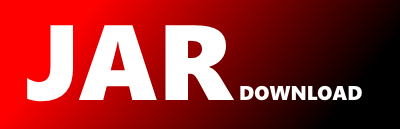
com.pulumi.awsnative.applicationautoscaling.kotlin.inputs.ScalableTargetScheduledActionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.applicationautoscaling.kotlin.inputs
import com.pulumi.awsnative.applicationautoscaling.inputs.ScalableTargetScheduledActionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* ``ScheduledAction`` is a property of the [AWS::ApplicationAutoScaling::ScalableTarget](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-applicationautoscaling-scalabletarget.html) resource that specifies a scheduled action for a scalable target.
* For more information, see [Scheduled scaling](https://docs.aws.amazon.com/autoscaling/application/userguide/application-auto-scaling-scheduled-scaling.html) in the *Application Auto Scaling User Guide*.
* @property endTime The date and time that the action is scheduled to end, in UTC.
* @property scalableTargetAction The new minimum and maximum capacity. You can set both values or just one. At the scheduled time, if the current capacity is below the minimum capacity, Application Auto Scaling scales out to the minimum capacity. If the current capacity is above the maximum capacity, Application Auto Scaling scales in to the maximum capacity.
* @property schedule The schedule for this action. The following formats are supported:
* + At expressions - "``at(yyyy-mm-ddThh:mm:ss)``"
* + Rate expressions - "``rate(value unit)``"
* + Cron expressions - "``cron(fields)``"
* At expressions are useful for one-time schedules. Cron expressions are useful for scheduled actions that run periodically at a specified date and time, and rate expressions are useful for scheduled actions that run at a regular interval.
* At and cron expressions use Universal Coordinated Time (UTC) by default.
* The cron format consists of six fields separated by white spaces: [Minutes] [Hours] [Day_of_Month] [Month] [Day_of_Week] [Year].
* For rate expressions, *value* is a positive integer and *unit* is ``minute`` | ``minutes`` | ``hour`` | ``hours`` | ``day`` | ``days``.
* @property scheduledActionName The name of the scheduled action. This name must be unique among all other scheduled actions on the specified scalable target.
* @property startTime The date and time that the action is scheduled to begin, in UTC.
* @property timezone The time zone used when referring to the date and time of a scheduled action, when the scheduled action uses an at or cron expression.
*/
public data class ScalableTargetScheduledActionArgs(
public val endTime: Output? = null,
public val scalableTargetAction: Output? = null,
public val schedule: Output,
public val scheduledActionName: Output,
public val startTime: Output? = null,
public val timezone: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.applicationautoscaling.inputs.ScalableTargetScheduledActionArgs =
com.pulumi.awsnative.applicationautoscaling.inputs.ScalableTargetScheduledActionArgs.builder()
.endTime(endTime?.applyValue({ args0 -> args0 }))
.scalableTargetAction(
scalableTargetAction?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.schedule(schedule.applyValue({ args0 -> args0 }))
.scheduledActionName(scheduledActionName.applyValue({ args0 -> args0 }))
.startTime(startTime?.applyValue({ args0 -> args0 }))
.timezone(timezone?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ScalableTargetScheduledActionArgs].
*/
@PulumiTagMarker
public class ScalableTargetScheduledActionArgsBuilder internal constructor() {
private var endTime: Output? = null
private var scalableTargetAction: Output? = null
private var schedule: Output? = null
private var scheduledActionName: Output? = null
private var startTime: Output? = null
private var timezone: Output? = null
/**
* @param value The date and time that the action is scheduled to end, in UTC.
*/
@JvmName("dpmiufkcphavegue")
public suspend fun endTime(`value`: Output) {
this.endTime = value
}
/**
* @param value The new minimum and maximum capacity. You can set both values or just one. At the scheduled time, if the current capacity is below the minimum capacity, Application Auto Scaling scales out to the minimum capacity. If the current capacity is above the maximum capacity, Application Auto Scaling scales in to the maximum capacity.
*/
@JvmName("keweapngiykqivlg")
public suspend fun scalableTargetAction(`value`: Output) {
this.scalableTargetAction = value
}
/**
* @param value The schedule for this action. The following formats are supported:
* + At expressions - "``at(yyyy-mm-ddThh:mm:ss)``"
* + Rate expressions - "``rate(value unit)``"
* + Cron expressions - "``cron(fields)``"
* At expressions are useful for one-time schedules. Cron expressions are useful for scheduled actions that run periodically at a specified date and time, and rate expressions are useful for scheduled actions that run at a regular interval.
* At and cron expressions use Universal Coordinated Time (UTC) by default.
* The cron format consists of six fields separated by white spaces: [Minutes] [Hours] [Day_of_Month] [Month] [Day_of_Week] [Year].
* For rate expressions, *value* is a positive integer and *unit* is ``minute`` | ``minutes`` | ``hour`` | ``hours`` | ``day`` | ``days``.
*/
@JvmName("qsfysqwjsmeevlmo")
public suspend fun schedule(`value`: Output) {
this.schedule = value
}
/**
* @param value The name of the scheduled action. This name must be unique among all other scheduled actions on the specified scalable target.
*/
@JvmName("hkxdapdksrjubbma")
public suspend fun scheduledActionName(`value`: Output) {
this.scheduledActionName = value
}
/**
* @param value The date and time that the action is scheduled to begin, in UTC.
*/
@JvmName("bnfplxymsfonwtum")
public suspend fun startTime(`value`: Output) {
this.startTime = value
}
/**
* @param value The time zone used when referring to the date and time of a scheduled action, when the scheduled action uses an at or cron expression.
*/
@JvmName("jdsxuffxnopseokf")
public suspend fun timezone(`value`: Output) {
this.timezone = value
}
/**
* @param value The date and time that the action is scheduled to end, in UTC.
*/
@JvmName("xuvonrmmqlvtjnkl")
public suspend fun endTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.endTime = mapped
}
/**
* @param value The new minimum and maximum capacity. You can set both values or just one. At the scheduled time, if the current capacity is below the minimum capacity, Application Auto Scaling scales out to the minimum capacity. If the current capacity is above the maximum capacity, Application Auto Scaling scales in to the maximum capacity.
*/
@JvmName("aumttjuyekfxkkxb")
public suspend fun scalableTargetAction(`value`: ScalableTargetActionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scalableTargetAction = mapped
}
/**
* @param argument The new minimum and maximum capacity. You can set both values or just one. At the scheduled time, if the current capacity is below the minimum capacity, Application Auto Scaling scales out to the minimum capacity. If the current capacity is above the maximum capacity, Application Auto Scaling scales in to the maximum capacity.
*/
@JvmName("bgtndmptcyiomtfj")
public suspend fun scalableTargetAction(argument: suspend ScalableTargetActionArgsBuilder.() -> Unit) {
val toBeMapped = ScalableTargetActionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.scalableTargetAction = mapped
}
/**
* @param value The schedule for this action. The following formats are supported:
* + At expressions - "``at(yyyy-mm-ddThh:mm:ss)``"
* + Rate expressions - "``rate(value unit)``"
* + Cron expressions - "``cron(fields)``"
* At expressions are useful for one-time schedules. Cron expressions are useful for scheduled actions that run periodically at a specified date and time, and rate expressions are useful for scheduled actions that run at a regular interval.
* At and cron expressions use Universal Coordinated Time (UTC) by default.
* The cron format consists of six fields separated by white spaces: [Minutes] [Hours] [Day_of_Month] [Month] [Day_of_Week] [Year].
* For rate expressions, *value* is a positive integer and *unit* is ``minute`` | ``minutes`` | ``hour`` | ``hours`` | ``day`` | ``days``.
*/
@JvmName("anuhorcxtimaihpj")
public suspend fun schedule(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.schedule = mapped
}
/**
* @param value The name of the scheduled action. This name must be unique among all other scheduled actions on the specified scalable target.
*/
@JvmName("gyyogrwmyenndjhr")
public suspend fun scheduledActionName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.scheduledActionName = mapped
}
/**
* @param value The date and time that the action is scheduled to begin, in UTC.
*/
@JvmName("rnwounlkobjyrjba")
public suspend fun startTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startTime = mapped
}
/**
* @param value The time zone used when referring to the date and time of a scheduled action, when the scheduled action uses an at or cron expression.
*/
@JvmName("jrgeouyrfmijjtlt")
public suspend fun timezone(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timezone = mapped
}
internal fun build(): ScalableTargetScheduledActionArgs = ScalableTargetScheduledActionArgs(
endTime = endTime,
scalableTargetAction = scalableTargetAction,
schedule = schedule ?: throw PulumiNullFieldException("schedule"),
scheduledActionName = scheduledActionName ?: throw PulumiNullFieldException("scheduledActionName"),
startTime = startTime,
timezone = timezone,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy