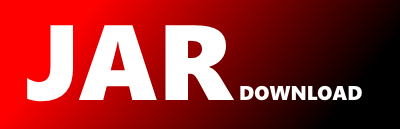
com.pulumi.aws.wafv2.kotlin.inputs.WebAclRuleStatementRateBasedStatementCustomKeyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.wafv2.kotlin.inputs
import com.pulumi.aws.wafv2.inputs.WebAclRuleStatementRateBasedStatementCustomKeyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property cookie Use the value of a cookie in the request as an aggregate key. See RateLimit `cookie` below for details.
* @property forwardedIp Use the first IP address in an HTTP header as an aggregate key. See `forwarded_ip` below for details.
* @property header Use the value of a header in the request as an aggregate key. See RateLimit `header` below for details.
* @property httpMethod Use the request's HTTP method as an aggregate key. See RateLimit `http_method` below for details.
* @property ip Use the request's originating IP address as an aggregate key. See `RateLimit ip` below for details.
* @property labelNamespace Use the specified label namespace as an aggregate key. See RateLimit `label_namespace` below for details.
* @property queryArgument Use the specified query argument as an aggregate key. See RateLimit `query_argument` below for details.
* @property queryString Use the request's query string as an aggregate key. See RateLimit `query_string` below for details.
* @property uriPath Use the request's URI path as an aggregate key. See RateLimit `uri_path` below for details.
*/
public data class WebAclRuleStatementRateBasedStatementCustomKeyArgs(
public val cookie: Output? = null,
public val forwardedIp: Output? =
null,
public val `header`: Output? = null,
public val httpMethod: Output? =
null,
public val ip: Output? = null,
public val labelNamespace: Output? = null,
public val queryArgument: Output? =
null,
public val queryString: Output? =
null,
public val uriPath: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.wafv2.inputs.WebAclRuleStatementRateBasedStatementCustomKeyArgs =
com.pulumi.aws.wafv2.inputs.WebAclRuleStatementRateBasedStatementCustomKeyArgs.builder()
.cookie(cookie?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.forwardedIp(forwardedIp?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.`header`(`header`?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.httpMethod(httpMethod?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ip(ip?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.labelNamespace(labelNamespace?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.queryArgument(queryArgument?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.queryString(queryString?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.uriPath(uriPath?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [WebAclRuleStatementRateBasedStatementCustomKeyArgs].
*/
@PulumiTagMarker
public class WebAclRuleStatementRateBasedStatementCustomKeyArgsBuilder internal constructor() {
private var cookie: Output? = null
private var forwardedIp: Output? =
null
private var `header`: Output? = null
private var httpMethod: Output? =
null
private var ip: Output? = null
private var labelNamespace:
Output? = null
private var queryArgument:
Output? = null
private var queryString: Output? =
null
private var uriPath: Output? = null
/**
* @param value Use the value of a cookie in the request as an aggregate key. See RateLimit `cookie` below for details.
*/
@JvmName("tgepsedcoaivwuxb")
public suspend fun cookie(`value`: Output) {
this.cookie = value
}
/**
* @param value Use the first IP address in an HTTP header as an aggregate key. See `forwarded_ip` below for details.
*/
@JvmName("cnsidbxsmpmdgbvt")
public suspend fun forwardedIp(`value`: Output) {
this.forwardedIp = value
}
/**
* @param value Use the value of a header in the request as an aggregate key. See RateLimit `header` below for details.
*/
@JvmName("gnadhtmslvxclswe")
public suspend fun `header`(`value`: Output) {
this.`header` = value
}
/**
* @param value Use the request's HTTP method as an aggregate key. See RateLimit `http_method` below for details.
*/
@JvmName("uqgsgcdyiwvawujt")
public suspend fun httpMethod(`value`: Output) {
this.httpMethod = value
}
/**
* @param value Use the request's originating IP address as an aggregate key. See `RateLimit ip` below for details.
*/
@JvmName("xqicareffilhykeb")
public suspend fun ip(`value`: Output) {
this.ip = value
}
/**
* @param value Use the specified label namespace as an aggregate key. See RateLimit `label_namespace` below for details.
*/
@JvmName("hnbufoddouwnsltl")
public suspend fun labelNamespace(`value`: Output) {
this.labelNamespace = value
}
/**
* @param value Use the specified query argument as an aggregate key. See RateLimit `query_argument` below for details.
*/
@JvmName("ragecyrdaglkbijh")
public suspend fun queryArgument(`value`: Output) {
this.queryArgument = value
}
/**
* @param value Use the request's query string as an aggregate key. See RateLimit `query_string` below for details.
*/
@JvmName("jyqyewnmistoubdx")
public suspend fun queryString(`value`: Output) {
this.queryString = value
}
/**
* @param value Use the request's URI path as an aggregate key. See RateLimit `uri_path` below for details.
*/
@JvmName("ukwjrsnpayxxllkt")
public suspend fun uriPath(`value`: Output) {
this.uriPath = value
}
/**
* @param value Use the value of a cookie in the request as an aggregate key. See RateLimit `cookie` below for details.
*/
@JvmName("wdiyltewmkivvckg")
public suspend fun cookie(`value`: WebAclRuleStatementRateBasedStatementCustomKeyCookieArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cookie = mapped
}
/**
* @param argument Use the value of a cookie in the request as an aggregate key. See RateLimit `cookie` below for details.
*/
@JvmName("iujbhaifqffsobbd")
public suspend fun cookie(argument: suspend WebAclRuleStatementRateBasedStatementCustomKeyCookieArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementRateBasedStatementCustomKeyCookieArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cookie = mapped
}
/**
* @param value Use the first IP address in an HTTP header as an aggregate key. See `forwarded_ip` below for details.
*/
@JvmName("ujoteikdtueoamcs")
public suspend fun forwardedIp(`value`: WebAclRuleStatementRateBasedStatementCustomKeyForwardedIpArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.forwardedIp = mapped
}
/**
* @param argument Use the first IP address in an HTTP header as an aggregate key. See `forwarded_ip` below for details.
*/
@JvmName("omrchkagqjygxwbn")
public suspend fun forwardedIp(argument: suspend WebAclRuleStatementRateBasedStatementCustomKeyForwardedIpArgsBuilder.() -> Unit) {
val toBeMapped =
WebAclRuleStatementRateBasedStatementCustomKeyForwardedIpArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.forwardedIp = mapped
}
/**
* @param value Use the value of a header in the request as an aggregate key. See RateLimit `header` below for details.
*/
@JvmName("uyamgoiftgerdcxh")
public suspend fun `header`(`value`: WebAclRuleStatementRateBasedStatementCustomKeyHeaderArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`header` = mapped
}
/**
* @param argument Use the value of a header in the request as an aggregate key. See RateLimit `header` below for details.
*/
@JvmName("xwhfaythslitqfxc")
public suspend fun `header`(argument: suspend WebAclRuleStatementRateBasedStatementCustomKeyHeaderArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementRateBasedStatementCustomKeyHeaderArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.`header` = mapped
}
/**
* @param value Use the request's HTTP method as an aggregate key. See RateLimit `http_method` below for details.
*/
@JvmName("jndbdwcekmjjtudg")
public suspend fun httpMethod(`value`: WebAclRuleStatementRateBasedStatementCustomKeyHttpMethodArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.httpMethod = mapped
}
/**
* @param argument Use the request's HTTP method as an aggregate key. See RateLimit `http_method` below for details.
*/
@JvmName("aduocvtamgfhqrfm")
public suspend fun httpMethod(argument: suspend WebAclRuleStatementRateBasedStatementCustomKeyHttpMethodArgsBuilder.() -> Unit) {
val toBeMapped =
WebAclRuleStatementRateBasedStatementCustomKeyHttpMethodArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.httpMethod = mapped
}
/**
* @param value Use the request's originating IP address as an aggregate key. See `RateLimit ip` below for details.
*/
@JvmName("okfhttlqcepocpmp")
public suspend fun ip(`value`: WebAclRuleStatementRateBasedStatementCustomKeyIpArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ip = mapped
}
/**
* @param argument Use the request's originating IP address as an aggregate key. See `RateLimit ip` below for details.
*/
@JvmName("bdscctaytrbkrkwy")
public suspend fun ip(argument: suspend WebAclRuleStatementRateBasedStatementCustomKeyIpArgsBuilder.() -> Unit) {
val toBeMapped = WebAclRuleStatementRateBasedStatementCustomKeyIpArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.ip = mapped
}
/**
* @param value Use the specified label namespace as an aggregate key. See RateLimit `label_namespace` below for details.
*/
@JvmName("glqbhlsowlqnqtag")
public suspend fun labelNamespace(`value`: WebAclRuleStatementRateBasedStatementCustomKeyLabelNamespaceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.labelNamespace = mapped
}
/**
* @param argument Use the specified label namespace as an aggregate key. See RateLimit `label_namespace` below for details.
*/
@JvmName("kbaewexysihxfkjt")
public suspend fun labelNamespace(argument: suspend WebAclRuleStatementRateBasedStatementCustomKeyLabelNamespaceArgsBuilder.() -> Unit) {
val toBeMapped =
WebAclRuleStatementRateBasedStatementCustomKeyLabelNamespaceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.labelNamespace = mapped
}
/**
* @param value Use the specified query argument as an aggregate key. See RateLimit `query_argument` below for details.
*/
@JvmName("flpcclyswhjkpyod")
public suspend fun queryArgument(`value`: WebAclRuleStatementRateBasedStatementCustomKeyQueryArgumentArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queryArgument = mapped
}
/**
* @param argument Use the specified query argument as an aggregate key. See RateLimit `query_argument` below for details.
*/
@JvmName("fiuabmbkfqbmrjdk")
public suspend fun queryArgument(argument: suspend WebAclRuleStatementRateBasedStatementCustomKeyQueryArgumentArgsBuilder.() -> Unit) {
val toBeMapped =
WebAclRuleStatementRateBasedStatementCustomKeyQueryArgumentArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.queryArgument = mapped
}
/**
* @param value Use the request's query string as an aggregate key. See RateLimit `query_string` below for details.
*/
@JvmName("nvhfxbixucifiaue")
public suspend fun queryString(`value`: WebAclRuleStatementRateBasedStatementCustomKeyQueryStringArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queryString = mapped
}
/**
* @param argument Use the request's query string as an aggregate key. See RateLimit `query_string` below for details.
*/
@JvmName("cqymgdkekqafepkw")
public suspend fun queryString(argument: suspend WebAclRuleStatementRateBasedStatementCustomKeyQueryStringArgsBuilder.() -> Unit) {
val toBeMapped =
WebAclRuleStatementRateBasedStatementCustomKeyQueryStringArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.queryString = mapped
}
/**
* @param value Use the request's URI path as an aggregate key. See RateLimit `uri_path` below for details.
*/
@JvmName("omljkitoemxmysjb")
public suspend fun uriPath(`value`: WebAclRuleStatementRateBasedStatementCustomKeyUriPathArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.uriPath = mapped
}
/**
* @param argument Use the request's URI path as an aggregate key. See RateLimit `uri_path` below for details.
*/
@JvmName("piahuchsorvgidpm")
public suspend fun uriPath(argument: suspend WebAclRuleStatementRateBasedStatementCustomKeyUriPathArgsBuilder.() -> Unit) {
val toBeMapped =
WebAclRuleStatementRateBasedStatementCustomKeyUriPathArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.uriPath = mapped
}
internal fun build(): WebAclRuleStatementRateBasedStatementCustomKeyArgs =
WebAclRuleStatementRateBasedStatementCustomKeyArgs(
cookie = cookie,
forwardedIp = forwardedIp,
`header` = `header`,
httpMethod = httpMethod,
ip = ip,
labelNamespace = labelNamespace,
queryArgument = queryArgument,
queryString = queryString,
uriPath = uriPath,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy