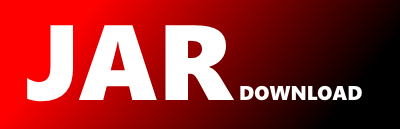
com.pulumi.aws.wafv2.kotlin.inputs.WebAclVisibilityConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.wafv2.kotlin.inputs
import com.pulumi.aws.wafv2.inputs.WebAclVisibilityConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property cloudwatchMetricsEnabled Whether the associated resource sends metrics to CloudWatch. For the list of available metrics, see [AWS WAF Metrics](https://docs.aws.amazon.com/waf/latest/developerguide/monitoring-cloudwatch.html#waf-metrics).
* @property metricName A friendly name of the CloudWatch metric. The name can contain only alphanumeric characters (A-Z, a-z, 0-9) hyphen(-) and underscore (\_), with length from one to 128 characters. It can't contain whitespace or metric names reserved for AWS WAF, for example `All` and `Default_Action`.
* @property sampledRequestsEnabled Whether AWS WAF should store a sampling of the web requests that match the rules. You can view the sampled requests through the AWS WAF console.
*/
public data class WebAclVisibilityConfigArgs(
public val cloudwatchMetricsEnabled: Output,
public val metricName: Output,
public val sampledRequestsEnabled: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.wafv2.inputs.WebAclVisibilityConfigArgs =
com.pulumi.aws.wafv2.inputs.WebAclVisibilityConfigArgs.builder()
.cloudwatchMetricsEnabled(cloudwatchMetricsEnabled.applyValue({ args0 -> args0 }))
.metricName(metricName.applyValue({ args0 -> args0 }))
.sampledRequestsEnabled(sampledRequestsEnabled.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WebAclVisibilityConfigArgs].
*/
@PulumiTagMarker
public class WebAclVisibilityConfigArgsBuilder internal constructor() {
private var cloudwatchMetricsEnabled: Output? = null
private var metricName: Output? = null
private var sampledRequestsEnabled: Output? = null
/**
* @param value Whether the associated resource sends metrics to CloudWatch. For the list of available metrics, see [AWS WAF Metrics](https://docs.aws.amazon.com/waf/latest/developerguide/monitoring-cloudwatch.html#waf-metrics).
*/
@JvmName("prqnxyrguddnpxdm")
public suspend fun cloudwatchMetricsEnabled(`value`: Output) {
this.cloudwatchMetricsEnabled = value
}
/**
* @param value A friendly name of the CloudWatch metric. The name can contain only alphanumeric characters (A-Z, a-z, 0-9) hyphen(-) and underscore (\_), with length from one to 128 characters. It can't contain whitespace or metric names reserved for AWS WAF, for example `All` and `Default_Action`.
*/
@JvmName("uggpniqcskoamabh")
public suspend fun metricName(`value`: Output) {
this.metricName = value
}
/**
* @param value Whether AWS WAF should store a sampling of the web requests that match the rules. You can view the sampled requests through the AWS WAF console.
*/
@JvmName("vvgxgtfnaurxlred")
public suspend fun sampledRequestsEnabled(`value`: Output) {
this.sampledRequestsEnabled = value
}
/**
* @param value Whether the associated resource sends metrics to CloudWatch. For the list of available metrics, see [AWS WAF Metrics](https://docs.aws.amazon.com/waf/latest/developerguide/monitoring-cloudwatch.html#waf-metrics).
*/
@JvmName("tpkefxfxrhmocoiw")
public suspend fun cloudwatchMetricsEnabled(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.cloudwatchMetricsEnabled = mapped
}
/**
* @param value A friendly name of the CloudWatch metric. The name can contain only alphanumeric characters (A-Z, a-z, 0-9) hyphen(-) and underscore (\_), with length from one to 128 characters. It can't contain whitespace or metric names reserved for AWS WAF, for example `All` and `Default_Action`.
*/
@JvmName("ariolluxnbglovfd")
public suspend fun metricName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.metricName = mapped
}
/**
* @param value Whether AWS WAF should store a sampling of the web requests that match the rules. You can view the sampled requests through the AWS WAF console.
*/
@JvmName("nspqgurjtxtjftmh")
public suspend fun sampledRequestsEnabled(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sampledRequestsEnabled = mapped
}
internal fun build(): WebAclVisibilityConfigArgs = WebAclVisibilityConfigArgs(
cloudwatchMetricsEnabled = cloudwatchMetricsEnabled ?: throw
PulumiNullFieldException("cloudwatchMetricsEnabled"),
metricName = metricName ?: throw PulumiNullFieldException("metricName"),
sampledRequestsEnabled = sampledRequestsEnabled ?: throw
PulumiNullFieldException("sampledRequestsEnabled"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy