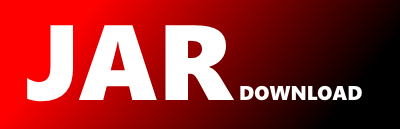
com.pulumi.awsnative.bedrock.kotlin.FlowArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.bedrock.kotlin
import com.pulumi.awsnative.bedrock.FlowArgs.builder
import com.pulumi.awsnative.bedrock.kotlin.inputs.FlowDefinitionArgs
import com.pulumi.awsnative.bedrock.kotlin.inputs.FlowDefinitionArgsBuilder
import com.pulumi.awsnative.bedrock.kotlin.inputs.FlowS3LocationArgs
import com.pulumi.awsnative.bedrock.kotlin.inputs.FlowS3LocationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Definition of AWS::Bedrock::Flow Resource Type
* @property customerEncryptionKeyArn A KMS key ARN
* @property definition
* @property definitionS3Location An Amazon S3 location.
* @property definitionString A JSON string containing a Definition with the same schema as the Definition property of this resource
* @property definitionSubstitutions
* @property description Description of the flow
* @property executionRoleArn ARN of a IAM role
* @property name Name for the flow
* @property tags
* @property testAliasTags
*/
public data class FlowArgs(
public val customerEncryptionKeyArn: Output? = null,
public val definition: Output? = null,
public val definitionS3Location: Output? = null,
public val definitionString: Output? = null,
public val definitionSubstitutions: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy