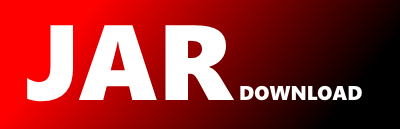
com.pulumi.aws.transfer.kotlin.ProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.transfer.kotlin
import com.pulumi.aws.transfer.ProfileArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides a AWS Transfer AS2 Profile resource.
* ## Example Usage
* ### Basic
*
* ```yaml
* resources:
* example:
* type: aws:transfer:Profile
* properties:
* as2Id: example
* certificateIds:
* - ${exampleAwsTransferCertificate.certificateId}
* usage: LOCAL
* ```
*
* ## Import
* Using `pulumi import`, import Transfer AS2 Profile using the `profile_id`. For example:
* ```sh
* $ pulumi import aws:transfer/profile:Profile example p-4221a88afd5f4362a
* ```
* @property as2Id The As2Id is the AS2 name as defined in the RFC 4130. For inbound ttransfers this is the AS2 From Header for the AS2 messages sent from the partner. For Outbound messages this is the AS2 To Header for the AS2 messages sent to the partner. his ID cannot include spaces.
* @property certificateIds The list of certificate Ids from the imported certificate operation.
* @property profileType The profile type should be LOCAL or PARTNER.
* @property tags A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public data class ProfileArgs(
public val as2Id: Output? = null,
public val certificateIds: Output>? = null,
public val profileType: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy