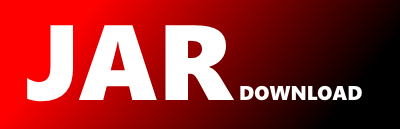
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatch.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.wafv2.kotlin.outputs
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property allQueryArguments Inspect all query arguments.
* @property body Inspect the request body, which immediately follows the request headers. See `body` below for details.
* @property cookies Inspect the cookies in the web request. See `cookies` below for details.
* @property headerOrders Inspect a string containing the list of the request's header names, ordered as they appear in the web request that AWS WAF receives for inspection. See `header_order` below for details.
* @property headers Inspect the request headers. See `headers` below for details.
* @property ja3Fingerprint Inspect the JA3 fingerprint. See `ja3_fingerprint` below for details.
* @property jsonBody Inspect the request body as JSON. See `json_body` for details.
* @property method Inspect the HTTP method. The method indicates the type of operation that the request is asking the origin to perform.
* @property queryString Inspect the query string. This is the part of a URL that appears after a `?` character, if any.
* @property singleHeader Inspect a single header. See `single_header` below for details.
* @property singleQueryArgument Inspect a single query argument. See `single_query_argument` below for details.
* @property uriPath Inspect the request URI path. This is the part of a web request that identifies a resource, for example, `/images/daily-ad.jpg`.
*/
public data class
WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatch(
public val allQueryArguments: WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchAllQueryArguments? =
null,
public val body: WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchBody? =
null,
public val cookies: WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchCookies? =
null,
public val headerOrders: List? =
null,
public val headers: List? =
null,
public val ja3Fingerprint: WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchJa3Fingerprint? =
null,
public val jsonBody: WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchJsonBody? =
null,
public val method: WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchMethod? =
null,
public val queryString: WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchQueryString? =
null,
public val singleHeader: WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchSingleHeader? =
null,
public val singleQueryArgument: WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchSingleQueryArgument? =
null,
public val uriPath: WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchUriPath? =
null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.wafv2.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatch): WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatch =
WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatch(
allQueryArguments = javaType.allQueryArguments().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchAllQueryArguments.Companion.toKotlin(args0)
})
}).orElse(null),
body = javaType.body().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchBody.Companion.toKotlin(args0)
})
}).orElse(null),
cookies = javaType.cookies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchCookies.Companion.toKotlin(args0)
})
}).orElse(null),
headerOrders = javaType.headerOrders().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchHeaderOrder.Companion.toKotlin(args0)
})
}),
headers = javaType.headers().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchHeader.Companion.toKotlin(args0)
})
}),
ja3Fingerprint = javaType.ja3Fingerprint().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchJa3Fingerprint.Companion.toKotlin(args0)
})
}).orElse(null),
jsonBody = javaType.jsonBody().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchJsonBody.Companion.toKotlin(args0)
})
}).orElse(null),
method = javaType.method().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchMethod.Companion.toKotlin(args0)
})
}).orElse(null),
queryString = javaType.queryString().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchQueryString.Companion.toKotlin(args0)
})
}).orElse(null),
singleHeader = javaType.singleHeader().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchSingleHeader.Companion.toKotlin(args0)
})
}).orElse(null),
singleQueryArgument = javaType.singleQueryArgument().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchSingleQueryArgument.Companion.toKotlin(args0)
})
}).orElse(null),
uriPath = javaType.uriPath().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.wafv2.kotlin.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatementXssMatchStatementFieldToMatchUriPath.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy