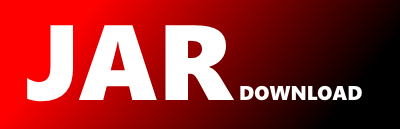
com.pulumi.awsnative.appconfig.kotlin.HostedConfigurationVersionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.appconfig.kotlin
import com.pulumi.awsnative.appconfig.HostedConfigurationVersionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::AppConfig::HostedConfigurationVersion
* @property applicationId The application ID.
* @property configurationProfileId The configuration profile ID.
* @property content The content of the configuration or the configuration data.
* @property contentType A standard MIME type describing the format of the configuration content.
* @property description A description of the hosted configuration version.
* @property latestVersionNumber An optional locking token used to prevent race conditions from overwriting configuration updates when creating a new version. To ensure your data is not overwritten when creating multiple hosted configuration versions in rapid succession, specify the version number of the latest hosted configuration version.
* @property versionLabel A user-defined label for an AWS AppConfig hosted configuration version.
*/
public data class HostedConfigurationVersionArgs(
public val applicationId: Output? = null,
public val configurationProfileId: Output? = null,
public val content: Output? = null,
public val contentType: Output? = null,
public val description: Output? = null,
public val latestVersionNumber: Output? = null,
public val versionLabel: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.appconfig.HostedConfigurationVersionArgs =
com.pulumi.awsnative.appconfig.HostedConfigurationVersionArgs.builder()
.applicationId(applicationId?.applyValue({ args0 -> args0 }))
.configurationProfileId(configurationProfileId?.applyValue({ args0 -> args0 }))
.content(content?.applyValue({ args0 -> args0 }))
.contentType(contentType?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.latestVersionNumber(latestVersionNumber?.applyValue({ args0 -> args0 }))
.versionLabel(versionLabel?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [HostedConfigurationVersionArgs].
*/
@PulumiTagMarker
public class HostedConfigurationVersionArgsBuilder internal constructor() {
private var applicationId: Output? = null
private var configurationProfileId: Output? = null
private var content: Output? = null
private var contentType: Output? = null
private var description: Output? = null
private var latestVersionNumber: Output? = null
private var versionLabel: Output? = null
/**
* @param value The application ID.
*/
@JvmName("vegjpidhhajtfdbl")
public suspend fun applicationId(`value`: Output) {
this.applicationId = value
}
/**
* @param value The configuration profile ID.
*/
@JvmName("jgtkcrklidlxfefb")
public suspend fun configurationProfileId(`value`: Output) {
this.configurationProfileId = value
}
/**
* @param value The content of the configuration or the configuration data.
*/
@JvmName("cbboqlsiiojlaimh")
public suspend fun content(`value`: Output) {
this.content = value
}
/**
* @param value A standard MIME type describing the format of the configuration content.
*/
@JvmName("wbhxjeqtduhercfs")
public suspend fun contentType(`value`: Output) {
this.contentType = value
}
/**
* @param value A description of the hosted configuration version.
*/
@JvmName("kshlauwvhfwatfps")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value An optional locking token used to prevent race conditions from overwriting configuration updates when creating a new version. To ensure your data is not overwritten when creating multiple hosted configuration versions in rapid succession, specify the version number of the latest hosted configuration version.
*/
@JvmName("xhwudmsfghywwrvy")
public suspend fun latestVersionNumber(`value`: Output) {
this.latestVersionNumber = value
}
/**
* @param value A user-defined label for an AWS AppConfig hosted configuration version.
*/
@JvmName("jngqhmxjdpxrotrk")
public suspend fun versionLabel(`value`: Output) {
this.versionLabel = value
}
/**
* @param value The application ID.
*/
@JvmName("rpxumvyhjiyapsls")
public suspend fun applicationId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.applicationId = mapped
}
/**
* @param value The configuration profile ID.
*/
@JvmName("xpvdhelgyxsjkshi")
public suspend fun configurationProfileId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.configurationProfileId = mapped
}
/**
* @param value The content of the configuration or the configuration data.
*/
@JvmName("ragwjamggkgtqpci")
public suspend fun content(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.content = mapped
}
/**
* @param value A standard MIME type describing the format of the configuration content.
*/
@JvmName("mbjowllsarnispwf")
public suspend fun contentType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.contentType = mapped
}
/**
* @param value A description of the hosted configuration version.
*/
@JvmName("jxadcpigfrrirnfg")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value An optional locking token used to prevent race conditions from overwriting configuration updates when creating a new version. To ensure your data is not overwritten when creating multiple hosted configuration versions in rapid succession, specify the version number of the latest hosted configuration version.
*/
@JvmName("fwmqfyrcovscruru")
public suspend fun latestVersionNumber(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.latestVersionNumber = mapped
}
/**
* @param value A user-defined label for an AWS AppConfig hosted configuration version.
*/
@JvmName("kblbnneoiotfcten")
public suspend fun versionLabel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.versionLabel = mapped
}
internal fun build(): HostedConfigurationVersionArgs = HostedConfigurationVersionArgs(
applicationId = applicationId,
configurationProfileId = configurationProfileId,
content = content,
contentType = contentType,
description = description,
latestVersionNumber = latestVersionNumber,
versionLabel = versionLabel,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy