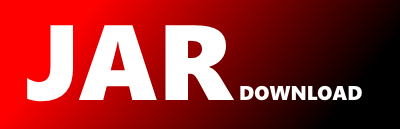
com.pulumi.awsnative.apigateway.kotlin.ModelArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.apigateway.kotlin
import com.pulumi.awsnative.apigateway.ModelArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* /* /*
* The ``AWS::ApiGateway::Model`` resource defines the structure of a request or response payload for an API method.
* @property contentType The content-type for the model.
* @property description The description of the model.
* @property name A name for the model. If you don't specify a name, CFN generates a unique physical ID and uses that ID for the model name. For more information, see [Name Type](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-name.html).
* If you specify a name, you cannot perform updates that require replacement of this resource. You can perform updates that require no or some interruption. If you must replace the resource, specify a new name.
* @property restApiId The string identifier of the associated RestApi.
* @property schema The schema for the model. For ``application/json`` models, this should be JSON schema draft 4 model. Do not include "\*/" characters in the description of any properties because such "\*/" characters may be interpreted as the closing marker for comments in some languages, such as Java or JavaScript, causing the installation of your API's SDK generated by API Gateway to fail.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGateway::Model` for more information about the expected schema for this property.
*/
public data class ModelArgs(
public val contentType: Output? = null,
public val description: Output? = null,
public val name: Output? = null,
public val restApiId: Output? = null,
public val schema: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.apigateway.ModelArgs =
com.pulumi.awsnative.apigateway.ModelArgs.builder()
.contentType(contentType?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.restApiId(restApiId?.applyValue({ args0 -> args0 }))
.schema(schema?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ModelArgs].
*/
@PulumiTagMarker
public class ModelArgsBuilder internal constructor() {
private var contentType: Output? = null
private var description: Output? = null
private var name: Output? = null
private var restApiId: Output? = null
private var schema: Output? = null
/**
* @param value The content-type for the model.
*/
@JvmName("qxmisfliuriiinkk")
public suspend fun contentType(`value`: Output) {
this.contentType = value
}
/**
* @param value The description of the model.
*/
@JvmName("ivddrxjdrflemufr")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value A name for the model. If you don't specify a name, CFN generates a unique physical ID and uses that ID for the model name. For more information, see [Name Type](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-name.html).
* If you specify a name, you cannot perform updates that require replacement of this resource. You can perform updates that require no or some interruption. If you must replace the resource, specify a new name.
*/
@JvmName("ausvpytnvjbrtdkx")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The string identifier of the associated RestApi.
*/
@JvmName("dtbdvcdfsedgxjvs")
public suspend fun restApiId(`value`: Output) {
this.restApiId = value
}
/**
* /* /*
* @param value The schema for the model. For ``application/json`` models, this should be JSON schema draft 4 model. Do not include "\*/" characters in the description of any properties because such "\*/" characters may be interpreted as the closing marker for comments in some languages, such as Java or JavaScript, causing the installation of your API's SDK generated by API Gateway to fail.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGateway::Model` for more information about the expected schema for this property.
*/
@JvmName("sxoxqmndxunminhm")
public suspend fun schema(`value`: Output) {
this.schema = value
}
/**
* @param value The content-type for the model.
*/
@JvmName("hjekxnoqnydohnrg")
public suspend fun contentType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.contentType = mapped
}
/**
* @param value The description of the model.
*/
@JvmName("radjfjegqbukkbgw")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value A name for the model. If you don't specify a name, CFN generates a unique physical ID and uses that ID for the model name. For more information, see [Name Type](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-name.html).
* If you specify a name, you cannot perform updates that require replacement of this resource. You can perform updates that require no or some interruption. If you must replace the resource, specify a new name.
*/
@JvmName("vxqekihaadlhgnux")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The string identifier of the associated RestApi.
*/
@JvmName("pbhxdvcqvohfcwaj")
public suspend fun restApiId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.restApiId = mapped
}
/**
* /* /*
* @param value The schema for the model. For ``application/json`` models, this should be JSON schema draft 4 model. Do not include "\*/" characters in the description of any properties because such "\*/" characters may be interpreted as the closing marker for comments in some languages, such as Java or JavaScript, causing the installation of your API's SDK generated by API Gateway to fail.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGateway::Model` for more information about the expected schema for this property.
*/
@JvmName("cmsihpsffjicuhfl")
public suspend fun schema(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.schema = mapped
}
internal fun build(): ModelArgs = ModelArgs(
contentType = contentType,
description = description,
name = name,
restApiId = restApiId,
schema = schema,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy