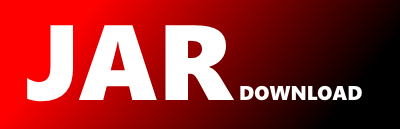
com.pulumi.aws.timestreaminfluxdb.kotlin.DbInstanceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.timestreaminfluxdb.kotlin
import com.pulumi.aws.timestreaminfluxdb.DbInstanceArgs.builder
import com.pulumi.aws.timestreaminfluxdb.kotlin.inputs.DbInstanceLogDeliveryConfigurationArgs
import com.pulumi.aws.timestreaminfluxdb.kotlin.inputs.DbInstanceLogDeliveryConfigurationArgsBuilder
import com.pulumi.aws.timestreaminfluxdb.kotlin.inputs.DbInstanceTimeoutsArgs
import com.pulumi.aws.timestreaminfluxdb.kotlin.inputs.DbInstanceTimeoutsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Resource for managing an Amazon Timestream for InfluxDB database instance.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.timestreaminfluxdb.DbInstance("example", {
* allocatedStorage: 20,
* bucket: "example-bucket-name",
* dbInstanceType: "db.influx.medium",
* username: "admin",
* password: "example-password",
* organization: "organization",
* vpcSubnetIds: [exampleid],
* vpcSecurityGroupIds: [exampleAwsSecurityGroup.id],
* name: "example-db-instance",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.timestreaminfluxdb.DbInstance("example",
* allocated_storage=20,
* bucket="example-bucket-name",
* db_instance_type="db.influx.medium",
* username="admin",
* password="example-password",
* organization="organization",
* vpc_subnet_ids=[exampleid],
* vpc_security_group_ids=[example_aws_security_group["id"]],
* name="example-db-instance")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.TimestreamInfluxDB.DbInstance("example", new()
* {
* AllocatedStorage = 20,
* Bucket = "example-bucket-name",
* DbInstanceType = "db.influx.medium",
* Username = "admin",
* Password = "example-password",
* Organization = "organization",
* VpcSubnetIds = new[]
* {
* exampleid,
* },
* VpcSecurityGroupIds = new[]
* {
* exampleAwsSecurityGroup.Id,
* },
* Name = "example-db-instance",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/timestreaminfluxdb"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := timestreaminfluxdb.NewDbInstance(ctx, "example", ×treaminfluxdb.DbInstanceArgs{
* AllocatedStorage: pulumi.Int(20),
* Bucket: pulumi.String("example-bucket-name"),
* DbInstanceType: pulumi.String("db.influx.medium"),
* Username: pulumi.String("admin"),
* Password: pulumi.String("example-password"),
* Organization: pulumi.String("organization"),
* VpcSubnetIds: pulumi.StringArray{
* exampleid,
* },
* VpcSecurityGroupIds: pulumi.StringArray{
* exampleAwsSecurityGroup.Id,
* },
* Name: pulumi.String("example-db-instance"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.timestreaminfluxdb.DbInstance;
* import com.pulumi.aws.timestreaminfluxdb.DbInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new DbInstance("example", DbInstanceArgs.builder()
* .allocatedStorage(20)
* .bucket("example-bucket-name")
* .dbInstanceType("db.influx.medium")
* .username("admin")
* .password("example-password")
* .organization("organization")
* .vpcSubnetIds(exampleid)
* .vpcSecurityGroupIds(exampleAwsSecurityGroup.id())
* .name("example-db-instance")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:timestreaminfluxdb:DbInstance
* properties:
* allocatedStorage: 20
* bucket: example-bucket-name
* dbInstanceType: db.influx.medium
* username: admin
* password: example-password
* organization: organization
* vpcSubnetIds:
* - ${exampleid}
* vpcSecurityGroupIds:
* - ${exampleAwsSecurityGroup.id}
* name: example-db-instance
* ```
*
* ### Usage with Prerequisite Resources
* All Timestream for InfluxDB instances require a VPC, subnet, and security group. The following example shows how these prerequisite resources can be created and used with `aws.timestreaminfluxdb.DbInstance`.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.ec2.Vpc("example", {cidrBlock: "10.0.0.0/16"});
* const exampleSubnet = new aws.ec2.Subnet("example", {
* vpcId: example.id,
* cidrBlock: "10.0.1.0/24",
* });
* const exampleSecurityGroup = new aws.ec2.SecurityGroup("example", {
* name: "example",
* vpcId: example.id,
* });
* const exampleDbInstance = new aws.timestreaminfluxdb.DbInstance("example", {
* allocatedStorage: 20,
* bucket: "example-bucket-name",
* dbInstanceType: "db.influx.medium",
* username: "admin",
* password: "example-password",
* organization: "organization",
* vpcSubnetIds: [exampleSubnet.id],
* vpcSecurityGroupIds: [exampleSecurityGroup.id],
* name: "example-db-instance",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.ec2.Vpc("example", cidr_block="10.0.0.0/16")
* example_subnet = aws.ec2.Subnet("example",
* vpc_id=example.id,
* cidr_block="10.0.1.0/24")
* example_security_group = aws.ec2.SecurityGroup("example",
* name="example",
* vpc_id=example.id)
* example_db_instance = aws.timestreaminfluxdb.DbInstance("example",
* allocated_storage=20,
* bucket="example-bucket-name",
* db_instance_type="db.influx.medium",
* username="admin",
* password="example-password",
* organization="organization",
* vpc_subnet_ids=[example_subnet.id],
* vpc_security_group_ids=[example_security_group.id],
* name="example-db-instance")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Ec2.Vpc("example", new()
* {
* CidrBlock = "10.0.0.0/16",
* });
* var exampleSubnet = new Aws.Ec2.Subnet("example", new()
* {
* VpcId = example.Id,
* CidrBlock = "10.0.1.0/24",
* });
* var exampleSecurityGroup = new Aws.Ec2.SecurityGroup("example", new()
* {
* Name = "example",
* VpcId = example.Id,
* });
* var exampleDbInstance = new Aws.TimestreamInfluxDB.DbInstance("example", new()
* {
* AllocatedStorage = 20,
* Bucket = "example-bucket-name",
* DbInstanceType = "db.influx.medium",
* Username = "admin",
* Password = "example-password",
* Organization = "organization",
* VpcSubnetIds = new[]
* {
* exampleSubnet.Id,
* },
* VpcSecurityGroupIds = new[]
* {
* exampleSecurityGroup.Id,
* },
* Name = "example-db-instance",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/timestreaminfluxdb"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := ec2.NewVpc(ctx, "example", &ec2.VpcArgs{
* CidrBlock: pulumi.String("10.0.0.0/16"),
* })
* if err != nil {
* return err
* }
* exampleSubnet, err := ec2.NewSubnet(ctx, "example", &ec2.SubnetArgs{
* VpcId: example.ID(),
* CidrBlock: pulumi.String("10.0.1.0/24"),
* })
* if err != nil {
* return err
* }
* exampleSecurityGroup, err := ec2.NewSecurityGroup(ctx, "example", &ec2.SecurityGroupArgs{
* Name: pulumi.String("example"),
* VpcId: example.ID(),
* })
* if err != nil {
* return err
* }
* _, err = timestreaminfluxdb.NewDbInstance(ctx, "example", ×treaminfluxdb.DbInstanceArgs{
* AllocatedStorage: pulumi.Int(20),
* Bucket: pulumi.String("example-bucket-name"),
* DbInstanceType: pulumi.String("db.influx.medium"),
* Username: pulumi.String("admin"),
* Password: pulumi.String("example-password"),
* Organization: pulumi.String("organization"),
* VpcSubnetIds: pulumi.StringArray{
* exampleSubnet.ID(),
* },
* VpcSecurityGroupIds: pulumi.StringArray{
* exampleSecurityGroup.ID(),
* },
* Name: pulumi.String("example-db-instance"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Vpc;
* import com.pulumi.aws.ec2.VpcArgs;
* import com.pulumi.aws.ec2.Subnet;
* import com.pulumi.aws.ec2.SubnetArgs;
* import com.pulumi.aws.ec2.SecurityGroup;
* import com.pulumi.aws.ec2.SecurityGroupArgs;
* import com.pulumi.aws.timestreaminfluxdb.DbInstance;
* import com.pulumi.aws.timestreaminfluxdb.DbInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Vpc("example", VpcArgs.builder()
* .cidrBlock("10.0.0.0/16")
* .build());
* var exampleSubnet = new Subnet("exampleSubnet", SubnetArgs.builder()
* .vpcId(example.id())
* .cidrBlock("10.0.1.0/24")
* .build());
* var exampleSecurityGroup = new SecurityGroup("exampleSecurityGroup", SecurityGroupArgs.builder()
* .name("example")
* .vpcId(example.id())
* .build());
* var exampleDbInstance = new DbInstance("exampleDbInstance", DbInstanceArgs.builder()
* .allocatedStorage(20)
* .bucket("example-bucket-name")
* .dbInstanceType("db.influx.medium")
* .username("admin")
* .password("example-password")
* .organization("organization")
* .vpcSubnetIds(exampleSubnet.id())
* .vpcSecurityGroupIds(exampleSecurityGroup.id())
* .name("example-db-instance")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ec2:Vpc
* properties:
* cidrBlock: 10.0.0.0/16
* exampleSubnet:
* type: aws:ec2:Subnet
* name: example
* properties:
* vpcId: ${example.id}
* cidrBlock: 10.0.1.0/24
* exampleSecurityGroup:
* type: aws:ec2:SecurityGroup
* name: example
* properties:
* name: example
* vpcId: ${example.id}
* exampleDbInstance:
* type: aws:timestreaminfluxdb:DbInstance
* name: example
* properties:
* allocatedStorage: 20
* bucket: example-bucket-name
* dbInstanceType: db.influx.medium
* username: admin
* password: example-password
* organization: organization
* vpcSubnetIds:
* - ${exampleSubnet.id}
* vpcSecurityGroupIds:
* - ${exampleSecurityGroup.id}
* name: example-db-instance
* ```
*
* ### Usage with S3 Log Delivery Enabled
* You can use an S3 bucket to store logs generated by your Timestream for InfluxDB instance. The following example shows what resources and arguments are required to configure an S3 bucket for logging, including the IAM policy that needs to be set in order to allow Timestream for InfluxDB to place logs in your S3 bucket. The configuration of the required VPC, security group, and subnet have been left out of the example for brevity.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const exampleBucketV2 = new aws.s3.BucketV2("example", {bucket: "example-s3-bucket"});
* const example = aws.iam.getPolicyDocumentOutput({
* statements: [{
* actions: ["s3:PutObject"],
* principals: [{
* type: "Service",
* identifiers: ["timestream-influxdb.amazonaws.com"],
* }],
* resources: [pulumi.interpolate`${exampleBucketV2.arn}/*`],
* }],
* });
* const exampleBucketPolicy = new aws.s3.BucketPolicy("example", {
* bucket: exampleBucketV2.id,
* policy: example.apply(example => example.json),
* });
* const exampleDbInstance = new aws.timestreaminfluxdb.DbInstance("example", {
* allocatedStorage: 20,
* bucket: "example-bucket-name",
* dbInstanceType: "db.influx.medium",
* username: "admin",
* password: "example-password",
* organization: "organization",
* vpcSubnetIds: [exampleAwsSubnet.id],
* vpcSecurityGroupIds: [exampleAwsSecurityGroup.id],
* name: "example-db-instance",
* logDeliveryConfiguration: {
* s3Configuration: {
* bucketName: exampleBucketV2.name,
* enabled: true,
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example_bucket_v2 = aws.s3.BucketV2("example", bucket="example-s3-bucket")
* example = aws.iam.get_policy_document_output(statements=[{
* "actions": ["s3:PutObject"],
* "principals": [{
* "type": "Service",
* "identifiers": ["timestream-influxdb.amazonaws.com"],
* }],
* "resources": [example_bucket_v2.arn.apply(lambda arn: f"{arn}/*")],
* }])
* example_bucket_policy = aws.s3.BucketPolicy("example",
* bucket=example_bucket_v2.id,
* policy=example.json)
* example_db_instance = aws.timestreaminfluxdb.DbInstance("example",
* allocated_storage=20,
* bucket="example-bucket-name",
* db_instance_type="db.influx.medium",
* username="admin",
* password="example-password",
* organization="organization",
* vpc_subnet_ids=[example_aws_subnet["id"]],
* vpc_security_group_ids=[example_aws_security_group["id"]],
* name="example-db-instance",
* log_delivery_configuration={
* "s3_configuration": {
* "bucket_name": example_bucket_v2.name,
* "enabled": True,
* },
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var exampleBucketV2 = new Aws.S3.BucketV2("example", new()
* {
* Bucket = "example-s3-bucket",
* });
* var example = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Actions = new[]
* {
* "s3:PutObject",
* },
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "Service",
* Identifiers = new[]
* {
* "timestream-influxdb.amazonaws.com",
* },
* },
* },
* Resources = new[]
* {
* $"{exampleBucketV2.Arn}/*",
* },
* },
* },
* });
* var exampleBucketPolicy = new Aws.S3.BucketPolicy("example", new()
* {
* Bucket = exampleBucketV2.Id,
* Policy = example.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var exampleDbInstance = new Aws.TimestreamInfluxDB.DbInstance("example", new()
* {
* AllocatedStorage = 20,
* Bucket = "example-bucket-name",
* DbInstanceType = "db.influx.medium",
* Username = "admin",
* Password = "example-password",
* Organization = "organization",
* VpcSubnetIds = new[]
* {
* exampleAwsSubnet.Id,
* },
* VpcSecurityGroupIds = new[]
* {
* exampleAwsSecurityGroup.Id,
* },
* Name = "example-db-instance",
* LogDeliveryConfiguration = new Aws.TimestreamInfluxDB.Inputs.DbInstanceLogDeliveryConfigurationArgs
* {
* S3Configuration = new Aws.TimestreamInfluxDB.Inputs.DbInstanceLogDeliveryConfigurationS3ConfigurationArgs
* {
* BucketName = exampleBucketV2.Name,
* Enabled = true,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/timestreaminfluxdb"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleBucketV2, err := s3.NewBucketV2(ctx, "example", &s3.BucketV2Args{
* Bucket: pulumi.String("example-s3-bucket"),
* })
* if err != nil {
* return err
* }
* example := iam.GetPolicyDocumentOutput(ctx, iam.GetPolicyDocumentOutputArgs{
* Statements: iam.GetPolicyDocumentStatementArray{
* &iam.GetPolicyDocumentStatementArgs{
* Actions: pulumi.StringArray{
* pulumi.String("s3:PutObject"),
* },
* Principals: iam.GetPolicyDocumentStatementPrincipalArray{
* &iam.GetPolicyDocumentStatementPrincipalArgs{
* Type: pulumi.String("Service"),
* Identifiers: pulumi.StringArray{
* pulumi.String("timestream-influxdb.amazonaws.com"),
* },
* },
* },
* Resources: pulumi.StringArray{
* exampleBucketV2.Arn.ApplyT(func(arn string) (string, error) {
* return fmt.Sprintf("%v/*", arn), nil
* }).(pulumi.StringOutput),
* },
* },
* },
* }, nil)
* _, err = s3.NewBucketPolicy(ctx, "example", &s3.BucketPolicyArgs{
* Bucket: exampleBucketV2.ID(),
* Policy: pulumi.String(example.ApplyT(func(example iam.GetPolicyDocumentResult) (*string, error) {
* return &example.Json, nil
* }).(pulumi.StringPtrOutput)),
* })
* if err != nil {
* return err
* }
* _, err = timestreaminfluxdb.NewDbInstance(ctx, "example", ×treaminfluxdb.DbInstanceArgs{
* AllocatedStorage: pulumi.Int(20),
* Bucket: pulumi.String("example-bucket-name"),
* DbInstanceType: pulumi.String("db.influx.medium"),
* Username: pulumi.String("admin"),
* Password: pulumi.String("example-password"),
* Organization: pulumi.String("organization"),
* VpcSubnetIds: pulumi.StringArray{
* exampleAwsSubnet.Id,
* },
* VpcSecurityGroupIds: pulumi.StringArray{
* exampleAwsSecurityGroup.Id,
* },
* Name: pulumi.String("example-db-instance"),
* LogDeliveryConfiguration: ×treaminfluxdb.DbInstanceLogDeliveryConfigurationArgs{
* S3Configuration: ×treaminfluxdb.DbInstanceLogDeliveryConfigurationS3ConfigurationArgs{
* BucketName: exampleBucketV2.Name,
* Enabled: pulumi.Bool(true),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.s3.BucketPolicy;
* import com.pulumi.aws.s3.BucketPolicyArgs;
* import com.pulumi.aws.timestreaminfluxdb.DbInstance;
* import com.pulumi.aws.timestreaminfluxdb.DbInstanceArgs;
* import com.pulumi.aws.timestreaminfluxdb.inputs.DbInstanceLogDeliveryConfigurationArgs;
* import com.pulumi.aws.timestreaminfluxdb.inputs.DbInstanceLogDeliveryConfigurationS3ConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleBucketV2 = new BucketV2("exampleBucketV2", BucketV2Args.builder()
* .bucket("example-s3-bucket")
* .build());
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .actions("s3:PutObject")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("timestream-influxdb.amazonaws.com")
* .build())
* .resources(exampleBucketV2.arn().applyValue(arn -> String.format("%s/*", arn)))
* .build())
* .build());
* var exampleBucketPolicy = new BucketPolicy("exampleBucketPolicy", BucketPolicyArgs.builder()
* .bucket(exampleBucketV2.id())
* .policy(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(example -> example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .build());
* var exampleDbInstance = new DbInstance("exampleDbInstance", DbInstanceArgs.builder()
* .allocatedStorage(20)
* .bucket("example-bucket-name")
* .dbInstanceType("db.influx.medium")
* .username("admin")
* .password("example-password")
* .organization("organization")
* .vpcSubnetIds(exampleAwsSubnet.id())
* .vpcSecurityGroupIds(exampleAwsSecurityGroup.id())
* .name("example-db-instance")
* .logDeliveryConfiguration(DbInstanceLogDeliveryConfigurationArgs.builder()
* .s3Configuration(DbInstanceLogDeliveryConfigurationS3ConfigurationArgs.builder()
* .bucketName(exampleBucketV2.name())
* .enabled(true)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleBucketV2:
* type: aws:s3:BucketV2
* name: example
* properties:
* bucket: example-s3-bucket
* exampleBucketPolicy:
* type: aws:s3:BucketPolicy
* name: example
* properties:
* bucket: ${exampleBucketV2.id}
* policy: ${example.json}
* exampleDbInstance:
* type: aws:timestreaminfluxdb:DbInstance
* name: example
* properties:
* allocatedStorage: 20
* bucket: example-bucket-name
* dbInstanceType: db.influx.medium
* username: admin
* password: example-password
* organization: organization
* vpcSubnetIds:
* - ${exampleAwsSubnet.id}
* vpcSecurityGroupIds:
* - ${exampleAwsSecurityGroup.id}
* name: example-db-instance
* logDeliveryConfiguration:
* s3Configuration:
* bucketName: ${exampleBucketV2.name}
* enabled: true
* variables:
* example:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - actions:
* - s3:PutObject
* principals:
* - type: Service
* identifiers:
* - timestream-influxdb.amazonaws.com
* resources:
* - ${exampleBucketV2.arn}/*
* ```
*
* ### Usage with MultiAZ Deployment
* To use multi-region availability, at least two subnets must be created in different availability zones and used with your Timestream for InfluxDB instance.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example1 = new aws.ec2.Subnet("example_1", {
* vpcId: exampleAwsVpc.id,
* cidrBlock: "10.0.1.0/24",
* availabilityZone: "us-west-2a",
* });
* const example2 = new aws.ec2.Subnet("example_2", {
* vpcId: exampleAwsVpc.id,
* cidrBlock: "10.0.2.0/24",
* availabilityZone: "us-west-2b",
* });
* const example = new aws.timestreaminfluxdb.DbInstance("example", {
* allocatedStorage: 20,
* bucket: "example-bucket-name",
* dbInstanceType: "db.influx.medium",
* deploymentType: "WITH_MULTIAZ_STANDBY",
* username: "admin",
* password: "example-password",
* organization: "organization",
* vpcSubnetIds: [
* example1.id,
* example2.id,
* ],
* vpcSecurityGroupIds: [exampleAwsSecurityGroup.id],
* name: "example-db-instance",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example1 = aws.ec2.Subnet("example_1",
* vpc_id=example_aws_vpc["id"],
* cidr_block="10.0.1.0/24",
* availability_zone="us-west-2a")
* example2 = aws.ec2.Subnet("example_2",
* vpc_id=example_aws_vpc["id"],
* cidr_block="10.0.2.0/24",
* availability_zone="us-west-2b")
* example = aws.timestreaminfluxdb.DbInstance("example",
* allocated_storage=20,
* bucket="example-bucket-name",
* db_instance_type="db.influx.medium",
* deployment_type="WITH_MULTIAZ_STANDBY",
* username="admin",
* password="example-password",
* organization="organization",
* vpc_subnet_ids=[
* example1.id,
* example2.id,
* ],
* vpc_security_group_ids=[example_aws_security_group["id"]],
* name="example-db-instance")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example1 = new Aws.Ec2.Subnet("example_1", new()
* {
* VpcId = exampleAwsVpc.Id,
* CidrBlock = "10.0.1.0/24",
* AvailabilityZone = "us-west-2a",
* });
* var example2 = new Aws.Ec2.Subnet("example_2", new()
* {
* VpcId = exampleAwsVpc.Id,
* CidrBlock = "10.0.2.0/24",
* AvailabilityZone = "us-west-2b",
* });
* var example = new Aws.TimestreamInfluxDB.DbInstance("example", new()
* {
* AllocatedStorage = 20,
* Bucket = "example-bucket-name",
* DbInstanceType = "db.influx.medium",
* DeploymentType = "WITH_MULTIAZ_STANDBY",
* Username = "admin",
* Password = "example-password",
* Organization = "organization",
* VpcSubnetIds = new[]
* {
* example1.Id,
* example2.Id,
* },
* VpcSecurityGroupIds = new[]
* {
* exampleAwsSecurityGroup.Id,
* },
* Name = "example-db-instance",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/timestreaminfluxdb"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example1, err := ec2.NewSubnet(ctx, "example_1", &ec2.SubnetArgs{
* VpcId: pulumi.Any(exampleAwsVpc.Id),
* CidrBlock: pulumi.String("10.0.1.0/24"),
* AvailabilityZone: pulumi.String("us-west-2a"),
* })
* if err != nil {
* return err
* }
* example2, err := ec2.NewSubnet(ctx, "example_2", &ec2.SubnetArgs{
* VpcId: pulumi.Any(exampleAwsVpc.Id),
* CidrBlock: pulumi.String("10.0.2.0/24"),
* AvailabilityZone: pulumi.String("us-west-2b"),
* })
* if err != nil {
* return err
* }
* _, err = timestreaminfluxdb.NewDbInstance(ctx, "example", ×treaminfluxdb.DbInstanceArgs{
* AllocatedStorage: pulumi.Int(20),
* Bucket: pulumi.String("example-bucket-name"),
* DbInstanceType: pulumi.String("db.influx.medium"),
* DeploymentType: pulumi.String("WITH_MULTIAZ_STANDBY"),
* Username: pulumi.String("admin"),
* Password: pulumi.String("example-password"),
* Organization: pulumi.String("organization"),
* VpcSubnetIds: pulumi.StringArray{
* example1.ID(),
* example2.ID(),
* },
* VpcSecurityGroupIds: pulumi.StringArray{
* exampleAwsSecurityGroup.Id,
* },
* Name: pulumi.String("example-db-instance"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Subnet;
* import com.pulumi.aws.ec2.SubnetArgs;
* import com.pulumi.aws.timestreaminfluxdb.DbInstance;
* import com.pulumi.aws.timestreaminfluxdb.DbInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example1 = new Subnet("example1", SubnetArgs.builder()
* .vpcId(exampleAwsVpc.id())
* .cidrBlock("10.0.1.0/24")
* .availabilityZone("us-west-2a")
* .build());
* var example2 = new Subnet("example2", SubnetArgs.builder()
* .vpcId(exampleAwsVpc.id())
* .cidrBlock("10.0.2.0/24")
* .availabilityZone("us-west-2b")
* .build());
* var example = new DbInstance("example", DbInstanceArgs.builder()
* .allocatedStorage(20)
* .bucket("example-bucket-name")
* .dbInstanceType("db.influx.medium")
* .deploymentType("WITH_MULTIAZ_STANDBY")
* .username("admin")
* .password("example-password")
* .organization("organization")
* .vpcSubnetIds(
* example1.id(),
* example2.id())
* .vpcSecurityGroupIds(exampleAwsSecurityGroup.id())
* .name("example-db-instance")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example1:
* type: aws:ec2:Subnet
* name: example_1
* properties:
* vpcId: ${exampleAwsVpc.id}
* cidrBlock: 10.0.1.0/24
* availabilityZone: us-west-2a
* example2:
* type: aws:ec2:Subnet
* name: example_2
* properties:
* vpcId: ${exampleAwsVpc.id}
* cidrBlock: 10.0.2.0/24
* availabilityZone: us-west-2b
* example:
* type: aws:timestreaminfluxdb:DbInstance
* properties:
* allocatedStorage: 20
* bucket: example-bucket-name
* dbInstanceType: db.influx.medium
* deploymentType: WITH_MULTIAZ_STANDBY
* username: admin
* password: example-password
* organization: organization
* vpcSubnetIds:
* - ${example1.id}
* - ${example2.id}
* vpcSecurityGroupIds:
* - ${exampleAwsSecurityGroup.id}
* name: example-db-instance
* ```
*
* ## Import
* Using `pulumi import`, import Timestream for InfluxDB Db Instance using its identifier. For example:
* ```sh
* $ pulumi import aws:timestreaminfluxdb/dbInstance:DbInstance example 12345abcde
* ```
* @property allocatedStorage Amount of storage in GiB (gibibytes). The minimum value is 20, the maximum value is 16384.
* @property bucket Name of the initial InfluxDB bucket. All InfluxDB data is stored in a bucket. A bucket combines the concept of a database and a retention period (the duration of time that each data point persists). A bucket belongs to an organization. Along with `organization`, `username`, and `password`, this argument will be stored in the secret referred to by the `influx_auth_parameters_secret_arn` attribute.
* @property dbInstanceType Timestream for InfluxDB DB instance type to run InfluxDB on. Valid options are: `"db.influx.medium"`, `"db.influx.large"`, `"db.influx.xlarge"`, `"db.influx.2xlarge"`, `"db.influx.4xlarge"`, `"db.influx.8xlarge"`, `"db.influx.12xlarge"`, and `"db.influx.16xlarge"`.
* @property dbParameterGroupIdentifier ID of the DB parameter group assigned to your DB instance. If added to an existing Timestream for InfluxDB instance or given a new value, will cause an in-place update to the instance. However, if an instance already has a value for `db_parameter_group_identifier`, removing `db_parameter_group_identifier` will cause the instance to be destroyed and recreated.
* @property dbStorageType Timestream for InfluxDB DB storage type to read and write InfluxDB data. You can choose between 3 different types of provisioned Influx IOPS included storage according to your workloads requirements: Influx IO Included 3000 IOPS, Influx IO Included 12000 IOPS, Influx IO Included 16000 IOPS. Valid options are: `"InfluxIOIncludedT1"`, `"InfluxIOIncludedT2"`, and `"InfluxIOIncludedT1"`. If you use `"InfluxIOIncludedT2" or "InfluxIOIncludedT3", the minimum value for `allocated_storage` is 400.
* @property deploymentType Specifies whether the DB instance will be deployed as a standalone instance or with a Multi-AZ standby for high availability. Valid options are: `"SINGLE_AZ"`, `"WITH_MULTIAZ_STANDBY"`.
* @property logDeliveryConfiguration Configuration for sending InfluxDB engine logs to a specified S3 bucket.
* @property name Name that uniquely identifies the DB instance when interacting with the Amazon Timestream for InfluxDB API and CLI commands. This name will also be a prefix included in the endpoint. DB instance names must be unique per customer and per region. The argument must start with a letter, cannot contain consecutive hyphens (`-`) and cannot end with a hyphen.
* @property organization Name of the initial organization for the initial admin user in InfluxDB. An InfluxDB organization is a workspace for a group of users. Along with `bucket`, `username`, and `password`, this argument will be stored in the secret referred to by the `influx_auth_parameters_secret_arn` attribute.
* @property password Password of the initial admin user created in InfluxDB. This password will allow you to access the InfluxDB UI to perform various administrative tasks and also use the InfluxDB CLI to create an operator token. Along with `bucket`, `username`, and `organization`, this argument will be stored in the secret referred to by the `influx_auth_parameters_secret_arn` attribute.
* @property publiclyAccessible Configures the DB instance with a public IP to facilitate access. Other resources, such as a VPC, a subnet, an internet gateway, and a route table with routes, are also required to enabled public access, in addition to this argument. See "Usage with Public Internet Access Enabled" for an example configuration with all required resources for public internet access.
* @property tags Map of tags assigned to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property timeouts
* @property username Username of the initial admin user created in InfluxDB. Must start with a letter and can't end with a hyphen or contain two consecutive hyphens. This username will allow you to access the InfluxDB UI to perform various administrative tasks and also use the InfluxDB CLI to create an operator token. Along with `bucket`, `organization`, and `password`, this argument will be stored in the secret referred to by the `influx_auth_parameters_secret_arn` attribute.
* @property vpcSecurityGroupIds List of VPC security group IDs to associate with the DB instance.
* @property vpcSubnetIds List of VPC subnet IDs to associate with the DB instance. Provide at least two VPC subnet IDs in different availability zones when deploying with a Multi-AZ standby.
* The following arguments are optional:
* */*/*/*/*/*/
*/
public data class DbInstanceArgs(
public val allocatedStorage: Output? = null,
public val bucket: Output? = null,
public val dbInstanceType: Output? = null,
public val dbParameterGroupIdentifier: Output? = null,
public val dbStorageType: Output? = null,
public val deploymentType: Output? = null,
public val logDeliveryConfiguration: Output? = null,
public val name: Output? = null,
public val organization: Output? = null,
public val password: Output? = null,
public val publiclyAccessible: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy