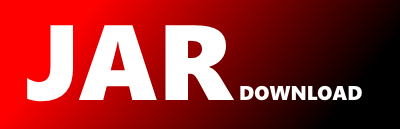
com.pulumi.awsnative.bedrock.kotlin.inputs.PromptVariantArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.bedrock.kotlin.inputs
import com.pulumi.awsnative.bedrock.inputs.PromptVariantArgs.builder
import com.pulumi.awsnative.bedrock.kotlin.enums.PromptTemplateType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Prompt variant
* @property inferenceConfiguration Contains inference configurations for the prompt variant.
* @property modelId ARN or name of a Bedrock model.
* @property name Name for a variant.
* @property templateConfiguration Contains configurations for the prompt template.
* @property templateType The type of prompt template to use.
*/
public data class PromptVariantArgs(
public val inferenceConfiguration: Output? = null,
public val modelId: Output? = null,
public val name: Output,
public val templateConfiguration: Output? = null,
public val templateType: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.bedrock.inputs.PromptVariantArgs =
com.pulumi.awsnative.bedrock.inputs.PromptVariantArgs.builder()
.inferenceConfiguration(
inferenceConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.modelId(modelId?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.templateConfiguration(
templateConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.templateType(templateType.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [PromptVariantArgs].
*/
@PulumiTagMarker
public class PromptVariantArgsBuilder internal constructor() {
private var inferenceConfiguration: Output? = null
private var modelId: Output? = null
private var name: Output? = null
private var templateConfiguration: Output? = null
private var templateType: Output? = null
/**
* @param value Contains inference configurations for the prompt variant.
*/
@JvmName("kiafmnqalenhrdyj")
public suspend fun inferenceConfiguration(`value`: Output) {
this.inferenceConfiguration = value
}
/**
* @param value ARN or name of a Bedrock model.
*/
@JvmName("waqvadqgpmijqhyw")
public suspend fun modelId(`value`: Output) {
this.modelId = value
}
/**
* @param value Name for a variant.
*/
@JvmName("megxnatgbegathbl")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Contains configurations for the prompt template.
*/
@JvmName("iimbnvtperxwrodd")
public suspend fun templateConfiguration(`value`: Output) {
this.templateConfiguration = value
}
/**
* @param value The type of prompt template to use.
*/
@JvmName("nqskrvsxwbporpvo")
public suspend fun templateType(`value`: Output) {
this.templateType = value
}
/**
* @param value Contains inference configurations for the prompt variant.
*/
@JvmName("gyoivvjokxgyutgu")
public suspend fun inferenceConfiguration(`value`: PromptInferenceConfigurationPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inferenceConfiguration = mapped
}
/**
* @param argument Contains inference configurations for the prompt variant.
*/
@JvmName("aeboolkrcvklsyao")
public suspend fun inferenceConfiguration(argument: suspend PromptInferenceConfigurationPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = PromptInferenceConfigurationPropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.inferenceConfiguration = mapped
}
/**
* @param value ARN or name of a Bedrock model.
*/
@JvmName("fihabomrodvypfmh")
public suspend fun modelId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.modelId = mapped
}
/**
* @param value Name for a variant.
*/
@JvmName("jcbjidnrbfbqadvi")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Contains configurations for the prompt template.
*/
@JvmName("pgraxtqfodylymws")
public suspend fun templateConfiguration(`value`: PromptTemplateConfigurationPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.templateConfiguration = mapped
}
/**
* @param argument Contains configurations for the prompt template.
*/
@JvmName("ghnaowyivalnquxp")
public suspend fun templateConfiguration(argument: suspend PromptTemplateConfigurationPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = PromptTemplateConfigurationPropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.templateConfiguration = mapped
}
/**
* @param value The type of prompt template to use.
*/
@JvmName("iojtlhoaijigthge")
public suspend fun templateType(`value`: PromptTemplateType) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.templateType = mapped
}
internal fun build(): PromptVariantArgs = PromptVariantArgs(
inferenceConfiguration = inferenceConfiguration,
modelId = modelId,
name = name ?: throw PulumiNullFieldException("name"),
templateConfiguration = templateConfiguration,
templateType = templateType ?: throw PulumiNullFieldException("templateType"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy