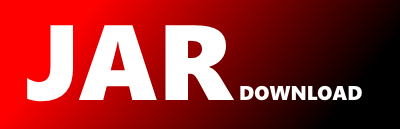
com.pulumi.aws.wafv2.kotlin.inputs.RuleGroupRuleStatementRateBasedStatementCustomKeyArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.wafv2.kotlin.inputs
import com.pulumi.aws.wafv2.inputs.RuleGroupRuleStatementRateBasedStatementCustomKeyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property cookie (Optional) Use the value of a cookie in the request as an aggregate key. See RateLimit `cookie` below for details.
* @property forwardedIp (Optional) Use the first IP address in an HTTP header as an aggregate key. See `forwarded_ip` below for details.
* @property header (Optional) Use the value of a header in the request as an aggregate key. See RateLimit `header` below for details.
* @property httpMethod (Optional) Use the request's HTTP method as an aggregate key. See RateLimit `http_method` below for details.
* @property ip (Optional) Use the request's originating IP address as an aggregate key. See `RateLimit ip` below for details.
* @property labelNamespace (Optional) Use the specified label namespace as an aggregate key. See RateLimit `label_namespace` below for details.
* @property queryArgument (Optional) Use the specified query argument as an aggregate key. See RateLimit `query_argument` below for details.
* @property queryString (Optional) Use the request's query string as an aggregate key. See RateLimit `query_string` below for details.
* @property uriPath (Optional) Use the request's URI path as an aggregate key. See RateLimit `uri_path` below for details.
*/
public data class RuleGroupRuleStatementRateBasedStatementCustomKeyArgs(
public val cookie: Output? = null,
public val forwardedIp: Output? =
null,
public val `header`: Output? = null,
public val httpMethod: Output? =
null,
public val ip: Output? = null,
public val labelNamespace: Output? = null,
public val queryArgument: Output? = null,
public val queryString: Output? =
null,
public val uriPath: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.wafv2.inputs.RuleGroupRuleStatementRateBasedStatementCustomKeyArgs =
com.pulumi.aws.wafv2.inputs.RuleGroupRuleStatementRateBasedStatementCustomKeyArgs.builder()
.cookie(cookie?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.forwardedIp(forwardedIp?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.`header`(`header`?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.httpMethod(httpMethod?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ip(ip?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.labelNamespace(labelNamespace?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.queryArgument(queryArgument?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.queryString(queryString?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.uriPath(uriPath?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [RuleGroupRuleStatementRateBasedStatementCustomKeyArgs].
*/
@PulumiTagMarker
public class RuleGroupRuleStatementRateBasedStatementCustomKeyArgsBuilder internal constructor() {
private var cookie: Output? = null
private var forwardedIp: Output? =
null
private var `header`: Output? = null
private var httpMethod: Output? =
null
private var ip: Output? = null
private var labelNamespace:
Output? = null
private var queryArgument:
Output? = null
private var queryString: Output? =
null
private var uriPath: Output? = null
/**
* @param value (Optional) Use the value of a cookie in the request as an aggregate key. See RateLimit `cookie` below for details.
*/
@JvmName("sxbbtnqajwptymtm")
public suspend fun cookie(`value`: Output) {
this.cookie = value
}
/**
* @param value (Optional) Use the first IP address in an HTTP header as an aggregate key. See `forwarded_ip` below for details.
*/
@JvmName("ntdoqakfrlasbjpp")
public suspend fun forwardedIp(`value`: Output) {
this.forwardedIp = value
}
/**
* @param value (Optional) Use the value of a header in the request as an aggregate key. See RateLimit `header` below for details.
*/
@JvmName("qlukqipxohseocmf")
public suspend fun `header`(`value`: Output) {
this.`header` = value
}
/**
* @param value (Optional) Use the request's HTTP method as an aggregate key. See RateLimit `http_method` below for details.
*/
@JvmName("isnbaukqtculmvrm")
public suspend fun httpMethod(`value`: Output) {
this.httpMethod = value
}
/**
* @param value (Optional) Use the request's originating IP address as an aggregate key. See `RateLimit ip` below for details.
*/
@JvmName("ijynjkihrvgxosfd")
public suspend fun ip(`value`: Output) {
this.ip = value
}
/**
* @param value (Optional) Use the specified label namespace as an aggregate key. See RateLimit `label_namespace` below for details.
*/
@JvmName("gjpntnovsuchavab")
public suspend fun labelNamespace(`value`: Output) {
this.labelNamespace = value
}
/**
* @param value (Optional) Use the specified query argument as an aggregate key. See RateLimit `query_argument` below for details.
*/
@JvmName("vxvebvqinrthfbos")
public suspend fun queryArgument(`value`: Output) {
this.queryArgument = value
}
/**
* @param value (Optional) Use the request's query string as an aggregate key. See RateLimit `query_string` below for details.
*/
@JvmName("ekkbwfxnyijandwo")
public suspend fun queryString(`value`: Output) {
this.queryString = value
}
/**
* @param value (Optional) Use the request's URI path as an aggregate key. See RateLimit `uri_path` below for details.
*/
@JvmName("bgvxumdgwqgudxdp")
public suspend fun uriPath(`value`: Output) {
this.uriPath = value
}
/**
* @param value (Optional) Use the value of a cookie in the request as an aggregate key. See RateLimit `cookie` below for details.
*/
@JvmName("hydkfkaihwlkcigq")
public suspend fun cookie(`value`: RuleGroupRuleStatementRateBasedStatementCustomKeyCookieArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cookie = mapped
}
/**
* @param argument (Optional) Use the value of a cookie in the request as an aggregate key. See RateLimit `cookie` below for details.
*/
@JvmName("fnoqnyrqqjlrkhxs")
public suspend fun cookie(argument: suspend RuleGroupRuleStatementRateBasedStatementCustomKeyCookieArgsBuilder.() -> Unit) {
val toBeMapped =
RuleGroupRuleStatementRateBasedStatementCustomKeyCookieArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cookie = mapped
}
/**
* @param value (Optional) Use the first IP address in an HTTP header as an aggregate key. See `forwarded_ip` below for details.
*/
@JvmName("jinjvnbcshexsxvd")
public suspend fun forwardedIp(`value`: RuleGroupRuleStatementRateBasedStatementCustomKeyForwardedIpArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.forwardedIp = mapped
}
/**
* @param argument (Optional) Use the first IP address in an HTTP header as an aggregate key. See `forwarded_ip` below for details.
*/
@JvmName("nhapybxrvtgrmncy")
public suspend fun forwardedIp(argument: suspend RuleGroupRuleStatementRateBasedStatementCustomKeyForwardedIpArgsBuilder.() -> Unit) {
val toBeMapped =
RuleGroupRuleStatementRateBasedStatementCustomKeyForwardedIpArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.forwardedIp = mapped
}
/**
* @param value (Optional) Use the value of a header in the request as an aggregate key. See RateLimit `header` below for details.
*/
@JvmName("xflawewupmnblwru")
public suspend fun `header`(`value`: RuleGroupRuleStatementRateBasedStatementCustomKeyHeaderArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`header` = mapped
}
/**
* @param argument (Optional) Use the value of a header in the request as an aggregate key. See RateLimit `header` below for details.
*/
@JvmName("xkimwygernlrervp")
public suspend fun `header`(argument: suspend RuleGroupRuleStatementRateBasedStatementCustomKeyHeaderArgsBuilder.() -> Unit) {
val toBeMapped =
RuleGroupRuleStatementRateBasedStatementCustomKeyHeaderArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.`header` = mapped
}
/**
* @param value (Optional) Use the request's HTTP method as an aggregate key. See RateLimit `http_method` below for details.
*/
@JvmName("qhtgxjwwktpiukse")
public suspend fun httpMethod(`value`: RuleGroupRuleStatementRateBasedStatementCustomKeyHttpMethodArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.httpMethod = mapped
}
/**
* @param argument (Optional) Use the request's HTTP method as an aggregate key. See RateLimit `http_method` below for details.
*/
@JvmName("udgxhbrifapnthiu")
public suspend fun httpMethod(argument: suspend RuleGroupRuleStatementRateBasedStatementCustomKeyHttpMethodArgsBuilder.() -> Unit) {
val toBeMapped =
RuleGroupRuleStatementRateBasedStatementCustomKeyHttpMethodArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.httpMethod = mapped
}
/**
* @param value (Optional) Use the request's originating IP address as an aggregate key. See `RateLimit ip` below for details.
*/
@JvmName("qhljsxvqnqtntqak")
public suspend fun ip(`value`: RuleGroupRuleStatementRateBasedStatementCustomKeyIpArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ip = mapped
}
/**
* @param argument (Optional) Use the request's originating IP address as an aggregate key. See `RateLimit ip` below for details.
*/
@JvmName("dffjcmkpyqqfnthd")
public suspend fun ip(argument: suspend RuleGroupRuleStatementRateBasedStatementCustomKeyIpArgsBuilder.() -> Unit) {
val toBeMapped = RuleGroupRuleStatementRateBasedStatementCustomKeyIpArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.ip = mapped
}
/**
* @param value (Optional) Use the specified label namespace as an aggregate key. See RateLimit `label_namespace` below for details.
*/
@JvmName("yvuervoutcepwysl")
public suspend fun labelNamespace(`value`: RuleGroupRuleStatementRateBasedStatementCustomKeyLabelNamespaceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.labelNamespace = mapped
}
/**
* @param argument (Optional) Use the specified label namespace as an aggregate key. See RateLimit `label_namespace` below for details.
*/
@JvmName("lwewweeswyajxbue")
public suspend fun labelNamespace(argument: suspend RuleGroupRuleStatementRateBasedStatementCustomKeyLabelNamespaceArgsBuilder.() -> Unit) {
val toBeMapped =
RuleGroupRuleStatementRateBasedStatementCustomKeyLabelNamespaceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.labelNamespace = mapped
}
/**
* @param value (Optional) Use the specified query argument as an aggregate key. See RateLimit `query_argument` below for details.
*/
@JvmName("ryfpafkecffwiusi")
public suspend fun queryArgument(`value`: RuleGroupRuleStatementRateBasedStatementCustomKeyQueryArgumentArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queryArgument = mapped
}
/**
* @param argument (Optional) Use the specified query argument as an aggregate key. See RateLimit `query_argument` below for details.
*/
@JvmName("tlldqgieteoqfenj")
public suspend fun queryArgument(argument: suspend RuleGroupRuleStatementRateBasedStatementCustomKeyQueryArgumentArgsBuilder.() -> Unit) {
val toBeMapped =
RuleGroupRuleStatementRateBasedStatementCustomKeyQueryArgumentArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.queryArgument = mapped
}
/**
* @param value (Optional) Use the request's query string as an aggregate key. See RateLimit `query_string` below for details.
*/
@JvmName("jpwmsockoqdxatmx")
public suspend fun queryString(`value`: RuleGroupRuleStatementRateBasedStatementCustomKeyQueryStringArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queryString = mapped
}
/**
* @param argument (Optional) Use the request's query string as an aggregate key. See RateLimit `query_string` below for details.
*/
@JvmName("uscstuytudpumbir")
public suspend fun queryString(argument: suspend RuleGroupRuleStatementRateBasedStatementCustomKeyQueryStringArgsBuilder.() -> Unit) {
val toBeMapped =
RuleGroupRuleStatementRateBasedStatementCustomKeyQueryStringArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.queryString = mapped
}
/**
* @param value (Optional) Use the request's URI path as an aggregate key. See RateLimit `uri_path` below for details.
*/
@JvmName("ruxigeebapkarutn")
public suspend fun uriPath(`value`: RuleGroupRuleStatementRateBasedStatementCustomKeyUriPathArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.uriPath = mapped
}
/**
* @param argument (Optional) Use the request's URI path as an aggregate key. See RateLimit `uri_path` below for details.
*/
@JvmName("mscebbdcwprsipet")
public suspend fun uriPath(argument: suspend RuleGroupRuleStatementRateBasedStatementCustomKeyUriPathArgsBuilder.() -> Unit) {
val toBeMapped =
RuleGroupRuleStatementRateBasedStatementCustomKeyUriPathArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.uriPath = mapped
}
internal fun build(): RuleGroupRuleStatementRateBasedStatementCustomKeyArgs =
RuleGroupRuleStatementRateBasedStatementCustomKeyArgs(
cookie = cookie,
forwardedIp = forwardedIp,
`header` = `header`,
httpMethod = httpMethod,
ip = ip,
labelNamespace = labelNamespace,
queryArgument = queryArgument,
queryString = queryString,
uriPath = uriPath,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy