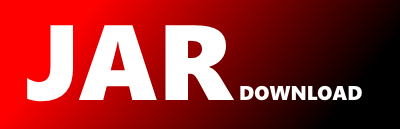
com.pulumi.aws.wafv2.kotlin.inputs.WebAclRuleStatementArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.wafv2.kotlin.inputs
import com.pulumi.aws.wafv2.inputs.WebAclRuleStatementArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property andStatement Logical rule statement used to combine other rule statements with AND logic. See `and_statement` below for details.
* @property byteMatchStatement Rule statement that defines a string match search for AWS WAF to apply to web requests. See `byte_match_statement` below for details.
* @property geoMatchStatement Rule statement used to identify web requests based on country of origin. See `geo_match_statement` below for details.
* @property ipSetReferenceStatement Rule statement used to detect web requests coming from particular IP addresses or address ranges. See `ip_set_reference_statement` below for details.
* @property labelMatchStatement Rule statement that defines a string match search against labels that have been added to the web request by rules that have already run in the web ACL. See `label_match_statement` below for details.
* @property managedRuleGroupStatement Rule statement used to run the rules that are defined in a managed rule group. This statement can not be nested. See `managed_rule_group_statement` below for details.
* @property notStatement Logical rule statement used to negate the results of another rule statement. See `not_statement` below for details.
* @property orStatement Logical rule statement used to combine other rule statements with OR logic. See `or_statement` below for details.
* @property rateBasedStatement Rate-based rule tracks the rate of requests for each originating `IP address`, and triggers the rule action when the rate exceeds a limit that you specify on the number of requests in any `5-minute` time span. This statement can not be nested. See `rate_based_statement` below for details.
* @property regexMatchStatement Rule statement used to search web request components for a match against a single regular expression. See `regex_match_statement` below for details.
* @property regexPatternSetReferenceStatement Rule statement used to search web request components for matches with regular expressions. See `regex_pattern_set_reference_statement` below for details.
* @property ruleGroupReferenceStatement Rule statement used to run the rules that are defined in an WAFv2 Rule Group. See `rule_group_reference_statement` below for details.
* @property sizeConstraintStatement Rule statement that compares a number of bytes against the size of a request component, using a comparison operator, such as greater than (>) or less than (<). See `size_constraint_statement` below for more details.
* @property sqliMatchStatement An SQL injection match condition identifies the part of web requests, such as the URI or the query string, that you want AWS WAF to inspect. See `sqli_match_statement` below for details.
* @property xssMatchStatement Rule statement that defines a cross-site scripting (XSS) match search for AWS WAF to apply to web requests. See `xss_match_statement` below for details.
*/
public data class WebAclRuleStatementArgs(
public val andStatement: Output? = null,
public val byteMatchStatement: Output? = null,
public val geoMatchStatement: Output? = null,
public val ipSetReferenceStatement: Output? =
null,
public val labelMatchStatement: Output? = null,
public val managedRuleGroupStatement: Output? =
null,
public val notStatement: Output? = null,
public val orStatement: Output? = null,
public val rateBasedStatement: Output? = null,
public val regexMatchStatement: Output? = null,
public val regexPatternSetReferenceStatement: Output? = null,
public val ruleGroupReferenceStatement: Output? = null,
public val sizeConstraintStatement: Output? =
null,
public val sqliMatchStatement: Output? = null,
public val xssMatchStatement: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.wafv2.inputs.WebAclRuleStatementArgs =
com.pulumi.aws.wafv2.inputs.WebAclRuleStatementArgs.builder()
.andStatement(andStatement?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.byteMatchStatement(
byteMatchStatement?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.geoMatchStatement(geoMatchStatement?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ipSetReferenceStatement(
ipSetReferenceStatement?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.labelMatchStatement(
labelMatchStatement?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.managedRuleGroupStatement(
managedRuleGroupStatement?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.notStatement(notStatement?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.orStatement(orStatement?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.rateBasedStatement(
rateBasedStatement?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.regexMatchStatement(
regexMatchStatement?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.regexPatternSetReferenceStatement(
regexPatternSetReferenceStatement?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.ruleGroupReferenceStatement(
ruleGroupReferenceStatement?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sizeConstraintStatement(
sizeConstraintStatement?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sqliMatchStatement(
sqliMatchStatement?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.xssMatchStatement(
xssMatchStatement?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [WebAclRuleStatementArgs].
*/
@PulumiTagMarker
public class WebAclRuleStatementArgsBuilder internal constructor() {
private var andStatement: Output? = null
private var byteMatchStatement: Output? = null
private var geoMatchStatement: Output? = null
private var ipSetReferenceStatement: Output? =
null
private var labelMatchStatement: Output? = null
private var managedRuleGroupStatement: Output? =
null
private var notStatement: Output? = null
private var orStatement: Output? = null
private var rateBasedStatement: Output? = null
private var regexMatchStatement: Output? = null
private var regexPatternSetReferenceStatement:
Output? = null
private var ruleGroupReferenceStatement:
Output? = null
private var sizeConstraintStatement: Output? =
null
private var sqliMatchStatement: Output? = null
private var xssMatchStatement: Output? = null
/**
* @param value Logical rule statement used to combine other rule statements with AND logic. See `and_statement` below for details.
*/
@JvmName("adtputgemknotdjn")
public suspend fun andStatement(`value`: Output) {
this.andStatement = value
}
/**
* @param value Rule statement that defines a string match search for AWS WAF to apply to web requests. See `byte_match_statement` below for details.
*/
@JvmName("nabrdovgcgljpqyh")
public suspend fun byteMatchStatement(`value`: Output) {
this.byteMatchStatement = value
}
/**
* @param value Rule statement used to identify web requests based on country of origin. See `geo_match_statement` below for details.
*/
@JvmName("hbjatlmaxegpigdk")
public suspend fun geoMatchStatement(`value`: Output) {
this.geoMatchStatement = value
}
/**
* @param value Rule statement used to detect web requests coming from particular IP addresses or address ranges. See `ip_set_reference_statement` below for details.
*/
@JvmName("ipwvubiwkwvkmrik")
public suspend fun ipSetReferenceStatement(`value`: Output) {
this.ipSetReferenceStatement = value
}
/**
* @param value Rule statement that defines a string match search against labels that have been added to the web request by rules that have already run in the web ACL. See `label_match_statement` below for details.
*/
@JvmName("erexqmkfouxnjqdc")
public suspend fun labelMatchStatement(`value`: Output) {
this.labelMatchStatement = value
}
/**
* @param value Rule statement used to run the rules that are defined in a managed rule group. This statement can not be nested. See `managed_rule_group_statement` below for details.
*/
@JvmName("gdymxguseqfcgpoo")
public suspend fun managedRuleGroupStatement(`value`: Output) {
this.managedRuleGroupStatement = value
}
/**
* @param value Logical rule statement used to negate the results of another rule statement. See `not_statement` below for details.
*/
@JvmName("cprwwxjcjcgxmvld")
public suspend fun notStatement(`value`: Output) {
this.notStatement = value
}
/**
* @param value Logical rule statement used to combine other rule statements with OR logic. See `or_statement` below for details.
*/
@JvmName("hcihkgeviudoatxk")
public suspend fun orStatement(`value`: Output) {
this.orStatement = value
}
/**
* @param value Rate-based rule tracks the rate of requests for each originating `IP address`, and triggers the rule action when the rate exceeds a limit that you specify on the number of requests in any `5-minute` time span. This statement can not be nested. See `rate_based_statement` below for details.
*/
@JvmName("ipplpihshmjcmdgi")
public suspend fun rateBasedStatement(`value`: Output) {
this.rateBasedStatement = value
}
/**
* @param value Rule statement used to search web request components for a match against a single regular expression. See `regex_match_statement` below for details.
*/
@JvmName("eoitmapgudwnwqkb")
public suspend fun regexMatchStatement(`value`: Output) {
this.regexMatchStatement = value
}
/**
* @param value Rule statement used to search web request components for matches with regular expressions. See `regex_pattern_set_reference_statement` below for details.
*/
@JvmName("ukkwgpvoingstrnr")
public suspend fun regexPatternSetReferenceStatement(`value`: Output) {
this.regexPatternSetReferenceStatement = value
}
/**
* @param value Rule statement used to run the rules that are defined in an WAFv2 Rule Group. See `rule_group_reference_statement` below for details.
*/
@JvmName("jpmfmoljvtmlnyoq")
public suspend fun ruleGroupReferenceStatement(`value`: Output) {
this.ruleGroupReferenceStatement = value
}
/**
* @param value Rule statement that compares a number of bytes against the size of a request component, using a comparison operator, such as greater than (>) or less than (<). See `size_constraint_statement` below for more details.
*/
@JvmName("kdcbucyiymjycrdy")
public suspend fun sizeConstraintStatement(`value`: Output) {
this.sizeConstraintStatement = value
}
/**
* @param value An SQL injection match condition identifies the part of web requests, such as the URI or the query string, that you want AWS WAF to inspect. See `sqli_match_statement` below for details.
*/
@JvmName("sbkupjyjydalfcbs")
public suspend fun sqliMatchStatement(`value`: Output) {
this.sqliMatchStatement = value
}
/**
* @param value Rule statement that defines a cross-site scripting (XSS) match search for AWS WAF to apply to web requests. See `xss_match_statement` below for details.
*/
@JvmName("nxixxwlugctolcsg")
public suspend fun xssMatchStatement(`value`: Output) {
this.xssMatchStatement = value
}
/**
* @param value Logical rule statement used to combine other rule statements with AND logic. See `and_statement` below for details.
*/
@JvmName("ntmtajgkhieuhunh")
public suspend fun andStatement(`value`: WebAclRuleStatementAndStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.andStatement = mapped
}
/**
* @param argument Logical rule statement used to combine other rule statements with AND logic. See `and_statement` below for details.
*/
@JvmName("gvvqnybqrxouumiv")
public suspend fun andStatement(argument: suspend WebAclRuleStatementAndStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementAndStatementArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.andStatement = mapped
}
/**
* @param value Rule statement that defines a string match search for AWS WAF to apply to web requests. See `byte_match_statement` below for details.
*/
@JvmName("qhwacovgjqnekxdn")
public suspend fun byteMatchStatement(`value`: WebAclRuleStatementByteMatchStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.byteMatchStatement = mapped
}
/**
* @param argument Rule statement that defines a string match search for AWS WAF to apply to web requests. See `byte_match_statement` below for details.
*/
@JvmName("mohwuygfgakjofps")
public suspend fun byteMatchStatement(argument: suspend WebAclRuleStatementByteMatchStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementByteMatchStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.byteMatchStatement = mapped
}
/**
* @param value Rule statement used to identify web requests based on country of origin. See `geo_match_statement` below for details.
*/
@JvmName("khgcavnosduyepoi")
public suspend fun geoMatchStatement(`value`: WebAclRuleStatementGeoMatchStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.geoMatchStatement = mapped
}
/**
* @param argument Rule statement used to identify web requests based on country of origin. See `geo_match_statement` below for details.
*/
@JvmName("piacjpuxcfcnhxdx")
public suspend fun geoMatchStatement(argument: suspend WebAclRuleStatementGeoMatchStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementGeoMatchStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.geoMatchStatement = mapped
}
/**
* @param value Rule statement used to detect web requests coming from particular IP addresses or address ranges. See `ip_set_reference_statement` below for details.
*/
@JvmName("kmjmdfrktlleegxc")
public suspend fun ipSetReferenceStatement(`value`: WebAclRuleStatementIpSetReferenceStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipSetReferenceStatement = mapped
}
/**
* @param argument Rule statement used to detect web requests coming from particular IP addresses or address ranges. See `ip_set_reference_statement` below for details.
*/
@JvmName("woflgqyqkqmaixbq")
public suspend fun ipSetReferenceStatement(argument: suspend WebAclRuleStatementIpSetReferenceStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementIpSetReferenceStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.ipSetReferenceStatement = mapped
}
/**
* @param value Rule statement that defines a string match search against labels that have been added to the web request by rules that have already run in the web ACL. See `label_match_statement` below for details.
*/
@JvmName("meanbhyhhfhqvaig")
public suspend fun labelMatchStatement(`value`: WebAclRuleStatementLabelMatchStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.labelMatchStatement = mapped
}
/**
* @param argument Rule statement that defines a string match search against labels that have been added to the web request by rules that have already run in the web ACL. See `label_match_statement` below for details.
*/
@JvmName("juocyucwxwlwonvy")
public suspend fun labelMatchStatement(argument: suspend WebAclRuleStatementLabelMatchStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementLabelMatchStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.labelMatchStatement = mapped
}
/**
* @param value Rule statement used to run the rules that are defined in a managed rule group. This statement can not be nested. See `managed_rule_group_statement` below for details.
*/
@JvmName("dgcjqiyyapihjyqd")
public suspend fun managedRuleGroupStatement(`value`: WebAclRuleStatementManagedRuleGroupStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.managedRuleGroupStatement = mapped
}
/**
* @param argument Rule statement used to run the rules that are defined in a managed rule group. This statement can not be nested. See `managed_rule_group_statement` below for details.
*/
@JvmName("nonuykpruajrnmur")
public suspend fun managedRuleGroupStatement(argument: suspend WebAclRuleStatementManagedRuleGroupStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementManagedRuleGroupStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.managedRuleGroupStatement = mapped
}
/**
* @param value Logical rule statement used to negate the results of another rule statement. See `not_statement` below for details.
*/
@JvmName("pgirexcixfwfvgjc")
public suspend fun notStatement(`value`: WebAclRuleStatementNotStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.notStatement = mapped
}
/**
* @param argument Logical rule statement used to negate the results of another rule statement. See `not_statement` below for details.
*/
@JvmName("ccdepjxafqjgeeyn")
public suspend fun notStatement(argument: suspend WebAclRuleStatementNotStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementNotStatementArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.notStatement = mapped
}
/**
* @param value Logical rule statement used to combine other rule statements with OR logic. See `or_statement` below for details.
*/
@JvmName("gbkqeftbpxxxkacf")
public suspend fun orStatement(`value`: WebAclRuleStatementOrStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.orStatement = mapped
}
/**
* @param argument Logical rule statement used to combine other rule statements with OR logic. See `or_statement` below for details.
*/
@JvmName("mtkxicfsclbfdqlp")
public suspend fun orStatement(argument: suspend WebAclRuleStatementOrStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementOrStatementArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.orStatement = mapped
}
/**
* @param value Rate-based rule tracks the rate of requests for each originating `IP address`, and triggers the rule action when the rate exceeds a limit that you specify on the number of requests in any `5-minute` time span. This statement can not be nested. See `rate_based_statement` below for details.
*/
@JvmName("svfelbwpkehabwcs")
public suspend fun rateBasedStatement(`value`: WebAclRuleStatementRateBasedStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rateBasedStatement = mapped
}
/**
* @param argument Rate-based rule tracks the rate of requests for each originating `IP address`, and triggers the rule action when the rate exceeds a limit that you specify on the number of requests in any `5-minute` time span. This statement can not be nested. See `rate_based_statement` below for details.
*/
@JvmName("uamwyimkbirdutxx")
public suspend fun rateBasedStatement(argument: suspend WebAclRuleStatementRateBasedStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementRateBasedStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rateBasedStatement = mapped
}
/**
* @param value Rule statement used to search web request components for a match against a single regular expression. See `regex_match_statement` below for details.
*/
@JvmName("lssojteejkygpaqx")
public suspend fun regexMatchStatement(`value`: WebAclRuleStatementRegexMatchStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.regexMatchStatement = mapped
}
/**
* @param argument Rule statement used to search web request components for a match against a single regular expression. See `regex_match_statement` below for details.
*/
@JvmName("xjdmdysufibkryou")
public suspend fun regexMatchStatement(argument: suspend WebAclRuleStatementRegexMatchStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementRegexMatchStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.regexMatchStatement = mapped
}
/**
* @param value Rule statement used to search web request components for matches with regular expressions. See `regex_pattern_set_reference_statement` below for details.
*/
@JvmName("lnoqpydfovnfwhsj")
public suspend fun regexPatternSetReferenceStatement(`value`: WebAclRuleStatementRegexPatternSetReferenceStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.regexPatternSetReferenceStatement = mapped
}
/**
* @param argument Rule statement used to search web request components for matches with regular expressions. See `regex_pattern_set_reference_statement` below for details.
*/
@JvmName("yiepgyparolgvrmi")
public suspend fun regexPatternSetReferenceStatement(argument: suspend WebAclRuleStatementRegexPatternSetReferenceStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementRegexPatternSetReferenceStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.regexPatternSetReferenceStatement = mapped
}
/**
* @param value Rule statement used to run the rules that are defined in an WAFv2 Rule Group. See `rule_group_reference_statement` below for details.
*/
@JvmName("bxxrchoqmksqngwx")
public suspend fun ruleGroupReferenceStatement(`value`: WebAclRuleStatementRuleGroupReferenceStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ruleGroupReferenceStatement = mapped
}
/**
* @param argument Rule statement used to run the rules that are defined in an WAFv2 Rule Group. See `rule_group_reference_statement` below for details.
*/
@JvmName("bwkmsrqxaxffiqnx")
public suspend fun ruleGroupReferenceStatement(argument: suspend WebAclRuleStatementRuleGroupReferenceStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementRuleGroupReferenceStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.ruleGroupReferenceStatement = mapped
}
/**
* @param value Rule statement that compares a number of bytes against the size of a request component, using a comparison operator, such as greater than (>) or less than (<). See `size_constraint_statement` below for more details.
*/
@JvmName("omlddkyhnwidjteq")
public suspend fun sizeConstraintStatement(`value`: WebAclRuleStatementSizeConstraintStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sizeConstraintStatement = mapped
}
/**
* @param argument Rule statement that compares a number of bytes against the size of a request component, using a comparison operator, such as greater than (>) or less than (<). See `size_constraint_statement` below for more details.
*/
@JvmName("dnaklwhiapknosjp")
public suspend fun sizeConstraintStatement(argument: suspend WebAclRuleStatementSizeConstraintStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementSizeConstraintStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sizeConstraintStatement = mapped
}
/**
* @param value An SQL injection match condition identifies the part of web requests, such as the URI or the query string, that you want AWS WAF to inspect. See `sqli_match_statement` below for details.
*/
@JvmName("phraabxoiaknhuna")
public suspend fun sqliMatchStatement(`value`: WebAclRuleStatementSqliMatchStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sqliMatchStatement = mapped
}
/**
* @param argument An SQL injection match condition identifies the part of web requests, such as the URI or the query string, that you want AWS WAF to inspect. See `sqli_match_statement` below for details.
*/
@JvmName("qjkyvxsqfeoxxaek")
public suspend fun sqliMatchStatement(argument: suspend WebAclRuleStatementSqliMatchStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementSqliMatchStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sqliMatchStatement = mapped
}
/**
* @param value Rule statement that defines a cross-site scripting (XSS) match search for AWS WAF to apply to web requests. See `xss_match_statement` below for details.
*/
@JvmName("rydurbyljqvqespd")
public suspend fun xssMatchStatement(`value`: WebAclRuleStatementXssMatchStatementArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.xssMatchStatement = mapped
}
/**
* @param argument Rule statement that defines a cross-site scripting (XSS) match search for AWS WAF to apply to web requests. See `xss_match_statement` below for details.
*/
@JvmName("pegyhcgmnkenitod")
public suspend fun xssMatchStatement(argument: suspend WebAclRuleStatementXssMatchStatementArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementXssMatchStatementArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.xssMatchStatement = mapped
}
internal fun build(): WebAclRuleStatementArgs = WebAclRuleStatementArgs(
andStatement = andStatement,
byteMatchStatement = byteMatchStatement,
geoMatchStatement = geoMatchStatement,
ipSetReferenceStatement = ipSetReferenceStatement,
labelMatchStatement = labelMatchStatement,
managedRuleGroupStatement = managedRuleGroupStatement,
notStatement = notStatement,
orStatement = orStatement,
rateBasedStatement = rateBasedStatement,
regexMatchStatement = regexMatchStatement,
regexPatternSetReferenceStatement = regexPatternSetReferenceStatement,
ruleGroupReferenceStatement = ruleGroupReferenceStatement,
sizeConstraintStatement = sizeConstraintStatement,
sqliMatchStatement = sqliMatchStatement,
xssMatchStatement = xssMatchStatement,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy