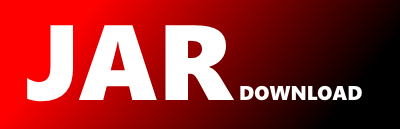
com.pulumi.aws.storagegateway.kotlin.StoragegatewayFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.storagegateway.kotlin
import com.pulumi.aws.storagegateway.StoragegatewayFunctions.getLocalDiskPlain
import com.pulumi.aws.storagegateway.kotlin.inputs.GetLocalDiskPlainArgs
import com.pulumi.aws.storagegateway.kotlin.inputs.GetLocalDiskPlainArgsBuilder
import com.pulumi.aws.storagegateway.kotlin.outputs.GetLocalDiskResult
import com.pulumi.aws.storagegateway.kotlin.outputs.GetLocalDiskResult.Companion.toKotlin
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
public object StoragegatewayFunctions {
/**
* Retrieve information about a Storage Gateway local disk. The disk identifier is useful for adding the disk as a cache or upload buffer to a gateway.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = aws.storagegateway.getLocalDisk({
* diskPath: testAwsVolumeAttachment.deviceName,
* gatewayArn: testAwsStoragegatewayGateway.arn,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test = aws.storagegateway.get_local_disk(disk_path=test_aws_volume_attachment["deviceName"],
* gateway_arn=test_aws_storagegateway_gateway["arn"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = Aws.StorageGateway.GetLocalDisk.Invoke(new()
* {
* DiskPath = testAwsVolumeAttachment.DeviceName,
* GatewayArn = testAwsStoragegatewayGateway.Arn,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/storagegateway"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storagegateway.GetLocalDisk(ctx, &storagegateway.GetLocalDiskArgs{
* DiskPath: pulumi.StringRef(testAwsVolumeAttachment.DeviceName),
* GatewayArn: testAwsStoragegatewayGateway.Arn,
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.storagegateway.StoragegatewayFunctions;
* import com.pulumi.aws.storagegateway.inputs.GetLocalDiskArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var test = StoragegatewayFunctions.getLocalDisk(GetLocalDiskArgs.builder()
* .diskPath(testAwsVolumeAttachment.deviceName())
* .gatewayArn(testAwsStoragegatewayGateway.arn())
* .build());
* }
* }
* ```
* ```yaml
* variables:
* test:
* fn::invoke:
* Function: aws:storagegateway:getLocalDisk
* Arguments:
* diskPath: ${testAwsVolumeAttachment.deviceName}
* gatewayArn: ${testAwsStoragegatewayGateway.arn}
* ```
*
* @param argument A collection of arguments for invoking getLocalDisk.
* @return A collection of values returned by getLocalDisk.
*/
public suspend fun getLocalDisk(argument: GetLocalDiskPlainArgs): GetLocalDiskResult =
toKotlin(getLocalDiskPlain(argument.toJava()).await())
/**
* @see [getLocalDisk].
* @param diskNode Device node of the local disk to retrieve. For example, `/dev/sdb`.
* @param diskPath Device path of the local disk to retrieve. For example, `/dev/xvdb` or `/dev/nvme1n1`.
* @param gatewayArn ARN of the gateway.
* @return A collection of values returned by getLocalDisk.
*/
public suspend fun getLocalDisk(
diskNode: String? = null,
diskPath: String? = null,
gatewayArn: String,
): GetLocalDiskResult {
val argument = GetLocalDiskPlainArgs(
diskNode = diskNode,
diskPath = diskPath,
gatewayArn = gatewayArn,
)
return toKotlin(getLocalDiskPlain(argument.toJava()).await())
}
/**
* @see [getLocalDisk].
* @param argument Builder for [com.pulumi.aws.storagegateway.kotlin.inputs.GetLocalDiskPlainArgs].
* @return A collection of values returned by getLocalDisk.
*/
public suspend fun getLocalDisk(argument: suspend GetLocalDiskPlainArgsBuilder.() -> Unit): GetLocalDiskResult {
val builder = GetLocalDiskPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return toKotlin(getLocalDiskPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy