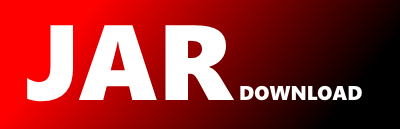
com.pulumi.awsnative.acmpca.kotlin.inputs.CertificateAuthorityCrlConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.acmpca.kotlin.inputs
import com.pulumi.awsnative.acmpca.inputs.CertificateAuthorityCrlConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Your certificate authority can create and maintain a certificate revocation list (CRL). A CRL contains information about certificates that have been revoked.
* @property crlDistributionPointExtensionConfiguration Configures the default behavior of the CRL Distribution Point extension for certificates issued by your CA. If this field is not provided, then the CRL Distribution Point extension will be present and contain the default CRL URL.
* @property customCname Name inserted into the certificate *CRL Distribution Points* extension that enables the use of an alias for the CRL distribution point. Use this value if you don't want the name of your S3 bucket to be public.
* > The content of a Canonical Name (CNAME) record must conform to [RFC2396](https://docs.aws.amazon.com/https://www.ietf.org/rfc/rfc2396.txt) restrictions on the use of special characters in URIs. Additionally, the value of the CNAME must not include a protocol prefix such as "http://" or "https://".
* @property enabled Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this value to enable certificate revocation for a new CA when you call the `CreateCertificateAuthority` operation or for an existing CA when you call the `UpdateCertificateAuthority` operation.
* @property expirationInDays Validity period of the CRL in days.
* @property s3BucketName Name of the S3 bucket that contains the CRL. If you do not provide a value for the *CustomCname* argument, the name of your S3 bucket is placed into the *CRL Distribution Points* extension of the issued certificate. You can change the name of your bucket by calling the [UpdateCertificateAuthority](https://docs.aws.amazon.com/privateca/latest/APIReference/API_UpdateCertificateAuthority.html) operation. You must specify a [bucket policy](https://docs.aws.amazon.com/privateca/latest/userguide/PcaCreateCa.html#s3-policies) that allows AWS Private CA to write the CRL to your bucket.
* > The `S3BucketName` parameter must conform to the [S3 bucket naming rules](https://docs.aws.amazon.com/AmazonS3/latest/userguide/bucketnamingrules.html) .
* @property s3ObjectAcl Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If you choose PUBLIC_READ, the CRL will be accessible over the public internet. If you choose BUCKET_OWNER_FULL_CONTROL, only the owner of the CRL S3 bucket can access the CRL, and your PKI clients may need an alternative method of access.
* If no value is specified, the default is PUBLIC_READ.
* *Note:* This default can cause CA creation to fail in some circumstances. If you have have enabled the Block Public Access (BPA) feature in your S3 account, then you must specify the value of this parameter as `BUCKET_OWNER_FULL_CONTROL` , and not doing so results in an error. If you have disabled BPA in S3, then you can specify either `BUCKET_OWNER_FULL_CONTROL` or `PUBLIC_READ` as the value.
* For more information, see [Blocking public access to the S3 bucket](https://docs.aws.amazon.com/privateca/latest/userguide/PcaCreateCa.html#s3-bpa) .
*/
public data class CertificateAuthorityCrlConfigurationArgs(
public val crlDistributionPointExtensionConfiguration: Output? = null,
public val customCname: Output? = null,
public val enabled: Output,
public val expirationInDays: Output? = null,
public val s3BucketName: Output? = null,
public val s3ObjectAcl: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.acmpca.inputs.CertificateAuthorityCrlConfigurationArgs = com.pulumi.awsnative.acmpca.inputs.CertificateAuthorityCrlConfigurationArgs.builder()
.crlDistributionPointExtensionConfiguration(
crlDistributionPointExtensionConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.customCname(customCname?.applyValue({ args0 -> args0 }))
.enabled(enabled.applyValue({ args0 -> args0 }))
.expirationInDays(expirationInDays?.applyValue({ args0 -> args0 }))
.s3BucketName(s3BucketName?.applyValue({ args0 -> args0 }))
.s3ObjectAcl(s3ObjectAcl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CertificateAuthorityCrlConfigurationArgs].
*/
@PulumiTagMarker
public class CertificateAuthorityCrlConfigurationArgsBuilder internal constructor() {
private var crlDistributionPointExtensionConfiguration:
Output? = null
private var customCname: Output? = null
private var enabled: Output? = null
private var expirationInDays: Output? = null
private var s3BucketName: Output? = null
private var s3ObjectAcl: Output? = null
/**
* @param value Configures the default behavior of the CRL Distribution Point extension for certificates issued by your CA. If this field is not provided, then the CRL Distribution Point extension will be present and contain the default CRL URL.
*/
@JvmName("sabimkbkudvnudla")
public suspend fun crlDistributionPointExtensionConfiguration(`value`: Output) {
this.crlDistributionPointExtensionConfiguration = value
}
/**
* @param value Name inserted into the certificate *CRL Distribution Points* extension that enables the use of an alias for the CRL distribution point. Use this value if you don't want the name of your S3 bucket to be public.
* > The content of a Canonical Name (CNAME) record must conform to [RFC2396](https://docs.aws.amazon.com/https://www.ietf.org/rfc/rfc2396.txt) restrictions on the use of special characters in URIs. Additionally, the value of the CNAME must not include a protocol prefix such as "http://" or "https://".
*/
@JvmName("mxmqpmsahkhvjwgo")
public suspend fun customCname(`value`: Output) {
this.customCname = value
}
/**
* @param value Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this value to enable certificate revocation for a new CA when you call the `CreateCertificateAuthority` operation or for an existing CA when you call the `UpdateCertificateAuthority` operation.
*/
@JvmName("ijcskwfvlafnuwfs")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value Validity period of the CRL in days.
*/
@JvmName("ehuehfpjhqeiaaun")
public suspend fun expirationInDays(`value`: Output) {
this.expirationInDays = value
}
/**
* @param value Name of the S3 bucket that contains the CRL. If you do not provide a value for the *CustomCname* argument, the name of your S3 bucket is placed into the *CRL Distribution Points* extension of the issued certificate. You can change the name of your bucket by calling the [UpdateCertificateAuthority](https://docs.aws.amazon.com/privateca/latest/APIReference/API_UpdateCertificateAuthority.html) operation. You must specify a [bucket policy](https://docs.aws.amazon.com/privateca/latest/userguide/PcaCreateCa.html#s3-policies) that allows AWS Private CA to write the CRL to your bucket.
* > The `S3BucketName` parameter must conform to the [S3 bucket naming rules](https://docs.aws.amazon.com/AmazonS3/latest/userguide/bucketnamingrules.html) .
*/
@JvmName("tbgsujdtnxcibplr")
public suspend fun s3BucketName(`value`: Output) {
this.s3BucketName = value
}
/**
* @param value Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If you choose PUBLIC_READ, the CRL will be accessible over the public internet. If you choose BUCKET_OWNER_FULL_CONTROL, only the owner of the CRL S3 bucket can access the CRL, and your PKI clients may need an alternative method of access.
* If no value is specified, the default is PUBLIC_READ.
* *Note:* This default can cause CA creation to fail in some circumstances. If you have have enabled the Block Public Access (BPA) feature in your S3 account, then you must specify the value of this parameter as `BUCKET_OWNER_FULL_CONTROL` , and not doing so results in an error. If you have disabled BPA in S3, then you can specify either `BUCKET_OWNER_FULL_CONTROL` or `PUBLIC_READ` as the value.
* For more information, see [Blocking public access to the S3 bucket](https://docs.aws.amazon.com/privateca/latest/userguide/PcaCreateCa.html#s3-bpa) .
*/
@JvmName("jmhbxsgfjripiaom")
public suspend fun s3ObjectAcl(`value`: Output) {
this.s3ObjectAcl = value
}
/**
* @param value Configures the default behavior of the CRL Distribution Point extension for certificates issued by your CA. If this field is not provided, then the CRL Distribution Point extension will be present and contain the default CRL URL.
*/
@JvmName("bhnnqlgeurjhjyfy")
public suspend fun crlDistributionPointExtensionConfiguration(`value`: CertificateAuthorityCrlDistributionPointExtensionConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crlDistributionPointExtensionConfiguration = mapped
}
/**
* @param argument Configures the default behavior of the CRL Distribution Point extension for certificates issued by your CA. If this field is not provided, then the CRL Distribution Point extension will be present and contain the default CRL URL.
*/
@JvmName("vqhhpyefyuitrhpb")
public suspend fun crlDistributionPointExtensionConfiguration(argument: suspend CertificateAuthorityCrlDistributionPointExtensionConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
CertificateAuthorityCrlDistributionPointExtensionConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.crlDistributionPointExtensionConfiguration = mapped
}
/**
* @param value Name inserted into the certificate *CRL Distribution Points* extension that enables the use of an alias for the CRL distribution point. Use this value if you don't want the name of your S3 bucket to be public.
* > The content of a Canonical Name (CNAME) record must conform to [RFC2396](https://docs.aws.amazon.com/https://www.ietf.org/rfc/rfc2396.txt) restrictions on the use of special characters in URIs. Additionally, the value of the CNAME must not include a protocol prefix such as "http://" or "https://".
*/
@JvmName("iyodhdhwcbiikyin")
public suspend fun customCname(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customCname = mapped
}
/**
* @param value Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this value to enable certificate revocation for a new CA when you call the `CreateCertificateAuthority` operation or for an existing CA when you call the `UpdateCertificateAuthority` operation.
*/
@JvmName("nwvvotdiyqgyplct")
public suspend fun enabled(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value Validity period of the CRL in days.
*/
@JvmName("nwvdubbumiyjoexx")
public suspend fun expirationInDays(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expirationInDays = mapped
}
/**
* @param value Name of the S3 bucket that contains the CRL. If you do not provide a value for the *CustomCname* argument, the name of your S3 bucket is placed into the *CRL Distribution Points* extension of the issued certificate. You can change the name of your bucket by calling the [UpdateCertificateAuthority](https://docs.aws.amazon.com/privateca/latest/APIReference/API_UpdateCertificateAuthority.html) operation. You must specify a [bucket policy](https://docs.aws.amazon.com/privateca/latest/userguide/PcaCreateCa.html#s3-policies) that allows AWS Private CA to write the CRL to your bucket.
* > The `S3BucketName` parameter must conform to the [S3 bucket naming rules](https://docs.aws.amazon.com/AmazonS3/latest/userguide/bucketnamingrules.html) .
*/
@JvmName("fnxbnhqsopgerilo")
public suspend fun s3BucketName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3BucketName = mapped
}
/**
* @param value Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If you choose PUBLIC_READ, the CRL will be accessible over the public internet. If you choose BUCKET_OWNER_FULL_CONTROL, only the owner of the CRL S3 bucket can access the CRL, and your PKI clients may need an alternative method of access.
* If no value is specified, the default is PUBLIC_READ.
* *Note:* This default can cause CA creation to fail in some circumstances. If you have have enabled the Block Public Access (BPA) feature in your S3 account, then you must specify the value of this parameter as `BUCKET_OWNER_FULL_CONTROL` , and not doing so results in an error. If you have disabled BPA in S3, then you can specify either `BUCKET_OWNER_FULL_CONTROL` or `PUBLIC_READ` as the value.
* For more information, see [Blocking public access to the S3 bucket](https://docs.aws.amazon.com/privateca/latest/userguide/PcaCreateCa.html#s3-bpa) .
*/
@JvmName("cleeemprcfkhnpea")
public suspend fun s3ObjectAcl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3ObjectAcl = mapped
}
internal fun build(): CertificateAuthorityCrlConfigurationArgs =
CertificateAuthorityCrlConfigurationArgs(
crlDistributionPointExtensionConfiguration = crlDistributionPointExtensionConfiguration,
customCname = customCname,
enabled = enabled ?: throw PulumiNullFieldException("enabled"),
expirationInDays = expirationInDays,
s3BucketName = s3BucketName,
s3ObjectAcl = s3ObjectAcl,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy