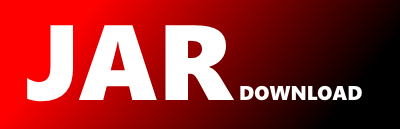
com.pulumi.awsnative.apprunner.kotlin.AutoScalingConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.apprunner.kotlin
import com.pulumi.awsnative.kotlin.outputs.CreateOnlyTag
import com.pulumi.awsnative.kotlin.outputs.CreateOnlyTag.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [AutoScalingConfiguration].
*/
@PulumiTagMarker
public class AutoScalingConfigurationResourceBuilder internal constructor() {
public var name: String? = null
public var args: AutoScalingConfigurationArgs = AutoScalingConfigurationArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AutoScalingConfigurationArgsBuilder.() -> Unit) {
val builder = AutoScalingConfigurationArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AutoScalingConfiguration {
val builtJavaResource =
com.pulumi.awsnative.apprunner.AutoScalingConfiguration(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AutoScalingConfiguration(builtJavaResource)
}
}
/**
* Describes an AWS App Runner automatic configuration resource that enables automatic scaling of instances used to process web requests. You can share an auto scaling configuration across multiple services.
*/
public class AutoScalingConfiguration internal constructor(
override val javaResource: com.pulumi.awsnative.apprunner.AutoScalingConfiguration,
) : KotlinCustomResource(javaResource, AutoScalingConfigurationMapper) {
/**
* The Amazon Resource Name (ARN) of this auto scaling configuration.
*/
public val autoScalingConfigurationArn: Output
get() = javaResource.autoScalingConfigurationArn().applyValue({ args0 -> args0 })
/**
* The customer-provided auto scaling configuration name. When you use it for the first time in an AWS Region, App Runner creates revision number 1 of this name. When you use the same name in subsequent calls, App Runner creates incremental revisions of the configuration. The auto scaling configuration name can be used in multiple revisions of a configuration.
*/
public val autoScalingConfigurationName: Output?
get() = javaResource.autoScalingConfigurationName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The revision of this auto scaling configuration. It's unique among all the active configurations ("Status": "ACTIVE") that share the same AutoScalingConfigurationName.
*/
public val autoScalingConfigurationRevision: Output
get() = javaResource.autoScalingConfigurationRevision().applyValue({ args0 -> args0 })
/**
* It's set to true for the configuration with the highest Revision among all configurations that share the same AutoScalingConfigurationName. It's set to false otherwise. App Runner temporarily doubles the number of provisioned instances during deployments, to maintain the same capacity for both old and new code.
*/
public val latest: Output
get() = javaResource.latest().applyValue({ args0 -> args0 })
/**
* The maximum number of concurrent requests that an instance processes. If the number of concurrent requests exceeds this limit, App Runner scales the service up to use more instances to process the requests.
*/
public val maxConcurrency: Output?
get() = javaResource.maxConcurrency().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The maximum number of instances that an App Runner service scales up to. At most MaxSize instances actively serve traffic for your service.
*/
public val maxSize: Output?
get() = javaResource.maxSize().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The minimum number of instances that App Runner provisions for a service. The service always has at least MinSize provisioned instances. Some of them actively serve traffic. The rest of them (provisioned and inactive instances) are a cost-effective compute capacity reserve and are ready to be quickly activated. You pay for memory usage of all the provisioned instances. You pay for CPU usage of only the active subset.
*/
public val minSize: Output?
get() = javaResource.minSize().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* A list of metadata items that you can associate with your auto scaling configuration resource. A tag is a key-value pair.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> toKotlin(args0) })
})
}).orElse(null)
})
}
public object AutoScalingConfigurationMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.apprunner.AutoScalingConfiguration::class == javaResource::class
override fun map(javaResource: Resource): AutoScalingConfiguration =
AutoScalingConfiguration(
javaResource as
com.pulumi.awsnative.apprunner.AutoScalingConfiguration,
)
}
/**
* @see [AutoScalingConfiguration].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AutoScalingConfiguration].
*/
public suspend fun autoScalingConfiguration(
name: String,
block: suspend AutoScalingConfigurationResourceBuilder.() -> Unit,
): AutoScalingConfiguration {
val builder = AutoScalingConfigurationResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AutoScalingConfiguration].
* @param name The _unique_ name of the resulting resource.
*/
public fun autoScalingConfiguration(name: String): AutoScalingConfiguration {
val builder = AutoScalingConfigurationResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy