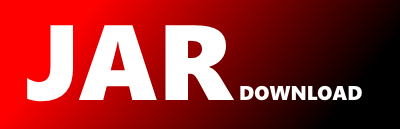
com.pulumi.awsnative.acmpca.kotlin.inputs.CertificateGeneralNameArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.acmpca.kotlin.inputs
import com.pulumi.awsnative.acmpca.inputs.CertificateGeneralNameArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Describes an ASN.1 X.400 ``GeneralName`` as defined in [RFC 5280](https://docs.aws.amazon.com/https://datatracker.ietf.org/doc/html/rfc5280). Only one of the following naming options should be provided. Providing more than one option results in an ``InvalidArgsException`` error.
* @property directoryName Contains information about the certificate subject. The certificate can be one issued by your private certificate authority (CA) or it can be your private CA certificate. The Subject field in the certificate identifies the entity that owns or controls the public key in the certificate. The entity can be a user, computer, device, or service. The Subject must contain an X.500 distinguished name (DN). A DN is a sequence of relative distinguished names (RDNs). The RDNs are separated by commas in the certificate. The DN must be unique for each entity, but your private CA can issue more than one certificate with the same DN to the same entity.
* @property dnsName Represents ``GeneralName`` as a DNS name.
* @property ediPartyName Represents ``GeneralName`` as an ``EdiPartyName`` object.
* @property ipAddress Represents ``GeneralName`` as an IPv4 or IPv6 address.
* @property otherName Represents ``GeneralName`` using an ``OtherName`` object.
* @property registeredId Represents ``GeneralName`` as an object identifier (OID).
* @property rfc822Name Represents ``GeneralName`` as an [RFC 822](https://docs.aws.amazon.com/https://datatracker.ietf.org/doc/html/rfc822) email address.
* @property uniformResourceIdentifier Represents ``GeneralName`` as a URI.
*/
public data class CertificateGeneralNameArgs(
public val directoryName: Output? = null,
public val dnsName: Output? = null,
public val ediPartyName: Output? = null,
public val ipAddress: Output? = null,
public val otherName: Output? = null,
public val registeredId: Output? = null,
public val rfc822Name: Output? = null,
public val uniformResourceIdentifier: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.acmpca.inputs.CertificateGeneralNameArgs =
com.pulumi.awsnative.acmpca.inputs.CertificateGeneralNameArgs.builder()
.directoryName(directoryName?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.dnsName(dnsName?.applyValue({ args0 -> args0 }))
.ediPartyName(ediPartyName?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ipAddress(ipAddress?.applyValue({ args0 -> args0 }))
.otherName(otherName?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.registeredId(registeredId?.applyValue({ args0 -> args0 }))
.rfc822Name(rfc822Name?.applyValue({ args0 -> args0 }))
.uniformResourceIdentifier(uniformResourceIdentifier?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CertificateGeneralNameArgs].
*/
@PulumiTagMarker
public class CertificateGeneralNameArgsBuilder internal constructor() {
private var directoryName: Output? = null
private var dnsName: Output? = null
private var ediPartyName: Output? = null
private var ipAddress: Output? = null
private var otherName: Output? = null
private var registeredId: Output? = null
private var rfc822Name: Output? = null
private var uniformResourceIdentifier: Output? = null
/**
* @param value Contains information about the certificate subject. The certificate can be one issued by your private certificate authority (CA) or it can be your private CA certificate. The Subject field in the certificate identifies the entity that owns or controls the public key in the certificate. The entity can be a user, computer, device, or service. The Subject must contain an X.500 distinguished name (DN). A DN is a sequence of relative distinguished names (RDNs). The RDNs are separated by commas in the certificate. The DN must be unique for each entity, but your private CA can issue more than one certificate with the same DN to the same entity.
*/
@JvmName("lbwphbgibsljaunp")
public suspend fun directoryName(`value`: Output) {
this.directoryName = value
}
/**
* @param value Represents ``GeneralName`` as a DNS name.
*/
@JvmName("bsotmgpshscsofng")
public suspend fun dnsName(`value`: Output) {
this.dnsName = value
}
/**
* @param value Represents ``GeneralName`` as an ``EdiPartyName`` object.
*/
@JvmName("ntlwlqfmaarjxatx")
public suspend fun ediPartyName(`value`: Output) {
this.ediPartyName = value
}
/**
* @param value Represents ``GeneralName`` as an IPv4 or IPv6 address.
*/
@JvmName("xhouabqvwptykxvy")
public suspend fun ipAddress(`value`: Output) {
this.ipAddress = value
}
/**
* @param value Represents ``GeneralName`` using an ``OtherName`` object.
*/
@JvmName("reqnqvgofxwlwymm")
public suspend fun otherName(`value`: Output) {
this.otherName = value
}
/**
* @param value Represents ``GeneralName`` as an object identifier (OID).
*/
@JvmName("qyudvqvftodnrfxs")
public suspend fun registeredId(`value`: Output) {
this.registeredId = value
}
/**
* @param value Represents ``GeneralName`` as an [RFC 822](https://docs.aws.amazon.com/https://datatracker.ietf.org/doc/html/rfc822) email address.
*/
@JvmName("dtucuycusoojlarh")
public suspend fun rfc822Name(`value`: Output) {
this.rfc822Name = value
}
/**
* @param value Represents ``GeneralName`` as a URI.
*/
@JvmName("pajfumlrhxelmpam")
public suspend fun uniformResourceIdentifier(`value`: Output) {
this.uniformResourceIdentifier = value
}
/**
* @param value Contains information about the certificate subject. The certificate can be one issued by your private certificate authority (CA) or it can be your private CA certificate. The Subject field in the certificate identifies the entity that owns or controls the public key in the certificate. The entity can be a user, computer, device, or service. The Subject must contain an X.500 distinguished name (DN). A DN is a sequence of relative distinguished names (RDNs). The RDNs are separated by commas in the certificate. The DN must be unique for each entity, but your private CA can issue more than one certificate with the same DN to the same entity.
*/
@JvmName("tojvsypmmmpvtgem")
public suspend fun directoryName(`value`: CertificateSubjectArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.directoryName = mapped
}
/**
* @param argument Contains information about the certificate subject. The certificate can be one issued by your private certificate authority (CA) or it can be your private CA certificate. The Subject field in the certificate identifies the entity that owns or controls the public key in the certificate. The entity can be a user, computer, device, or service. The Subject must contain an X.500 distinguished name (DN). A DN is a sequence of relative distinguished names (RDNs). The RDNs are separated by commas in the certificate. The DN must be unique for each entity, but your private CA can issue more than one certificate with the same DN to the same entity.
*/
@JvmName("tbuheifmjfixwugm")
public suspend fun directoryName(argument: suspend CertificateSubjectArgsBuilder.() -> Unit) {
val toBeMapped = CertificateSubjectArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.directoryName = mapped
}
/**
* @param value Represents ``GeneralName`` as a DNS name.
*/
@JvmName("allgitcpgeqacweq")
public suspend fun dnsName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dnsName = mapped
}
/**
* @param value Represents ``GeneralName`` as an ``EdiPartyName`` object.
*/
@JvmName("dpgjlpputviqwljw")
public suspend fun ediPartyName(`value`: CertificateEdiPartyNameArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ediPartyName = mapped
}
/**
* @param argument Represents ``GeneralName`` as an ``EdiPartyName`` object.
*/
@JvmName("utxafcrmruvhgwye")
public suspend fun ediPartyName(argument: suspend CertificateEdiPartyNameArgsBuilder.() -> Unit) {
val toBeMapped = CertificateEdiPartyNameArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.ediPartyName = mapped
}
/**
* @param value Represents ``GeneralName`` as an IPv4 or IPv6 address.
*/
@JvmName("fsgmokvqqnrqhckc")
public suspend fun ipAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipAddress = mapped
}
/**
* @param value Represents ``GeneralName`` using an ``OtherName`` object.
*/
@JvmName("rkecbbibbevtrakp")
public suspend fun otherName(`value`: CertificateOtherNameArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.otherName = mapped
}
/**
* @param argument Represents ``GeneralName`` using an ``OtherName`` object.
*/
@JvmName("bhoexvwmeyefbfkg")
public suspend fun otherName(argument: suspend CertificateOtherNameArgsBuilder.() -> Unit) {
val toBeMapped = CertificateOtherNameArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.otherName = mapped
}
/**
* @param value Represents ``GeneralName`` as an object identifier (OID).
*/
@JvmName("ierdejsnbecfwmph")
public suspend fun registeredId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.registeredId = mapped
}
/**
* @param value Represents ``GeneralName`` as an [RFC 822](https://docs.aws.amazon.com/https://datatracker.ietf.org/doc/html/rfc822) email address.
*/
@JvmName("lsnrddnnccofvqhr")
public suspend fun rfc822Name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rfc822Name = mapped
}
/**
* @param value Represents ``GeneralName`` as a URI.
*/
@JvmName("tygmsjxuahbpnnfu")
public suspend fun uniformResourceIdentifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.uniformResourceIdentifier = mapped
}
internal fun build(): CertificateGeneralNameArgs = CertificateGeneralNameArgs(
directoryName = directoryName,
dnsName = dnsName,
ediPartyName = ediPartyName,
ipAddress = ipAddress,
otherName = otherName,
registeredId = registeredId,
rfc822Name = rfc822Name,
uniformResourceIdentifier = uniformResourceIdentifier,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy