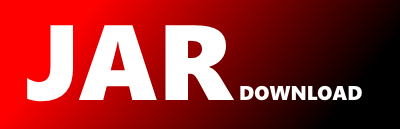
com.pulumi.aws.vpc.kotlin.EndpointServicePrivateDnsVerificationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.vpc.kotlin
import com.pulumi.aws.vpc.EndpointServicePrivateDnsVerificationArgs.builder
import com.pulumi.aws.vpc.kotlin.inputs.EndpointServicePrivateDnsVerificationTimeoutsArgs
import com.pulumi.aws.vpc.kotlin.inputs.EndpointServicePrivateDnsVerificationTimeoutsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Resource for managing an AWS VPC (Virtual Private Cloud) Endpoint Service Private DNS Verification.
* This resource begins the verification process by calling the [`StartVpcEndpointServicePrivateDnsVerification`](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_StartVpcEndpointServicePrivateDnsVerification.html) API.
* The service provider should add a record to the DNS server _before_ creating this resource.
* For additional details, refer to the AWS documentation on [managing VPC endpoint service DNS names](https://docs.aws.amazon.com/vpc/latest/privatelink/manage-dns-names.html).
* > Destruction of this resource will not stop the verification process, only remove the resource from state.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.vpc.EndpointServicePrivateDnsVerification("example", {serviceId: exampleAwsVpcEndpointService.id});
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.vpc.EndpointServicePrivateDnsVerification("example", service_id=example_aws_vpc_endpoint_service["id"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Vpc.EndpointServicePrivateDnsVerification("example", new()
* {
* ServiceId = exampleAwsVpcEndpointService.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/vpc"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := vpc.NewEndpointServicePrivateDnsVerification(ctx, "example", &vpc.EndpointServicePrivateDnsVerificationArgs{
* ServiceId: pulumi.Any(exampleAwsVpcEndpointService.Id),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.vpc.EndpointServicePrivateDnsVerification;
* import com.pulumi.aws.vpc.EndpointServicePrivateDnsVerificationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new EndpointServicePrivateDnsVerification("example", EndpointServicePrivateDnsVerificationArgs.builder()
* .serviceId(exampleAwsVpcEndpointService.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:vpc:EndpointServicePrivateDnsVerification
* properties:
* serviceId: ${exampleAwsVpcEndpointService.id}
* ```
*
* ## Import
* You cannot import this resource.
* @property serviceId ID of the endpoint service.
* The following arguments are optional:
* @property timeouts
* @property waitForVerification Whether to wait until the endpoint service returns a `Verified` status for the configured private DNS name.
*/
public data class EndpointServicePrivateDnsVerificationArgs(
public val serviceId: Output? = null,
public val timeouts: Output? = null,
public val waitForVerification: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.vpc.EndpointServicePrivateDnsVerificationArgs =
com.pulumi.aws.vpc.EndpointServicePrivateDnsVerificationArgs.builder()
.serviceId(serviceId?.applyValue({ args0 -> args0 }))
.timeouts(timeouts?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.waitForVerification(waitForVerification?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EndpointServicePrivateDnsVerificationArgs].
*/
@PulumiTagMarker
public class EndpointServicePrivateDnsVerificationArgsBuilder internal constructor() {
private var serviceId: Output? = null
private var timeouts: Output? = null
private var waitForVerification: Output? = null
/**
* @param value ID of the endpoint service.
* The following arguments are optional:
*/
@JvmName("klcauqkerlrnigrc")
public suspend fun serviceId(`value`: Output) {
this.serviceId = value
}
/**
* @param value
*/
@JvmName("yigiujgqntshfbqu")
public suspend fun timeouts(`value`: Output) {
this.timeouts = value
}
/**
* @param value Whether to wait until the endpoint service returns a `Verified` status for the configured private DNS name.
*/
@JvmName("mdfshyekrfisasuh")
public suspend fun waitForVerification(`value`: Output) {
this.waitForVerification = value
}
/**
* @param value ID of the endpoint service.
* The following arguments are optional:
*/
@JvmName("sjxmvnanbwrshclq")
public suspend fun serviceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceId = mapped
}
/**
* @param value
*/
@JvmName("dpknvycmhhxokayt")
public suspend fun timeouts(`value`: EndpointServicePrivateDnsVerificationTimeoutsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeouts = mapped
}
/**
* @param argument
*/
@JvmName("gpslprasdyapauly")
public suspend fun timeouts(argument: suspend EndpointServicePrivateDnsVerificationTimeoutsArgsBuilder.() -> Unit) {
val toBeMapped = EndpointServicePrivateDnsVerificationTimeoutsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.timeouts = mapped
}
/**
* @param value Whether to wait until the endpoint service returns a `Verified` status for the configured private DNS name.
*/
@JvmName("mkhrnhxkwywwqhpp")
public suspend fun waitForVerification(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.waitForVerification = mapped
}
internal fun build(): EndpointServicePrivateDnsVerificationArgs =
EndpointServicePrivateDnsVerificationArgs(
serviceId = serviceId,
timeouts = timeouts,
waitForVerification = waitForVerification,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy