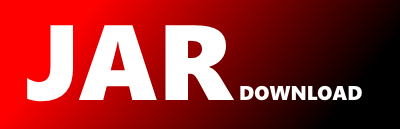
com.pulumi.aws.wafv2.kotlin.Wafv2Functions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.wafv2.kotlin
import com.pulumi.aws.wafv2.Wafv2Functions.getIpSetPlain
import com.pulumi.aws.wafv2.Wafv2Functions.getRegexPatternSetPlain
import com.pulumi.aws.wafv2.Wafv2Functions.getRuleGroupPlain
import com.pulumi.aws.wafv2.Wafv2Functions.getWebAclPlain
import com.pulumi.aws.wafv2.kotlin.inputs.GetIpSetPlainArgs
import com.pulumi.aws.wafv2.kotlin.inputs.GetIpSetPlainArgsBuilder
import com.pulumi.aws.wafv2.kotlin.inputs.GetRegexPatternSetPlainArgs
import com.pulumi.aws.wafv2.kotlin.inputs.GetRegexPatternSetPlainArgsBuilder
import com.pulumi.aws.wafv2.kotlin.inputs.GetRuleGroupPlainArgs
import com.pulumi.aws.wafv2.kotlin.inputs.GetRuleGroupPlainArgsBuilder
import com.pulumi.aws.wafv2.kotlin.inputs.GetWebAclPlainArgs
import com.pulumi.aws.wafv2.kotlin.inputs.GetWebAclPlainArgsBuilder
import com.pulumi.aws.wafv2.kotlin.outputs.GetIpSetResult
import com.pulumi.aws.wafv2.kotlin.outputs.GetRegexPatternSetResult
import com.pulumi.aws.wafv2.kotlin.outputs.GetRuleGroupResult
import com.pulumi.aws.wafv2.kotlin.outputs.GetWebAclResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.aws.wafv2.kotlin.outputs.GetIpSetResult.Companion.toKotlin as getIpSetResultToKotlin
import com.pulumi.aws.wafv2.kotlin.outputs.GetRegexPatternSetResult.Companion.toKotlin as getRegexPatternSetResultToKotlin
import com.pulumi.aws.wafv2.kotlin.outputs.GetRuleGroupResult.Companion.toKotlin as getRuleGroupResultToKotlin
import com.pulumi.aws.wafv2.kotlin.outputs.GetWebAclResult.Companion.toKotlin as getWebAclResultToKotlin
public object Wafv2Functions {
/**
* Retrieves the summary of a WAFv2 IP Set.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.wafv2.getIpSet({
* name: "some-ip-set",
* scope: "REGIONAL",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.wafv2.get_ip_set(name="some-ip-set",
* scope="REGIONAL")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.WafV2.GetIpSet.Invoke(new()
* {
* Name = "some-ip-set",
* Scope = "REGIONAL",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/wafv2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := wafv2.LookupIpSet(ctx, &wafv2.LookupIpSetArgs{
* Name: "some-ip-set",
* Scope: "REGIONAL",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.wafv2.Wafv2Functions;
* import com.pulumi.aws.wafv2.inputs.GetIpSetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = Wafv2Functions.getIpSet(GetIpSetArgs.builder()
* .name("some-ip-set")
* .scope("REGIONAL")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: aws:wafv2:getIpSet
* Arguments:
* name: some-ip-set
* scope: REGIONAL
* ```
*
* @param argument A collection of arguments for invoking getIpSet.
* @return A collection of values returned by getIpSet.
*/
public suspend fun getIpSet(argument: GetIpSetPlainArgs): GetIpSetResult =
getIpSetResultToKotlin(getIpSetPlain(argument.toJava()).await())
/**
* @see [getIpSet].
* @param name Name of the WAFv2 IP Set.
* @param scope Specifies whether this is for an AWS CloudFront distribution or for a regional application. Valid values are `CLOUDFRONT` or `REGIONAL`. To work with CloudFront, you must also specify the region `us-east-1` (N. Virginia) on the AWS provider.
* @return A collection of values returned by getIpSet.
*/
public suspend fun getIpSet(name: String, scope: String): GetIpSetResult {
val argument = GetIpSetPlainArgs(
name = name,
scope = scope,
)
return getIpSetResultToKotlin(getIpSetPlain(argument.toJava()).await())
}
/**
* @see [getIpSet].
* @param argument Builder for [com.pulumi.aws.wafv2.kotlin.inputs.GetIpSetPlainArgs].
* @return A collection of values returned by getIpSet.
*/
public suspend fun getIpSet(argument: suspend GetIpSetPlainArgsBuilder.() -> Unit): GetIpSetResult {
val builder = GetIpSetPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getIpSetResultToKotlin(getIpSetPlain(builtArgument.toJava()).await())
}
/**
* Retrieves the summary of a WAFv2 Regex Pattern Set.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.wafv2.getRegexPatternSet({
* name: "some-regex-pattern-set",
* scope: "REGIONAL",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.wafv2.get_regex_pattern_set(name="some-regex-pattern-set",
* scope="REGIONAL")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.WafV2.GetRegexPatternSet.Invoke(new()
* {
* Name = "some-regex-pattern-set",
* Scope = "REGIONAL",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/wafv2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := wafv2.LookupRegexPatternSet(ctx, &wafv2.LookupRegexPatternSetArgs{
* Name: "some-regex-pattern-set",
* Scope: "REGIONAL",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.wafv2.Wafv2Functions;
* import com.pulumi.aws.wafv2.inputs.GetRegexPatternSetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = Wafv2Functions.getRegexPatternSet(GetRegexPatternSetArgs.builder()
* .name("some-regex-pattern-set")
* .scope("REGIONAL")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: aws:wafv2:getRegexPatternSet
* Arguments:
* name: some-regex-pattern-set
* scope: REGIONAL
* ```
*
* @param argument A collection of arguments for invoking getRegexPatternSet.
* @return A collection of values returned by getRegexPatternSet.
*/
public suspend fun getRegexPatternSet(argument: GetRegexPatternSetPlainArgs): GetRegexPatternSetResult =
getRegexPatternSetResultToKotlin(getRegexPatternSetPlain(argument.toJava()).await())
/**
* @see [getRegexPatternSet].
* @param name Name of the WAFv2 Regex Pattern Set.
* @param scope Specifies whether this is for an AWS CloudFront distribution or for a regional application. Valid values are `CLOUDFRONT` or `REGIONAL`. To work with CloudFront, you must also specify the region `us-east-1` (N. Virginia) on the AWS provider.
* @return A collection of values returned by getRegexPatternSet.
*/
public suspend fun getRegexPatternSet(name: String, scope: String): GetRegexPatternSetResult {
val argument = GetRegexPatternSetPlainArgs(
name = name,
scope = scope,
)
return getRegexPatternSetResultToKotlin(getRegexPatternSetPlain(argument.toJava()).await())
}
/**
* @see [getRegexPatternSet].
* @param argument Builder for [com.pulumi.aws.wafv2.kotlin.inputs.GetRegexPatternSetPlainArgs].
* @return A collection of values returned by getRegexPatternSet.
*/
public suspend fun getRegexPatternSet(argument: suspend GetRegexPatternSetPlainArgsBuilder.() -> Unit): GetRegexPatternSetResult {
val builder = GetRegexPatternSetPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRegexPatternSetResultToKotlin(getRegexPatternSetPlain(builtArgument.toJava()).await())
}
/**
* Retrieves the summary of a WAFv2 Rule Group.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.wafv2.getRuleGroup({
* name: "some-rule-group",
* scope: "REGIONAL",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.wafv2.get_rule_group(name="some-rule-group",
* scope="REGIONAL")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.WafV2.GetRuleGroup.Invoke(new()
* {
* Name = "some-rule-group",
* Scope = "REGIONAL",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/wafv2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := wafv2.LookupRuleGroup(ctx, &wafv2.LookupRuleGroupArgs{
* Name: "some-rule-group",
* Scope: "REGIONAL",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.wafv2.Wafv2Functions;
* import com.pulumi.aws.wafv2.inputs.GetRuleGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = Wafv2Functions.getRuleGroup(GetRuleGroupArgs.builder()
* .name("some-rule-group")
* .scope("REGIONAL")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: aws:wafv2:getRuleGroup
* Arguments:
* name: some-rule-group
* scope: REGIONAL
* ```
*
* @param argument A collection of arguments for invoking getRuleGroup.
* @return A collection of values returned by getRuleGroup.
*/
public suspend fun getRuleGroup(argument: GetRuleGroupPlainArgs): GetRuleGroupResult =
getRuleGroupResultToKotlin(getRuleGroupPlain(argument.toJava()).await())
/**
* @see [getRuleGroup].
* @param name Name of the WAFv2 Rule Group.
* @param scope Specifies whether this is for an AWS CloudFront distribution or for a regional application. Valid values are `CLOUDFRONT` or `REGIONAL`. To work with CloudFront, you must also specify the region `us-east-1` (N. Virginia) on the AWS provider.
* @return A collection of values returned by getRuleGroup.
*/
public suspend fun getRuleGroup(name: String, scope: String): GetRuleGroupResult {
val argument = GetRuleGroupPlainArgs(
name = name,
scope = scope,
)
return getRuleGroupResultToKotlin(getRuleGroupPlain(argument.toJava()).await())
}
/**
* @see [getRuleGroup].
* @param argument Builder for [com.pulumi.aws.wafv2.kotlin.inputs.GetRuleGroupPlainArgs].
* @return A collection of values returned by getRuleGroup.
*/
public suspend fun getRuleGroup(argument: suspend GetRuleGroupPlainArgsBuilder.() -> Unit): GetRuleGroupResult {
val builder = GetRuleGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRuleGroupResultToKotlin(getRuleGroupPlain(builtArgument.toJava()).await())
}
/**
* Retrieves the summary of a WAFv2 Web ACL.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.wafv2.getWebAcl({
* name: "some-web-acl",
* scope: "REGIONAL",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.wafv2.get_web_acl(name="some-web-acl",
* scope="REGIONAL")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.WafV2.GetWebAcl.Invoke(new()
* {
* Name = "some-web-acl",
* Scope = "REGIONAL",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/wafv2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := wafv2.LookupWebAcl(ctx, &wafv2.LookupWebAclArgs{
* Name: "some-web-acl",
* Scope: "REGIONAL",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.wafv2.Wafv2Functions;
* import com.pulumi.aws.wafv2.inputs.GetWebAclArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = Wafv2Functions.getWebAcl(GetWebAclArgs.builder()
* .name("some-web-acl")
* .scope("REGIONAL")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: aws:wafv2:getWebAcl
* Arguments:
* name: some-web-acl
* scope: REGIONAL
* ```
*
* @param argument A collection of arguments for invoking getWebAcl.
* @return A collection of values returned by getWebAcl.
*/
public suspend fun getWebAcl(argument: GetWebAclPlainArgs): GetWebAclResult =
getWebAclResultToKotlin(getWebAclPlain(argument.toJava()).await())
/**
* @see [getWebAcl].
* @param name Name of the WAFv2 Web ACL.
* @param scope Specifies whether this is for an AWS CloudFront distribution or for a regional application. Valid values are `CLOUDFRONT` or `REGIONAL`. To work with CloudFront, you must also specify the region `us-east-1` (N. Virginia) on the AWS provider.
* @return A collection of values returned by getWebAcl.
*/
public suspend fun getWebAcl(name: String, scope: String): GetWebAclResult {
val argument = GetWebAclPlainArgs(
name = name,
scope = scope,
)
return getWebAclResultToKotlin(getWebAclPlain(argument.toJava()).await())
}
/**
* @see [getWebAcl].
* @param argument Builder for [com.pulumi.aws.wafv2.kotlin.inputs.GetWebAclPlainArgs].
* @return A collection of values returned by getWebAcl.
*/
public suspend fun getWebAcl(argument: suspend GetWebAclPlainArgsBuilder.() -> Unit): GetWebAclResult {
val builder = GetWebAclPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWebAclResultToKotlin(getWebAclPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy