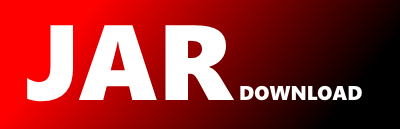
com.pulumi.awsnative.amplifyuibuilder.kotlin.inputs.FormFieldInputConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.amplifyuibuilder.kotlin.inputs
import com.pulumi.awsnative.amplifyuibuilder.inputs.FormFieldInputConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property defaultChecked Specifies whether a field has a default value.
* @property defaultCountryCode The default country code for a phone number.
* @property defaultValue The default value for the field.
* @property descriptiveText The text to display to describe the field.
* @property fileUploaderConfig The configuration for the file uploader field.
* @property isArray Specifies whether to render the field as an array. This property is ignored if the `dataSourceType` for the form is a Data Store.
* @property maxValue The maximum value to display for the field.
* @property minValue The minimum value to display for the field.
* @property name The name of the field.
* @property placeholder The text to display as a placeholder for the field.
* @property readOnly Specifies a read only field.
* @property required Specifies a field that requires input.
* @property step The stepping increment for a numeric value in a field.
* @property type The input type for the field.
* @property value The value for the field.
* @property valueMappings The information to use to customize the input fields with data at runtime.
*/
public data class FormFieldInputConfigArgs(
public val defaultChecked: Output? = null,
public val defaultCountryCode: Output? = null,
public val defaultValue: Output? = null,
public val descriptiveText: Output? = null,
public val fileUploaderConfig: Output? = null,
public val isArray: Output? = null,
public val maxValue: Output? = null,
public val minValue: Output? = null,
public val name: Output? = null,
public val placeholder: Output? = null,
public val readOnly: Output? = null,
public val required: Output? = null,
public val step: Output? = null,
public val type: Output,
public val `value`: Output? = null,
public val valueMappings: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.amplifyuibuilder.inputs.FormFieldInputConfigArgs =
com.pulumi.awsnative.amplifyuibuilder.inputs.FormFieldInputConfigArgs.builder()
.defaultChecked(defaultChecked?.applyValue({ args0 -> args0 }))
.defaultCountryCode(defaultCountryCode?.applyValue({ args0 -> args0 }))
.defaultValue(defaultValue?.applyValue({ args0 -> args0 }))
.descriptiveText(descriptiveText?.applyValue({ args0 -> args0 }))
.fileUploaderConfig(
fileUploaderConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.isArray(isArray?.applyValue({ args0 -> args0 }))
.maxValue(maxValue?.applyValue({ args0 -> args0 }))
.minValue(minValue?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.placeholder(placeholder?.applyValue({ args0 -> args0 }))
.readOnly(readOnly?.applyValue({ args0 -> args0 }))
.required(required?.applyValue({ args0 -> args0 }))
.step(step?.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 }))
.`value`(`value`?.applyValue({ args0 -> args0 }))
.valueMappings(valueMappings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [FormFieldInputConfigArgs].
*/
@PulumiTagMarker
public class FormFieldInputConfigArgsBuilder internal constructor() {
private var defaultChecked: Output? = null
private var defaultCountryCode: Output? = null
private var defaultValue: Output? = null
private var descriptiveText: Output? = null
private var fileUploaderConfig: Output? = null
private var isArray: Output? = null
private var maxValue: Output? = null
private var minValue: Output? = null
private var name: Output? = null
private var placeholder: Output? = null
private var readOnly: Output? = null
private var required: Output? = null
private var step: Output? = null
private var type: Output? = null
private var `value`: Output? = null
private var valueMappings: Output? = null
/**
* @param value Specifies whether a field has a default value.
*/
@JvmName("ajlwowswwykrckmh")
public suspend fun defaultChecked(`value`: Output) {
this.defaultChecked = value
}
/**
* @param value The default country code for a phone number.
*/
@JvmName("ydropjkwkejftsbm")
public suspend fun defaultCountryCode(`value`: Output) {
this.defaultCountryCode = value
}
/**
* @param value The default value for the field.
*/
@JvmName("xoitxucdemybcbvi")
public suspend fun defaultValue(`value`: Output) {
this.defaultValue = value
}
/**
* @param value The text to display to describe the field.
*/
@JvmName("ghgiwkvxkrucwkoo")
public suspend fun descriptiveText(`value`: Output) {
this.descriptiveText = value
}
/**
* @param value The configuration for the file uploader field.
*/
@JvmName("vbsbegjlftlmdipt")
public suspend fun fileUploaderConfig(`value`: Output) {
this.fileUploaderConfig = value
}
/**
* @param value Specifies whether to render the field as an array. This property is ignored if the `dataSourceType` for the form is a Data Store.
*/
@JvmName("pantspkekyfxprfq")
public suspend fun isArray(`value`: Output) {
this.isArray = value
}
/**
* @param value The maximum value to display for the field.
*/
@JvmName("jvykoncrlpddfhdk")
public suspend fun maxValue(`value`: Output) {
this.maxValue = value
}
/**
* @param value The minimum value to display for the field.
*/
@JvmName("xysooimonsltdqeb")
public suspend fun minValue(`value`: Output) {
this.minValue = value
}
/**
* @param value The name of the field.
*/
@JvmName("pghtqdjygllgiqtp")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The text to display as a placeholder for the field.
*/
@JvmName("monvyoxwtqktjvlw")
public suspend fun placeholder(`value`: Output) {
this.placeholder = value
}
/**
* @param value Specifies a read only field.
*/
@JvmName("bdiprtpccxiqpcyu")
public suspend fun readOnly(`value`: Output) {
this.readOnly = value
}
/**
* @param value Specifies a field that requires input.
*/
@JvmName("kcptaexlxbtnanmx")
public suspend fun required(`value`: Output) {
this.required = value
}
/**
* @param value The stepping increment for a numeric value in a field.
*/
@JvmName("mcuygyjtotmfkulx")
public suspend fun step(`value`: Output) {
this.step = value
}
/**
* @param value The input type for the field.
*/
@JvmName("goaiukcrobbuiero")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value The value for the field.
*/
@JvmName("nsatfbjluaxwswsy")
public suspend fun `value`(`value`: Output) {
this.`value` = value
}
/**
* @param value The information to use to customize the input fields with data at runtime.
*/
@JvmName("btmlxgcimqevdfcc")
public suspend fun valueMappings(`value`: Output) {
this.valueMappings = value
}
/**
* @param value Specifies whether a field has a default value.
*/
@JvmName("hsajkotltspyfotv")
public suspend fun defaultChecked(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultChecked = mapped
}
/**
* @param value The default country code for a phone number.
*/
@JvmName("vfgkbcipfhmcehui")
public suspend fun defaultCountryCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultCountryCode = mapped
}
/**
* @param value The default value for the field.
*/
@JvmName("rqydkysonbflwelj")
public suspend fun defaultValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultValue = mapped
}
/**
* @param value The text to display to describe the field.
*/
@JvmName("slvjvwmdxpvgwkij")
public suspend fun descriptiveText(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.descriptiveText = mapped
}
/**
* @param value The configuration for the file uploader field.
*/
@JvmName("nlbdxlibdpwwewpj")
public suspend fun fileUploaderConfig(`value`: FormFileUploaderFieldConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fileUploaderConfig = mapped
}
/**
* @param argument The configuration for the file uploader field.
*/
@JvmName("ojwkmwpsausxavxi")
public suspend fun fileUploaderConfig(argument: suspend FormFileUploaderFieldConfigArgsBuilder.() -> Unit) {
val toBeMapped = FormFileUploaderFieldConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.fileUploaderConfig = mapped
}
/**
* @param value Specifies whether to render the field as an array. This property is ignored if the `dataSourceType` for the form is a Data Store.
*/
@JvmName("udqukdjspnmbnnxn")
public suspend fun isArray(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isArray = mapped
}
/**
* @param value The maximum value to display for the field.
*/
@JvmName("lfxtkrrmrkhhkesx")
public suspend fun maxValue(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxValue = mapped
}
/**
* @param value The minimum value to display for the field.
*/
@JvmName("lnllajqfcpedrlnr")
public suspend fun minValue(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minValue = mapped
}
/**
* @param value The name of the field.
*/
@JvmName("jxllhlbjematsrda")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The text to display as a placeholder for the field.
*/
@JvmName("ygemhsaxjxhggrtm")
public suspend fun placeholder(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.placeholder = mapped
}
/**
* @param value Specifies a read only field.
*/
@JvmName("kuhqvklatxoqmjpm")
public suspend fun readOnly(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.readOnly = mapped
}
/**
* @param value Specifies a field that requires input.
*/
@JvmName("rwnqseifslcjiytm")
public suspend fun required(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.required = mapped
}
/**
* @param value The stepping increment for a numeric value in a field.
*/
@JvmName("wxlhivgdletpoepm")
public suspend fun step(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.step = mapped
}
/**
* @param value The input type for the field.
*/
@JvmName("ykamalrtnxqqwhws")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
/**
* @param value The value for the field.
*/
@JvmName("ocelvbtipetslngl")
public suspend fun `value`(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`value` = mapped
}
/**
* @param value The information to use to customize the input fields with data at runtime.
*/
@JvmName("dpkxhilxbljnlcmn")
public suspend fun valueMappings(`value`: FormValueMappingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.valueMappings = mapped
}
/**
* @param argument The information to use to customize the input fields with data at runtime.
*/
@JvmName("deioyjfvkwtsktkg")
public suspend fun valueMappings(argument: suspend FormValueMappingsArgsBuilder.() -> Unit) {
val toBeMapped = FormValueMappingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.valueMappings = mapped
}
internal fun build(): FormFieldInputConfigArgs = FormFieldInputConfigArgs(
defaultChecked = defaultChecked,
defaultCountryCode = defaultCountryCode,
defaultValue = defaultValue,
descriptiveText = descriptiveText,
fileUploaderConfig = fileUploaderConfig,
isArray = isArray,
maxValue = maxValue,
minValue = minValue,
name = name,
placeholder = placeholder,
readOnly = readOnly,
required = required,
step = step,
type = type ?: throw PulumiNullFieldException("type"),
`value` = `value`,
valueMappings = valueMappings,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy