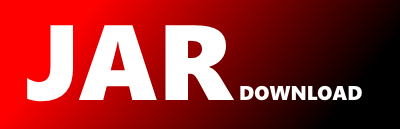
com.pulumi.awsnative.apigateway.kotlin.inputs.DeploymentCanarySettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.apigateway.kotlin.inputs
import com.pulumi.awsnative.apigateway.inputs.DeploymentCanarySettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Double
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* The ``DeploymentCanarySettings`` property type specifies settings for the canary deployment.
* @property percentTraffic The percentage (0.0-100.0) of traffic routed to the canary deployment.
* @property stageVariableOverrides A stage variable overrides used for the canary release deployment. They can override existing stage variables or add new stage variables for the canary release deployment. These stage variables are represented as a string-to-string map between stage variable names and their values.
* @property useStageCache A Boolean flag to indicate whether the canary release deployment uses the stage cache or not.
*/
public data class DeploymentCanarySettingsArgs(
public val percentTraffic: Output? = null,
public val stageVariableOverrides: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy