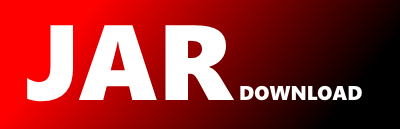
com.pulumi.aws.xray.kotlin.EncryptionConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.xray.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [EncryptionConfig].
*/
@PulumiTagMarker
public class EncryptionConfigResourceBuilder internal constructor() {
public var name: String? = null
public var args: EncryptionConfigArgs = EncryptionConfigArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend EncryptionConfigArgsBuilder.() -> Unit) {
val builder = EncryptionConfigArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): EncryptionConfig {
val builtJavaResource = com.pulumi.aws.xray.EncryptionConfig(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return EncryptionConfig(builtJavaResource)
}
}
/**
* Creates and manages an AWS XRay Encryption Config.
* > **NOTE:** Removing this resource from the provider has no effect to the encryption configuration within X-Ray.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.xray.EncryptionConfig("example", {type: "NONE"});
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.xray.EncryptionConfig("example", type="NONE")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Xray.EncryptionConfig("example", new()
* {
* Type = "NONE",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/xray"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := xray.NewEncryptionConfig(ctx, "example", &xray.EncryptionConfigArgs{
* Type: pulumi.String("NONE"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.xray.EncryptionConfig;
* import com.pulumi.aws.xray.EncryptionConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new EncryptionConfig("example", EncryptionConfigArgs.builder()
* .type("NONE")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:xray:EncryptionConfig
* properties:
* type: NONE
* ```
*
* ### With KMS Key
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const current = aws.getCallerIdentity({});
* const example = current.then(current => aws.iam.getPolicyDocument({
* statements: [{
* sid: "Enable IAM User Permissions",
* effect: "Allow",
* principals: [{
* type: "AWS",
* identifiers: [`arn:aws:iam::${current.accountId}:root`],
* }],
* actions: ["kms:*"],
* resources: ["*"],
* }],
* }));
* const exampleKey = new aws.kms.Key("example", {
* description: "Some Key",
* deletionWindowInDays: 7,
* policy: example.then(example => example.json),
* });
* const exampleEncryptionConfig = new aws.xray.EncryptionConfig("example", {
* type: "KMS",
* keyId: exampleKey.arn,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* current = aws.get_caller_identity()
* example = aws.iam.get_policy_document(statements=[{
* "sid": "Enable IAM User Permissions",
* "effect": "Allow",
* "principals": [{
* "type": "AWS",
* "identifiers": [f"arn:aws:iam::{current.account_id}:root"],
* }],
* "actions": ["kms:*"],
* "resources": ["*"],
* }])
* example_key = aws.kms.Key("example",
* description="Some Key",
* deletion_window_in_days=7,
* policy=example.json)
* example_encryption_config = aws.xray.EncryptionConfig("example",
* type="KMS",
* key_id=example_key.arn)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var current = Aws.GetCallerIdentity.Invoke();
* var example = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Sid = "Enable IAM User Permissions",
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "AWS",
* Identifiers = new[]
* {
* $"arn:aws:iam::{current.Apply(getCallerIdentityResult => getCallerIdentityResult.AccountId)}:root",
* },
* },
* },
* Actions = new[]
* {
* "kms:*",
* },
* Resources = new[]
* {
* "*",
* },
* },
* },
* });
* var exampleKey = new Aws.Kms.Key("example", new()
* {
* Description = "Some Key",
* DeletionWindowInDays = 7,
* Policy = example.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var exampleEncryptionConfig = new Aws.Xray.EncryptionConfig("example", new()
* {
* Type = "KMS",
* KeyId = exampleKey.Arn,
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/xray"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := aws.GetCallerIdentity(ctx, &aws.GetCallerIdentityArgs{}, nil)
* if err != nil {
* return err
* }
* example, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Sid: pulumi.StringRef("Enable IAM User Permissions"),
* Effect: pulumi.StringRef("Allow"),
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "AWS",
* Identifiers: []string{
* fmt.Sprintf("arn:aws:iam::%v:root", current.AccountId),
* },
* },
* },
* Actions: []string{
* "kms:*",
* },
* Resources: []string{
* "*",
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* exampleKey, err := kms.NewKey(ctx, "example", &kms.KeyArgs{
* Description: pulumi.String("Some Key"),
* DeletionWindowInDays: pulumi.Int(7),
* Policy: pulumi.String(example.Json),
* })
* if err != nil {
* return err
* }
* _, err = xray.NewEncryptionConfig(ctx, "example", &xray.EncryptionConfigArgs{
* Type: pulumi.String("KMS"),
* KeyId: exampleKey.Arn,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetCallerIdentityArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.kms.Key;
* import com.pulumi.aws.kms.KeyArgs;
* import com.pulumi.aws.xray.EncryptionConfig;
* import com.pulumi.aws.xray.EncryptionConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = AwsFunctions.getCallerIdentity();
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .sid("Enable IAM User Permissions")
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("AWS")
* .identifiers(String.format("arn:aws:iam::%s:root", current.applyValue(getCallerIdentityResult -> getCallerIdentityResult.accountId())))
* .build())
* .actions("kms:*")
* .resources("*")
* .build())
* .build());
* var exampleKey = new Key("exampleKey", KeyArgs.builder()
* .description("Some Key")
* .deletionWindowInDays(7)
* .policy(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* var exampleEncryptionConfig = new EncryptionConfig("exampleEncryptionConfig", EncryptionConfigArgs.builder()
* .type("KMS")
* .keyId(exampleKey.arn())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleKey:
* type: aws:kms:Key
* name: example
* properties:
* description: Some Key
* deletionWindowInDays: 7
* policy: ${example.json}
* exampleEncryptionConfig:
* type: aws:xray:EncryptionConfig
* name: example
* properties:
* type: KMS
* keyId: ${exampleKey.arn}
* variables:
* current:
* fn::invoke:
* Function: aws:getCallerIdentity
* Arguments: {}
* example:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - sid: Enable IAM User Permissions
* effect: Allow
* principals:
* - type: AWS
* identifiers:
* - arn:aws:iam::${current.accountId}:root
* actions:
* - kms:*
* resources:
* - '*'
* ```
*
* ## Import
* Using `pulumi import`, import XRay Encryption Config using the region name. For example:
* ```sh
* $ pulumi import aws:xray/encryptionConfig:EncryptionConfig example us-west-2
* ```
*/
public class EncryptionConfig internal constructor(
override val javaResource: com.pulumi.aws.xray.EncryptionConfig,
) : KotlinCustomResource(javaResource, EncryptionConfigMapper) {
/**
* An AWS KMS customer master key (CMK) ARN.
*/
public val keyId: Output?
get() = javaResource.keyId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The type of encryption. Set to `KMS` to use your own key for encryption. Set to `NONE` for default encryption.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
}
public object EncryptionConfigMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.aws.xray.EncryptionConfig::class == javaResource::class
override fun map(javaResource: Resource): EncryptionConfig = EncryptionConfig(
javaResource as
com.pulumi.aws.xray.EncryptionConfig,
)
}
/**
* @see [EncryptionConfig].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [EncryptionConfig].
*/
public suspend fun encryptionConfig(
name: String,
block: suspend EncryptionConfigResourceBuilder.() -> Unit,
): EncryptionConfig {
val builder = EncryptionConfigResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [EncryptionConfig].
* @param name The _unique_ name of the resulting resource.
*/
public fun encryptionConfig(name: String): EncryptionConfig {
val builder = EncryptionConfigResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy