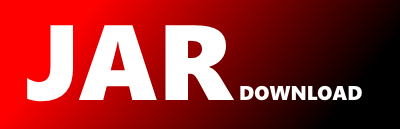
com.pulumi.awsnative.autoscaling.kotlin.inputs.ScalingPolicyPredictiveScalingPredefinedMetricPairArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.autoscaling.kotlin.inputs
import com.pulumi.awsnative.autoscaling.inputs.ScalingPolicyPredictiveScalingPredefinedMetricPairArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property predefinedMetricType Indicates which metrics to use. There are two different types of metrics for each metric type: one is a load metric and one is a scaling metric. For example, if the metric type is `ASGCPUUtilization` , the Auto Scaling group's total CPU metric is used as the load metric, and the average CPU metric is used for the scaling metric.
* @property resourceLabel A label that uniquely identifies a specific Application Load Balancer target group from which to determine the total and average request count served by your Auto Scaling group. You can't specify a resource label unless the target group is attached to the Auto Scaling group.
* You create the resource label by appending the final portion of the load balancer ARN and the final portion of the target group ARN into a single value, separated by a forward slash (/). The format of the resource label is:
* `app/my-alb/778d41231b141a0f/targetgroup/my-alb-target-group/943f017f100becff` .
* Where:
* - app// is the final portion of the load balancer ARN
* - targetgroup// is the final portion of the target group ARN.
* To find the ARN for an Application Load Balancer, use the [DescribeLoadBalancers](https://docs.aws.amazon.com/elasticloadbalancing/latest/APIReference/API_DescribeLoadBalancers.html) API operation. To find the ARN for the target group, use the [DescribeTargetGroups](https://docs.aws.amazon.com/elasticloadbalancing/latest/APIReference/API_DescribeTargetGroups.html) API operation.
*/
public data class ScalingPolicyPredictiveScalingPredefinedMetricPairArgs(
public val predefinedMetricType: Output,
public val resourceLabel: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.autoscaling.inputs.ScalingPolicyPredictiveScalingPredefinedMetricPairArgs =
com.pulumi.awsnative.autoscaling.inputs.ScalingPolicyPredictiveScalingPredefinedMetricPairArgs.builder()
.predefinedMetricType(predefinedMetricType.applyValue({ args0 -> args0 }))
.resourceLabel(resourceLabel?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ScalingPolicyPredictiveScalingPredefinedMetricPairArgs].
*/
@PulumiTagMarker
public class ScalingPolicyPredictiveScalingPredefinedMetricPairArgsBuilder internal constructor() {
private var predefinedMetricType: Output? = null
private var resourceLabel: Output? = null
/**
* @param value Indicates which metrics to use. There are two different types of metrics for each metric type: one is a load metric and one is a scaling metric. For example, if the metric type is `ASGCPUUtilization` , the Auto Scaling group's total CPU metric is used as the load metric, and the average CPU metric is used for the scaling metric.
*/
@JvmName("lmujmskwnhlmcmpm")
public suspend fun predefinedMetricType(`value`: Output) {
this.predefinedMetricType = value
}
/**
* @param value A label that uniquely identifies a specific Application Load Balancer target group from which to determine the total and average request count served by your Auto Scaling group. You can't specify a resource label unless the target group is attached to the Auto Scaling group.
* You create the resource label by appending the final portion of the load balancer ARN and the final portion of the target group ARN into a single value, separated by a forward slash (/). The format of the resource label is:
* `app/my-alb/778d41231b141a0f/targetgroup/my-alb-target-group/943f017f100becff` .
* Where:
* - app// is the final portion of the load balancer ARN
* - targetgroup// is the final portion of the target group ARN.
* To find the ARN for an Application Load Balancer, use the [DescribeLoadBalancers](https://docs.aws.amazon.com/elasticloadbalancing/latest/APIReference/API_DescribeLoadBalancers.html) API operation. To find the ARN for the target group, use the [DescribeTargetGroups](https://docs.aws.amazon.com/elasticloadbalancing/latest/APIReference/API_DescribeTargetGroups.html) API operation.
*/
@JvmName("dsxffefiadcbaojl")
public suspend fun resourceLabel(`value`: Output) {
this.resourceLabel = value
}
/**
* @param value Indicates which metrics to use. There are two different types of metrics for each metric type: one is a load metric and one is a scaling metric. For example, if the metric type is `ASGCPUUtilization` , the Auto Scaling group's total CPU metric is used as the load metric, and the average CPU metric is used for the scaling metric.
*/
@JvmName("kwxfynqfxnmuucbk")
public suspend fun predefinedMetricType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.predefinedMetricType = mapped
}
/**
* @param value A label that uniquely identifies a specific Application Load Balancer target group from which to determine the total and average request count served by your Auto Scaling group. You can't specify a resource label unless the target group is attached to the Auto Scaling group.
* You create the resource label by appending the final portion of the load balancer ARN and the final portion of the target group ARN into a single value, separated by a forward slash (/). The format of the resource label is:
* `app/my-alb/778d41231b141a0f/targetgroup/my-alb-target-group/943f017f100becff` .
* Where:
* - app// is the final portion of the load balancer ARN
* - targetgroup// is the final portion of the target group ARN.
* To find the ARN for an Application Load Balancer, use the [DescribeLoadBalancers](https://docs.aws.amazon.com/elasticloadbalancing/latest/APIReference/API_DescribeLoadBalancers.html) API operation. To find the ARN for the target group, use the [DescribeTargetGroups](https://docs.aws.amazon.com/elasticloadbalancing/latest/APIReference/API_DescribeTargetGroups.html) API operation.
*/
@JvmName("eqmdcnfohfqtluqp")
public suspend fun resourceLabel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceLabel = mapped
}
internal fun build(): ScalingPolicyPredictiveScalingPredefinedMetricPairArgs =
ScalingPolicyPredictiveScalingPredefinedMetricPairArgs(
predefinedMetricType = predefinedMetricType ?: throw
PulumiNullFieldException("predefinedMetricType"),
resourceLabel = resourceLabel,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy