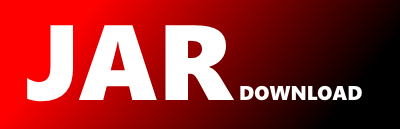
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatement.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.wafv2.kotlin.outputs
import kotlin.Suppress
/**
*
* @property andStatement A logical rule statement used to combine other rule statements with AND logic. See AND Statement below for details.
* @property byteMatchStatement A rule statement that defines a string match search for AWS WAF to apply to web requests. See Byte Match Statement below for details.
* @property geoMatchStatement A rule statement used to identify web requests based on country of origin. See GEO Match Statement below for details.
* @property ipSetReferenceStatement A rule statement used to detect web requests coming from particular IP addresses or address ranges. See IP Set Reference Statement below for details.
* @property labelMatchStatement A rule statement that defines a string match search against labels that have been added to the web request by rules that have already run in the web ACL. See Label Match Statement below for details.
* @property notStatement A logical rule statement used to negate the results of another rule statement. See NOT Statement below for details.
* @property orStatement A logical rule statement used to combine other rule statements with OR logic. See OR Statement below for details.
* @property rateBasedStatement A rate-based rule tracks the rate of requests for each originating `IP address`, and triggers the rule action when the rate exceeds a limit that you specify on the number of requests in any `5-minute` time span. This statement can not be nested. See Rate Based Statement below for details.
* @property regexMatchStatement A rule statement used to search web request components for a match against a single regular expression. See Regex Match Statement below for details.
* @property regexPatternSetReferenceStatement A rule statement used to search web request components for matches with regular expressions. See Regex Pattern Set Reference Statement below for details.
* @property sizeConstraintStatement A rule statement that compares a number of bytes against the size of a request component, using a comparison operator, such as greater than (>) or less than (<). See Size Constraint Statement below for more details.
* @property sqliMatchStatement An SQL injection match condition identifies the part of web requests, such as the URI or the query string, that you want AWS WAF to inspect. See SQL Injection Match Statement below for details.
* @property xssMatchStatement A rule statement that defines a cross-site scripting (XSS) match search for AWS WAF to apply to web requests. See XSS Match Statement below for details.
*/
public data class RuleGroupRuleStatement(
public val andStatement: RuleGroupRuleStatementAndStatement? = null,
public val byteMatchStatement: RuleGroupRuleStatementByteMatchStatement? = null,
public val geoMatchStatement: RuleGroupRuleStatementGeoMatchStatement? = null,
public val ipSetReferenceStatement: RuleGroupRuleStatementIpSetReferenceStatement? = null,
public val labelMatchStatement: RuleGroupRuleStatementLabelMatchStatement? = null,
public val notStatement: RuleGroupRuleStatementNotStatement? = null,
public val orStatement: RuleGroupRuleStatementOrStatement? = null,
public val rateBasedStatement: RuleGroupRuleStatementRateBasedStatement? = null,
public val regexMatchStatement: RuleGroupRuleStatementRegexMatchStatement? = null,
public val regexPatternSetReferenceStatement: RuleGroupRuleStatementRegexPatternSetReferenceStatement? = null,
public val sizeConstraintStatement: RuleGroupRuleStatementSizeConstraintStatement? = null,
public val sqliMatchStatement: RuleGroupRuleStatementSqliMatchStatement? = null,
public val xssMatchStatement: RuleGroupRuleStatementXssMatchStatement? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.wafv2.outputs.RuleGroupRuleStatement): RuleGroupRuleStatement = RuleGroupRuleStatement(
andStatement = javaType.andStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementAndStatement.Companion.toKotlin(args0)
})
}).orElse(null),
byteMatchStatement = javaType.byteMatchStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementByteMatchStatement.Companion.toKotlin(args0)
})
}).orElse(null),
geoMatchStatement = javaType.geoMatchStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementGeoMatchStatement.Companion.toKotlin(args0)
})
}).orElse(null),
ipSetReferenceStatement = javaType.ipSetReferenceStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementIpSetReferenceStatement.Companion.toKotlin(args0)
})
}).orElse(null),
labelMatchStatement = javaType.labelMatchStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementLabelMatchStatement.Companion.toKotlin(args0)
})
}).orElse(null),
notStatement = javaType.notStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementNotStatement.Companion.toKotlin(args0)
})
}).orElse(null),
orStatement = javaType.orStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementOrStatement.Companion.toKotlin(args0)
})
}).orElse(null),
rateBasedStatement = javaType.rateBasedStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementRateBasedStatement.Companion.toKotlin(args0)
})
}).orElse(null),
regexMatchStatement = javaType.regexMatchStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementRegexMatchStatement.Companion.toKotlin(args0)
})
}).orElse(null),
regexPatternSetReferenceStatement = javaType.regexPatternSetReferenceStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementRegexPatternSetReferenceStatement.Companion.toKotlin(args0)
})
}).orElse(null),
sizeConstraintStatement = javaType.sizeConstraintStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementSizeConstraintStatement.Companion.toKotlin(args0)
})
}).orElse(null),
sqliMatchStatement = javaType.sqliMatchStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementSqliMatchStatement.Companion.toKotlin(args0)
})
}).orElse(null),
xssMatchStatement = javaType.xssMatchStatement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.RuleGroupRuleStatementXssMatchStatement.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy