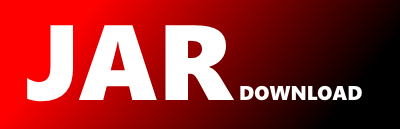
com.pulumi.aws.transfer.kotlin.Workflow.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.transfer.kotlin
import com.pulumi.aws.transfer.kotlin.outputs.WorkflowOnExceptionStep
import com.pulumi.aws.transfer.kotlin.outputs.WorkflowStep
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.transfer.kotlin.outputs.WorkflowOnExceptionStep.Companion.toKotlin as workflowOnExceptionStepToKotlin
import com.pulumi.aws.transfer.kotlin.outputs.WorkflowStep.Companion.toKotlin as workflowStepToKotlin
/**
* Builder for [Workflow].
*/
@PulumiTagMarker
public class WorkflowResourceBuilder internal constructor() {
public var name: String? = null
public var args: WorkflowArgs = WorkflowArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend WorkflowArgsBuilder.() -> Unit) {
val builder = WorkflowArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Workflow {
val builtJavaResource = com.pulumi.aws.transfer.Workflow(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Workflow(builtJavaResource)
}
}
/**
* Provides a AWS Transfer Workflow resource.
* ## Example Usage
* ### Basic single step example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.transfer.Workflow("example", {steps: [{
* deleteStepDetails: {
* name: "example",
* sourceFileLocation: "${original.file}",
* },
* type: "DELETE",
* }]});
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.transfer.Workflow("example", steps=[{
* "delete_step_details": {
* "name": "example",
* "source_file_location": "${original.file}",
* },
* "type": "DELETE",
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Transfer.Workflow("example", new()
* {
* Steps = new[]
* {
* new Aws.Transfer.Inputs.WorkflowStepArgs
* {
* DeleteStepDetails = new Aws.Transfer.Inputs.WorkflowStepDeleteStepDetailsArgs
* {
* Name = "example",
* SourceFileLocation = "${original.file}",
* },
* Type = "DELETE",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/transfer"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := transfer.NewWorkflow(ctx, "example", &transfer.WorkflowArgs{
* Steps: transfer.WorkflowStepArray{
* &transfer.WorkflowStepArgs{
* DeleteStepDetails: &transfer.WorkflowStepDeleteStepDetailsArgs{
* Name: pulumi.String("example"),
* SourceFileLocation: pulumi.String("${original.file}"),
* },
* Type: pulumi.String("DELETE"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.transfer.Workflow;
* import com.pulumi.aws.transfer.WorkflowArgs;
* import com.pulumi.aws.transfer.inputs.WorkflowStepArgs;
* import com.pulumi.aws.transfer.inputs.WorkflowStepDeleteStepDetailsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Workflow("example", WorkflowArgs.builder()
* .steps(WorkflowStepArgs.builder()
* .deleteStepDetails(WorkflowStepDeleteStepDetailsArgs.builder()
* .name("example")
* .sourceFileLocation("${original.file}")
* .build())
* .type("DELETE")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:transfer:Workflow
* properties:
* steps:
* - deleteStepDetails:
* name: example
* sourceFileLocation: ${original.file}
* type: DELETE
* ```
*
* ### Multistep example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.transfer.Workflow("example", {steps: [
* {
* customStepDetails: {
* name: "example",
* sourceFileLocation: "${original.file}",
* target: exampleAwsLambdaFunction.arn,
* timeoutSeconds: 60,
* },
* type: "CUSTOM",
* },
* {
* tagStepDetails: {
* name: "example",
* sourceFileLocation: "${original.file}",
* tags: [{
* key: "Name",
* value: "Hello World",
* }],
* },
* type: "TAG",
* },
* ]});
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.transfer.Workflow("example", steps=[
* {
* "custom_step_details": {
* "name": "example",
* "source_file_location": "${original.file}",
* "target": example_aws_lambda_function["arn"],
* "timeout_seconds": 60,
* },
* "type": "CUSTOM",
* },
* {
* "tag_step_details": {
* "name": "example",
* "source_file_location": "${original.file}",
* "tags": [{
* "key": "Name",
* "value": "Hello World",
* }],
* },
* "type": "TAG",
* },
* ])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Transfer.Workflow("example", new()
* {
* Steps = new[]
* {
* new Aws.Transfer.Inputs.WorkflowStepArgs
* {
* CustomStepDetails = new Aws.Transfer.Inputs.WorkflowStepCustomStepDetailsArgs
* {
* Name = "example",
* SourceFileLocation = "${original.file}",
* Target = exampleAwsLambdaFunction.Arn,
* TimeoutSeconds = 60,
* },
* Type = "CUSTOM",
* },
* new Aws.Transfer.Inputs.WorkflowStepArgs
* {
* TagStepDetails = new Aws.Transfer.Inputs.WorkflowStepTagStepDetailsArgs
* {
* Name = "example",
* SourceFileLocation = "${original.file}",
* Tags = new[]
* {
* new Aws.Transfer.Inputs.WorkflowStepTagStepDetailsTagArgs
* {
* Key = "Name",
* Value = "Hello World",
* },
* },
* },
* Type = "TAG",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/transfer"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := transfer.NewWorkflow(ctx, "example", &transfer.WorkflowArgs{
* Steps: transfer.WorkflowStepArray{
* &transfer.WorkflowStepArgs{
* CustomStepDetails: &transfer.WorkflowStepCustomStepDetailsArgs{
* Name: pulumi.String("example"),
* SourceFileLocation: pulumi.String("${original.file}"),
* Target: pulumi.Any(exampleAwsLambdaFunction.Arn),
* TimeoutSeconds: pulumi.Int(60),
* },
* Type: pulumi.String("CUSTOM"),
* },
* &transfer.WorkflowStepArgs{
* TagStepDetails: &transfer.WorkflowStepTagStepDetailsArgs{
* Name: pulumi.String("example"),
* SourceFileLocation: pulumi.String("${original.file}"),
* Tags: transfer.WorkflowStepTagStepDetailsTagArray{
* &transfer.WorkflowStepTagStepDetailsTagArgs{
* Key: pulumi.String("Name"),
* Value: pulumi.String("Hello World"),
* },
* },
* },
* Type: pulumi.String("TAG"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.transfer.Workflow;
* import com.pulumi.aws.transfer.WorkflowArgs;
* import com.pulumi.aws.transfer.inputs.WorkflowStepArgs;
* import com.pulumi.aws.transfer.inputs.WorkflowStepCustomStepDetailsArgs;
* import com.pulumi.aws.transfer.inputs.WorkflowStepTagStepDetailsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Workflow("example", WorkflowArgs.builder()
* .steps(
* WorkflowStepArgs.builder()
* .customStepDetails(WorkflowStepCustomStepDetailsArgs.builder()
* .name("example")
* .sourceFileLocation("${original.file}")
* .target(exampleAwsLambdaFunction.arn())
* .timeoutSeconds(60)
* .build())
* .type("CUSTOM")
* .build(),
* WorkflowStepArgs.builder()
* .tagStepDetails(WorkflowStepTagStepDetailsArgs.builder()
* .name("example")
* .sourceFileLocation("${original.file}")
* .tags(WorkflowStepTagStepDetailsTagArgs.builder()
* .key("Name")
* .value("Hello World")
* .build())
* .build())
* .type("TAG")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:transfer:Workflow
* properties:
* steps:
* - customStepDetails:
* name: example
* sourceFileLocation: ${original.file}
* target: ${exampleAwsLambdaFunction.arn}
* timeoutSeconds: 60
* type: CUSTOM
* - tagStepDetails:
* name: example
* sourceFileLocation: ${original.file}
* tags:
* - key: Name
* value: Hello World
* type: TAG
* ```
*
* ## Import
* Using `pulumi import`, import Transfer Workflows using the `worflow_id`. For example:
* ```sh
* $ pulumi import aws:transfer/workflow:Workflow example example
* ```
*/
public class Workflow internal constructor(
override val javaResource: com.pulumi.aws.transfer.Workflow,
) : KotlinCustomResource(javaResource, WorkflowMapper) {
/**
* The Workflow ARN.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* A textual description for the workflow.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies the steps (actions) to take if errors are encountered during execution of the workflow. See Workflow Steps below.
*/
public val onExceptionSteps: Output>?
get() = javaResource.onExceptionSteps().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
workflowOnExceptionStepToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Specifies the details for the steps that are in the specified workflow. See Workflow Steps below.
*/
public val steps: Output>
get() = javaResource.steps().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
workflowStepToKotlin(args0)
})
})
})
/**
* A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy