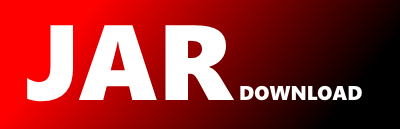
com.pulumi.awsnative.codedeploy.kotlin.DeploymentConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.codedeploy.kotlin
import com.pulumi.awsnative.codedeploy.DeploymentConfigArgs.builder
import com.pulumi.awsnative.codedeploy.kotlin.inputs.DeploymentConfigMinimumHealthyHostsArgs
import com.pulumi.awsnative.codedeploy.kotlin.inputs.DeploymentConfigMinimumHealthyHostsArgsBuilder
import com.pulumi.awsnative.codedeploy.kotlin.inputs.DeploymentConfigTrafficRoutingConfigArgs
import com.pulumi.awsnative.codedeploy.kotlin.inputs.DeploymentConfigTrafficRoutingConfigArgsBuilder
import com.pulumi.awsnative.codedeploy.kotlin.inputs.DeploymentConfigZonalConfigArgs
import com.pulumi.awsnative.codedeploy.kotlin.inputs.DeploymentConfigZonalConfigArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::CodeDeploy::DeploymentConfig
* @property computePlatform The destination platform type for the deployment (Lambda, Server, or ECS).
* @property deploymentConfigName A name for the deployment configuration. If you don't specify a name, AWS CloudFormation generates a unique physical ID and uses that ID for the deployment configuration name. For more information, see Name Type.
* @property minimumHealthyHosts The minimum number of healthy instances that should be available at any time during the deployment. There are two parameters expected in the input: type and value.
* @property trafficRoutingConfig The configuration that specifies how the deployment traffic is routed.
* @property zonalConfig The zonal deployment config that specifies how the zonal deployment behaves
*/
public data class DeploymentConfigArgs(
public val computePlatform: Output? = null,
public val deploymentConfigName: Output? = null,
public val minimumHealthyHosts: Output? = null,
public val trafficRoutingConfig: Output? = null,
public val zonalConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.codedeploy.DeploymentConfigArgs =
com.pulumi.awsnative.codedeploy.DeploymentConfigArgs.builder()
.computePlatform(computePlatform?.applyValue({ args0 -> args0 }))
.deploymentConfigName(deploymentConfigName?.applyValue({ args0 -> args0 }))
.minimumHealthyHosts(
minimumHealthyHosts?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.trafficRoutingConfig(
trafficRoutingConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.zonalConfig(zonalConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [DeploymentConfigArgs].
*/
@PulumiTagMarker
public class DeploymentConfigArgsBuilder internal constructor() {
private var computePlatform: Output? = null
private var deploymentConfigName: Output? = null
private var minimumHealthyHosts: Output? = null
private var trafficRoutingConfig: Output? = null
private var zonalConfig: Output? = null
/**
* @param value The destination platform type for the deployment (Lambda, Server, or ECS).
*/
@JvmName("ljevwnyqmpcqjkbc")
public suspend fun computePlatform(`value`: Output) {
this.computePlatform = value
}
/**
* @param value A name for the deployment configuration. If you don't specify a name, AWS CloudFormation generates a unique physical ID and uses that ID for the deployment configuration name. For more information, see Name Type.
*/
@JvmName("oyxqrgtioivefoqa")
public suspend fun deploymentConfigName(`value`: Output) {
this.deploymentConfigName = value
}
/**
* @param value The minimum number of healthy instances that should be available at any time during the deployment. There are two parameters expected in the input: type and value.
*/
@JvmName("vxgtehbrgmbmoymi")
public suspend fun minimumHealthyHosts(`value`: Output) {
this.minimumHealthyHosts = value
}
/**
* @param value The configuration that specifies how the deployment traffic is routed.
*/
@JvmName("ouegqekjhvsvahpc")
public suspend fun trafficRoutingConfig(`value`: Output) {
this.trafficRoutingConfig = value
}
/**
* @param value The zonal deployment config that specifies how the zonal deployment behaves
*/
@JvmName("ayhgsgwvwcwnyfbl")
public suspend fun zonalConfig(`value`: Output) {
this.zonalConfig = value
}
/**
* @param value The destination platform type for the deployment (Lambda, Server, or ECS).
*/
@JvmName("cdiwmxibolkhhjkk")
public suspend fun computePlatform(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.computePlatform = mapped
}
/**
* @param value A name for the deployment configuration. If you don't specify a name, AWS CloudFormation generates a unique physical ID and uses that ID for the deployment configuration name. For more information, see Name Type.
*/
@JvmName("jnbobrmofiftibtv")
public suspend fun deploymentConfigName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deploymentConfigName = mapped
}
/**
* @param value The minimum number of healthy instances that should be available at any time during the deployment. There are two parameters expected in the input: type and value.
*/
@JvmName("ugsqllaaucysonvh")
public suspend fun minimumHealthyHosts(`value`: DeploymentConfigMinimumHealthyHostsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minimumHealthyHosts = mapped
}
/**
* @param argument The minimum number of healthy instances that should be available at any time during the deployment. There are two parameters expected in the input: type and value.
*/
@JvmName("sxwyntiyofgmriql")
public suspend fun minimumHealthyHosts(argument: suspend DeploymentConfigMinimumHealthyHostsArgsBuilder.() -> Unit) {
val toBeMapped = DeploymentConfigMinimumHealthyHostsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.minimumHealthyHosts = mapped
}
/**
* @param value The configuration that specifies how the deployment traffic is routed.
*/
@JvmName("xiaxuifujhwrgqeq")
public suspend fun trafficRoutingConfig(`value`: DeploymentConfigTrafficRoutingConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.trafficRoutingConfig = mapped
}
/**
* @param argument The configuration that specifies how the deployment traffic is routed.
*/
@JvmName("gvvxemydsrkikmpu")
public suspend fun trafficRoutingConfig(argument: suspend DeploymentConfigTrafficRoutingConfigArgsBuilder.() -> Unit) {
val toBeMapped = DeploymentConfigTrafficRoutingConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.trafficRoutingConfig = mapped
}
/**
* @param value The zonal deployment config that specifies how the zonal deployment behaves
*/
@JvmName("mmswcddirtiilies")
public suspend fun zonalConfig(`value`: DeploymentConfigZonalConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.zonalConfig = mapped
}
/**
* @param argument The zonal deployment config that specifies how the zonal deployment behaves
*/
@JvmName("sukjlhuuvsvbmvew")
public suspend fun zonalConfig(argument: suspend DeploymentConfigZonalConfigArgsBuilder.() -> Unit) {
val toBeMapped = DeploymentConfigZonalConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.zonalConfig = mapped
}
internal fun build(): DeploymentConfigArgs = DeploymentConfigArgs(
computePlatform = computePlatform,
deploymentConfigName = deploymentConfigName,
minimumHealthyHosts = minimumHealthyHosts,
trafficRoutingConfig = trafficRoutingConfig,
zonalConfig = zonalConfig,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy