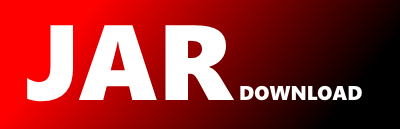
com.pulumi.awsnative.cloudformation.kotlin.ResourceVersionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.cloudformation.kotlin
import com.pulumi.awsnative.cloudformation.ResourceVersionArgs.builder
import com.pulumi.awsnative.cloudformation.kotlin.inputs.ResourceVersionLoggingConfigArgs
import com.pulumi.awsnative.cloudformation.kotlin.inputs.ResourceVersionLoggingConfigArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* A resource that has been registered in the CloudFormation Registry.
* ## Example Usage
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* @property executionRoleArn The Amazon Resource Name (ARN) of the IAM execution role to use to register the type. If your resource type calls AWS APIs in any of its handlers, you must create an IAM execution role that includes the necessary permissions to call those AWS APIs, and provision that execution role in your account. CloudFormation then assumes that execution role to provide your resource type with the appropriate credentials.
* @property loggingConfig Specifies logging configuration information for a type.
* @property schemaHandlerPackage A url to the S3 bucket containing the schema handler package that contains the schema, event handlers, and associated files for the type you want to register.
* For information on generating a schema handler package for the type you want to register, see submit in the CloudFormation CLI User Guide.
* @property typeName The name of the type being registered.
* We recommend that type names adhere to the following pattern: company_or_organization::service::type.
*/
public data class ResourceVersionArgs(
public val executionRoleArn: Output? = null,
public val loggingConfig: Output? = null,
public val schemaHandlerPackage: Output? = null,
public val typeName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.cloudformation.ResourceVersionArgs =
com.pulumi.awsnative.cloudformation.ResourceVersionArgs.builder()
.executionRoleArn(executionRoleArn?.applyValue({ args0 -> args0 }))
.loggingConfig(loggingConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.schemaHandlerPackage(schemaHandlerPackage?.applyValue({ args0 -> args0 }))
.typeName(typeName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ResourceVersionArgs].
*/
@PulumiTagMarker
public class ResourceVersionArgsBuilder internal constructor() {
private var executionRoleArn: Output? = null
private var loggingConfig: Output? = null
private var schemaHandlerPackage: Output? = null
private var typeName: Output? = null
/**
* @param value The Amazon Resource Name (ARN) of the IAM execution role to use to register the type. If your resource type calls AWS APIs in any of its handlers, you must create an IAM execution role that includes the necessary permissions to call those AWS APIs, and provision that execution role in your account. CloudFormation then assumes that execution role to provide your resource type with the appropriate credentials.
*/
@JvmName("paodvnqewlsrnmst")
public suspend fun executionRoleArn(`value`: Output) {
this.executionRoleArn = value
}
/**
* @param value Specifies logging configuration information for a type.
*/
@JvmName("txtrhlawunrejayo")
public suspend fun loggingConfig(`value`: Output) {
this.loggingConfig = value
}
/**
* @param value A url to the S3 bucket containing the schema handler package that contains the schema, event handlers, and associated files for the type you want to register.
* For information on generating a schema handler package for the type you want to register, see submit in the CloudFormation CLI User Guide.
*/
@JvmName("pgnubuxlxkaciybe")
public suspend fun schemaHandlerPackage(`value`: Output) {
this.schemaHandlerPackage = value
}
/**
* @param value The name of the type being registered.
* We recommend that type names adhere to the following pattern: company_or_organization::service::type.
*/
@JvmName("awdyfghpbmamtmpx")
public suspend fun typeName(`value`: Output) {
this.typeName = value
}
/**
* @param value The Amazon Resource Name (ARN) of the IAM execution role to use to register the type. If your resource type calls AWS APIs in any of its handlers, you must create an IAM execution role that includes the necessary permissions to call those AWS APIs, and provision that execution role in your account. CloudFormation then assumes that execution role to provide your resource type with the appropriate credentials.
*/
@JvmName("rgycklmbxbmmxhhd")
public suspend fun executionRoleArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.executionRoleArn = mapped
}
/**
* @param value Specifies logging configuration information for a type.
*/
@JvmName("ebtsafanoawtdjpq")
public suspend fun loggingConfig(`value`: ResourceVersionLoggingConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.loggingConfig = mapped
}
/**
* @param argument Specifies logging configuration information for a type.
*/
@JvmName("gjylubgdpdlhvyku")
public suspend fun loggingConfig(argument: suspend ResourceVersionLoggingConfigArgsBuilder.() -> Unit) {
val toBeMapped = ResourceVersionLoggingConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.loggingConfig = mapped
}
/**
* @param value A url to the S3 bucket containing the schema handler package that contains the schema, event handlers, and associated files for the type you want to register.
* For information on generating a schema handler package for the type you want to register, see submit in the CloudFormation CLI User Guide.
*/
@JvmName("vngagypydmmidsih")
public suspend fun schemaHandlerPackage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.schemaHandlerPackage = mapped
}
/**
* @param value The name of the type being registered.
* We recommend that type names adhere to the following pattern: company_or_organization::service::type.
*/
@JvmName("fbnimifseteajoml")
public suspend fun typeName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.typeName = mapped
}
internal fun build(): ResourceVersionArgs = ResourceVersionArgs(
executionRoleArn = executionRoleArn,
loggingConfig = loggingConfig,
schemaHandlerPackage = schemaHandlerPackage,
typeName = typeName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy