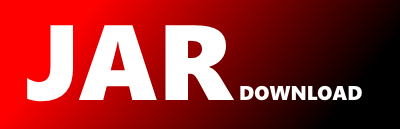
com.pulumi.aws.vpclattice.kotlin.inputs.TargetGroupConfigHealthCheckArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.vpclattice.kotlin.inputs
import com.pulumi.aws.vpclattice.inputs.TargetGroupConfigHealthCheckArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property enabled Indicates whether health checking is enabled. Defaults to `true`.
* @property healthCheckIntervalSeconds The approximate amount of time, in seconds, between health checks of an individual target. The range is 5–300 seconds. The default is 30 seconds.
* @property healthCheckTimeoutSeconds The amount of time, in seconds, to wait before reporting a target as unhealthy. The range is 1–120 seconds. The default is 5 seconds.
* * `healthy_threshold_count ` - (Optional) The number of consecutive successful health checks required before considering an unhealthy target healthy. The range is 2–10. The default is 5.
* @property healthyThresholdCount
* @property matcher The codes to use when checking for a successful response from a target. These are called _Success codes_ in the console.
* @property path The destination for health checks on the targets. If the protocol version is HTTP/1.1 or HTTP/2, specify a valid URI (for example, /path?query). The default path is `/`. Health checks are not supported if the protocol version is gRPC, however, you can choose HTTP/1.1 or HTTP/2 and specify a valid URI.
* @property port The port used when performing health checks on targets. The default setting is the port that a target receives traffic on.
* @property protocol The protocol used when performing health checks on targets. The possible protocols are `HTTP` and `HTTPS`.
* @property protocolVersion The protocol version used when performing health checks on targets. The possible protocol versions are `HTTP1` and `HTTP2`. The default is `HTTP1`.
* @property unhealthyThresholdCount The number of consecutive failed health checks required before considering a target unhealthy. The range is 2–10. The default is 2.
*/
public data class TargetGroupConfigHealthCheckArgs(
public val enabled: Output? = null,
public val healthCheckIntervalSeconds: Output? = null,
public val healthCheckTimeoutSeconds: Output? = null,
public val healthyThresholdCount: Output? = null,
public val matcher: Output? = null,
public val path: Output? = null,
public val port: Output? = null,
public val protocol: Output? = null,
public val protocolVersion: Output? = null,
public val unhealthyThresholdCount: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.vpclattice.inputs.TargetGroupConfigHealthCheckArgs =
com.pulumi.aws.vpclattice.inputs.TargetGroupConfigHealthCheckArgs.builder()
.enabled(enabled?.applyValue({ args0 -> args0 }))
.healthCheckIntervalSeconds(healthCheckIntervalSeconds?.applyValue({ args0 -> args0 }))
.healthCheckTimeoutSeconds(healthCheckTimeoutSeconds?.applyValue({ args0 -> args0 }))
.healthyThresholdCount(healthyThresholdCount?.applyValue({ args0 -> args0 }))
.matcher(matcher?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.path(path?.applyValue({ args0 -> args0 }))
.port(port?.applyValue({ args0 -> args0 }))
.protocol(protocol?.applyValue({ args0 -> args0 }))
.protocolVersion(protocolVersion?.applyValue({ args0 -> args0 }))
.unhealthyThresholdCount(unhealthyThresholdCount?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TargetGroupConfigHealthCheckArgs].
*/
@PulumiTagMarker
public class TargetGroupConfigHealthCheckArgsBuilder internal constructor() {
private var enabled: Output? = null
private var healthCheckIntervalSeconds: Output? = null
private var healthCheckTimeoutSeconds: Output? = null
private var healthyThresholdCount: Output? = null
private var matcher: Output? = null
private var path: Output? = null
private var port: Output? = null
private var protocol: Output? = null
private var protocolVersion: Output? = null
private var unhealthyThresholdCount: Output? = null
/**
* @param value Indicates whether health checking is enabled. Defaults to `true`.
*/
@JvmName("armckendogdvudvg")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value The approximate amount of time, in seconds, between health checks of an individual target. The range is 5–300 seconds. The default is 30 seconds.
*/
@JvmName("aoofufuiunkhoekv")
public suspend fun healthCheckIntervalSeconds(`value`: Output) {
this.healthCheckIntervalSeconds = value
}
/**
* @param value The amount of time, in seconds, to wait before reporting a target as unhealthy. The range is 1–120 seconds. The default is 5 seconds.
* * `healthy_threshold_count ` - (Optional) The number of consecutive successful health checks required before considering an unhealthy target healthy. The range is 2–10. The default is 5.
*/
@JvmName("ubvfokvtxxyuhsse")
public suspend fun healthCheckTimeoutSeconds(`value`: Output) {
this.healthCheckTimeoutSeconds = value
}
/**
* @param value
*/
@JvmName("iblkujxamgshrghw")
public suspend fun healthyThresholdCount(`value`: Output) {
this.healthyThresholdCount = value
}
/**
* @param value The codes to use when checking for a successful response from a target. These are called _Success codes_ in the console.
*/
@JvmName("kvewgviocfhfhvsf")
public suspend fun matcher(`value`: Output) {
this.matcher = value
}
/**
* @param value The destination for health checks on the targets. If the protocol version is HTTP/1.1 or HTTP/2, specify a valid URI (for example, /path?query). The default path is `/`. Health checks are not supported if the protocol version is gRPC, however, you can choose HTTP/1.1 or HTTP/2 and specify a valid URI.
*/
@JvmName("jkilomopmrmdyryl")
public suspend fun path(`value`: Output) {
this.path = value
}
/**
* @param value The port used when performing health checks on targets. The default setting is the port that a target receives traffic on.
*/
@JvmName("stxjgqjpqewfaujf")
public suspend fun port(`value`: Output) {
this.port = value
}
/**
* @param value The protocol used when performing health checks on targets. The possible protocols are `HTTP` and `HTTPS`.
*/
@JvmName("marfkgdndldpsgxl")
public suspend fun protocol(`value`: Output) {
this.protocol = value
}
/**
* @param value The protocol version used when performing health checks on targets. The possible protocol versions are `HTTP1` and `HTTP2`. The default is `HTTP1`.
*/
@JvmName("gwstuhysfwuhmjwp")
public suspend fun protocolVersion(`value`: Output) {
this.protocolVersion = value
}
/**
* @param value The number of consecutive failed health checks required before considering a target unhealthy. The range is 2–10. The default is 2.
*/
@JvmName("asyoyxxujwmxctqj")
public suspend fun unhealthyThresholdCount(`value`: Output) {
this.unhealthyThresholdCount = value
}
/**
* @param value Indicates whether health checking is enabled. Defaults to `true`.
*/
@JvmName("wwdrmcamthskeplp")
public suspend fun enabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value The approximate amount of time, in seconds, between health checks of an individual target. The range is 5–300 seconds. The default is 30 seconds.
*/
@JvmName("hbjyriimacxdavoy")
public suspend fun healthCheckIntervalSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.healthCheckIntervalSeconds = mapped
}
/**
* @param value The amount of time, in seconds, to wait before reporting a target as unhealthy. The range is 1–120 seconds. The default is 5 seconds.
* * `healthy_threshold_count ` - (Optional) The number of consecutive successful health checks required before considering an unhealthy target healthy. The range is 2–10. The default is 5.
*/
@JvmName("gmurvjlnnbytebjy")
public suspend fun healthCheckTimeoutSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.healthCheckTimeoutSeconds = mapped
}
/**
* @param value
*/
@JvmName("khsaipinpqcrcdhb")
public suspend fun healthyThresholdCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.healthyThresholdCount = mapped
}
/**
* @param value The codes to use when checking for a successful response from a target. These are called _Success codes_ in the console.
*/
@JvmName("ogiscauwjvdwnymx")
public suspend fun matcher(`value`: TargetGroupConfigHealthCheckMatcherArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.matcher = mapped
}
/**
* @param argument The codes to use when checking for a successful response from a target. These are called _Success codes_ in the console.
*/
@JvmName("nbmocxdjgwkfkdkj")
public suspend fun matcher(argument: suspend TargetGroupConfigHealthCheckMatcherArgsBuilder.() -> Unit) {
val toBeMapped = TargetGroupConfigHealthCheckMatcherArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.matcher = mapped
}
/**
* @param value The destination for health checks on the targets. If the protocol version is HTTP/1.1 or HTTP/2, specify a valid URI (for example, /path?query). The default path is `/`. Health checks are not supported if the protocol version is gRPC, however, you can choose HTTP/1.1 or HTTP/2 and specify a valid URI.
*/
@JvmName("ncktdcysmklpwueb")
public suspend fun path(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.path = mapped
}
/**
* @param value The port used when performing health checks on targets. The default setting is the port that a target receives traffic on.
*/
@JvmName("divgnjxrecmfspyi")
public suspend fun port(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.port = mapped
}
/**
* @param value The protocol used when performing health checks on targets. The possible protocols are `HTTP` and `HTTPS`.
*/
@JvmName("decqkwbtkxqmyare")
public suspend fun protocol(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.protocol = mapped
}
/**
* @param value The protocol version used when performing health checks on targets. The possible protocol versions are `HTTP1` and `HTTP2`. The default is `HTTP1`.
*/
@JvmName("gqyoguiifccnymkn")
public suspend fun protocolVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.protocolVersion = mapped
}
/**
* @param value The number of consecutive failed health checks required before considering a target unhealthy. The range is 2–10. The default is 2.
*/
@JvmName("tntrfipmcdelwvyo")
public suspend fun unhealthyThresholdCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.unhealthyThresholdCount = mapped
}
internal fun build(): TargetGroupConfigHealthCheckArgs = TargetGroupConfigHealthCheckArgs(
enabled = enabled,
healthCheckIntervalSeconds = healthCheckIntervalSeconds,
healthCheckTimeoutSeconds = healthCheckTimeoutSeconds,
healthyThresholdCount = healthyThresholdCount,
matcher = matcher,
path = path,
port = port,
protocol = protocol,
protocolVersion = protocolVersion,
unhealthyThresholdCount = unhealthyThresholdCount,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy