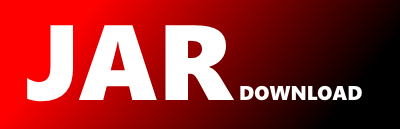
com.pulumi.awsnative.autoscaling.kotlin.inputs.ScalingPolicyTargetTrackingConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.autoscaling.kotlin.inputs
import com.pulumi.awsnative.autoscaling.inputs.ScalingPolicyTargetTrackingConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Double
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property customizedMetricSpecification A customized metric. You must specify either a predefined metric or a customized metric.
* @property disableScaleIn Indicates whether scaling in by the target tracking scaling policy is disabled. If scaling in is disabled, the target tracking scaling policy doesn't remove instances from the Auto Scaling group. Otherwise, the target tracking scaling policy can remove instances from the Auto Scaling group. The default is `false` .
* @property predefinedMetricSpecification A predefined metric. You must specify either a predefined metric or a customized metric.
* @property targetValue The target value for the metric.
* > Some metrics are based on a count instead of a percentage, such as the request count for an Application Load Balancer or the number of messages in an SQS queue. If the scaling policy specifies one of these metrics, specify the target utilization as the optimal average request or message count per instance during any one-minute interval.
*/
public data class ScalingPolicyTargetTrackingConfigurationArgs(
public val customizedMetricSpecification: Output? =
null,
public val disableScaleIn: Output? = null,
public val predefinedMetricSpecification: Output? =
null,
public val targetValue: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.autoscaling.inputs.ScalingPolicyTargetTrackingConfigurationArgs =
com.pulumi.awsnative.autoscaling.inputs.ScalingPolicyTargetTrackingConfigurationArgs.builder()
.customizedMetricSpecification(
customizedMetricSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.disableScaleIn(disableScaleIn?.applyValue({ args0 -> args0 }))
.predefinedMetricSpecification(
predefinedMetricSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.targetValue(targetValue.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ScalingPolicyTargetTrackingConfigurationArgs].
*/
@PulumiTagMarker
public class ScalingPolicyTargetTrackingConfigurationArgsBuilder internal constructor() {
private var customizedMetricSpecification: Output? =
null
private var disableScaleIn: Output? = null
private var predefinedMetricSpecification: Output? =
null
private var targetValue: Output? = null
/**
* @param value A customized metric. You must specify either a predefined metric or a customized metric.
*/
@JvmName("trgrenvcosyxcpqo")
public suspend fun customizedMetricSpecification(`value`: Output) {
this.customizedMetricSpecification = value
}
/**
* @param value Indicates whether scaling in by the target tracking scaling policy is disabled. If scaling in is disabled, the target tracking scaling policy doesn't remove instances from the Auto Scaling group. Otherwise, the target tracking scaling policy can remove instances from the Auto Scaling group. The default is `false` .
*/
@JvmName("ljrrnuwvsmhkuoth")
public suspend fun disableScaleIn(`value`: Output) {
this.disableScaleIn = value
}
/**
* @param value A predefined metric. You must specify either a predefined metric or a customized metric.
*/
@JvmName("tsacsxhvlmldwtmq")
public suspend fun predefinedMetricSpecification(`value`: Output) {
this.predefinedMetricSpecification = value
}
/**
* @param value The target value for the metric.
* > Some metrics are based on a count instead of a percentage, such as the request count for an Application Load Balancer or the number of messages in an SQS queue. If the scaling policy specifies one of these metrics, specify the target utilization as the optimal average request or message count per instance during any one-minute interval.
*/
@JvmName("loixhvqipqpnopmm")
public suspend fun targetValue(`value`: Output) {
this.targetValue = value
}
/**
* @param value A customized metric. You must specify either a predefined metric or a customized metric.
*/
@JvmName("cdxlldbhuswhdqel")
public suspend fun customizedMetricSpecification(`value`: ScalingPolicyCustomizedMetricSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customizedMetricSpecification = mapped
}
/**
* @param argument A customized metric. You must specify either a predefined metric or a customized metric.
*/
@JvmName("mudlhhvubphdjliq")
public suspend fun customizedMetricSpecification(argument: suspend ScalingPolicyCustomizedMetricSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = ScalingPolicyCustomizedMetricSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.customizedMetricSpecification = mapped
}
/**
* @param value Indicates whether scaling in by the target tracking scaling policy is disabled. If scaling in is disabled, the target tracking scaling policy doesn't remove instances from the Auto Scaling group. Otherwise, the target tracking scaling policy can remove instances from the Auto Scaling group. The default is `false` .
*/
@JvmName("jwreyaitcuimbmxp")
public suspend fun disableScaleIn(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableScaleIn = mapped
}
/**
* @param value A predefined metric. You must specify either a predefined metric or a customized metric.
*/
@JvmName("ajcjkjkaphtqiakl")
public suspend fun predefinedMetricSpecification(`value`: ScalingPolicyPredefinedMetricSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.predefinedMetricSpecification = mapped
}
/**
* @param argument A predefined metric. You must specify either a predefined metric or a customized metric.
*/
@JvmName("qhcnaqvvqcrdcvej")
public suspend fun predefinedMetricSpecification(argument: suspend ScalingPolicyPredefinedMetricSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = ScalingPolicyPredefinedMetricSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.predefinedMetricSpecification = mapped
}
/**
* @param value The target value for the metric.
* > Some metrics are based on a count instead of a percentage, such as the request count for an Application Load Balancer or the number of messages in an SQS queue. If the scaling policy specifies one of these metrics, specify the target utilization as the optimal average request or message count per instance during any one-minute interval.
*/
@JvmName("apivauqhjolboxag")
public suspend fun targetValue(`value`: Double) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.targetValue = mapped
}
internal fun build(): ScalingPolicyTargetTrackingConfigurationArgs =
ScalingPolicyTargetTrackingConfigurationArgs(
customizedMetricSpecification = customizedMetricSpecification,
disableScaleIn = disableScaleIn,
predefinedMetricSpecification = predefinedMetricSpecification,
targetValue = targetValue ?: throw PulumiNullFieldException("targetValue"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy