayType("FILE_FSX_SMB")
* .smbActiveDirectorySettings(GatewaySmbActiveDirectorySettingsArgs.builder()
* .domainName("corp.example.com")
* .password("avoid-plaintext-passwords")
* .username("Admin")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:storagegateway:Gateway
* properties:
* gatewayIpAddress: 1.2.3.4
* gatewayName: example
* gatewayTimezone: GMT
* gatewayType: FILE_FSX_SMB
* smbActiveDirectorySettings:
* domainName: corp.example.com
* password: avoid-plaintext-passwords
* username: Admin
* ```
*
* ### S3 File Gateway
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.storagegateway.Gateway("example", {
* gatewayIpAddress: "1.2.3.4",
* gatewayName: "example",
* gatewayTimezone: "GMT",
* gatewayType: "FILE_S3",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.storagegateway.Gateway("example",
* gateway_ip_address="1.2.3.4",
* gateway_name="example",
* gateway_timezone="GMT",
* gateway_type="FILE_S3")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.StorageGateway.Gateway("example", new()
* {
* GatewayIpAddress = "1.2.3.4",
* GatewayName = "example",
* GatewayTimezone = "GMT",
* GatewayType = "FILE_S3",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/storagegateway"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storagegateway.NewGateway(ctx, "example", &storagegateway.GatewayArgs{
* GatewayIpAddress: pulumi.String("1.2.3.4"),
* GatewayName: pulumi.String("example"),
* GatewayTimezone: pulumi.String("GMT"),
* GatewayType: pulumi.String("FILE_S3"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.storagegateway.Gateway;
* import com.pulumi.aws.storagegateway.GatewayArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Gateway("example", GatewayArgs.builder()
* .gatewayIpAddress("1.2.3.4")
* .gatewayName("example")
* .gatewayTimezone("GMT")
* .gatewayType("FILE_S3")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:storagegateway:Gateway
* properties:
* gatewayIpAddress: 1.2.3.4
* gatewayName: example
* gatewayTimezone: GMT
* gatewayType: FILE_S3
* ```
*
* ### Tape Gateway
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.storagegateway.Gateway("example", {
* gatewayIpAddress: "1.2.3.4",
* gatewayName: "example",
* gatewayTimezone: "GMT",
* gatewayType: "VTL",
* mediumChangerType: "AWS-Gateway-VTL",
* tapeDriveType: "IBM-ULT3580-TD5",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.storagegateway.Gateway("example",
* gateway_ip_address="1.2.3.4",
* gateway_name="example",
* gateway_timezone="GMT",
* gateway_type="VTL",
* medium_changer_type="AWS-Gateway-VTL",
* tape_drive_type="IBM-ULT3580-TD5")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.StorageGateway.Gateway("example", new()
* {
* GatewayIpAddress = "1.2.3.4",
* GatewayName = "example",
* GatewayTimezone = "GMT",
* GatewayType = "VTL",
* MediumChangerType = "AWS-Gateway-VTL",
* TapeDriveType = "IBM-ULT3580-TD5",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/storagegateway"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storagegateway.NewGateway(ctx, "example", &storagegateway.GatewayArgs{
* GatewayIpAddress: pulumi.String("1.2.3.4"),
* GatewayName: pulumi.String("example"),
* GatewayTimezone: pulumi.String("GMT"),
* GatewayType: pulumi.String("VTL"),
* MediumChangerType: pulumi.String("AWS-Gateway-VTL"),
* TapeDriveType: pulumi.String("IBM-ULT3580-TD5"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.storagegateway.Gateway;
* import com.pulumi.aws.storagegateway.GatewayArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Gateway("example", GatewayArgs.builder()
* .gatewayIpAddress("1.2.3.4")
* .gatewayName("example")
* .gatewayTimezone("GMT")
* .gatewayType("VTL")
* .mediumChangerType("AWS-Gateway-VTL")
* .tapeDriveType("IBM-ULT3580-TD5")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:storagegateway:Gateway
* properties:
* gatewayIpAddress: 1.2.3.4
* gatewayName: example
* gatewayTimezone: GMT
* gatewayType: VTL
* mediumChangerType: AWS-Gateway-VTL
* tapeDriveType: IBM-ULT3580-TD5
* ```
*
* ### Volume Gateway (Cached)
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.storagegateway.Gateway("example", {
* gatewayIpAddress: "1.2.3.4",
* gatewayName: "example",
* gatewayTimezone: "GMT",
* gatewayType: "CACHED",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.storagegateway.Gateway("example",
* gateway_ip_address="1.2.3.4",
* gateway_name="example",
* gateway_timezone="GMT",
* gateway_type="CACHED")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.StorageGateway.Gateway("example", new()
* {
* GatewayIpAddress = "1.2.3.4",
* GatewayName = "example",
* GatewayTimezone = "GMT",
* GatewayType = "CACHED",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/storagegateway"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storagegateway.NewGateway(ctx, "example", &storagegateway.GatewayArgs{
* GatewayIpAddress: pulumi.String("1.2.3.4"),
* GatewayName: pulumi.String("example"),
* GatewayTimezone: pulumi.String("GMT"),
* GatewayType: pulumi.String("CACHED"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.storagegateway.Gateway;
* import com.pulumi.aws.storagegateway.GatewayArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Gateway("example", GatewayArgs.builder()
* .gatewayIpAddress("1.2.3.4")
* .gatewayName("example")
* .gatewayTimezone("GMT")
* .gatewayType("CACHED")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:storagegateway:Gateway
* properties:
* gatewayIpAddress: 1.2.3.4
* gatewayName: example
* gatewayTimezone: GMT
* gatewayType: CACHED
* ```
*
* ### Volume Gateway (Stored)
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.storagegateway.Gateway("example", {
* gatewayIpAddress: "1.2.3.4",
* gatewayName: "example",
* gatewayTimezone: "GMT",
* gatewayType: "STORED",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.storagegateway.Gateway("example",
* gateway_ip_address="1.2.3.4",
* gateway_name="example",
* gateway_timezone="GMT",
* gateway_type="STORED")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.StorageGateway.Gateway("example", new()
* {
* GatewayIpAddress = "1.2.3.4",
* GatewayName = "example",
* GatewayTimezone = "GMT",
* GatewayType = "STORED",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/storagegateway"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storagegateway.NewGateway(ctx, "example", &storagegateway.GatewayArgs{
* GatewayIpAddress: pulumi.String("1.2.3.4"),
* GatewayName: pulumi.String("example"),
* GatewayTimezone: pulumi.String("GMT"),
* GatewayType: pulumi.String("STORED"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.storagegateway.Gateway;
* import com.pulumi.aws.storagegateway.GatewayArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Gateway("example", GatewayArgs.builder()
* .gatewayIpAddress("1.2.3.4")
* .gatewayName("example")
* .gatewayTimezone("GMT")
* .gatewayType("STORED")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:storagegateway:Gateway
* properties:
* gatewayIpAddress: 1.2.3.4
* gatewayName: example
* gatewayTimezone: GMT
* gatewayType: STORED
* ```
*
* ## Import
* Using `pulumi import`, import `aws_storagegateway_gateway` using the gateway Amazon Resource Name (ARN). For example:
* ```sh
* $ pulumi import aws:storagegateway/gateway:Gateway example arn:aws:storagegateway:us-east-1:123456789012:gateway/sgw-12345678
* ```
* Certain resource arguments, like `gateway_ip_address` do not have a Storage Gateway API method for reading the information after creation, either omit the argument from the Pulumi program or use `ignore_changes` to hide the difference. For example:
*/
public class Gateway internal constructor(
override val javaResource: com.pulumi.aws.storagegateway.Gateway,
) : KotlinCustomResource(javaResource, GatewayMapper) {
/**
* Gateway activation key during resource creation. Conflicts with `gateway_ip_address`. Additional information is available in the [Storage Gateway User Guide](https://docs.aws.amazon.com/storagegateway/latest/userguide/get-activation-key.html).
*/
public val activationKey: Output
get() = javaResource.activationKey().applyValue({ args0 -> args0 })
/**
* Amazon Resource Name (ARN) of the gateway.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The average download bandwidth rate limit in bits per second. This is supported for the `CACHED`, `STORED`, and `VTL` gateway types.
*/
public val averageDownloadRateLimitInBitsPerSec: Output?
get() = javaResource.averageDownloadRateLimitInBitsPerSec().applyValue({ args0 ->
args0.map({ args0 -> args0 }).orElse(null)
})
/**
* The average upload bandwidth rate limit in bits per second. This is supported for the `CACHED`, `STORED`, and `VTL` gateway types.
*/
public val averageUploadRateLimitInBitsPerSec: Output?
get() = javaResource.averageUploadRateLimitInBitsPerSec().applyValue({ args0 ->
args0.map({ args0 -> args0 }).orElse(null)
})
/**
* The Amazon Resource Name (ARN) of the Amazon CloudWatch log group to use to monitor and log events in the gateway.
*/
public val cloudwatchLogGroupArn: Output?
get() = javaResource.cloudwatchLogGroupArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The ID of the Amazon EC2 instance that was used to launch the gateway.
*/
public val ec2InstanceId: Output
get() = javaResource.ec2InstanceId().applyValue({ args0 -> args0 })
/**
* The type of endpoint for your gateway.
*/
public val endpointType: Output
get() = javaResource.endpointType().applyValue({ args0 -> args0 })
/**
* Identifier of the gateway.
*/
public val gatewayId: Output
get() = javaResource.gatewayId().applyValue({ args0 -> args0 })
/**
* Gateway IP address to retrieve activation key during resource creation. Conflicts with `activation_key`. Gateway must be accessible on port 80 from where this provider is running. Additional information is available in the [Storage Gateway User Guide](https://docs.aws.amazon.com/storagegateway/latest/userguide/get-activation-key.html).
*/
public val gatewayIpAddress: Output
get() = javaResource.gatewayIpAddress().applyValue({ args0 -> args0 })
/**
* Name of the gateway.
*/
public val gatewayName: Output
get() = javaResource.gatewayName().applyValue({ args0 -> args0 })
/**
* An array that contains descriptions of the gateway network interfaces. See Gateway Network Interface.
*/
public val gatewayNetworkInterfaces: Output>
get() = javaResource.gatewayNetworkInterfaces().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> gatewayGatewayNetworkInterfaceToKotlin(args0) })
})
})
/**
* Time zone for the gateway. The time zone is of the format "GMT", "GMT-hr:mm", or "GMT+hr:mm". For example, `GMT-4:00` indicates the time is 4 hours behind GMT. The time zone is used, for example, for scheduling snapshots and your gateway's maintenance schedule.
*/
public val gatewayTimezone: Output
get() = javaResource.gatewayTimezone().applyValue({ args0 -> args0 })
/**
* Type of the gateway. The default value is `STORED`. Valid values: `CACHED`, `FILE_FSX_SMB`, `FILE_S3`, `STORED`, `VTL`.
*/
public val gatewayType: Output?
get() = javaResource.gatewayType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* VPC endpoint address to be used when activating your gateway. This should be used when your instance is in a private subnet. Requires HTTP access from client computer running this provider. More info on what ports are required by your VPC Endpoint Security group in [Activating a Gateway in a Virtual Private Cloud](https://docs.aws.amazon.com/storagegateway/latest/userguide/gateway-private-link.html).
*/
public val gatewayVpcEndpoint: Output?
get() = javaResource.gatewayVpcEndpoint().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The type of hypervisor environment used by the host.
*/
public val hostEnvironment: Output
get() = javaResource.hostEnvironment().applyValue({ args0 -> args0 })
/**
* The gateway's weekly maintenance start time information, including day and time of the week. The maintenance time is the time in your gateway's time zone. More details below.
*/
public val maintenanceStartTime: Output
get() = javaResource.maintenanceStartTime().applyValue({ args0 ->
args0.let({ args0 ->
gatewayMaintenanceStartTimeToKotlin(args0)
})
})
/**
* Type of medium changer to use for tape gateway. This provider cannot detect drift of this argument. Valid values: `STK-L700`, `AWS-Gateway-VTL`, `IBM-03584L32-0402`.
*/
public val mediumChangerType: Output?
get() = javaResource.mediumChangerType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Nested argument with Active Directory domain join information for Server Message Block (SMB) file shares. Only valid for `FILE_S3` and `FILE_FSX_SMB` gateway types. Must be set before creating `ActiveDirectory` authentication SMB file shares. More details below.
*/
public val smbActiveDirectorySettings: Output?
get() = javaResource.smbActiveDirectorySettings().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> gatewaySmbActiveDirectorySettingsToKotlin(args0) })
}).orElse(null)
})
/**
* Specifies whether the shares on this gateway appear when listing shares.
*/
public val smbFileShareVisibility: Output?
get() = javaResource.smbFileShareVisibility().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Guest password for Server Message Block (SMB) file shares. Only valid for `FILE_S3` and `FILE_FSX_SMB` gateway types. Must be set before creating `GuestAccess` authentication SMB file shares. This provider can only detect drift of the existence of a guest password, not its actual value from the gateway. This provider can however update the password with changing the argument.
*/
public val smbGuestPassword: Output?
get() = javaResource.smbGuestPassword().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies the type of security strategy. Valid values are: `ClientSpecified`, `MandatorySigning`, and `MandatoryEncryption`. See [Setting a Security Level for Your Gateway](https://docs.aws.amazon.com/storagegateway/latest/userguide/managing-gateway-file.html#security-strategy) for more information.
*/
public val smbSecurityStrategy: Output
get() = javaResource.smbSecurityStrategy().applyValue({ args0 -> args0 })
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy