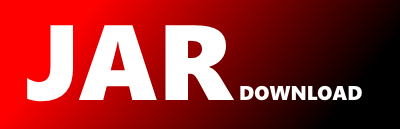
com.pulumi.aws.wafv2.kotlin.inputs.WebAclRuleActionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.wafv2.kotlin.inputs
import com.pulumi.aws.wafv2.inputs.WebAclRuleActionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property allow Instructs AWS WAF to allow the web request. See `allow` below for details.
* @property block Instructs AWS WAF to block the web request. See `block` below for details.
* @property captcha Instructs AWS WAF to run a Captcha check against the web request. See `captcha` below for details.
* @property challenge Instructs AWS WAF to run a check against the request to verify that the request is coming from a legitimate client session. See `challenge` below for details.
* @property count Instructs AWS WAF to count the web request and allow it. See `count` below for details.
*/
public data class WebAclRuleActionArgs(
public val allow: Output? = null,
public val block: Output? = null,
public val captcha: Output? = null,
public val challenge: Output? = null,
public val count: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.wafv2.inputs.WebAclRuleActionArgs =
com.pulumi.aws.wafv2.inputs.WebAclRuleActionArgs.builder()
.allow(allow?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.block(block?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.captcha(captcha?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.challenge(challenge?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.count(count?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [WebAclRuleActionArgs].
*/
@PulumiTagMarker
public class WebAclRuleActionArgsBuilder internal constructor() {
private var allow: Output? = null
private var block: Output? = null
private var captcha: Output? = null
private var challenge: Output? = null
private var count: Output? = null
/**
* @param value Instructs AWS WAF to allow the web request. See `allow` below for details.
*/
@JvmName("wodwnwjitoldchky")
public suspend fun allow(`value`: Output) {
this.allow = value
}
/**
* @param value Instructs AWS WAF to block the web request. See `block` below for details.
*/
@JvmName("qnctscitnssdatpk")
public suspend fun block(`value`: Output) {
this.block = value
}
/**
* @param value Instructs AWS WAF to run a Captcha check against the web request. See `captcha` below for details.
*/
@JvmName("ewqwhskvndctwano")
public suspend fun captcha(`value`: Output) {
this.captcha = value
}
/**
* @param value Instructs AWS WAF to run a check against the request to verify that the request is coming from a legitimate client session. See `challenge` below for details.
*/
@JvmName("kidkbxsgiphpoxwc")
public suspend fun challenge(`value`: Output) {
this.challenge = value
}
/**
* @param value Instructs AWS WAF to count the web request and allow it. See `count` below for details.
*/
@JvmName("ouapghwfbxmmudlb")
public suspend fun count(`value`: Output) {
this.count = value
}
/**
* @param value Instructs AWS WAF to allow the web request. See `allow` below for details.
*/
@JvmName("jagiamgxonmpqvrl")
public suspend fun allow(`value`: WebAclRuleActionAllowArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allow = mapped
}
/**
* @param argument Instructs AWS WAF to allow the web request. See `allow` below for details.
*/
@JvmName("nnrduolqnivdbdnt")
public suspend fun allow(argument: suspend WebAclRuleActionAllowArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleActionAllowArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.allow = mapped
}
/**
* @param value Instructs AWS WAF to block the web request. See `block` below for details.
*/
@JvmName("sirkcgbhvkfxafeq")
public suspend fun block(`value`: WebAclRuleActionBlockArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.block = mapped
}
/**
* @param argument Instructs AWS WAF to block the web request. See `block` below for details.
*/
@JvmName("iypqyvvplkrgleyk")
public suspend fun block(argument: suspend WebAclRuleActionBlockArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleActionBlockArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.block = mapped
}
/**
* @param value Instructs AWS WAF to run a Captcha check against the web request. See `captcha` below for details.
*/
@JvmName("nlmgkeshbqyhhwkl")
public suspend fun captcha(`value`: WebAclRuleActionCaptchaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.captcha = mapped
}
/**
* @param argument Instructs AWS WAF to run a Captcha check against the web request. See `captcha` below for details.
*/
@JvmName("wycbiptjffpewrkr")
public suspend fun captcha(argument: suspend WebAclRuleActionCaptchaArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleActionCaptchaArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.captcha = mapped
}
/**
* @param value Instructs AWS WAF to run a check against the request to verify that the request is coming from a legitimate client session. See `challenge` below for details.
*/
@JvmName("coimysgjtpcxeauv")
public suspend fun challenge(`value`: WebAclRuleActionChallengeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.challenge = mapped
}
/**
* @param argument Instructs AWS WAF to run a check against the request to verify that the request is coming from a legitimate client session. See `challenge` below for details.
*/
@JvmName("ceuiobbmrsnadpxt")
public suspend fun challenge(argument: suspend WebAclRuleActionChallengeArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleActionChallengeArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.challenge = mapped
}
/**
* @param value Instructs AWS WAF to count the web request and allow it. See `count` below for details.
*/
@JvmName("qpbuufqbmywrjmgo")
public suspend fun count(`value`: WebAclRuleActionCountArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.count = mapped
}
/**
* @param argument Instructs AWS WAF to count the web request and allow it. See `count` below for details.
*/
@JvmName("ygvvxotyjxqmnarw")
public suspend fun count(argument: suspend WebAclRuleActionCountArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleActionCountArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.count = mapped
}
internal fun build(): WebAclRuleActionArgs = WebAclRuleActionArgs(
allow = allow,
block = block,
captcha = captcha,
challenge = challenge,
count = count,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy