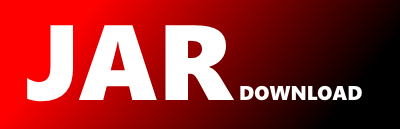
com.pulumi.aws.storagegateway.kotlin.inputs.GatewayMaintenanceStartTimeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.storagegateway.kotlin.inputs
import com.pulumi.aws.storagegateway.inputs.GatewayMaintenanceStartTimeArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property dayOfMonth The day of the month component of the maintenance start time represented as an ordinal number from 1 to 28, where 1 represents the first day of the month and 28 represents the last day of the month.
* @property dayOfWeek The day of the week component of the maintenance start time week represented as an ordinal number from 0 to 6, where 0 represents Sunday and 6 Saturday.
* @property hourOfDay The hour component of the maintenance start time represented as _hh_, where _hh_ is the hour (00 to 23). The hour of the day is in the time zone of the gateway.
* @property minuteOfHour The minute component of the maintenance start time represented as _mm_, where _mm_ is the minute (00 to 59). The minute of the hour is in the time zone of the gateway.
*/
public data class GatewayMaintenanceStartTimeArgs(
public val dayOfMonth: Output? = null,
public val dayOfWeek: Output? = null,
public val hourOfDay: Output,
public val minuteOfHour: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.storagegateway.inputs.GatewayMaintenanceStartTimeArgs =
com.pulumi.aws.storagegateway.inputs.GatewayMaintenanceStartTimeArgs.builder()
.dayOfMonth(dayOfMonth?.applyValue({ args0 -> args0 }))
.dayOfWeek(dayOfWeek?.applyValue({ args0 -> args0 }))
.hourOfDay(hourOfDay.applyValue({ args0 -> args0 }))
.minuteOfHour(minuteOfHour?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [GatewayMaintenanceStartTimeArgs].
*/
@PulumiTagMarker
public class GatewayMaintenanceStartTimeArgsBuilder internal constructor() {
private var dayOfMonth: Output? = null
private var dayOfWeek: Output? = null
private var hourOfDay: Output? = null
private var minuteOfHour: Output? = null
/**
* @param value The day of the month component of the maintenance start time represented as an ordinal number from 1 to 28, where 1 represents the first day of the month and 28 represents the last day of the month.
*/
@JvmName("rbcnrbjnnldbqdss")
public suspend fun dayOfMonth(`value`: Output) {
this.dayOfMonth = value
}
/**
* @param value The day of the week component of the maintenance start time week represented as an ordinal number from 0 to 6, where 0 represents Sunday and 6 Saturday.
*/
@JvmName("ympmcpkobrajbivy")
public suspend fun dayOfWeek(`value`: Output) {
this.dayOfWeek = value
}
/**
* @param value The hour component of the maintenance start time represented as _hh_, where _hh_ is the hour (00 to 23). The hour of the day is in the time zone of the gateway.
*/
@JvmName("wgtjpxlrnetrbmmn")
public suspend fun hourOfDay(`value`: Output) {
this.hourOfDay = value
}
/**
* @param value The minute component of the maintenance start time represented as _mm_, where _mm_ is the minute (00 to 59). The minute of the hour is in the time zone of the gateway.
*/
@JvmName("gnggsgxmfttseyig")
public suspend fun minuteOfHour(`value`: Output) {
this.minuteOfHour = value
}
/**
* @param value The day of the month component of the maintenance start time represented as an ordinal number from 1 to 28, where 1 represents the first day of the month and 28 represents the last day of the month.
*/
@JvmName("hcilqqrneksglbwg")
public suspend fun dayOfMonth(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dayOfMonth = mapped
}
/**
* @param value The day of the week component of the maintenance start time week represented as an ordinal number from 0 to 6, where 0 represents Sunday and 6 Saturday.
*/
@JvmName("xvlowqtayvhfigln")
public suspend fun dayOfWeek(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dayOfWeek = mapped
}
/**
* @param value The hour component of the maintenance start time represented as _hh_, where _hh_ is the hour (00 to 23). The hour of the day is in the time zone of the gateway.
*/
@JvmName("kodoxomxbftossyc")
public suspend fun hourOfDay(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.hourOfDay = mapped
}
/**
* @param value The minute component of the maintenance start time represented as _mm_, where _mm_ is the minute (00 to 59). The minute of the hour is in the time zone of the gateway.
*/
@JvmName("jnummdfqaljqjalx")
public suspend fun minuteOfHour(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minuteOfHour = mapped
}
internal fun build(): GatewayMaintenanceStartTimeArgs = GatewayMaintenanceStartTimeArgs(
dayOfMonth = dayOfMonth,
dayOfWeek = dayOfWeek,
hourOfDay = hourOfDay ?: throw PulumiNullFieldException("hourOfDay"),
minuteOfHour = minuteOfHour,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy