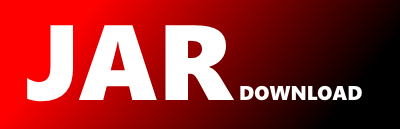
com.pulumi.awsnative.apigatewayv2.kotlin.IntegrationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.apigatewayv2.kotlin
import com.pulumi.awsnative.apigatewayv2.IntegrationArgs.builder
import com.pulumi.awsnative.apigatewayv2.kotlin.inputs.IntegrationTlsConfigArgs
import com.pulumi.awsnative.apigatewayv2.kotlin.inputs.IntegrationTlsConfigArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::ApiGatewayV2::Integration
* @property apiId The API identifier.
* @property connectionId The ID of the VPC link for a private integration. Supported only for HTTP APIs.
* @property connectionType The type of the network connection to the integration endpoint. Specify `INTERNET` for connections through the public routable internet or `VPC_LINK` for private connections between API Gateway and resources in a VPC. The default value is `INTERNET` .
* @property contentHandlingStrategy Supported only for WebSocket APIs. Specifies how to handle response payload content type conversions. Supported values are `CONVERT_TO_BINARY` and `CONVERT_TO_TEXT` , with the following behaviors:
* `CONVERT_TO_BINARY` : Converts a response payload from a Base64-encoded string to the corresponding binary blob.
* `CONVERT_TO_TEXT` : Converts a response payload from a binary blob to a Base64-encoded string.
* If this property is not defined, the response payload will be passed through from the integration response to the route response or method response without modification.
* @property credentialsArn Specifies the credentials required for the integration, if any. For AWS integrations, three options are available. To specify an IAM Role for API Gateway to assume, use the role's Amazon Resource Name (ARN). To require that the caller's identity be passed through from the request, specify the string `arn:aws:iam::*:user/*` . To use resource-based permissions on supported AWS services, don't specify this parameter.
* @property description The description of the integration.
* @property integrationMethod Specifies the integration's HTTP method type. For WebSocket APIs, if you use a Lambda integration, you must set the integration method to `POST` .
* @property integrationSubtype Supported only for HTTP API `AWS_PROXY` integrations. Specifies the AWS service action to invoke. To learn more, see [Integration subtype reference](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-aws-services-reference.html) .
* @property integrationType The integration type of an integration. One of the following:
* `AWS` : for integrating the route or method request with an AWS service action, including the Lambda function-invoking action. With the Lambda function-invoking action, this is referred to as the Lambda custom integration. With any other AWS service action, this is known as AWS integration. Supported only for WebSocket APIs.
* `AWS_PROXY` : for integrating the route or method request with a Lambda function or other AWS service action. This integration is also referred to as a Lambda proxy integration.
* `HTTP` : for integrating the route or method request with an HTTP endpoint. This integration is also referred to as the HTTP custom integration. Supported only for WebSocket APIs.
* `HTTP_PROXY` : for integrating the route or method request with an HTTP endpoint, with the client request passed through as-is. This is also referred to as HTTP proxy integration. For HTTP API private integrations, use an `HTTP_PROXY` integration.
* `MOCK` : for integrating the route or method request with API Gateway as a "loopback" endpoint without invoking any backend. Supported only for WebSocket APIs.
* @property integrationUri For a Lambda integration, specify the URI of a Lambda function.
* For an HTTP integration, specify a fully-qualified URL.
* For an HTTP API private integration, specify the ARN of an Application Load Balancer listener, Network Load Balancer listener, or AWS Cloud Map service. If you specify the ARN of an AWS Cloud Map service, API Gateway uses `DiscoverInstances` to identify resources. You can use query parameters to target specific resources. To learn more, see [DiscoverInstances](https://docs.aws.amazon.com/cloud-map/latest/api/API_DiscoverInstances.html) . For private integrations, all resources must be owned by the same AWS account .
* @property passthroughBehavior Specifies the pass-through behavior for incoming requests based on the `Content-Type` header in the request, and the available mapping templates specified as the `requestTemplates` property on the `Integration` resource. There are three valid values: `WHEN_NO_MATCH` , `WHEN_NO_TEMPLATES` , and `NEVER` . Supported only for WebSocket APIs.
* `WHEN_NO_MATCH` passes the request body for unmapped content types through to the integration backend without transformation.
* `NEVER` rejects unmapped content types with an `HTTP 415 Unsupported Media Type` response.
* `WHEN_NO_TEMPLATES` allows pass-through when the integration has no content types mapped to templates. However, if there is at least one content type defined, unmapped content types will be rejected with the same `HTTP 415 Unsupported Media Type` response.
* @property payloadFormatVersion Specifies the format of the payload sent to an integration. Required for HTTP APIs. For HTTP APIs, supported values for Lambda proxy integrations are `1.0` and `2.0` . For all other integrations, `1.0` is the only supported value. To learn more, see [Working with AWS Lambda proxy integrations for HTTP APIs](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-lambda.html) .
* @property requestParameters For WebSocket APIs, a key-value map specifying request parameters that are passed from the method request to the backend. The key is an integration request parameter name and the associated value is a method request parameter value or static value that must be enclosed within single quotes and pre-encoded as required by the backend. The method request parameter value must match the pattern of `method.request. {location} . {name}` , where `{location}` is `querystring` , `path` , or `header` ; and `{name}` must be a valid and unique method request parameter name.
* For HTTP API integrations with a specified `integrationSubtype` , request parameters are a key-value map specifying parameters that are passed to `AWS_PROXY` integrations. You can provide static values, or map request data, stage variables, or context variables that are evaluated at runtime. To learn more, see [Working with AWS service integrations for HTTP APIs](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-aws-services.html) .
* For HTTP API integrations without a specified `integrationSubtype` request parameters are a key-value map specifying how to transform HTTP requests before sending them to the backend. The key should follow the pattern :. where action can be `append` , `overwrite` or `remove` . For values, you can provide static values, or map request data, stage variables, or context variables that are evaluated at runtime. To learn more, see [Transforming API requests and responses](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-parameter-mapping.html) .
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGatewayV2::Integration` for more information about the expected schema for this property.
* @property requestTemplates Represents a map of Velocity templates that are applied on the request payload based on the value of the Content-Type header sent by the client. The content type value is the key in this map, and the template (as a String) is the value. Supported only for WebSocket APIs.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGatewayV2::Integration` for more information about the expected schema for this property.
* @property responseParameters Supported only for HTTP APIs. You use response parameters to transform the HTTP response from a backend integration before returning the response to clients. Specify a key-value map from a selection key to response parameters. The selection key must be a valid HTTP status code within the range of 200-599. The value is of type [`ResponseParameterList`](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-apigatewayv2-integration-responseparameterlist.html) . To learn more, see [Transforming API requests and responses](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-parameter-mapping.html) .
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGatewayV2::Integration` for more information about the expected schema for this property.
* @property templateSelectionExpression The template selection expression for the integration. Supported only for WebSocket APIs.
* @property timeoutInMillis Custom timeout between 50 and 29,000 milliseconds for WebSocket APIs and between 50 and 30,000 milliseconds for HTTP APIs. The default timeout is 29 seconds for WebSocket APIs and 30 seconds for HTTP APIs.
* @property tlsConfig The TLS configuration for a private integration. If you specify a TLS configuration, private integration traffic uses the HTTPS protocol. Supported only for HTTP APIs.
* */
*/
public data class IntegrationArgs(
public val apiId: Output? = null,
public val connectionId: Output? = null,
public val connectionType: Output? = null,
public val contentHandlingStrategy: Output? = null,
public val credentialsArn: Output? = null,
public val description: Output? = null,
public val integrationMethod: Output? = null,
public val integrationSubtype: Output? = null,
public val integrationType: Output? = null,
public val integrationUri: Output? = null,
public val passthroughBehavior: Output? = null,
public val payloadFormatVersion: Output? = null,
public val requestParameters: Output? = null,
public val requestTemplates: Output? = null,
public val responseParameters: Output? = null,
public val templateSelectionExpression: Output? = null,
public val timeoutInMillis: Output? = null,
public val tlsConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.apigatewayv2.IntegrationArgs =
com.pulumi.awsnative.apigatewayv2.IntegrationArgs.builder()
.apiId(apiId?.applyValue({ args0 -> args0 }))
.connectionId(connectionId?.applyValue({ args0 -> args0 }))
.connectionType(connectionType?.applyValue({ args0 -> args0 }))
.contentHandlingStrategy(contentHandlingStrategy?.applyValue({ args0 -> args0 }))
.credentialsArn(credentialsArn?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.integrationMethod(integrationMethod?.applyValue({ args0 -> args0 }))
.integrationSubtype(integrationSubtype?.applyValue({ args0 -> args0 }))
.integrationType(integrationType?.applyValue({ args0 -> args0 }))
.integrationUri(integrationUri?.applyValue({ args0 -> args0 }))
.passthroughBehavior(passthroughBehavior?.applyValue({ args0 -> args0 }))
.payloadFormatVersion(payloadFormatVersion?.applyValue({ args0 -> args0 }))
.requestParameters(requestParameters?.applyValue({ args0 -> args0 }))
.requestTemplates(requestTemplates?.applyValue({ args0 -> args0 }))
.responseParameters(responseParameters?.applyValue({ args0 -> args0 }))
.templateSelectionExpression(templateSelectionExpression?.applyValue({ args0 -> args0 }))
.timeoutInMillis(timeoutInMillis?.applyValue({ args0 -> args0 }))
.tlsConfig(tlsConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [IntegrationArgs].
*/
@PulumiTagMarker
public class IntegrationArgsBuilder internal constructor() {
private var apiId: Output? = null
private var connectionId: Output? = null
private var connectionType: Output? = null
private var contentHandlingStrategy: Output? = null
private var credentialsArn: Output? = null
private var description: Output? = null
private var integrationMethod: Output? = null
private var integrationSubtype: Output? = null
private var integrationType: Output? = null
private var integrationUri: Output? = null
private var passthroughBehavior: Output? = null
private var payloadFormatVersion: Output? = null
private var requestParameters: Output? = null
private var requestTemplates: Output? = null
private var responseParameters: Output? = null
private var templateSelectionExpression: Output? = null
private var timeoutInMillis: Output? = null
private var tlsConfig: Output? = null
/**
* @param value The API identifier.
*/
@JvmName("fbulfiqwkdeujhqw")
public suspend fun apiId(`value`: Output) {
this.apiId = value
}
/**
* @param value The ID of the VPC link for a private integration. Supported only for HTTP APIs.
*/
@JvmName("bxexlfhpwauylboa")
public suspend fun connectionId(`value`: Output) {
this.connectionId = value
}
/**
* @param value The type of the network connection to the integration endpoint. Specify `INTERNET` for connections through the public routable internet or `VPC_LINK` for private connections between API Gateway and resources in a VPC. The default value is `INTERNET` .
*/
@JvmName("mlrqgrdnaaeijofb")
public suspend fun connectionType(`value`: Output) {
this.connectionType = value
}
/**
* @param value Supported only for WebSocket APIs. Specifies how to handle response payload content type conversions. Supported values are `CONVERT_TO_BINARY` and `CONVERT_TO_TEXT` , with the following behaviors:
* `CONVERT_TO_BINARY` : Converts a response payload from a Base64-encoded string to the corresponding binary blob.
* `CONVERT_TO_TEXT` : Converts a response payload from a binary blob to a Base64-encoded string.
* If this property is not defined, the response payload will be passed through from the integration response to the route response or method response without modification.
*/
@JvmName("sianxvbhvwoamclk")
public suspend fun contentHandlingStrategy(`value`: Output) {
this.contentHandlingStrategy = value
}
/**
* @param value Specifies the credentials required for the integration, if any. For AWS integrations, three options are available. To specify an IAM Role for API Gateway to assume, use the role's Amazon Resource Name (ARN). To require that the caller's identity be passed through from the request, specify the string `arn:aws:iam::*:user/*` . To use resource-based permissions on supported AWS services, don't specify this parameter.
* */
*/
@JvmName("cnjncsmkqihwygmj")
public suspend fun credentialsArn(`value`: Output) {
this.credentialsArn = value
}
/**
* @param value The description of the integration.
*/
@JvmName("ofobvqamfhsokqyg")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Specifies the integration's HTTP method type. For WebSocket APIs, if you use a Lambda integration, you must set the integration method to `POST` .
*/
@JvmName("kmgholclwduspnth")
public suspend fun integrationMethod(`value`: Output) {
this.integrationMethod = value
}
/**
* @param value Supported only for HTTP API `AWS_PROXY` integrations. Specifies the AWS service action to invoke. To learn more, see [Integration subtype reference](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-aws-services-reference.html) .
*/
@JvmName("eqinxhphsajbrjhu")
public suspend fun integrationSubtype(`value`: Output) {
this.integrationSubtype = value
}
/**
* @param value The integration type of an integration. One of the following:
* `AWS` : for integrating the route or method request with an AWS service action, including the Lambda function-invoking action. With the Lambda function-invoking action, this is referred to as the Lambda custom integration. With any other AWS service action, this is known as AWS integration. Supported only for WebSocket APIs.
* `AWS_PROXY` : for integrating the route or method request with a Lambda function or other AWS service action. This integration is also referred to as a Lambda proxy integration.
* `HTTP` : for integrating the route or method request with an HTTP endpoint. This integration is also referred to as the HTTP custom integration. Supported only for WebSocket APIs.
* `HTTP_PROXY` : for integrating the route or method request with an HTTP endpoint, with the client request passed through as-is. This is also referred to as HTTP proxy integration. For HTTP API private integrations, use an `HTTP_PROXY` integration.
* `MOCK` : for integrating the route or method request with API Gateway as a "loopback" endpoint without invoking any backend. Supported only for WebSocket APIs.
*/
@JvmName("leprexyhxejhbjus")
public suspend fun integrationType(`value`: Output) {
this.integrationType = value
}
/**
* @param value For a Lambda integration, specify the URI of a Lambda function.
* For an HTTP integration, specify a fully-qualified URL.
* For an HTTP API private integration, specify the ARN of an Application Load Balancer listener, Network Load Balancer listener, or AWS Cloud Map service. If you specify the ARN of an AWS Cloud Map service, API Gateway uses `DiscoverInstances` to identify resources. You can use query parameters to target specific resources. To learn more, see [DiscoverInstances](https://docs.aws.amazon.com/cloud-map/latest/api/API_DiscoverInstances.html) . For private integrations, all resources must be owned by the same AWS account .
*/
@JvmName("hqpkceaeemepsjsa")
public suspend fun integrationUri(`value`: Output) {
this.integrationUri = value
}
/**
* @param value Specifies the pass-through behavior for incoming requests based on the `Content-Type` header in the request, and the available mapping templates specified as the `requestTemplates` property on the `Integration` resource. There are three valid values: `WHEN_NO_MATCH` , `WHEN_NO_TEMPLATES` , and `NEVER` . Supported only for WebSocket APIs.
* `WHEN_NO_MATCH` passes the request body for unmapped content types through to the integration backend without transformation.
* `NEVER` rejects unmapped content types with an `HTTP 415 Unsupported Media Type` response.
* `WHEN_NO_TEMPLATES` allows pass-through when the integration has no content types mapped to templates. However, if there is at least one content type defined, unmapped content types will be rejected with the same `HTTP 415 Unsupported Media Type` response.
*/
@JvmName("qighafapvuvjunoo")
public suspend fun passthroughBehavior(`value`: Output) {
this.passthroughBehavior = value
}
/**
* @param value Specifies the format of the payload sent to an integration. Required for HTTP APIs. For HTTP APIs, supported values for Lambda proxy integrations are `1.0` and `2.0` . For all other integrations, `1.0` is the only supported value. To learn more, see [Working with AWS Lambda proxy integrations for HTTP APIs](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-lambda.html) .
*/
@JvmName("aqiscibderfmufky")
public suspend fun payloadFormatVersion(`value`: Output) {
this.payloadFormatVersion = value
}
/**
* @param value For WebSocket APIs, a key-value map specifying request parameters that are passed from the method request to the backend. The key is an integration request parameter name and the associated value is a method request parameter value or static value that must be enclosed within single quotes and pre-encoded as required by the backend. The method request parameter value must match the pattern of `method.request. {location} . {name}` , where `{location}` is `querystring` , `path` , or `header` ; and `{name}` must be a valid and unique method request parameter name.
* For HTTP API integrations with a specified `integrationSubtype` , request parameters are a key-value map specifying parameters that are passed to `AWS_PROXY` integrations. You can provide static values, or map request data, stage variables, or context variables that are evaluated at runtime. To learn more, see [Working with AWS service integrations for HTTP APIs](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-aws-services.html) .
* For HTTP API integrations without a specified `integrationSubtype` request parameters are a key-value map specifying how to transform HTTP requests before sending them to the backend. The key should follow the pattern :. where action can be `append` , `overwrite` or `remove` . For values, you can provide static values, or map request data, stage variables, or context variables that are evaluated at runtime. To learn more, see [Transforming API requests and responses](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-parameter-mapping.html) .
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGatewayV2::Integration` for more information about the expected schema for this property.
*/
@JvmName("uwtrslxebtpgibuw")
public suspend fun requestParameters(`value`: Output) {
this.requestParameters = value
}
/**
* @param value Represents a map of Velocity templates that are applied on the request payload based on the value of the Content-Type header sent by the client. The content type value is the key in this map, and the template (as a String) is the value. Supported only for WebSocket APIs.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGatewayV2::Integration` for more information about the expected schema for this property.
*/
@JvmName("xbnuwtnwwthoelpa")
public suspend fun requestTemplates(`value`: Output) {
this.requestTemplates = value
}
/**
* @param value Supported only for HTTP APIs. You use response parameters to transform the HTTP response from a backend integration before returning the response to clients. Specify a key-value map from a selection key to response parameters. The selection key must be a valid HTTP status code within the range of 200-599. The value is of type [`ResponseParameterList`](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-apigatewayv2-integration-responseparameterlist.html) . To learn more, see [Transforming API requests and responses](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-parameter-mapping.html) .
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGatewayV2::Integration` for more information about the expected schema for this property.
*/
@JvmName("pjkyvxqaxyeiktvl")
public suspend fun responseParameters(`value`: Output) {
this.responseParameters = value
}
/**
* @param value The template selection expression for the integration. Supported only for WebSocket APIs.
*/
@JvmName("bkwatvqwnisnkmnh")
public suspend fun templateSelectionExpression(`value`: Output) {
this.templateSelectionExpression = value
}
/**
* @param value Custom timeout between 50 and 29,000 milliseconds for WebSocket APIs and between 50 and 30,000 milliseconds for HTTP APIs. The default timeout is 29 seconds for WebSocket APIs and 30 seconds for HTTP APIs.
*/
@JvmName("qhifwcqdjkgkwtkx")
public suspend fun timeoutInMillis(`value`: Output) {
this.timeoutInMillis = value
}
/**
* @param value The TLS configuration for a private integration. If you specify a TLS configuration, private integration traffic uses the HTTPS protocol. Supported only for HTTP APIs.
*/
@JvmName("pnidelkfxtrvlith")
public suspend fun tlsConfig(`value`: Output) {
this.tlsConfig = value
}
/**
* @param value The API identifier.
*/
@JvmName("voofhcqwgcghuiao")
public suspend fun apiId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiId = mapped
}
/**
* @param value The ID of the VPC link for a private integration. Supported only for HTTP APIs.
*/
@JvmName("calcswplkkmrcbbx")
public suspend fun connectionId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectionId = mapped
}
/**
* @param value The type of the network connection to the integration endpoint. Specify `INTERNET` for connections through the public routable internet or `VPC_LINK` for private connections between API Gateway and resources in a VPC. The default value is `INTERNET` .
*/
@JvmName("mmufqrfywomlorie")
public suspend fun connectionType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectionType = mapped
}
/**
* @param value Supported only for WebSocket APIs. Specifies how to handle response payload content type conversions. Supported values are `CONVERT_TO_BINARY` and `CONVERT_TO_TEXT` , with the following behaviors:
* `CONVERT_TO_BINARY` : Converts a response payload from a Base64-encoded string to the corresponding binary blob.
* `CONVERT_TO_TEXT` : Converts a response payload from a binary blob to a Base64-encoded string.
* If this property is not defined, the response payload will be passed through from the integration response to the route response or method response without modification.
*/
@JvmName("paxcwobhqdrtcuqj")
public suspend fun contentHandlingStrategy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.contentHandlingStrategy = mapped
}
/**
* @param value Specifies the credentials required for the integration, if any. For AWS integrations, three options are available. To specify an IAM Role for API Gateway to assume, use the role's Amazon Resource Name (ARN). To require that the caller's identity be passed through from the request, specify the string `arn:aws:iam::*:user/*` . To use resource-based permissions on supported AWS services, don't specify this parameter.
* */
*/
@JvmName("hrfmjayeeaqfbemx")
public suspend fun credentialsArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.credentialsArn = mapped
}
/**
* @param value The description of the integration.
*/
@JvmName("vrakgkeyvbnwvmvt")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Specifies the integration's HTTP method type. For WebSocket APIs, if you use a Lambda integration, you must set the integration method to `POST` .
*/
@JvmName("tjhuwynpomskqllx")
public suspend fun integrationMethod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.integrationMethod = mapped
}
/**
* @param value Supported only for HTTP API `AWS_PROXY` integrations. Specifies the AWS service action to invoke. To learn more, see [Integration subtype reference](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-aws-services-reference.html) .
*/
@JvmName("nopccrppcdycxmpd")
public suspend fun integrationSubtype(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.integrationSubtype = mapped
}
/**
* @param value The integration type of an integration. One of the following:
* `AWS` : for integrating the route or method request with an AWS service action, including the Lambda function-invoking action. With the Lambda function-invoking action, this is referred to as the Lambda custom integration. With any other AWS service action, this is known as AWS integration. Supported only for WebSocket APIs.
* `AWS_PROXY` : for integrating the route or method request with a Lambda function or other AWS service action. This integration is also referred to as a Lambda proxy integration.
* `HTTP` : for integrating the route or method request with an HTTP endpoint. This integration is also referred to as the HTTP custom integration. Supported only for WebSocket APIs.
* `HTTP_PROXY` : for integrating the route or method request with an HTTP endpoint, with the client request passed through as-is. This is also referred to as HTTP proxy integration. For HTTP API private integrations, use an `HTTP_PROXY` integration.
* `MOCK` : for integrating the route or method request with API Gateway as a "loopback" endpoint without invoking any backend. Supported only for WebSocket APIs.
*/
@JvmName("luyslssflxbasicg")
public suspend fun integrationType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.integrationType = mapped
}
/**
* @param value For a Lambda integration, specify the URI of a Lambda function.
* For an HTTP integration, specify a fully-qualified URL.
* For an HTTP API private integration, specify the ARN of an Application Load Balancer listener, Network Load Balancer listener, or AWS Cloud Map service. If you specify the ARN of an AWS Cloud Map service, API Gateway uses `DiscoverInstances` to identify resources. You can use query parameters to target specific resources. To learn more, see [DiscoverInstances](https://docs.aws.amazon.com/cloud-map/latest/api/API_DiscoverInstances.html) . For private integrations, all resources must be owned by the same AWS account .
*/
@JvmName("dqniwnxfacueqbys")
public suspend fun integrationUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.integrationUri = mapped
}
/**
* @param value Specifies the pass-through behavior for incoming requests based on the `Content-Type` header in the request, and the available mapping templates specified as the `requestTemplates` property on the `Integration` resource. There are three valid values: `WHEN_NO_MATCH` , `WHEN_NO_TEMPLATES` , and `NEVER` . Supported only for WebSocket APIs.
* `WHEN_NO_MATCH` passes the request body for unmapped content types through to the integration backend without transformation.
* `NEVER` rejects unmapped content types with an `HTTP 415 Unsupported Media Type` response.
* `WHEN_NO_TEMPLATES` allows pass-through when the integration has no content types mapped to templates. However, if there is at least one content type defined, unmapped content types will be rejected with the same `HTTP 415 Unsupported Media Type` response.
*/
@JvmName("iesxxdqcbxurfvaf")
public suspend fun passthroughBehavior(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.passthroughBehavior = mapped
}
/**
* @param value Specifies the format of the payload sent to an integration. Required for HTTP APIs. For HTTP APIs, supported values for Lambda proxy integrations are `1.0` and `2.0` . For all other integrations, `1.0` is the only supported value. To learn more, see [Working with AWS Lambda proxy integrations for HTTP APIs](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-lambda.html) .
*/
@JvmName("arshkoiundrxnyxp")
public suspend fun payloadFormatVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.payloadFormatVersion = mapped
}
/**
* @param value For WebSocket APIs, a key-value map specifying request parameters that are passed from the method request to the backend. The key is an integration request parameter name and the associated value is a method request parameter value or static value that must be enclosed within single quotes and pre-encoded as required by the backend. The method request parameter value must match the pattern of `method.request. {location} . {name}` , where `{location}` is `querystring` , `path` , or `header` ; and `{name}` must be a valid and unique method request parameter name.
* For HTTP API integrations with a specified `integrationSubtype` , request parameters are a key-value map specifying parameters that are passed to `AWS_PROXY` integrations. You can provide static values, or map request data, stage variables, or context variables that are evaluated at runtime. To learn more, see [Working with AWS service integrations for HTTP APIs](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-aws-services.html) .
* For HTTP API integrations without a specified `integrationSubtype` request parameters are a key-value map specifying how to transform HTTP requests before sending them to the backend. The key should follow the pattern :. where action can be `append` , `overwrite` or `remove` . For values, you can provide static values, or map request data, stage variables, or context variables that are evaluated at runtime. To learn more, see [Transforming API requests and responses](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-parameter-mapping.html) .
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGatewayV2::Integration` for more information about the expected schema for this property.
*/
@JvmName("sepgebvmaaictrog")
public suspend fun requestParameters(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requestParameters = mapped
}
/**
* @param value Represents a map of Velocity templates that are applied on the request payload based on the value of the Content-Type header sent by the client. The content type value is the key in this map, and the template (as a String) is the value. Supported only for WebSocket APIs.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGatewayV2::Integration` for more information about the expected schema for this property.
*/
@JvmName("lrxjrjfwqyaluoqn")
public suspend fun requestTemplates(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requestTemplates = mapped
}
/**
* @param value Supported only for HTTP APIs. You use response parameters to transform the HTTP response from a backend integration before returning the response to clients. Specify a key-value map from a selection key to response parameters. The selection key must be a valid HTTP status code within the range of 200-599. The value is of type [`ResponseParameterList`](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-apigatewayv2-integration-responseparameterlist.html) . To learn more, see [Transforming API requests and responses](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-parameter-mapping.html) .
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGatewayV2::Integration` for more information about the expected schema for this property.
*/
@JvmName("fdinpgsitgmimmxx")
public suspend fun responseParameters(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.responseParameters = mapped
}
/**
* @param value The template selection expression for the integration. Supported only for WebSocket APIs.
*/
@JvmName("ruikbaxymoarargr")
public suspend fun templateSelectionExpression(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.templateSelectionExpression = mapped
}
/**
* @param value Custom timeout between 50 and 29,000 milliseconds for WebSocket APIs and between 50 and 30,000 milliseconds for HTTP APIs. The default timeout is 29 seconds for WebSocket APIs and 30 seconds for HTTP APIs.
*/
@JvmName("byjbtitdudhdcvhx")
public suspend fun timeoutInMillis(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutInMillis = mapped
}
/**
* @param value The TLS configuration for a private integration. If you specify a TLS configuration, private integration traffic uses the HTTPS protocol. Supported only for HTTP APIs.
*/
@JvmName("draavpgtnnrljtdf")
public suspend fun tlsConfig(`value`: IntegrationTlsConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsConfig = mapped
}
/**
* @param argument The TLS configuration for a private integration. If you specify a TLS configuration, private integration traffic uses the HTTPS protocol. Supported only for HTTP APIs.
*/
@JvmName("bmjryfchsacucgnu")
public suspend fun tlsConfig(argument: suspend IntegrationTlsConfigArgsBuilder.() -> Unit) {
val toBeMapped = IntegrationTlsConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.tlsConfig = mapped
}
internal fun build(): IntegrationArgs = IntegrationArgs(
apiId = apiId,
connectionId = connectionId,
connectionType = connectionType,
contentHandlingStrategy = contentHandlingStrategy,
credentialsArn = credentialsArn,
description = description,
integrationMethod = integrationMethod,
integrationSubtype = integrationSubtype,
integrationType = integrationType,
integrationUri = integrationUri,
passthroughBehavior = passthroughBehavior,
payloadFormatVersion = payloadFormatVersion,
requestParameters = requestParameters,
requestTemplates = requestTemplates,
responseParameters = responseParameters,
templateSelectionExpression = templateSelectionExpression,
timeoutInMillis = timeoutInMillis,
tlsConfig = tlsConfig,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy